How Can You Write JSON to a File Effectively?
In the digital age, data is the lifeblood of applications, systems, and services. As we navigate through various programming tasks, the need to store and manage data efficiently becomes paramount. One of the most popular formats for data interchange is JSON (JavaScript Object Notation), prized for its simplicity and readability. Whether you’re a seasoned developer or just starting your coding journey, understanding how to write JSON to a file is an essential skill that can enhance your data management capabilities and streamline your applications.
Writing JSON to a file involves not just the technical aspects of file handling but also an appreciation for the structure and format of JSON itself. This lightweight data format, which is easy for humans to read and write, and easy for machines to parse and generate, is widely used in web APIs and configuration files. By mastering the process of writing JSON to a file, you can effectively store complex data structures, making it easier to retrieve and manipulate them later.
In this article, we will explore the various methods and best practices for writing JSON data to a file across different programming languages. From understanding the nuances of JSON formatting to implementing file I/O operations, we will provide you with the tools and knowledge necessary to handle JSON data with confidence. Get ready to dive into the world of JSON and unlock
Choosing the Right Format for JSON Data
When writing JSON to a file, it is essential to ensure that the data structure adheres to JSON standards. JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. The primary requirement is that the data must be correctly formatted, including:
- Data types: Strings, numbers, arrays, objects, booleans, and null.
- Proper use of quotes: String values must be enclosed in double quotes.
- Commas: Elements within arrays and properties within objects should be separated by commas.
To illustrate the JSON format, consider the following example:
“`json
{
“name”: “John Doe”,
“age”: 30,
“isEmployed”: true,
“skills”: [“JavaScript”, “Python”, “Java”],
“address”: {
“street”: “123 Main St”,
“city”: “Anytown”,
“zip”: “12345”
}
}
“`
This example showcases various data types, including nested objects and arrays, which are common in JSON data.
Writing JSON to a File in Different Programming Languages
Various programming languages provide libraries to facilitate writing JSON data to files. Below are examples in Python, JavaScript, and Java.
Language | Code Example |
---|---|
Python |
“`python import json data = { with open(‘data.json’, ‘w’) as json_file: |
JavaScript |
“`javascript const fs = require(‘fs’); const data = { fs.writeFileSync(‘data.json’, JSON.stringify(data, null, 2)); |
Java |
“`java import org.json.JSONObject; import java.nio.file.Files; import java.nio.file.Paths; JSONObject data = new JSONObject(); Files.write(Paths.get(“data.json”), data.toString(2).getBytes()); |
Each code snippet demonstrates how to serialize a data structure into JSON format and write it to a file. It is crucial to handle file operations carefully, ensuring that the file is properly opened and closed to avoid data corruption.
Error Handling During File Writing
When writing JSON data to a file, it is important to implement error handling to manage potential issues that may arise during file operations. Common errors include:
- File permission issues: The program may not have the necessary permissions to write to the specified directory.
- Disk space limitations: Insufficient disk space can lead to incomplete writes.
- Data serialization errors: Issues may occur if the data contains non-serializable objects.
Implementing error handling can be achieved using try-catch blocks or equivalent structures in various programming languages. For instance, in Python, the following code demonstrates basic error handling:
“`python
try:
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file)
except IOError as e:
print(f”An IOError occurred: {e}”)
except Exception as e:
print(f”An unexpected error occurred: {e}”)
“`
Incorporating these practices ensures that the JSON writing process is robust and reliable.
Writing JSON to a File in Various Programming Languages
Writing JSON data to a file can differ based on the programming language being utilized. Below are examples in several popular languages, illustrating how to efficiently perform this operation.
Python
In Python, you can use the built-in `json` module to write JSON data to a file. The following steps outline the process:
“`python
import json
data = {
“name”: “John Doe”,
“age”: 30,
“city”: “New York”
}
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file, indent=4)
“`
Key Points:
- Use `json.dump()` to write JSON data directly to a file.
- The `indent` parameter is optional and makes the output more readable.
JavaScript (Node.js)
In Node.js, the built-in `fs` module allows you to interact with the filesystem. Here’s how to write JSON data to a file:
“`javascript
const fs = require(‘fs’);
const data = {
name: “John Doe”,
age: 30,
city: “New York”
};
fs.writeFile(‘data.json’, JSON.stringify(data, null, 4), (err) => {
if (err) throw err;
console.log(‘Data written to file’);
});
“`
Key Points:
- Use `JSON.stringify()` to convert the JavaScript object to a JSON string.
- The second parameter of `JSON.stringify()` specifies the indentation for readability.
Java
In Java, you can use libraries like Jackson or Gson to write JSON data to a file. Here’s an example using Jackson:
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
public class WriteJson {
public static void main(String[] args) throws Exception {
ObjectMapper mapper = new ObjectMapper();
Person person = new Person(“John Doe”, 30, “New York”);
mapper.writeValue(new File(“data.json”), person);
}
}
class Person {
public String name;
public int age;
public String city;
public Person(String name, int age, String city) {
this.name = name;
this.age = age;
this.city = city;
}
}
“`
Key Points:
- The `ObjectMapper` class from the Jackson library is used for converting objects to JSON.
- Make sure to handle exceptions appropriately in production code.
C
In C, you can utilize the `Newtonsoft.Json` library, also known as Json.NET, to write JSON data to a file. Here’s a simple example:
“`csharp
using Newtonsoft.Json;
using System.IO;
public class Program
{
public static void Main()
{
var person = new Person { Name = “John Doe”, Age = 30, City = “New York” };
File.WriteAllText(“data.json”, JsonConvert.SerializeObject(person, Formatting.Indented));
}
}
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public string City { get; set; }
}
“`
Key Points:
- Use `JsonConvert.SerializeObject()` to convert an object to a JSON string.
- The `Formatting.Indented` option enhances readability.
PHP
In PHP, the `json_encode()` function converts an array or object into a JSON format, which can then be written to a file using `file_put_contents()`:
“`php
$data = [
“name” => “John Doe”,
“age” => 30,
“city” => “New York”
];
file_put_contents(‘data.json’, json_encode($data, JSON_PRETTY_PRINT));
“`
Key Points:
- `JSON_PRETTY_PRINT` formats the JSON output for improved readability.
- Ensure proper error handling in a production environment.
Summary of Key Functions
Language | Function/Method | Description |
---|---|---|
Python | `json.dump()` | Writes JSON data to a file. |
JavaScript | `fs.writeFile()` | Writes data to a file in Node.js. |
Java | `ObjectMapper.writeValue()` | Serializes an object to JSON and writes to a file. |
C | `JsonConvert.SerializeObject()` | Converts an object to JSON format. |
PHP | `file_put_contents()` | Writes a string to a file. |
This table provides a quick reference for the primary functions used in each language for writing JSON data to files.
Expert Insights on Writing JSON to a File
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “Writing JSON to a file is a fundamental skill for data scientists. It allows for efficient data storage and retrieval, ensuring that structured data can be easily shared and processed across various platforms.”
Michael Torres (Software Engineer, CodeCraft Solutions). “When writing JSON to a file, it is crucial to handle exceptions properly. This ensures that any issues during the write process do not lead to data corruption, which can be detrimental in production environments.”
Linda Patel (Lead Developer, DataFlow Technologies). “Utilizing libraries specifically designed for JSON manipulation can greatly simplify the process of writing JSON to a file. These libraries often provide built-in methods that enhance performance and reduce the likelihood of errors.”
Frequently Asked Questions (FAQs)
What is the process to write JSON data to a file?
To write JSON data to a file, first convert the data structure (like a dictionary in Python) into a JSON string using a serialization method such as `json.dumps()`. Then, open a file in write mode and use the `write()` method to save the JSON string to the file.
Which programming languages support writing JSON to files?
Most modern programming languages, including Python, JavaScript, Java, C, and Ruby, support writing JSON to files. They typically provide libraries or built-in functions for JSON serialization and file handling.
Are there any libraries recommended for writing JSON to a file?
In Python, the `json` module is commonly used for writing JSON to files. In JavaScript, the `fs` module can be used in conjunction with `JSON.stringify()`. For Java, the Jackson or Gson libraries are popular choices.
What file extension is typically used for JSON files?
The standard file extension for JSON files is `.json`. This extension helps indicate the file format and ensures compatibility with various applications that read JSON data.
Can I write JSON data to a file in a pretty-printed format?
Yes, you can write JSON data in a pretty-printed format by using the `json.dump()` method with the `indent` parameter in Python. This formats the JSON output with line breaks and indentation for improved readability.
What happens if I try to write to a file that is already open?
If you attempt to write to a file that is already open in a conflicting mode (e.g., open for reading), you may encounter an error. It’s essential to ensure that the file is opened in a write-compatible mode, such as ‘w’ or ‘a’, to avoid conflicts.
Writing JSON to a file is a fundamental skill for developers working with data interchange formats. JSON, or JavaScript Object Notation, is widely used due to its lightweight nature and ease of readability, making it an ideal choice for data storage and transmission. The process typically involves converting data structures, such as arrays or objects, into a JSON string and then writing that string to a file using appropriate file handling methods in various programming languages.
One of the key insights is the importance of ensuring that the data being written adheres to the JSON format. This includes proper syntax, such as using double quotes for strings and ensuring that data types are correctly represented. Additionally, developers should be aware of the potential pitfalls, such as handling special characters and managing file permissions, which can affect the successful writing of JSON data to a file.
Moreover, utilizing libraries and built-in functions can significantly simplify the process of writing JSON to a file. Most programming environments provide robust support for JSON serialization and file operations, allowing developers to focus on the logic of their applications rather than the intricacies of data formatting. By leveraging these tools, developers can enhance efficiency and reduce the likelihood of errors in data handling.
mastering the technique of writing
Author Profile
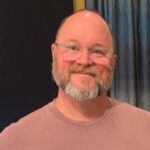
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?