Why Do We Use While Loops in JavaScript? Exploring Their Purpose and Benefits
In the world of programming, efficiency and control are paramount, and JavaScript offers a robust toolkit for developers to harness these qualities. Among these tools is the while loop, a fundamental construct that allows for the execution of a block of code as long as a specified condition remains true. But why do we use while loops in JavaScript? Understanding their purpose not only enhances your coding skills but also equips you to tackle complex problems with ease. As we delve into the intricacies of while loops, we will uncover their versatility and the scenarios in which they shine brightest.
While loops are particularly valuable when the number of iterations is not predetermined, providing a dynamic way to handle repetitive tasks. This flexibility allows developers to create more responsive and adaptable code, making it easier to manage situations where the loop’s termination condition is reliant on external factors or user input. Additionally, while loops can simplify code readability by reducing the need for cumbersome counters or multiple conditional checks, ultimately leading to cleaner and more maintainable scripts.
As we explore the mechanics and applications of while loops in JavaScript, you’ll discover how they can streamline your coding process and enhance your problem-solving capabilities. From simple iterations to more complex scenarios, the power of while loops lies in their ability to execute code efficiently, making them an
Understanding the While Loop
The while loop in JavaScript is a fundamental control structure that allows developers to execute a block of code repeatedly as long as a specified condition evaluates to true. This loop provides flexibility in scenarios where the number of iterations is not known beforehand, making it particularly useful for tasks that require repetitive actions based on dynamic data.
The syntax of a while loop is straightforward:
“`javascript
while (condition) {
// code to be executed
}
“`
Here, the `condition` is evaluated before each iteration. If it returns true, the code block inside the loop executes. If it returns , the loop terminates, and execution continues with the code following the loop.
When to Use While Loops
While loops are particularly advantageous in scenarios such as:
- Dynamic Iteration: When the number of iterations is not known beforehand.
- Waiting for a Condition: When you need to wait for a specific condition to be met before proceeding.
- Processing Data: Iterating through data structures that require conditional processing.
Example of a While Loop
Consider a scenario where you need to read user input until a specific value is entered. The following example illustrates this:
“`javascript
let input;
while (input !== ‘exit’) {
input = prompt(‘Enter a value (type “exit” to quit):’);
console.log(`You entered: ${input}`);
}
“`
In this example, the loop continues to prompt the user for input until they type “exit”. This demonstrates the dynamic nature of the while loop, as the number of iterations is determined by user input.
Comparing While Loops to Other Loop Structures
While loops can be contrasted with other looping constructs such as `for` loops and `do…while` loops. The following table highlights their key differences:
Loop Type | Condition Check Location | Use Case |
---|---|---|
While Loop | Before the loop | When the number of iterations is not known |
For Loop | Before the loop | When the number of iterations is known |
Do…While Loop | After the loop | When the loop should execute at least once |
Potential Pitfalls of While Loops
Despite their usefulness, while loops can lead to issues if not managed correctly:
- Infinite Loops: If the condition never evaluates to , the loop continues indefinitely, which can freeze or crash the application.
- Performance Issues: Excessive iterations without proper exit conditions can lead to performance degradation.
To mitigate these risks, it is crucial to ensure that the loop’s condition will eventually become and to include break statements if necessary to exit the loop under specific circumstances.
Understanding the Functionality of While Loops
While loops in JavaScript provide a mechanism for executing a block of code repeatedly as long as a specified condition evaluates to true. This control structure is especially useful in scenarios where the number of iterations is not predetermined, allowing for dynamic execution based on variable conditions.
Syntax of a While Loop
The basic syntax for a while loop is as follows:
“`javascript
while (condition) {
// code block to be executed
}
“`
- Condition: A boolean expression that determines whether the loop continues to run.
- Code Block: The set of instructions that will execute repeatedly as long as the condition remains true.
Use Cases for While Loops
While loops are particularly advantageous in several situations:
- Indeterminate Iterations: When the number of iterations is not known beforehand, such as when reading data until an end condition is reached.
- User Input Validation: To repeatedly prompt the user for input until valid data is received.
- Dynamic Data Processing: Processing data that may change in size or conditions during execution, such as reading from a stream.
Example of a While Loop
“`javascript
let count = 0;
while (count < 5) { console.log("Count is: " + count); count++; } ``` In the example above, the loop will print the current value of `count` and increment it until `count` reaches 5. Key Characteristics of While Loops
- Infinite Loops: If the condition never evaluates to , the while loop will run indefinitely. This can lead to performance issues or application crashes.
- Pre-checking Condition: The condition is evaluated before the execution of the loop’s body, ensuring that the code block may not execute at all if the condition is from the start.
Best Practices
- Ensure Condition Changes: Always ensure that the loop’s condition will eventually become to prevent infinite loops.
- Use Descriptive Conditions: Write clear and descriptive conditions to enhance code readability.
- Limit Scope of Variables: Keep loop variables scoped appropriately to avoid conflicts with other parts of your code.
Comparison with Other Loop Types
Feature | While Loop | For Loop | Do…While Loop |
---|---|---|---|
Condition Check | Before executing the loop | Before executing the loop | After executing the loop |
Initialization | Must be done externally | Done within the loop statement | Must be done externally |
Use Case | Indeterminate iterations | Known number of iterations | Must execute at least once |
Conclusion
While loops serve a critical function in JavaScript for situations where the number of iterations is uncertain. By understanding their structure, use cases, and best practices, developers can effectively leverage while loops to manage dynamic data and conditions.
Understanding the Importance of While Loops in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “While loops are essential in JavaScript for scenarios where the number of iterations is not predetermined. They allow developers to create dynamic and flexible code that can adapt to varying conditions, making them invaluable in real-time applications.”
Mark Thompson (Lead JavaScript Developer, CodeCraft Solutions). “Using while loops effectively can enhance performance in specific cases, such as when processing large datasets. They enable the implementation of custom exit conditions, which can lead to more efficient memory usage compared to traditional for loops.”
Linda Martinez (JavaScript Educator, Web Development Academy). “While loops serve as a fundamental teaching tool for understanding control flow in programming. They illustrate the concept of iteration and condition checking, which are crucial for beginners to grasp before moving on to more complex programming constructs.”
Frequently Asked Questions (FAQs)
Why do we use while loops in JavaScript?
While loops are used in JavaScript to execute a block of code repeatedly as long as a specified condition evaluates to true. They are particularly useful for scenarios where the number of iterations is not known beforehand.
What is the syntax of a while loop in JavaScript?
The syntax of a while loop in JavaScript is as follows:
“`javascript
while (condition) {
// code to be executed
}
“`
The loop continues to execute until the condition becomes .
When should I prefer a while loop over a for loop?
A while loop is preferred when the number of iterations is not predetermined, such as when waiting for user input or processing data until a specific condition is met. In contrast, a for loop is more suitable when the number of iterations is known in advance.
Can a while loop be infinite in JavaScript?
Yes, a while loop can become infinite if the condition never evaluates to . This can lead to performance issues or application crashes, so it is essential to ensure that the loop has a clear exit condition.
How can I break out of a while loop in JavaScript?
You can break out of a while loop using the `break` statement. When the `break` statement is executed, control is transferred to the statement immediately following the loop.
What are common use cases for while loops in JavaScript?
Common use cases for while loops include reading data from a stream until the end is reached, waiting for a specific user input, and processing items in a collection until a certain condition is met.
While loops in JavaScript serve as a fundamental control structure that allows developers to execute a block of code repeatedly as long as a specified condition remains true. This feature is particularly useful for scenarios where the number of iterations is not known in advance, enabling dynamic and flexible programming. By utilizing while loops, programmers can efficiently handle tasks such as iterating through data sets, managing user input, and implementing algorithms that require repeated actions until a certain state is achieved.
One of the key advantages of while loops is their simplicity and ease of use. The syntax is straightforward, making it accessible for both novice and experienced developers. Additionally, while loops can be combined with other control structures, such as if statements, to create more complex logic flows. This versatility allows for a wide range of applications, from basic scripts to intricate web applications that require real-time data processing.
Moreover, while loops can contribute to improved performance in certain scenarios. By avoiding the overhead associated with other looping constructs, such as for loops, they can be more efficient when dealing with large datasets or complex computations. However, it is crucial to implement proper exit conditions to prevent infinite loops, which can lead to application crashes or unresponsive scripts.
In summary, while loops
Author Profile
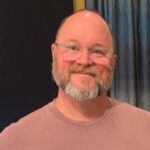
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?