What Is the Purpose of the ‘with’ Statement in Python and Why Should You Use It?
In the world of programming, efficiency and clarity are paramount, especially when managing resources such as files, network connections, or database transactions. Python, a language celebrated for its readability and simplicity, offers a powerful tool known as the `with` statement. This elegant construct not only streamlines code but also enhances its safety and reliability. For developers who seek to write cleaner, more maintainable code, understanding the purpose and functionality of the `with` statement is essential.
At its core, the `with` statement is designed to simplify resource management by ensuring that necessary cleanup actions are taken automatically. This is particularly useful in scenarios where resources need to be explicitly acquired and released, such as opening and closing files. By encapsulating these operations within a `with` block, Python developers can avoid common pitfalls, such as forgetting to release resources, which can lead to memory leaks or file corruption.
Moreover, the `with` statement promotes a clear and concise coding style that enhances readability. It allows programmers to focus on the primary logic of their code without getting bogged down by repetitive setup and teardown procedures. As we delve deeper into the intricacies of the `with` statement, we will explore its syntax, key benefits, and practical applications, equipping you with the knowledge to leverage this
The Purpose of the With Statement in Python
The `with` statement in Python is utilized for resource management and exception handling. It simplifies the process of acquiring and releasing resources such as files, network connections, and database connections. By using the `with` statement, developers can ensure that resources are properly managed, reducing the risk of resource leaks and making the code cleaner and more readable.
When a resource is used within a `with` block, the resource is automatically acquired at the beginning of the block and released at the end, even if an exception occurs during the block’s execution. This is particularly beneficial in scenarios where manual resource management would lead to complex and error-prone code.
Key benefits of using the `with` statement include:
- Automatic Resource Management: Automatically handles resource cleanup, which minimizes the likelihood of resource leaks.
- Improved Readability: Code is easier to read and maintain, as the `with` statement clearly indicates the scope of resource usage.
- Exception Safety: Ensures that resources are properly released even when exceptions are raised, preventing potential issues.
How the With Statement Works
The `with` statement works by utilizing context managers. A context manager is an object that defines the runtime context to be established when executing a `with` statement. The context manager must implement two methods: `__enter__()` and `__exit__()`.
- `__enter__()`: This method is executed when the execution flow enters the `with` block. It can be used to set up resources or perform initialization tasks.
- `__exit__()`: This method is executed when the execution flow leaves the `with` block. It is responsible for cleaning up resources, regardless of whether the block was exited normally or via an exception.
Here’s a simple example of using the `with` statement with a file:
“`python
with open(‘example.txt’, ‘r’) as file:
content = file.read()
“`
In this example, the file is opened and read within the `with` block. Once the block is exited, the file is automatically closed.
Common Use Cases for the With Statement
The `with` statement is commonly used in various scenarios, including:
- File Handling: Automatically closes files after their block of code is executed.
- Database Connections: Manages connections to databases, ensuring they are closed properly.
- Thread Locks: Acquires and releases locks in multi-threaded environments to avoid deadlocks.
Comparison Table of With Statement vs. Manual Resource Management
Feature | With Statement | Manual Management |
---|---|---|
Resource Cleanup | Automatic | Manual |
Readability | High | Moderate to Low |
Exception Safety | Guaranteed | Risk of Leaks |
Code Length | Shorter | Longer |
The comparison table highlights the advantages of using the `with` statement over manual resource management, emphasizing its effectiveness in ensuring clean, concise, and safe code.
Understanding the With Statement
The `with` statement in Python is used to wrap the execution of a block of code within methods defined by a context manager. This construct simplifies exception handling and ensures that resources are properly managed.
Key Benefits of Using the With Statement
The `with` statement provides several advantages:
- Automatic Resource Management: It automatically handles resource allocation and deallocation, which is particularly useful for file operations.
- Cleaner Code: The syntax is concise, reducing boilerplate code and enhancing readability.
- Exception Safety: It guarantees that resources are released, even if an error occurs within the block.
How the With Statement Works
The `with` statement is typically used with objects that implement the context management protocol, which includes two methods: `__enter__()` and `__exit__()`. Here’s how it functions:
- Entering the Context: When the block is entered, the `__enter__()` method is called. This method can set up the context and return a resource.
- Executing the Block: The code within the `with` block is executed.
- Exiting the Context: Upon exiting, the `__exit__()` method is invoked, which handles cleanup operations, such as closing files.
Example of the With Statement in Use
A common use case for the `with` statement is file handling. Below is an example demonstrating its functionality:
“`python
with open(‘example.txt’, ‘r’) as file:
content = file.read()
At this point, the file is automatically closed.
“`
In this example:
- The `open()` function returns a file object that is managed by the `with` statement.
- The file is read within the block, and once the block is exited, the file is closed automatically.
Custom Context Managers
You can create custom context managers using classes or the `contextlib` module. Here’s how to implement both approaches:
Using a Class:
“`python
class MyContextManager:
def __enter__(self):
print(“Entering the context”)
return self
def __exit__(self, exc_type, exc_value, traceback):
print(“Exiting the context”)
with MyContextManager() as manager:
print(“Inside the context”)
“`
Using contextlib:
“`python
from contextlib import contextmanager
@contextmanager
def my_context_manager():
print(“Entering the context”)
yield
print(“Exiting the context”)
with my_context_manager():
print(“Inside the context”)
“`
Common Use Cases for the With Statement
The `with` statement is widely used in various scenarios:
- File I/O: Simplifies file operations by ensuring files are closed properly.
- Database Connections: Manages database connections, ensuring they are closed after usage.
- Locking Mechanisms: Facilitates the acquisition and release of locks in multi-threaded applications.
Use Case | Description |
---|---|
File Handling | Automatically closes files after operations. |
Database Access | Ensures connections are properly released. |
Thread Synchronization | Manages locks, preventing deadlocks. |
The `with` statement is a powerful feature in Python that enhances resource management, improves code clarity, and ensures safety during the execution of code blocks. Its ability to work seamlessly with context managers makes it an essential tool for developers.
Understanding the Role of the With Statement in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). The ‘with’ statement in Python is crucial for resource management, particularly when dealing with file operations and network connections. It ensures that resources are properly acquired and released, minimizing the risk of memory leaks and other resource-related issues.
Michael Chen (Lead Software Engineer, CodeCraft Solutions). Utilizing the ‘with’ statement enhances code readability and maintainability. By encapsulating the setup and teardown of resources, it allows developers to focus on the core logic of their code without worrying about the cleanup process.
Sarah Thompson (Python Educator, LearnPython Academy). The ‘with’ statement is a fundamental aspect of Python’s context management protocol. It simplifies exception handling by ensuring that resources are released properly, even if an error occurs during the execution of the block, thus promoting robust programming practices.
Frequently Asked Questions (FAQs)
What is the purpose of the with statement in Python?
The with statement in Python is used to wrap the execution of a block of code within methods defined by a context manager. Its primary purpose is to ensure that resources are properly managed, such as opening and closing files, without requiring explicit cleanup code.
How does the with statement improve code readability?
The with statement enhances code readability by clearly defining the scope of resource management. It reduces boilerplate code associated with try-finally blocks, making it easier to understand the resource lifecycle at a glance.
What types of resources can be managed using the with statement?
The with statement can manage various resources, including file streams, network connections, database connections, and locks. Any object that implements the context management protocol, which includes the __enter__ and __exit__ methods, can be used with the with statement.
Can you nest with statements in Python?
Yes, you can nest with statements in Python. This allows for managing multiple resources simultaneously, ensuring that each resource is properly acquired and released in the correct order.
What happens if an exception occurs within a with statement?
If an exception occurs within a with statement, the __exit__ method of the context manager is still called. This allows for proper cleanup and handling of the exception, ensuring that resources are released even in the event of an error.
Is the with statement limited to file handling in Python?
No, the with statement is not limited to file handling. It can be used with any context manager, including those for threading, database connections, and custom resource management, providing a flexible approach to resource management across various applications.
The purpose of the ‘with’ statement in Python is primarily to simplify resource management and ensure that resources are properly cleaned up after their use. This is particularly important when dealing with file operations, network connections, or any other resources that require explicit acquisition and release. By using the ‘with’ statement, developers can avoid common pitfalls associated with resource management, such as forgetting to close files or release locks, which can lead to resource leaks and other unintended consequences.
Another significant advantage of the ‘with’ statement is its ability to enhance code readability and maintainability. The context management provided by ‘with’ allows developers to encapsulate setup and teardown logic in a clear and concise manner. This not only makes the code easier to understand but also reduces the likelihood of errors. The ‘with’ statement effectively abstracts the complexity of resource management, allowing developers to focus on the core functionality of their code.
In addition, the ‘with’ statement is built upon the context management protocol, which can be implemented in custom classes. This extensibility allows developers to create their own context managers, providing a powerful tool for managing resources in a way that is tailored to specific needs. Overall, the ‘with’ statement is an essential feature in Python that promotes best practices
Author Profile
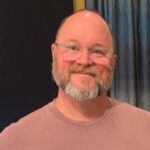
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?