What Is Indentation in Python and Why Is It So Important?
### What Is Indentation In Python?
In the world of programming, clarity and structure are paramount, and Python stands out as a language that embodies these principles through its unique approach to code formatting. One of the most distinctive features of Python is its use of indentation, which not only enhances the readability of the code but also plays a crucial role in defining the flow of execution. For both novice programmers and seasoned developers, understanding how indentation works in Python is essential for writing clean, efficient, and error-free code.
Indentation in Python serves as a visual cue that indicates the grouping of statements, particularly in control structures like loops and conditionals. Unlike many other programming languages that rely on braces or keywords to delineate code blocks, Python uses whitespace to achieve this, making the code more intuitive and easier to follow. This approach encourages developers to write neatly formatted code, which can significantly reduce the likelihood of errors and improve collaboration among team members.
As we delve deeper into the topic of indentation in Python, we will explore its fundamental principles, the importance of maintaining consistent indentation levels, and the common pitfalls that can arise from improper formatting. Whether you’re just starting your coding journey or looking to refine your skills, understanding indentation is a vital step toward mastering Python and unlocking its full potential.
Understanding Indentation in Python
Indentation in Python is a critical aspect of the language’s syntax, serving as a means to define the structure and flow of the code. Unlike many programming languages that use braces or keywords to denote blocks of code, Python relies on whitespace indentation to indicate the grouping of statements. This design choice emphasizes readability and simplicity, making Python code easy to follow.
In Python, the level of indentation determines the scope and context of control structures such as loops, conditionals, and function definitions. Proper indentation is not merely a stylistic choice; it is a syntactical requirement. Failure to maintain consistent indentation can result in errors or unexpected behavior.
Rules and Best Practices for Indentation
To effectively use indentation in Python, adhere to the following guidelines:
- Use Spaces or Tabs: Python allows both spaces and tabs for indentation, but it is essential to choose one method and stick with it throughout your code. The Python community strongly recommends using spaces over tabs. The standard is to use four spaces per indentation level.
- Consistent Indentation: All lines of code within the same block must have the same level of indentation. Mixing different types or levels of indentation can lead to `IndentationError`.
- Nested Structures: When dealing with nested control structures, each new block should be indented further than its parent block.
- Avoid Excessive Indentation: While indentation is necessary for defining blocks, excessive nesting can lead to code that is difficult to read and maintain.
Indentation Type | Description | Recommendation |
---|---|---|
Spaces | Most common method; provides consistent alignment. | Use four spaces per indentation level. |
Tabs | Less common; can lead to inconsistent appearance across different editors. | Avoid mixing tabs and spaces; if used, be consistent. |
Common Indentation Errors
When working with indentation in Python, several common errors can arise:
- IndentationError: This error occurs when the indentation is not consistent. For example:
python
if condition:
do_something()
do_something_else() # Correct indentation
do_another_thing() # Incorrect indentation
- TabError: This error is raised when a mix of tabs and spaces is used. To avoid this, ensure your editor is configured to replace tabs with spaces.
- Unexpected Indent: This occurs when a line of code is indented unexpectedly, often leading to logic errors in the program flow.
Understanding and implementing proper indentation is vital for writing clean, efficient, and readable Python code. By adhering to established conventions and best practices, programmers can avoid common pitfalls and enhance the maintainability of their code.
Understanding Indentation in Python
In Python, indentation is a critical syntactic element that defines the structure of the code. Unlike many programming languages that use braces or keywords to indicate blocks of code, Python relies on indentation levels to determine the grouping of statements.
Importance of Indentation
Indentation in Python serves several key purposes:
- Defines Code Blocks: Indentation indicates which statements belong to which code blocks, such as functions, loops, and conditionals.
- Enhances Readability: Properly indented code is easier to read and understand, facilitating collaboration among developers.
- Prevents Syntax Errors: Incorrect indentation can lead to syntax errors or unexpected behavior in code execution.
Indentation Levels
Python does not enforce a specific number of spaces for indentation; however, it is recommended to use four spaces per indentation level. Below is a comparison of different indentation practices:
Indentation Style | Description |
---|---|
2 spaces | Common in some coding styles, but less preferred in Python. |
4 spaces (recommended) | Standard practice in Python for readability and consistency. |
Tabs | Can cause issues if mixed with spaces; Python 3 disallows mixing. |
Examples of Indentation
The following examples illustrate the use of indentation in various contexts:
Conditional Statement Example:
python
if condition:
# This block is executed if condition is True
do_something()
else:
# This block is executed if condition is
do_something_else()
Loop Example:
python
for i in range(5):
# This block repeats for each iteration
print(i)
Function Definition Example:
python
def my_function():
# This block belongs to my_function
print(“Hello, World!”)
Common Indentation Errors
Indentation errors often arise in Python due to inconsistent use of spaces and tabs. The following are common mistakes:
- Mixed Indentation: Combining spaces and tabs can lead to `IndentationError`.
- Improper Block Structure: Failing to indent code that should be in a block results in logical errors.
- Excessive or Insufficient Indentation: Over-indenting or under-indenting can create confusion in code execution flow.
Best Practices for Indentation
To maintain clarity and prevent errors in your Python code, consider the following best practices:
- Use Spaces Consistently: Stick to either spaces or tabs, with four spaces being the standard.
- Configure Your Editor: Set your code editor to replace tabs with spaces automatically.
- Follow PEP 8 Guidelines: Adhering to Python’s official style guide enhances code quality and readability.
By understanding and applying these principles of indentation, Python developers can write clean, efficient, and error-free code.
Understanding Indentation in Python: Insights from Programming Experts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Indentation in Python is not merely a matter of style; it is a fundamental aspect of the language’s syntax. Unlike many programming languages that use braces or keywords to define code blocks, Python relies on indentation to determine the structure of the code. This design choice promotes readability and enforces a clean coding style, which is essential for collaborative projects.”
Michael Chen (Lead Python Developer, Open Source Community). “The importance of indentation in Python cannot be overstated. It dictates the flow of control and the grouping of statements. Misplaced indentation can lead to unexpected behavior and bugs that are often difficult to trace. Therefore, mastering proper indentation is crucial for any Python programmer aiming to write efficient and error-free code.”
Sarah Johnson (Computer Science Professor, University of Technology). “Teaching Python often highlights the role of indentation as a teaching tool for new programmers. It encourages them to think about the logical structure of their code rather than just the syntax. By understanding how indentation affects code execution, students develop a deeper appreciation for programming principles and best practices.”
Frequently Asked Questions (FAQs)
What is indentation in Python?
Indentation in Python refers to the use of whitespace at the beginning of a line to define the structure and flow of code. It indicates a block of code that belongs to a particular control structure, such as loops, functions, and conditionals.
Why is indentation important in Python?
Indentation is crucial in Python because it determines the grouping of statements. Unlike many other programming languages that use braces or keywords to define blocks, Python relies solely on indentation, making it essential for the correct execution of code.
How many spaces should be used for indentation in Python?
The standard practice is to use four spaces per indentation level. This convention is widely accepted in the Python community and helps maintain code readability and consistency.
Can tabs be used for indentation in Python?
While tabs can technically be used for indentation in Python, it is recommended to use spaces instead. Mixing tabs and spaces can lead to errors and inconsistencies, as Python treats them differently.
What happens if indentation is incorrect in Python?
Incorrect indentation will lead to an `IndentationError` or `TabError`, which prevents the code from executing. This error indicates that the structure of the code is not properly defined, causing confusion in the flow of execution.
Is there a way to check for indentation issues in Python code?
Yes, code editors and integrated development environments (IDEs) often provide features to highlight indentation issues. Additionally, running a linter like `pylint` or `flake8` can help identify and correct indentation problems in Python code.
Indentation in Python is a fundamental aspect of the language’s syntax that dictates the structure and flow of the code. Unlike many programming languages that use braces or keywords to define code blocks, Python relies on consistent indentation to delineate the scope of loops, functions, and conditional statements. This unique approach not only enhances readability but also enforces a uniform coding style, which is essential for collaborative projects and maintaining code quality.
Proper indentation is crucial for avoiding syntax errors and ensuring that the code executes as intended. Python interprets blocks of code based on their indentation level, meaning that inconsistent indentation can lead to unexpected behavior or runtime errors. As such, developers must adhere to a consistent indentation style—typically using four spaces per indentation level—to maintain clarity and prevent logical errors in their programs.
In summary, understanding and applying indentation correctly is vital for any Python programmer. It is not merely a stylistic choice but a necessary component of the language that influences how code is interpreted. By embracing proper indentation practices, developers can write cleaner, more efficient, and more maintainable code, ultimately leading to better programming outcomes.
Author Profile
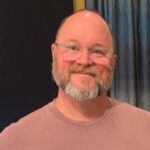
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?