What Is an Identifier in Python and Why Does It Matter?
Definition of Identifiers in Python
Identifiers in Python are names used to identify variables, functions, classes, modules, and other objects. These names are essential for referring to these entities within the code. A valid identifier must adhere to specific rules and conventions established by the Python programming language.
Rules for Creating Identifiers
To ensure that an identifier is valid in Python, it must conform to the following rules:
- An identifier can include letters (both uppercase and lowercase), digits, and underscores (`_`).
- It cannot start with a digit.
- Identifiers are case-sensitive. For instance, `myVariable` and `myvariable` are considered different identifiers.
- Special characters (such as `@`, “, `$`, etc.) and spaces are not allowed.
- Identifiers should not be any of Python’s reserved keywords (e.g., `def`, `class`, `if`, `else`, etc.).
Best Practices for Naming Identifiers
While Python allows flexibility in naming identifiers, following best practices enhances code readability and maintainability:
- Use descriptive names that convey the purpose of the identifier.
- Prefer lowercase letters for variable names and function names, using underscores to separate words (e.g., `my_function`).
- Use CamelCase for class names (e.g., `MyClass`).
- Keep identifiers concise but meaningful; avoid overly long names that obscure the purpose.
- Avoid using single-character names except in cases of loop counters (e.g., `i`, `j`).
- Use underscores to indicate private variables or functions (e.g., `_private_variable`).
Examples of Valid and Invalid Identifiers
The following table illustrates valid and invalid identifiers according to the rules mentioned:
Identifier | Valid/Invalid | Reason |
---|---|---|
myVariable | Valid | Starts with a letter, contains letters and an underscore |
variable1 | Valid | Starts with a letter, contains letters and digits |
1stVariable | Invalid | Starts with a digit |
my-variable | Invalid | Contains a hyphen, which is not allowed |
def | Invalid | Is a reserved keyword |
Using Identifiers in Code
Identifiers are utilized in various ways throughout Python code. Here are a few examples:
- Variable Declaration:
“`python
age = 25
name = “Alice”
“`
- Function Definition:
“`python
def calculate_area(radius):
return 3.14 * radius * radius
“`
- Class Definition:
“`python
class Animal:
def __init__(self, name):
self.name = name
“`
In each instance, identifiers provide a means to reference data and functions, facilitating interaction within the code. The adherence to naming conventions and rules ensures clarity and prevents errors during coding.
Understanding Identifiers in Python: Insights from Experts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Identifiers in Python are crucial as they serve as the names for variables, functions, classes, and other objects. They must adhere to specific naming conventions, such as starting with a letter or underscore and containing only alphanumeric characters and underscores.”
Michael Chen (Python Instructor, Code Academy). “In Python, identifiers are case-sensitive, meaning ‘Variable’ and ‘variable’ would be considered two distinct identifiers. This characteristic is essential for maintaining clarity in code and avoiding potential bugs.”
Sarah Lopez (Lead Developer, Open Source Projects). “Choosing meaningful identifiers is a best practice in Python programming. Well-named identifiers enhance code readability and maintainability, making it easier for teams to collaborate and understand each other’s work.”
Frequently Asked Questions (FAQs)
What is an identifier in Python?
An identifier in Python is a name used to identify a variable, function, class, module, or other objects. It serves as a reference to these entities in the code.
What are the rules for naming identifiers in Python?
Identifiers must start with a letter (a-z, A-Z) or an underscore (_), followed by letters, digits (0-9), or underscores. They cannot contain spaces or special characters, and they are case-sensitive.
Can identifiers in Python be the same as reserved keywords?
No, identifiers cannot be the same as Python reserved keywords, such as `if`, `else`, `while`, `for`, etc. Using a keyword as an identifier will result in a syntax error.
Are there any length restrictions for identifiers in Python?
There are no explicit length restrictions for identifiers in Python. However, it is advisable to keep them concise and meaningful for better readability and maintainability of the code.
What is the significance of using meaningful identifiers in Python?
Meaningful identifiers enhance code readability and maintainability, making it easier for developers to understand the purpose of variables and functions. This practice reduces confusion and errors in complex programs.
Can identifiers start with a digit in Python?
No, identifiers cannot start with a digit. They must begin with a letter or an underscore. Starting an identifier with a digit will lead to a syntax error.
In Python, an identifier is a name used to identify a variable, function, class, module, or other object. Identifiers are fundamental to writing code as they allow programmers to refer to data and functions in a meaningful way. Python has specific rules governing the formation of identifiers, which include starting with a letter or underscore, followed by letters, digits, or underscores. Additionally, identifiers are case-sensitive, meaning that ‘Variable’ and ‘variable’ would be considered distinct identifiers.
It is crucial to choose identifiers that are descriptive and convey the purpose of the variable or function they represent. This practice enhances code readability and maintainability, making it easier for others (or oneself at a later time) to understand the code’s intent. Moreover, certain keywords in Python are reserved and cannot be used as identifiers, which programmers must keep in mind to avoid syntax errors.
In summary, understanding identifiers in Python is essential for effective programming. Properly defined identifiers contribute to clearer code and facilitate collaboration among developers. By adhering to naming conventions and Python’s identifier rules, programmers can create robust and understandable codebases.
Author Profile
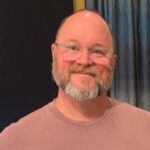
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?