What Is Case in Python: Understanding Its Importance and Usage?
What Is Case In Python?
In the world of programming, the way we write and structure our code can significantly impact its readability and functionality. One of the fundamental concepts that every Python programmer must grasp is the notion of “case.” While it may seem like a small detail, understanding how case sensitivity works in Python can be the difference between a successful script and a frustrating debugging session. Whether you’re a novice coder or a seasoned developer, mastering case conventions is essential for writing clean, efficient, and error-free code.
In Python, case refers to the distinction between uppercase and lowercase letters in variable names, function names, and other identifiers. This language is case-sensitive, meaning that `Variable`, `variable`, and `VARIABLE` are treated as three distinct identifiers. This characteristic not only influences how you name your variables and functions but also affects how you interact with built-in functions and libraries. By adhering to Python’s case conventions, you can enhance the clarity of your code and ensure that it adheres to best practices.
Moreover, understanding case in Python extends beyond mere syntax; it reflects the language’s design philosophy that emphasizes readability and simplicity. Different naming conventions, such as snake_case and CamelCase, serve specific purposes and help convey the intended use of variables and functions at a glance
Understanding Case Sensitivity in Python
In Python, case sensitivity refers to the way the language distinguishes between uppercase and lowercase letters in identifiers such as variable names, function names, and class names. This means that `Variable`, `variable`, and `VARIABLE` would be considered three distinct identifiers. Understanding case sensitivity is crucial for writing error-free code and maintaining readability.
Common Case Styles in Python
Python developers typically follow certain conventions when naming identifiers, which not only enhance code readability but also help in maintaining consistency. Here are some common case styles:
- CamelCase: The first letter of each word is capitalized, and no spaces or underscores are used. This style is often used for class names.
Example: `MyClassName`
- snake_case: All letters are lowercase, and words are separated by underscores. This style is commonly used for variable names and function names.
Example: `my_function_name`
- UPPER_SNAKE_CASE: Similar to snake_case, but all letters are uppercase. This style is typically used for constants.
Example: `MAX_LIMIT`
- lowercase: All letters are in lowercase and used primarily for module names.
Example: `mymodule`
Case Sensitivity in Python Identifiers
To further illustrate how case sensitivity affects identifiers, consider the following example:
“`python
def MyFunction():
pass
def myfunction():
pass
myFunction = 10
“`
In this example:
- `MyFunction` and `myfunction` are two different functions due to the difference in case.
- `myFunction` is a variable that is distinct from both function names.
This distinction is crucial when calling functions or referencing variables, as it can lead to errors if the incorrect case is used.
Examples of Case Sensitivity Issues
Being mindful of case sensitivity can help avoid common pitfalls. Here are some examples of potential issues:
- Reference Errors: Using the wrong case can result in `NameError` if the identifier does not exist.
- Logical Errors: Using a variable with a similar name but different casing can lead to unexpected behavior in the program.
Best Practices for Using Case in Python
To maintain clarity and prevent errors related to case sensitivity, consider the following best practices:
- Always use consistent naming conventions throughout your code.
- Follow Python’s PEP 8 style guide for naming identifiers.
- Avoid using names that differ only by case to prevent confusion.
Summary Table of Case Styles
Case Style | Description | Usage |
---|---|---|
CamelCase | First letter of each word capitalized | Class names |
snake_case | All lowercase with underscores | Variable and function names |
UPPER_SNAKE_CASE | All uppercase with underscores | Constants |
lowercase | All lowercase | Module names |
By adhering to these guidelines, developers can enhance the maintainability and readability of their Python code while minimizing case-related errors.
Understanding Case Sensitivity in Python
In Python, case sensitivity refers to the distinction between uppercase and lowercase letters in identifiers, such as variable names, function names, and class names. This feature means that `Variable`, `variable`, and `VARIABLE` are considered three different identifiers.
Key points regarding case sensitivity in Python include:
- Identifiers: All identifiers must adhere to specific naming conventions, and their case impacts how they are referenced.
- Built-in Functions: Python’s built-in functions are case-sensitive, so using `Print()` instead of `print()` will lead to a `NameError`.
- Consistency: Maintaining consistent casing in naming conventions is crucial for code readability and maintenance.
Common Naming Conventions
Python developers often use specific naming conventions to enhance code clarity. Here are some of the widely accepted styles:
- Snake Case: Uses underscores to separate words (e.g., `my_variable`). Commonly used for variable names and function names.
- Camel Case: Capitalizes the first letter of each word except the first (e.g., `myVariable`). Often used in class names.
- Pascal Case: Capitalizes the first letter of each word (e.g., `MyVariable`). Typically used for class names in some codebases.
Case Style | Example | Common Use |
---|---|---|
Snake Case | `my_variable` | Variables, Functions |
Camel Case | `myVariable` | Variables |
Pascal Case | `MyVariable` | Class Names |
Variable Naming Practices
When naming variables in Python, consider the following best practices:
- Descriptive Names: Use names that clearly indicate the variable’s purpose, such as `student_age` instead of `sa`.
- Avoid Reserved Words: Do not use Python’s reserved keywords (e.g., `def`, `class`, `if`) as variable names.
- Length: While names should be descriptive, they should also be concise. Aim for a balance between clarity and brevity.
Functions and Method Naming
Function and method names also follow specific conventions:
- Use snake case for function names (e.g., `calculate_total`).
- Ensure the name conveys the function’s action (e.g., `fetch_data_from_api`).
Class Naming Conventions
Class names in Python typically use Pascal case. Key attributes include:
- Class names should be nouns, representing entities or concepts (e.g., `Animal`, `Car`).
- Maintain clarity by keeping class names descriptive and relevant to their functionality.
Case Sensitivity in Data Types
In addition to identifiers, case sensitivity extends to certain data types, particularly strings. For instance:
- The strings `hello` and `Hello` are distinct.
- String comparison is case-sensitive, which can affect conditional checks and data processing.
Conclusion on Case Sensitivity
Understanding case sensitivity in Python is crucial for writing effective and error-free code. Adhering to established naming conventions not only prevents errors but also enhances code readability and maintainability. By being mindful of case in identifiers and following best practices, developers can create more robust and clear Python applications.
Understanding Case Sensitivity in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, case sensitivity is a fundamental aspect of the language’s design. Variable names, function names, and class names are all case-sensitive, meaning that ‘Variable’, ‘variable’, and ‘VARIABLE’ would be interpreted as three distinct identifiers. This characteristic is crucial for developers to understand, as it can lead to bugs if not properly managed.”
Mark Thompson (Lead Python Developer, CodeCraft Solutions). “Python’s case sensitivity allows for greater flexibility in naming conventions. Developers can use similar names with different cases to differentiate between variables, but this practice should be approached with caution. Consistent naming conventions are essential for maintaining code readability and preventing confusion among team members.”
Lisa Nguyen (Python Educator, Code Academy). “When teaching Python, I emphasize the importance of case sensitivity to my students. It’s not just a technical detail; it reflects the broader principles of programming where precision is key. Misunderstanding how case works can lead to frustrating debugging sessions, so I encourage new programmers to adopt a disciplined approach to naming their variables and functions from the outset.”
Frequently Asked Questions (FAQs)
What is case in Python?
Case in Python refers to the distinction between uppercase and lowercase letters in identifiers, such as variable names, function names, and class names. Python is case-sensitive, meaning that `Variable`, `variable`, and `VARIABLE` are considered three different identifiers.
What are the different types of case conventions used in Python?
Python commonly uses several case conventions, including snake_case (e.g., `my_variable`), camelCase (e.g., `myVariable`), and PascalCase (e.g., `MyClass`). Snake_case is preferred for variable and function names, while PascalCase is typically used for class names.
Why is case sensitivity important in Python?
Case sensitivity is crucial in Python as it allows for the creation of identifiers that can coexist without conflict. This feature enhances code readability and maintainability, enabling developers to use meaningful names that convey purpose without ambiguity.
How does case affect built-in functions in Python?
Case affects built-in functions in Python because they must be called using the exact case as defined. For example, using `Print()` instead of `print()` will result in a NameError, as Python does not recognize the incorrect casing.
Can I use special characters in variable names along with case?
No, special characters (except for underscores) cannot be used in variable names in Python. Variable names must start with a letter or an underscore and can include letters, numbers, and underscores. Case can be used to differentiate similar names, but special characters are not permitted.
Is it a good practice to mix cases in variable names?
It is generally not recommended to mix cases in variable names, as it can lead to confusion and reduce code readability. Following established naming conventions, such as using snake_case for variables and camelCase for parameters, promotes clarity and consistency in code.
In Python, the term “case” primarily refers to the way letters are capitalized in identifiers, strings, and other textual data. Python is case-sensitive, meaning that variables and identifiers with different cases are treated as distinct entities. For instance, the variables `myVariable`, `MyVariable`, and `MYVARIABLE` would be recognized as three separate identifiers. This characteristic is crucial for developers to understand, as it affects how they write and interact with code.
Additionally, Python employs various conventions for naming variables and functions, often categorized by their case style. Common styles include snake_case, which uses underscores to separate words; camelCase, where the first letter of each subsequent word is capitalized; and PascalCase, which capitalizes the first letter of each word, including the first. Adhering to these conventions enhances code readability and maintainability, making it easier for teams to collaborate on projects.
Moreover, understanding case sensitivity is essential when working with string manipulation in Python. Functions such as `lower()`, `upper()`, and `title()` allow developers to convert strings to different cases, facilitating tasks like comparison, formatting, and user input validation. By leveraging these string methods, programmers can ensure their applications handle text data correctly
Author Profile
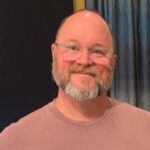
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?