What Is Caching in Python and How Can It Improve Your Code Performance?
What Is Caching In Python?
In the fast-paced world of software development, efficiency is key, and one powerful technique that can significantly enhance performance is caching. Imagine a scenario where your application can retrieve data almost instantaneously, eliminating the need to repeatedly fetch it from a slower source. This is where caching comes into play, and in the realm of Python programming, it serves as a vital tool for optimizing resource usage and speeding up applications. Whether you’re working on web applications, data processing, or any task that involves repeated data access, understanding caching can elevate your coding prowess and improve user experience.
At its core, caching in Python is about storing copies of frequently accessed data in a temporary storage location, allowing for quicker retrieval on subsequent requests. This mechanism can drastically reduce the time it takes to access data, especially when dealing with expensive operations such as database queries or complex computations. By leveraging caching, developers can ensure that their applications run smoothly and efficiently, even under heavy load.
Python offers various caching strategies and libraries, enabling developers to implement caching solutions tailored to their specific needs. From simple in-memory caching to more sophisticated distributed caching systems, the possibilities are extensive. As we delve deeper into the world of caching in Python, we will explore its benefits, common use cases,
Understanding the Basics of Caching
Caching in Python refers to the technique of storing frequently accessed data in a temporary storage area, or cache, to improve the performance of applications. By reducing the need to repeatedly fetch or compute data, caching can significantly speed up processing times and decrease resource usage.
There are various caching mechanisms available in Python, which can be broadly categorized into:
- Memory Caching: Utilizes the RAM for storing cache data, allowing for faster access.
- Disk Caching: Stores cache data on the disk, which is slower than memory but can hold larger datasets.
- Distributed Caching: Involves multiple machines working together to provide cache data, suitable for large-scale applications.
Common Caching Libraries in Python
Several libraries are available to implement caching in Python applications:
- functools.lru_cache: A built-in decorator that caches the return values of a function based on its input arguments.
- cachetools: A flexible caching library that provides various cache implementations and eviction policies.
- Django’s caching framework: A powerful framework that integrates caching into web applications seamlessly.
- Flask-Caching: An extension for Flask applications that provides caching capabilities.
Implementing Caching with functools.lru_cache
The `functools.lru_cache` decorator is a simple and efficient way to implement caching for function calls. It uses a Least Recently Used (LRU) eviction policy, which automatically removes the least recently accessed items when the cache limit is reached.
Here’s a basic example:
“`python
from functools import lru_cache
@lru_cache(maxsize=32)
def expensive_function(x):
Simulate an expensive computation
return x * x
Usage
result = expensive_function(4)
“`
In this example, calling `expensive_function(4)` for the first time will compute the result and store it in the cache. Subsequent calls with the same argument will return the cached value, bypassing the computation.
Configuring Cache Settings
When using caching libraries, you can configure various settings to optimize performance. Here is a summary of common configuration options:
Configuration Option | Description |
---|---|
maxsize | Determines the maximum number of items to store in the cache. |
ttl (Time to Live) | Specifies how long an item should remain in the cache before being evicted. |
cache type | Indicates whether to use memory, disk, or distributed caching. |
eviction policy | Defines the strategy for removing items from the cache (e.g., LRU, FIFO). |
Best Practices for Caching
To effectively utilize caching in Python, consider the following best practices:
- Identify Cacheable Data: Only cache data that is expensive to compute or frequently accessed.
- Set Appropriate TTL: Choose a time-to-live that balances freshness and performance.
- Monitor Cache Performance: Regularly assess cache hit/miss ratios to evaluate effectiveness.
- Avoid Over-Caching: Be cautious of caching too much data, which can lead to memory bloat.
By adhering to these principles, developers can harness the full potential of caching in Python applications, leading to enhanced performance and a better user experience.
Understanding Caching in Python
Caching is a technique that stores the results of expensive function calls and reuses them when the same inputs occur again. In Python, caching helps improve performance by reducing the need for repeated calculations or data retrieval.
How Caching Works
When a function is called, the input parameters and the resulting output are stored in a cache. If the function is called again with the same parameters, the cache returns the stored result instead of executing the function again. This mechanism can significantly speed up applications, especially those that involve complex computations or frequent data access.
Types of Caching in Python
Caching can be implemented in several ways, including:
- Memory Caching: Stores data in RAM, allowing for fast access.
- File-based Caching: Saves data to a file system for persistence across sessions.
- Database Caching: Utilizes a database to store and retrieve cached data.
Type | Pros | Cons |
---|---|---|
Memory Caching | Fast access, low latency | Limited by available memory |
File-based Caching | Persistent across sessions | Slower access compared to memory |
Database Caching | Scalable and persistent | Higher latency, requires database overhead |
Implementing Caching in Python
Python offers various libraries and techniques for caching. The most common include:
- `functools.lru_cache`: A built-in decorator for caching function outputs based on least-recently-used (LRU) strategy.
- `cachetools`: A third-party library providing additional caching strategies and features.
- `diskcache`: A disk and file-based caching library that allows for larger cache sizes without memory constraints.
Example of Using `functools.lru_cache`
Here is a simple example demonstrating how to use the `lru_cache` decorator:
“`python
from functools import lru_cache
@lru_cache(maxsize=128)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(10)) Output: 55
```
In this example, the Fibonacci function leverages caching to store previously computed results, significantly reducing the time complexity from exponential to linear.
Best Practices for Caching
To effectively implement caching in Python, consider the following best practices:
- Choose the Right Cache Size: Balance between memory usage and hit rate.
- Invalidation Strategy: Implement a strategy for cache invalidation to ensure stale data does not persist.
- Monitor Cache Performance: Regularly assess cache hit rates and memory usage to optimize performance.
Potential Issues with Caching
While caching can enhance performance, it may introduce challenges such as:
- Stale Data: Cached data may become outdated if the underlying data changes.
- Memory Overhead: Caching large datasets can lead to increased memory consumption.
- Complexity: Implementing and managing caching mechanisms adds complexity to the codebase.
By understanding and applying these caching techniques and practices, Python developers can significantly enhance application performance and resource efficiency.
Understanding Caching in Python: Perspectives from Professionals
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Caching in Python is a crucial performance optimization technique that allows developers to store the results of expensive function calls and reuse them when the same inputs occur again. This not only speeds up applications but also reduces the load on resources, making it a fundamental practice in high-performance computing.”
Michael Thompson (Lead Data Scientist, Data Insights Group). “In the realm of data processing, caching can significantly enhance the efficiency of algorithms, particularly in machine learning workflows. By caching intermediate results, we can avoid redundant computations, thereby accelerating the training and inference processes.”
Sarah Lee (Python Developer Advocate, Open Source Community). “Utilizing caching mechanisms such as `functools.lru_cache` in Python can simplify the implementation of caching strategies. This built-in decorator allows developers to effortlessly cache function outputs, making it an invaluable tool for optimizing code without extensive overhead.”
Frequently Asked Questions (FAQs)
What is caching in Python?
Caching in Python refers to the process of storing the results of expensive function calls and reusing them when the same inputs occur again, thereby improving performance and reducing latency.
Why is caching important in Python applications?
Caching is important because it minimizes the need for repeated calculations or data retrieval, which can significantly enhance the efficiency of applications, especially those that handle large datasets or perform complex computations.
How does Python implement caching?
Python implements caching through various mechanisms, including built-in decorators like `functools.lru_cache`, which automatically caches the results of function calls, and third-party libraries such as `cachetools` and `diskcache` for more advanced caching strategies.
What are the types of caching available in Python?
The types of caching available in Python include in-memory caching, file-based caching, and distributed caching. Each type serves different use cases depending on the application’s requirements and scale.
What are the limitations of caching in Python?
Limitations of caching in Python include potential memory consumption, cache invalidation challenges, and the risk of stale data if the underlying data changes but the cache does not update accordingly.
How can I clear the cache in Python?
You can clear the cache in Python by using the `cache_clear()` method on cached functions or by manually deleting cache entries in custom caching implementations. For `functools.lru_cache`, simply call the method to reset the cache.
Caching in Python is a technique used to store the results of expensive function calls and reuse them when the same inputs occur again. This approach significantly improves the performance of applications by reducing the time and resources required for repeated computations. Python offers various caching mechanisms, including built-in decorators such as `functools.lru_cache`, which allows developers to easily implement caching for their functions without extensive boilerplate code.
One of the key takeaways from the discussion on caching in Python is its ability to enhance efficiency in data processing and retrieval. By leveraging caching, developers can minimize latency and optimize resource utilization, especially in scenarios involving heavy computations or frequent data access. Additionally, caching strategies can be customized to suit specific application needs, allowing for fine-tuning based on memory constraints and expected usage patterns.
Furthermore, understanding the trade-offs associated with caching is crucial. While caching can lead to significant performance gains, it also introduces complexities such as cache invalidation and memory management. Developers must carefully consider these factors to implement effective caching solutions that maintain data consistency and reliability. Overall, caching is an invaluable technique in Python programming that, when applied judiciously, can lead to substantial improvements in application performance.
Author Profile
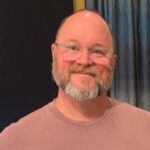
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?