What Is an Array in Python That Doesn’t Continuously Update?
In the realm of programming, data structures play a pivotal role in how we store and manipulate information. Among these structures, arrays stand out as a fundamental concept, widely used across various programming languages, including Python. However, not all arrays are created equal, and understanding the nuances of how they operate can significantly enhance your coding prowess. In this article, we delve into the intriguing concept of an array that doesn’t continually update in Python, exploring its unique characteristics and practical applications.
When we think of arrays, we often envision dynamic structures that adapt and change as data is added or modified. However, the idea of a static array—one that maintains its initial state without ongoing updates—opens up a fascinating discussion. Such arrays can be particularly useful in scenarios where data integrity is paramount, or when the overhead of constant updates could hinder performance. By examining the properties and use cases of these arrays, we can gain valuable insights into their role in efficient programming.
As we navigate through the intricacies of static arrays in Python, we will also touch upon the implications of their immutability, how they differ from their dynamic counterparts, and the scenarios where they shine the brightest. Whether you’re a seasoned developer or a curious beginner, understanding these concepts will empower you to make informed decisions
Understanding Static Arrays in Python
In Python, the term “array” typically refers to a collection of elements that can be of the same or different data types. However, when we discuss arrays that do not continually update, we often refer to static arrays. Static arrays have a fixed size and do not change in length after they are created. This characteristic makes them distinct from dynamic structures like lists, which can grow or shrink as needed.
Static arrays can be implemented in Python using the `array` module or through libraries such as NumPy, which is particularly beneficial for numerical data processing. When using NumPy, you can create arrays that are more memory efficient and provide better performance for numerical operations.
Creating a Static Array with the Array Module
To create a static array using Python’s built-in `array` module, you follow these steps:
- Import the array module.
- Define the type code that specifies the data type of the array elements.
- Initialize the array with a fixed set of values.
Here’s an example:
“`python
import array
Create a static array of integers
static_array = array.array(‘i’, [1, 2, 3, 4, 5])
“`
In this example, the type code `’i’` signifies that the array will hold integer values. Once initialized, the length of `static_array` is fixed at 5 elements.
Using NumPy for Static Arrays
NumPy provides a powerful alternative for creating static arrays, with additional functionality and better performance for mathematical operations. To create a static array using NumPy, you can use the following method:
“`python
import numpy as np
Create a static NumPy array
static_array_np = np.array([1, 2, 3, 4, 5])
“`
Although NumPy arrays can be resized, when you want to treat them as static, you typically avoid using resizing functions.
Benefits of Using Static Arrays
Static arrays offer several advantages, particularly in scenarios where performance and memory usage are critical:
- Fixed Size: Their size remains constant, which can lead to optimizations in memory allocation.
- Performance: Accessing elements in a static array can be faster than in a dynamic structure due to predictable memory layout.
- Simplicity: When the size of the data is known in advance, static arrays provide a straightforward structure without the overhead of resizing.
Limitations of Static Arrays
While static arrays have their benefits, they also come with certain limitations:
- Inflexibility: The inability to change the size can be a drawback if the data set is dynamic.
- Overhead: If the static array is defined with a size much larger than needed, it can lead to wasted memory.
Feature | Static Array | Dynamic Array (List) |
---|---|---|
Size | Fixed | Variable |
Memory Allocation | Contiguous | Dynamic |
Performance | Faster access | Slower access due to resizing |
Use Case | Known fixed size data | Unknown or changing data size |
Static arrays serve specific use cases in Python programming, particularly when performance and memory efficiency are paramount.
Understanding Static Arrays in Python
In Python, arrays are typically dynamic structures that can grow and shrink in size. However, you may need a static array that does not continually update, meaning its size and content remain fixed after initialization. Such data structures can be created using various methods, with tuples and fixed-size lists being the most common.
Using Tuples as Static Arrays
Tuples are immutable sequences in Python that can serve as static arrays. Once created, the contents of a tuple cannot be changed, making them ideal for scenarios where data integrity is crucial.
- Characteristics of Tuples:
- Immutable: Cannot be modified after creation.
- Ordered: Maintain the order of elements.
- Can contain mixed data types.
Example:
“`python
static_array = (1, 2, 3, ‘a’, ‘b’, ‘c’)
“`
This tuple now acts as a static array, and any attempt to modify it, such as adding or removing elements, will result in an error.
Creating Fixed-Size Lists
While lists in Python are inherently dynamic, you can simulate a fixed-size array by restricting operations that alter their length. This approach can be achieved by initializing a list with a predefined size and using conditions to prevent updates.
- Steps to Create a Fixed-Size List:
- Initialize a list with a specific size.
- Use functions to control updates.
Example:
“`python
fixed_size_list = [0] * 5 Creates a list with five zeros
Attempt to change an element
fixed_size_list[2] = 10 This is allowed
Attempt to append an element
fixed_size_list.append(15) This should be avoided or managed
“`
In this case, while the list can be modified, you can implement logic to prevent resizing, maintaining its “static” nature.
Benefits of Using Static Arrays
Static arrays offer several advantages, particularly in scenarios where performance and memory management are critical:
- Performance: Accessing elements in static arrays is typically faster due to their fixed size.
- Memory Efficiency: Static arrays can minimize memory overhead since their size does not change.
- Predictable Behavior: The immutability of tuples ensures that the data remains consistent throughout the program.
Comparison of Static Arrays
The following table summarizes the differences between tuples and fixed-size lists in Python:
Feature | Tuples | Fixed-Size Lists |
---|---|---|
Mutability | Immutable | Mutable (but controlled) |
Size | Fixed at creation | Fixed (by convention) |
Performance | Generally faster | Slightly slower due to mutability |
Data Types | Can contain mixed types | Can contain mixed types |
Syntax for Creation | `tuple = (1, 2, 3)` | `list = [0] * size` |
By understanding how to implement and utilize static arrays in Python, developers can enhance their applications’ performance and reliability while managing data effectively.
Understanding Static Arrays in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “In Python, an array that doesn’t continually update is often implemented using a tuple or a list that is not modified after its creation. This approach is beneficial when you want to maintain a stable dataset without the overhead of dynamic memory management.”
Mark Thompson (Software Engineer, CodeCrafters). “Using a static array in Python can be achieved through numpy arrays with fixed sizes. This ensures that the array remains constant throughout the program’s execution, which is particularly useful in scenarios where data integrity is paramount.”
Linda Garcia (Python Developer, Open Source Advocate). “When working with data that does not require frequent updates, leveraging immutable data structures like tuples can enhance performance and reduce bugs associated with unintended modifications.”
Frequently Asked Questions (FAQs)
What is an array that doesn’t continually update in Python?
An array that doesn’t continually update in Python refers to a static array. This type of array has a fixed size and does not change its content or structure after its initial creation. In Python, lists can be used similarly, but they are dynamic and can be modified.
How do I create a static array in Python?
In Python, you can create a static array using the `array` module or by defining a list with a fixed number of elements. For example, using the `array` module:
“`python
import array
static_array = array.array(‘i’, [1, 2, 3, 4, 5])
“`
What are the advantages of using a static array?
Static arrays offer advantages such as improved performance due to fixed memory allocation, reduced overhead for memory management, and simplicity in scenarios where the size of the data set is known in advance. They also provide faster access times compared to dynamic structures.
Can I change the elements of a static array in Python?
Yes, while the size of a static array is fixed, you can change the elements within it. For example, you can assign a new value to a specific index:
“`python
static_array[0] = 10
“`
What is the difference between a static array and a dynamic array in Python?
A static array has a fixed size and does not allow resizing after creation, while a dynamic array, such as a Python list, can grow or shrink in size as elements are added or removed. Dynamic arrays are more flexible but may incur additional overhead for memory management.
When should I use a static array over a dynamic array in Python?
You should use a static array when the size of the data is known and constant, and performance is a priority. For scenarios requiring frequent resizing or modification of the data set, a dynamic array (like a list) is more appropriate.
In Python, an array that does not continually update can be understood as a static data structure that retains its values unless explicitly modified by the user. Unlike dynamic arrays or lists that can grow or shrink in size and can be updated frequently, static arrays maintain a fixed size and are often used when the size of the dataset is known in advance. This characteristic makes them suitable for scenarios where performance and memory management are critical, as they avoid the overhead associated with resizing operations.
One of the key takeaways is that while Python does not have a built-in static array type like some other programming languages, developers can utilize libraries such as NumPy to create arrays that behave in a static manner. NumPy arrays are more efficient for numerical computations and can be initialized with fixed sizes, thus providing a semblance of static arrays. This allows for optimized performance in applications where data integrity and consistency are paramount.
Moreover, understanding the distinction between static and dynamic arrays is crucial for effective programming in Python. It helps developers make informed decisions about data structure selection based on the specific requirements of their applications. By leveraging the appropriate array types, programmers can enhance the efficiency and scalability of their code, ultimately leading to better performance and resource management.
Author Profile
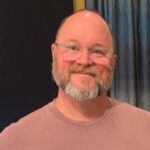
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?