What Is a Circular Import in Python and How Can You Avoid It?
In the world of Python programming, the elegance of modular design often meets the complexities of interdependent modules. One common pitfall that developers encounter in this landscape is the phenomenon known as circular imports. As projects grow in size and complexity, understanding the implications of circular imports becomes crucial for maintaining clean, efficient, and error-free code. Whether you’re a seasoned developer or just starting your coding journey, grasping the concept of circular imports can save you from frustrating debugging sessions and enhance your coding practices.
Circular imports occur when two or more modules attempt to import each other directly or indirectly, creating a loop in the import chain. This seemingly innocuous situation can lead to unexpected behaviors and runtime errors, often leaving developers scratching their heads. The challenge lies in the fact that Python’s import system is designed to load modules only once, which means that if a module is halfway loaded and another module tries to access it, it may not have all the definitions available, leading to incomplete or broken functionality.
Understanding circular imports is not just about avoiding errors; it’s also about fostering better design principles in your code. By recognizing the signs of circular dependencies, developers can refactor their code to promote clearer relationships between modules, enhancing maintainability and readability. In the following sections, we will delve deeper
Understanding Circular Imports
Circular imports occur when two or more modules attempt to import each other directly or indirectly. This can lead to complications in code execution, as the interpreter may end up in a situation where it is trying to load a module that is not fully initialized.
For example, consider two modules, `A` and `B`:
- Module `A` imports Module `B`.
- Module `B` imports Module `A`.
This scenario creates a circular dependency that can lead to issues such as `ImportError` or unexpected behavior due to incomplete initialization of one of the modules.
Consequences of Circular Imports
The main consequences of circular imports include:
- ImportError: The Python interpreter raises an error if it cannot resolve the dependencies in the correct order.
- Incomplete Initialization: If a module is partially initialized when it is imported, it may lead to runtime errors or unexpected behavior.
- Performance Issues: Circular imports can lead to longer loading times due to multiple attempts to resolve dependencies.
Issue | Description |
---|---|
ImportError | Occurs when the interpreter cannot resolve the import paths. |
Runtime Errors | Can happen if the module’s functions or variables are accessed before they are fully defined. |
Performance Bottlenecks | Increased loading time due to circular resolution attempts. |
Avoiding Circular Imports
To prevent circular imports, consider the following strategies:
- Reorganize Code Structure: Refactor your code to minimize dependencies between modules. Group related functions and classes into a single module if possible.
- Use Import Statements Wisely: Place import statements within functions or classes instead of at the top of the module. This defers the import until it is actually needed.
- Create a Separate Module for Shared Code: If two modules need to share functions or classes, create a third module that contains these shared components.
- Utilize Lazy Imports: Implement lazy imports using the `importlib` module to load dependencies only when necessary.
Example of a Circular Import
Consider the following example demonstrating circular imports:
Module A (`module_a.py`):
“`python
from module_b import func_b
def func_a():
print(“Function A”)
func_b()
“`
Module B (`module_b.py`):
“`python
from module_a import func_a
def func_b():
print(“Function B”)
func_a()
“`
When you try to execute either module, Python will encounter a circular import, leading to an ImportError. By understanding the implications of circular imports and employing best practices, developers can create cleaner and more maintainable code.
Understanding Circular Imports
Circular imports in Python occur when two or more modules depend on each other directly or indirectly. This situation can lead to issues during the execution of the code, primarily when one module is importing another that has not yet been fully defined.
How Circular Imports Occur
Circular imports typically arise in situations such as:
- Direct Circular Imports: Module A imports Module B, and Module B imports Module A.
- Indirect Circular Imports: Module A imports Module B, Module B imports Module C, and Module C imports Module A.
Example of Circular Imports
Consider the following example demonstrating direct circular imports:
Module A: `module_a.py`
“`python
from module_b import function_b
def function_a():
print(“Function A”)
function_b()
“`
Module B: `module_b.py`
“`python
from module_a import function_a
def function_b():
print(“Function B”)
function_a()
“`
When you attempt to run `function_a()` from `module_a`, Python will raise an `ImportError` due to the circular dependency.
Issues Caused by Circular Imports
Circular imports can lead to several complications:
- ImportError: Python cannot resolve the dependencies since one module is trying to load while the other is not fully initialized.
- Incomplete Module State: If a module is partially loaded when another module tries to access it, it may result in accessing uninitialized variables or functions.
Strategies to Resolve Circular Imports
To mitigate circular import issues, consider the following strategies:
- Refactoring Code: Break down large modules into smaller, more cohesive modules that minimize interdependencies.
- Using Local Imports: Move import statements inside functions or methods to delay the import until necessary. This approach can prevent circular dependencies during module loading.
Example:
“`python
In module_a.py
def function_a():
from module_b import function_b Local import
print(“Function A”)
function_b()
“`
- Creating a Separate Module: Extract common functionality used by both modules into a separate module that both can import without creating a circular dependency.
Best Practices to Avoid Circular Imports
To prevent circular imports, adhere to these best practices:
- Organize Code Logically: Maintain a clear structure to your codebase to minimize interdependencies.
- Limit Inter-module Dependencies: Only allow necessary imports between modules to avoid tight coupling.
- Use Interface Modules: Consider implementing an interface module for shared functionalities that can be imported by multiple modules without direct dependencies.
By following these practices, developers can create a more maintainable codebase while avoiding the pitfalls associated with circular imports.
Understanding Circular Imports in Python: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Circular imports occur when two or more modules depend on each other, creating a loop that can lead to import errors. This situation often arises in large codebases where module interdependencies are complex. To mitigate this, developers should consider restructuring their code to minimize interdependencies or utilize local imports within functions.
James Liu (Python Developer Advocate, CodeCraft). Circular imports can significantly hinder the maintainability of a Python project. They not only complicate the import mechanism but can also lead to runtime errors that are difficult to debug. Adopting a clear module hierarchy and using design patterns like dependency injection can help prevent such issues from arising.
Linda Martinez (Lead Python Instructor, Code Academy). Understanding circular imports is crucial for any Python developer. They can cause unexpected behavior in your programs, particularly when modules are not loaded in the expected order. To avoid circular imports, it’s advisable to keep your modules focused on specific functionalities and utilize interfaces or abstract classes to decouple dependencies.
Frequently Asked Questions (FAQs)
What is a circular import in Python?
A circular import occurs when two or more modules depend on each other directly or indirectly, creating a loop in the import structure. This can lead to issues where one module is not fully initialized when the other tries to import it.
How does a circular import affect code execution?
Circular imports can cause ImportError exceptions or result in partially initialized modules, leading to runtime errors. This happens because Python executes the import statements in the order they are encountered, which can create conflicts in the module dependencies.
What are common scenarios that lead to circular imports?
Common scenarios include two modules that import each other to access functions or classes, or when a third module imports both of the first two modules. This interdependence can create a circular reference that Python cannot resolve.
How can circular imports be resolved in Python?
Circular imports can be resolved by restructuring the code to eliminate interdependencies, using local imports within functions, or combining related modules into a single module. This helps to ensure that the necessary components are fully loaded before they are accessed.
Are there any tools or techniques to detect circular imports?
Yes, tools like `pylint`, `flake8`, and `pydeps` can help detect circular imports in Python projects. These tools analyze the codebase and report any circular dependencies, allowing developers to address them efficiently.
Can circular imports be avoided altogether?
While it may not be possible to eliminate circular imports entirely in complex systems, good design practices, such as modular programming and clear separation of concerns, can significantly reduce their occurrence.
A circular import in Python occurs when two or more modules attempt to import each other directly or indirectly, creating a cycle. This situation can lead to issues such as incomplete initialization of modules, resulting in runtime errors or unexpected behavior. Understanding the structure of module dependencies is crucial to avoid these pitfalls, as circular imports can complicate the codebase and hinder maintainability.
To prevent circular imports, developers can adopt several strategies. One effective approach is to restructure the code by consolidating related functions or classes into a single module. Alternatively, using import statements within functions or methods, rather than at the top of the module, can help mitigate the issue by delaying the import until it is actually needed. Moreover, employing design patterns such as dependency injection can also aid in managing module dependencies more effectively.
In summary, recognizing and addressing circular imports is essential for maintaining a clean and efficient codebase in Python. By being mindful of module interactions and employing best practices in code organization, developers can minimize the risk of encountering circular import issues. This proactive approach not only enhances the stability of the application but also improves overall code readability and maintainability.
Author Profile
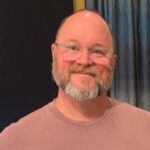
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?