What Does the ‘T’ Mean in Python? Unraveling the Mystery Behind the Code!
In the world of Python programming, understanding the nuances of data types and their representations is crucial for effective coding. One such intriguing aspect is the letter ‘T’—a seemingly simple character that can hold significant meaning in various contexts within the language. Whether you’re a seasoned developer or just starting your journey in Python, grasping what ‘T’ signifies can enhance your ability to write cleaner, more efficient code. This article delves into the multifaceted role of ‘T’ in Python, exploring its implications in type annotations, generics, and beyond.
At its core, ‘T’ often serves as a placeholder or a type variable, particularly in the realm of type hinting and generics. This allows developers to create more flexible and reusable code by specifying that a function or class can operate on different data types while maintaining type safety. Understanding how ‘T’ is used in this context not only aids in writing better code but also improves collaboration with other developers who rely on clear type definitions.
Moreover, the significance of ‘T’ extends into the realm of Python’s type system, where it plays a pivotal role in enhancing code readability and maintainability. As Python continues to evolve, the importance of clear type annotations becomes increasingly apparent, making the exploration of ‘T’ not just an
Understanding the Type `T` in Python
In Python, the letter `T` often represents a type variable in the context of type hinting and generics. It is commonly used in type annotations to denote that a function or a class can work with different data types, providing flexibility and improving code readability.
Type variables are defined using the `TypeVar` function from the `typing` module. When you declare a type variable, you can specify constraints on the types that can be used, which can help prevent errors and maintain type safety.
Defining a Type Variable
To create a type variable, you use the `TypeVar` function like this:
python
from typing import TypeVar
T = TypeVar(‘T’)
This simple line of code defines a type variable `T` that can be used in various contexts. Here is how it can be applied:
- Generic Functions: A function that can operate on different types.
- Generic Classes: A class that can hold or manipulate different types of data.
Example of Using Type Variables
Consider a function that returns the first element of a list. By using a type variable, you can ensure that the function is type-safe and works with any data type.
python
from typing import List, TypeVar
T = TypeVar(‘T’)
def get_first_element(elements: List[T]) -> T:
return elements[0]
In this example, `get_first_element` accepts a list of any type `T` and returns an element of the same type. This is particularly useful in maintaining consistency across various data types.
Using Constraints with Type Variables
You can also impose constraints on type variables to restrict them to specific types. This is useful when you want to ensure that the variable adheres to certain interfaces or classes.
python
from typing import TypeVar, Generic
T = TypeVar(‘T’, bound=’MyBaseClass’)
class MyGenericClass(Generic[T]):
def __init__(self, value: T):
self.value = value
In this case, `T` can only be a type that is a subclass of `MyBaseClass`. This adds an extra layer of type safety.
Summary of Benefits
Using type variables like `T` in Python provides several advantages:
- Flexibility: Allows functions and classes to accept multiple data types.
- Type Safety: Helps catch type-related errors during development.
- Readability: Improves code documentation and clarity.
Feature | Description |
---|---|
Flexibility | Can work with any data type. |
Type Safety | Catches errors at runtime. |
Readability | Enhances understanding of code functionality. |
By leveraging type variables, Python developers can write more robust, maintainable, and understandable code.
Understanding the Type Hinting in Python
In Python, the letter `T` is often associated with type hinting, particularly in the context of generic programming. The `T` is commonly used as a placeholder for a type variable, especially in type annotations. This allows developers to create functions and classes that can operate on different types while still providing type safety.
### Type Variable `T`
– **Definition**: A type variable is a special construct that allows you to define a generic type. In Python, this is often done using the `typing` module.
– **Syntax**: The type variable is usually defined as follows:
python
from typing import TypeVar
T = TypeVar(‘T’)
### Usage of `T`
Type variables, such as `T`, can be used in various scenarios, including:
– **Generic Functions**: Allows a function to accept arguments of any type.
python
from typing import TypeVar, List
T = TypeVar(‘T’)
def first_element(lst: List[T]) -> T:
return lst[0]
– **Generic Classes**: Enables the creation of classes that can work with multiple types.
python
from typing import Generic
class Box(Generic[T]):
def __init__(self, content: T):
self.content = content
def get_content(self) -> T:
return self.content
### Advantages of Using `T`
Using type variables like `T` enhances code quality and maintainability:
– **Type Safety**: Ensures that the types of variables are checked at compile time, reducing runtime errors.
– **Code Clarity**: Makes it clear that a function or class can work with different types, improving readability.
– **Better IDE Support**: Integrated Development Environments (IDEs) can provide better autocompletion and type checking.
### Example of Generic Function with `T`
Here’s an example that illustrates the use of `T` in a generic function:
python
from typing import List, TypeVar
T = TypeVar(‘T’)
def last_element(lst: List[T]) -> T:
if not lst:
raise ValueError(“List cannot be empty.”)
return lst[-1]
### Example of Generic Class with `T`
An example of a generic class using `T` can be observed in the following code:
python
from typing import Generic, TypeVar
T = TypeVar(‘T’)
class Container(Generic[T]):
def __init__(self, item: T):
self.item = item
def get_item(self) -> T:
return self.item
### Conclusion
In summary, the use of `T` as a type variable in Python enables developers to create flexible, reusable, and type-safe code. By leveraging the capabilities of the `typing` module, Python allows for advanced type hinting that can greatly enhance the functionality and clarity of codebases.
Understanding the Significance of ‘T’ in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, ‘T’ often represents a type variable in generic programming. It is essential for creating functions and classes that can operate on various data types while maintaining type safety.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “When discussing Python, ‘T’ can also refer to a specific instance of a type in type hints, especially when using the typing module. This allows developers to write more readable and maintainable code.”
Laura Smith (Python Educator and Author, LearnPythonNow). “Understanding what ‘T’ means in Python is crucial for anyone looking to master the language. It encapsulates the concept of type hinting, which enhances code clarity and reduces runtime errors.”
Frequently Asked Questions (FAQs)
What does the ‘T’ represent in Python type hints?
The ‘T’ in Python type hints typically refers to a generic type variable. It is commonly used in the context of type annotations to indicate that a function or class can operate on a variety of types, maintaining type safety.
How is ‘T’ used in generic programming in Python?
‘T’ is used as a placeholder for a specific type when defining generic classes or functions. By using ‘T’, developers can create functions that can accept arguments of any type while still providing type hints for better code clarity and error checking.
Can ‘T’ be defined as a specific type in Python?
Yes, ‘T’ can be defined as a specific type by using type constraints. For example, you can define ‘T’ to be a subtype of a particular class or interface, ensuring that only compatible types can be used.
Is ‘T’ case-sensitive in Python type hints?
Yes, ‘T’ is case-sensitive in Python. It is conventionally capitalized to signify that it is a type variable, while lowercase letters typically represent instances or specific objects.
Where can I find examples of using ‘T’ in Python?
Examples of using ‘T’ in Python can be found in the official Python documentation, particularly in the sections on typing and generics. Additionally, many online tutorials and coding platforms provide practical examples of its application.
What are the benefits of using ‘T’ in Python programming?
Using ‘T’ enhances code readability and maintainability by providing clear type information. It also enables better static type checking, which can help catch errors before runtime, improving overall code quality.
In Python, the letter “T” can refer to several concepts depending on the context in which it is used. One prominent interpretation is its association with type hints, particularly in the context of generic programming. In this scenario, “T” typically represents a type variable that can be used to indicate that a function or class can operate on different data types while maintaining type safety. This enhances code readability and helps developers catch type-related errors during development.
Another significant use of “T” is in the context of the built-in function `type()`, which is crucial for determining the type of an object at runtime. Understanding the type of an object is essential for debugging and ensuring that operations performed on that object are valid. Additionally, “T” may also appear in various libraries and frameworks, where it can represent specific types or be part of naming conventions that denote a class or function’s purpose.
Overall, the letter “T” in Python serves as a versatile symbol that can denote type variables, enhance type hinting practices, and facilitate clearer code documentation. Developers should familiarize themselves with its various applications to leverage Python’s dynamic typing capabilities effectively. By doing so, they can write more robust and maintainable code, ultimately leading to improved software
Author Profile
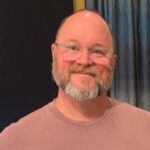
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?