What Does ‘I’ Mean in Python? Unraveling the Mystery Behind the Letter!
In the world of programming, clarity and precision are paramount, and Python stands out as a language that embodies these principles. Among its many features, the use of the letter “I” holds significant importance, particularly for those looking to enhance their coding skills and deepen their understanding of Python’s syntax and functionality. Whether you’re a novice programmer or an experienced developer, grasping what “I” signifies in Python can unlock new levels of efficiency and effectiveness in your code.
As we delve into the intricacies of Python, it’s essential to recognize that “I” can represent various concepts, depending on the context in which it is used. From variable naming conventions to its role in loops and comprehensions, understanding the implications of “I” can help clarify how Python executes tasks and manages data. This exploration will not only demystify its usage but also illustrate how it fits into the broader landscape of Python programming.
By examining the multifaceted nature of “I,” readers will gain insights into best practices and the underlying principles that guide Python’s design. This knowledge is crucial for anyone looking to write clean, efficient, and maintainable code. So, let’s embark on this journey to uncover the significance of “I” in Python and discover how it can enhance your programming
Understanding the `I` in Python
In Python, the letter `I` often appears in various contexts, such as variable names, identifiers, or even as part of specific language constructs. However, one of the most notable uses of `I` is in the context of complex numbers, where it represents the imaginary unit.
The imaginary unit is denoted as `j` in Python, which is consistent with electrical engineering conventions, while `I` is typically used in mathematical contexts. When dealing with complex numbers in Python, it is crucial to understand how to utilize these representations effectively.
Complex Numbers in Python
Python supports complex numbers natively. A complex number is composed of a real part and an imaginary part. In Python, a complex number can be created by adding a real number to an imaginary number, with the imaginary unit represented by `j`.
For example:
“`python
a = 3 + 4j Here, 3 is the real part and 4j is the imaginary part.
“`
Key Properties of Complex Numbers
- Real Part: The real component of the complex number.
- Imaginary Part: The coefficient of the imaginary unit `j`.
- Magnitude: The distance from the origin in the complex plane, calculated using the formula \( \sqrt{(real^2 + imag^2)} \).
- Phase: The angle in the polar representation, calculated using the formula \( \text{atan2}(imag, real) \).
The following table summarizes the essential components of complex numbers in Python:
Component | Description | Python Example |
---|---|---|
Real Part | The real component of a complex number. | z.real |
Imaginary Part | The imaginary component of a complex number. | z.imag |
Magnitude | The length of the vector in the complex plane. | abs(z) |
Phase | The angle of the vector in radians. | cmath.phase(z) |
Using the `cmath` Module
Python’s `cmath` module provides access to mathematical functions for complex numbers. This module includes functions such as `exp`, `log`, and trigonometric functions that operate directly on complex numbers.
Example usage:
“`python
import cmath
z = 3 + 4j
magnitude = abs(z) Magnitude
phase = cmath.phase(z) Phase
“`
These functions enable complex number manipulations, making it easier for developers to perform calculations required in various applications, from engineering to data science.
Understanding the role of `I` in Python, particularly in relation to complex numbers, is essential for effective programming in areas that involve complex calculations. Utilizing Python’s built-in capabilities and modules allows for seamless integration of complex number operations in your projects.
Understanding the `I` in Python
In Python, the letter `I` can refer to several concepts depending on the context in which it is used. Below are the primary interpretations of `I` in Python programming:
1. Variable Naming
In Python, `I` is commonly used as a variable name. It is often seen in loops, especially in contexts involving iterations. For instance:
“`python
for I in range(5):
print(I)
“`
Here, `I` serves as an index variable that takes on values from 0 to 4 during the loop execution.
2. Complex Numbers
Python has built-in support for complex numbers, which are represented as `a + bj`. However, in mathematics, the imaginary unit is often denoted by `i`. In Python, the equivalent is `j`, but understanding the mathematical convention can help clarify when discussing complex numbers.
- Example of complex number creation:
“`python
z = 3 + 4j Here, 4j represents the imaginary part.
“`
3. Convention in Mathematics
When dealing with mathematical computations or algorithms, `I` may represent the imaginary unit in theoretical discussions, especially when explaining concepts such as Fourier transforms or other areas of complex analysis.
4. Custom Classes or Modules
Developers often create classes or modules with the name `I`. For example, in an object-oriented design, `I` might signify an interface or a particular functionality. In such cases, the context of `I` is defined by the developer’s implementation.
“`python
class IExample:
def method(self):
pass
“`
5. Libraries and Frameworks
Specific libraries or frameworks may define `I` to represent particular functionalities. For instance, in data science or machine learning contexts, `I` might be an abbreviation for ‘input’ or other domain-specific terms.
Context | Usage |
---|---|
Variable Naming | Loop index |
Complex Numbers | Imaginary unit (often `j`) |
Mathematical Concepts | Theoretical discussions |
Custom Classes | User-defined interfaces |
Libraries | Domain-specific terminology |
6. Python’s Interactive Mode
In the Python interactive shell or REPL (Read-Eval-Print Loop), the variable `I` can also be used as a shorthand for input or to store temporary values. The interactive nature allows quick experimentation with variable names like `I`:
“`python
I = 10
print(I) Output: 10
“`
Using `I` in this manner is common in exploratory programming sessions.
7. Importance of Context
Ultimately, the meaning of `I` in Python is heavily context-dependent. As a programmer, it is crucial to interpret variable names and symbols based on their usage within the codebase. Understanding common conventions can aid in reading and writing Python code effectively.
Understanding the Significance of ‘I’ in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, ‘I’ is often used as a variable name in loops, particularly in for-loops. It serves as a conventional placeholder that represents the index or iterator, allowing developers to write clean and readable code.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “The letter ‘I’ in Python can also signify an imaginary unit in complex numbers. This usage is crucial in mathematical computations where complex arithmetic is involved, showcasing Python’s versatility in handling various data types.”
Sarah Thompson (Python Educator and Author, LearnPythonFast). “While ‘I’ is commonly used in programming contexts, it is essential to understand that variable naming should be meaningful. Although ‘I’ is traditional for iteration, using descriptive names enhances code readability and maintainability.”
Frequently Asked Questions (FAQs)
What does ‘I’ represent in Python programming?
‘I’ is often used as a variable name in Python, particularly in loops or iterations, to represent an index or counter. It is a convention derived from mathematical notation.
What is the significance of ‘I’ in Python’s object-oriented programming?
In object-oriented programming, ‘I’ can be used as a placeholder for instances of classes or objects, though it is not a standard convention. Developers typically choose more descriptive names for better code readability.
Does ‘I’ have a specific meaning in Python libraries or frameworks?
‘I’ does not have a universal meaning across Python libraries or frameworks. Its interpretation depends on the context in which it is used, such as variable naming or specific library documentation.
How is ‘I’ used in Python list comprehensions?
In list comprehensions, ‘I’ can serve as a loop variable to iterate over elements in a collection, facilitating the creation of new lists based on existing data.
Can ‘I’ be used as a function name in Python?
Yes, ‘I’ can be used as a function name in Python. However, it is advisable to use more descriptive names to enhance code clarity and maintainability.
Is there a difference between ‘I’ and ‘i’ in Python?
Yes, ‘I’ and ‘i’ are treated as distinct identifiers in Python due to its case sensitivity. This means they can represent different variables or functions within the same scope.
In Python, the keyword ‘I’ does not hold any special significance on its own, as it is not a reserved keyword in the language. However, it is commonly used as a variable name in various contexts, particularly in loops or list comprehensions. Its usage is often a matter of convention, where ‘i’ is frequently employed as a counter or index variable, especially in iterative processes. This practice stems from its historical use in mathematics and programming, where ‘i’ typically denotes an integer or index.
Moreover, understanding the context in which ‘I’ or ‘i’ is utilized is crucial for effective coding practices. While it may be tempting to use single-letter variable names for brevity, it is essential to consider readability and maintainability of the code. In larger or more complex programs, descriptive variable names are preferred as they enhance clarity and help other developers (or even oneself at a later time) to understand the code more easily.
In summary, while ‘I’ itself does not have inherent meaning in Python, its common usage as a variable name highlights the importance of context and coding conventions. Developers should strive to balance brevity with clarity when naming variables, ensuring that their code remains understandable and maintainable for future reference
Author Profile
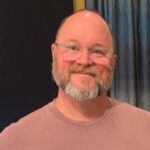
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?