What Does the Hashtag Symbol Mean in TypeScript?
In the ever-evolving landscape of programming languages, TypeScript has emerged as a powerful tool that enhances JavaScript by introducing static typing and other advanced features. As developers dive deeper into this robust language, they often encounter various symbols and syntax that can be perplexing, one of which is the hashtag (). While it may seem like a simple character at first glance, the hashtag holds significant meaning in TypeScript, serving as a gateway to understanding some of the language’s more intricate functionalities.
In this article, we will unravel the mystery behind the hashtag in TypeScript, exploring its various applications and implications within the code. From its role in decorators to its use in private fields, the hashtag is not just a stylistic choice but a critical element that can influence how developers structure and manage their code. By examining its significance, we will equip you with the knowledge to harness its power effectively, enhancing your programming skills and broadening your understanding of TypeScript’s capabilities.
Join us as we delve into the nuances of the hashtag in TypeScript, shedding light on its purpose and the contexts in which it is employed. Whether you’re a seasoned developer or just starting your journey with TypeScript, this exploration will provide valuable insights that can elevate your coding practices and deepen your appreciation for this dynamic language
Understanding Hashtags in TypeScript
In TypeScript, the term “hashtag” often refers to the use of the “ symbol which denotes private fields in classes. This feature allows developers to encapsulate properties, making them accessible only within the class itself. The of private fields enhances data encapsulation, a key principle of object-oriented programming.
Private fields serve several purposes:
- Encapsulation: By restricting access to the internal state of an object, you reduce the risk of unintended interference and promote a cleaner interface.
- Clarity: By using “, developers signal to others that these properties are intended for internal use only.
- Type Safety: TypeScript ensures that private fields cannot be accessed from outside the class, reducing runtime errors.
Here is a simple example demonstrating the use of private fields:
“`typescript
class BankAccount {
balance: number;
constructor(initialBalance: number) {
this.balance = initialBalance;
}
deposit(amount: number) {
this.balance += amount;
}
getBalance(): number {
return this.balance;
}
}
const account = new BankAccount(100);
account.deposit(50);
console.log(account.getBalance()); // Outputs: 150
// console.log(account.balance); // Error: Property ‘balance’ is not accessible outside class ‘BankAccount’.
“`
In the example above, `balance` is a private field and cannot be accessed outside the `BankAccount` class.
Comparison of Access Modifiers in TypeScript
TypeScript provides three types of access modifiers: public, private, and protected. Each serves different purposes in terms of visibility and accessibility.
Modifier | Description | Access Level |
---|---|---|
public | Accessible from anywhere, both inside and outside the class. | Unlimited |
private | Accessible only within the class it is defined. | Class only |
protected | Accessible within the class and by derived classes. | Class + Derived classes |
This table highlights the differences between the access modifiers, allowing developers to choose the appropriate level of access based on their needs.
Using private fields with the “ symbol enhances TypeScript’s capability to enforce encapsulation, ensuring that class properties remain secure and properly managed. This feature is particularly useful in large codebases where maintaining a clear and manageable architecture is crucial.
Understanding the Hashtag in TypeScript
In TypeScript, the hashtag (“) symbol is used primarily to denote private fields in classes. This feature enhances encapsulation and helps in controlling access to class members. Here’s a detailed examination of its implications and usage.
Private Fields in TypeScript
Private fields allow developers to declare properties that cannot be accessed outside the class in which they are defined. This is crucial for maintaining data integrity and encapsulation.
- Syntax: To define a private field, prefix the field name with a “.
“`typescript
class MyClass {
privateField: number;
constructor(value: number) {
this.privateField = value;
}
getPrivateField() {
return this.privateField;
}
}
“`
- Access: Attempting to access a private field from outside the class results in a syntax error.
“`typescript
const obj = new MyClass(10);
console.log(obj.privateField); // Error: Private field ‘privateField’ must be declared in an enclosing class
“`
Benefits of Using Private Fields
Utilizing private fields in TypeScript provides several advantages:
- Encapsulation: Keeps the internal state of the object hidden and secure from unintended modifications.
- Improved Readability: Clearly indicates which properties are intended for internal use only.
- Avoids Naming Conflicts: Prevents accidental overwrites of properties in subclasses or external code.
Comparison with Other Access Modifiers
TypeScript also supports other access modifiers such as `public` and `protected`. Below is a comparison of these modifiers:
Modifier | Description |
---|---|
Public | Members can be accessed from anywhere. This is the default. |
Protected | Members can be accessed within the class and subclasses only. |
Private | Members can be accessed only within the class itself. |
Private (Hashtag) | Similar to `private`, but with stricter access control preventing any access outside the class. |
Limitations of Private Fields
While private fields are powerful, they come with limitations:
- No Inheritance: Private fields are not accessible in subclasses, even though they can be inherited.
“`typescript
class Parent {
privateField: number;
constructor(value: number) {
this.privateField = value;
}
}
class Child extends Parent {
constructor(value: number) {
super(value);
// Can’t access this.privateField
}
}
“`
- Compatibility: As of now, private fields are part of the ECMAScript proposal, which may affect compatibility with older JavaScript environments.
Utilizing the hashtag in TypeScript to define private fields enhances encapsulation and ensures that class properties remain secure from external access. This feature strengthens the object-oriented programming paradigm within TypeScript, promoting better software design practices.
Understanding the Role of Hashtags in TypeScript Development
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In TypeScript, the hashtag symbol is often used as a shorthand for decorators, which are a powerful feature that allows developers to modify the behavior of classes and methods. This capability enhances code readability and maintainability, making it easier to implement cross-cutting concerns like logging and validation.”
James Liu (TypeScript Advocate and Author, Modern Web Development). “While many associate hashtags with social media, in TypeScript, they signify a specific syntax for private fields in classes. This allows developers to encapsulate data more effectively, promoting better object-oriented programming practices and reducing the likelihood of unintended access to class properties.”
Sarah Thompson (Lead Developer, CodeCraft Solutions). “The use of hashtags in TypeScript is indicative of the language’s evolution towards more robust and expressive features. It provides a clear and concise way to define private members, which is essential for maintaining clean and secure codebases in large-scale applications.”
Frequently Asked Questions (FAQs)
What does the hashtag symbol () represent in TypeScript?
The hashtag symbol in TypeScript is used to denote private class members. It indicates that the property or method is not accessible outside the class, enhancing encapsulation.
How do I declare a private member using a hashtag in TypeScript?
To declare a private member, prefix the member name with a hashtag. For example: `privateMethod() { … }` defines a private method within a class.
Can I access private members from outside the class in TypeScript?
No, private members prefixed with a hashtag cannot be accessed from outside the class. This restriction enforces encapsulation and protects the class’s internal state.
Are there any alternatives to using hashtags for private members in TypeScript?
Yes, TypeScript also supports the `private` keyword as an alternative for declaring private members. However, using hashtags provides a more strict enforcement of privacy.
How do hashtags in TypeScript compare to other programming languages?
Hashtags for private members in TypeScript are similar to private fields in languages like Java and C. However, the syntax differs, as TypeScript uses the hashtag symbol specifically for this purpose.
What are the benefits of using private members with hashtags in TypeScript?
Using private members with hashtags improves code maintainability and readability by clearly indicating which members are intended for internal use only, thus preventing unintended access or modification.
In TypeScript, the term “hashtag” typically refers to the use of the hash symbol () in various contexts, often associated with decorators and private class fields. The hashtag is not a standalone feature but rather a part of the syntax that signifies specific functionalities, such as indicating private properties in classes. This usage aligns with the broader JavaScript ecosystem, where the hash symbol has gained traction in modern programming paradigms.
One of the most significant aspects of the hashtag in TypeScript is its role in defining private fields. By prefixing a class property with the hashtag, developers can enforce encapsulation, ensuring that certain properties are not accessible outside the class definition. This feature enhances code security and maintainability, allowing developers to create robust applications with clearer data access patterns.
Additionally, the hashtag is integral to the implementation of decorators in TypeScript. Decorators are a powerful feature that allows developers to modify the behavior of classes and their members. The hashtag indicates that a function is being applied as a decorator, enabling advanced programming techniques such as aspect-oriented programming and dependency injection.
In summary, the hashtag in TypeScript serves as a crucial element for defining private class fields and implementing decorators. Understanding its implications can significantly enhance a
Author Profile
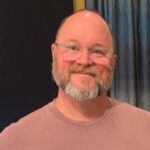
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?