What Does -1 Mean in Java? Understanding Its Significance and Usage
In the world of programming, every symbol and number carries significance, and in Java, the value `-1` is no exception. It often appears in various contexts, from array indexing to error handling, and understanding its implications can be crucial for both novice and seasoned developers alike. Whether you’re debugging a complex piece of code or trying to grasp the nuances of Java’s data structures, recognizing the role of `-1` can provide clarity and enhance your coding skills. This article delves into the multifaceted meanings of `-1` in Java, unraveling its importance in different scenarios and helping you leverage it effectively in your programming endeavors.
At its core, `-1` is frequently used as a sentinel value in Java, signaling conditions such as the absence of an element or an invalid index. For instance, when working with arrays or lists, developers often return `-1` to indicate that a search operation did not yield a result. This practice not only aids in error detection but also helps maintain cleaner, more readable code. Additionally, understanding how `-1` interacts with Java’s data structures can lead to more efficient algorithms and better resource management.
Moreover, the use of `-1` extends beyond mere indexing; it also plays a vital role in control flow
Understanding -1 in Java
In Java, the value `-1` can have several specific meanings depending on the context in which it is used. It is often utilized as a sentinel value, a placeholder, or an indicator of an error or an invalid state. Below are some of the most common scenarios where `-1` is employed in Java programming.
Common Uses of -1
- Array Indexing: In many cases, `-1` is used to signify that an index is out of bounds. For example, if a search function does not find the desired element, it may return `-1` to indicate that the element is not present in the array.
- String Searching: The `indexOf` method in the `String` class returns `-1` if the specified substring is not found. This is crucial for conditional checks when processing text.
- Error Codes: In various APIs or libraries, `-1` may represent an error condition or an unsuccessful operation, similar to how some other programming languages handle error states.
- Loop Control: In iterative algorithms, `-1` can be used to control loops, particularly when counting down or checking conditions.
Examples of -1 in Java
Here are some examples that illustrate the use of `-1` in different contexts:
“`java
// Example of using -1 with an array index
int[] numbers = {10, 20, 30};
int index = -1;
for (int i = 0; i < numbers.length; i++) {
if (numbers[i] == 20) {
index = i; // Found the element
break;
}
}
System.out.println(index); // Outputs: 1
// Example of using -1 with String.indexOf
String text = "Hello World";
int position = text.indexOf("Java");
if (position == -1) {
System.out.println("Substring not found."); // Outputs: Substring not found.
}
```
Table of Contexts for -1 Usage
Context | Meaning | Example |
---|---|---|
Array Indexing | Element not found | Return -1 if an element is absent |
String Searching | Substring not found | String.indexOf(“test”) returns -1 |
Error Codes | Indicates failure | Function returns -1 on error |
Loop Control | Control flow indication | Used for breaking out of loops |
Conclusion on the Significance of -1
The significance of `-1` in Java extends beyond just being a negative number; it serves as an integral part of controlling logic and flow within applications. Understanding its context-specific meaning can greatly enhance code readability and maintainability. Whether as an index placeholder, an error indicator, or a control mechanism, `-1` plays a pivotal role in Java programming practices.
Understanding -1 in Java
In Java, the value `-1` can have various meanings depending on the context in which it is used. It is often employed in programming to indicate specific conditions, especially in scenarios involving indexing, flags, or error handling.
Common Uses of -1
- Array Indexing: When working with arrays or lists, `-1` is frequently used to indicate that a value is not found. For instance, searching algorithms might return `-1` if the target element does not exist within the array.
- String Methods: The `String` class in Java provides methods such as `indexOf()` and `lastIndexOf()`. These methods return `-1` if the specified substring is not found:
“`java
String str = “Hello, World!”;
int index = str.indexOf(“Java”); // index will be -1
“`
- Flag Values: In certain algorithms, `-1` may be used as a sentinel value to signify a special condition, such as an error or an uninitialized state. This is particularly common in functions that return an integer value.
Examples of -1 Usage
Context | Code Example | Description |
---|---|---|
Array Search | `int index = Arrays.binarySearch(arr, target);` | Returns `-1` if `target` is not found in `arr`. |
String Search | `int pos = str.indexOf(“example”);` | Returns `-1` if “example” is not found in `str`. |
Loop Control | `for (int i = -1; i < array.length; i++) { ... }` | `-1` can be used to start a loop before the first index. |
Handling -1 in Java Code
When dealing with methods that can return `-1`, it is crucial to implement appropriate checks to avoid potential errors in the application. Here are some best practices:
- Check for -1 Before Use:
“`java
int index = str.indexOf(“test”);
if (index != -1) {
System.out.println(“Found at index: ” + index);
} else {
System.out.println(“Not found.”);
}
“`
- Use Exceptions for Error Handling: Instead of relying solely on `-1` for error signaling, consider throwing exceptions for better clarity and maintainability of the code.
- Utilize Optional Types: In Java 8 and later, you can use `Optional
` to handle cases where a value may not be present, enhancing code readability.
Conclusion on Using -1
The usage of `-1` in Java serves multiple purposes, primarily as an indication of non-existence, special conditions, or error states. Understanding its significance in various contexts can help developers write more robust and error-resistant code. Proper handling of scenarios where `-1` is returned ensures that applications function correctly without unexpected behaviors.
Understanding the Significance of -1 in Java Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). In Java, the value -1 is often used as a sentinel value to indicate the absence of a valid index. For example, when searching for an element in an array, if the element is not found, returning -1 clearly communicates that the search was unsuccessful.
Michael Chen (Java Developer, CodeCraft Solutions). In the context of Java, -1 can also serve as a flag in various algorithms, particularly in string manipulation methods like indexOf(). When a character or substring is not present, returning -1 allows developers to handle such cases gracefully without throwing exceptions.
Linda Patel (Computer Science Professor, State University). The use of -1 in Java is a common practice in programming logic. It is crucial for developers to understand its implications, especially when dealing with loops and conditional statements, as it can significantly affect the flow of the program and the handling of edge cases.
Frequently Asked Questions (FAQs)
What does -1 represent in Java?
- In Java, -1 commonly indicates an error or a special condition, such as the absence of a valid index in an array or a failure in a search operation.
Why is -1 used in array indexing?
- Java arrays are zero-indexed, meaning the first element is at index 0. A return value of -1 often signifies that a search operation, such as `indexOf`, did not find the specified element.
How does -1 relate to string operations in Java?
- In string operations, methods like `indexOf` return -1 if the substring is not found within the string, indicating that the search was unsuccessful.
Can -1 be used as a valid index in Java collections?
- No, -1 is not a valid index for Java collections like `ArrayList` or arrays. Attempting to access an index of -1 will result in an `ArrayIndexOutOfBoundsException`.
What should I do if my method returns -1?
- If a method returns -1, check the context of the operation. It usually indicates that the sought-after element or index does not exist, and you should handle this condition appropriately in your code.
Are there other meanings for -1 in Java?
- Yes, -1 can also be used as a sentinel value in various contexts, such as indicating an uninitialized state or a default value in custom implementations. Always refer to the specific documentation for context.
In Java, the value of -1 can have various meanings depending on the context in which it is used. It is commonly employed as a sentinel value to indicate an error or an invalid state. For instance, when searching for an index in an array or a list, returning -1 signifies that the desired element was not found. This convention helps developers quickly identify issues without the need for additional error handling mechanisms.
Additionally, -1 can be significant in mathematical operations and comparisons. In certain algorithms, such as those involving loops or recursive functions, -1 may be used to adjust indices or to signify the end of a sequence. Understanding the context in which -1 is applied is crucial for effective debugging and code maintenance, as it can lead to different interpretations based on the surrounding logic.
Overall, recognizing the implications of using -1 in Java is essential for developers. It serves as a powerful tool for signaling errors and managing control flow within applications. By adhering to established conventions and thoroughly documenting its use, developers can enhance code clarity and facilitate easier collaboration within teams.
Author Profile
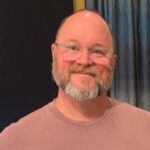
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?