Why Does My WebSocket Close Before the Connection is Established?
In the fast-paced world of web development, real-time communication has become a cornerstone of modern applications. WebSockets, a protocol designed to facilitate interactive communication between a client and a server, offer a seamless way to push data in real-time. However, developers often encounter perplexing issues that can derail their efforts, one of the most frustrating being the error message: “WebSocket is closed before the connection is established.” This cryptic notification can leave even seasoned programmers scratching their heads, pondering what went wrong in their seemingly straightforward implementation.
Understanding the nuances of WebSocket connections is essential for anyone looking to harness the full potential of this powerful technology. When a WebSocket fails to establish a connection, it can stem from a variety of factors, including network issues, server configurations, or even client-side errors. Each of these elements plays a crucial role in the handshake process that initiates a WebSocket connection, and a failure at any point can lead to the dreaded closed state.
In this article, we will delve into the intricacies of WebSocket connections, exploring common pitfalls that lead to this error and offering insights into troubleshooting strategies. By dissecting the underlying causes and providing practical solutions, we aim to equip developers with the knowledge they need to overcome this challenge and
Understanding WebSocket Connection Closure
A WebSocket connection can be closed for various reasons, and understanding these reasons is crucial for troubleshooting issues such as “WebSocket is closed before the connection is established.” Here are some common scenarios that lead to premature closure:
- Network Issues: Temporary loss of connectivity can cause the WebSocket to close unexpectedly.
- Server Configuration: Misconfigured server settings, such as timeout limits or maximum connection limits, can lead to early disconnection.
- Client-side Errors: JavaScript errors or resource constraints on the client can prevent a successful connection.
- Protocol Mismatch: Incompatibility between the client and server WebSocket protocols can result in closure before establishment.
Common Error Messages
When dealing with WebSocket connections, developers may encounter several error messages that provide insight into the problem. Here are a few common messages:
Error Message | Possible Cause |
---|---|
“WebSocket is closed before the connection is established” | Network failure or server response issues |
“Connection refused” | Incorrect WebSocket server URL or port |
“Unexpected server response” | Server not properly configured for WebSocket |
“Failed to fetch” | CORS policy blocking the connection |
Troubleshooting Steps
To resolve the issue of WebSocket closures before the connection is established, consider the following troubleshooting steps:
- Check Network Connectivity: Ensure that the network is stable and that there are no firewalls or proxies interfering with the connection.
- Verify Server Configuration: Review server settings to confirm that WebSocket support is enabled and that there are no restrictive timeout settings.
- Inspect Client Code: Debug the client-side code for potential errors or exceptions that may disrupt the connection process.
- Test with Different Browsers: Sometimes, compatibility issues arise with specific browsers. Testing with multiple browsers can help identify the problem.
- Examine Server Logs: Check server logs for any errors or warnings related to WebSocket connections.
Best Practices for WebSocket Connections
Implementing best practices can help minimize issues related to WebSocket connections. Here are some recommendations:
- Use a Reliable WebSocket Library: Choose a well-maintained library that handles WebSocket connections efficiently.
- Implement Reconnection Logic: In cases of disconnection, automatically attempt to reconnect after a specified interval.
- Monitor Connection Status: Use events like `onopen`, `onclose`, and `onerror` to monitor the connection status and take appropriate actions.
- Employ Heartbeat Mechanisms: Regularly send heartbeat messages to keep the connection alive and detect disconnections promptly.
By following these troubleshooting steps and best practices, developers can significantly reduce the occurrence of premature WebSocket closures and enhance the reliability of their applications.
Understanding WebSocket Connection Lifecycle
WebSocket connections undergo specific phases during their lifecycle, which are crucial for understanding the error “WebSocket is closed before the connection is established.” The lifecycle can be divided into three primary states:
- Connecting: The initial phase when the WebSocket client attempts to open a connection to the server.
- Open: The connection has been established successfully, allowing for data transmission.
- Closed: The connection is terminated, either by the client or the server.
Understanding these states can help diagnose issues related to premature disconnections.
Common Causes of WebSocket Closure
Several factors can lead to a WebSocket being closed before the connection is fully established:
- Network Issues: Unstable or slow internet connections may hinder the completion of the handshake process.
- Server Configuration: Incorrect server settings can reject connections. Ensure the WebSocket server is properly configured to accept incoming connections.
- Client-side Errors: Bugs or misconfigurations in the client code can prevent a successful connection.
- Timeouts: If the handshake does not complete within a specified timeframe, the connection may be closed by the client or server.
- Cross-origin Requests: Ensure that the WebSocket server allows cross-origin requests if the client is hosted on a different domain.
Troubleshooting Steps
To address the issue effectively, follow these troubleshooting steps:
- Check Network Conditions:
- Test the network stability and latency.
- Use tools like Ping or Traceroute to identify any connectivity issues.
- Review Server Logs:
- Check the server logs for any error messages during connection attempts.
- Ensure that the WebSocket server is up and running.
- Inspect Client Code:
- Validate the WebSocket URL being used in the client code.
- Ensure that the WebSocket connection is initiated correctly.
- Adjust Timeouts:
- Increase timeout settings on both the client and server side if necessary.
- Cross-Origin Resource Sharing (CORS):
- Confirm that the server allows requests from the client’s origin.
- Implement appropriate CORS headers in the server configuration.
Using Browser Development Tools
Browser development tools can provide insights into WebSocket connections. Utilize the following features:
- Network Tab: Monitor WebSocket connections and observe the handshake process.
- Console Tab: Look for error messages that may indicate issues with connection attempts.
- Performance Tab: Analyze the time taken for the WebSocket connection to establish.
Tool | Functionality |
---|---|
Network | Monitor WebSocket frames and events |
Console | Display errors and log messages |
Performance | Evaluate connection timings and delays |
Best Practices for WebSocket Implementation
To minimize connection issues, adhere to these best practices:
- Use a reliable WebSocket library that handles reconnections and errors gracefully.
- Implement error-handling mechanisms to retry connections automatically.
- Keep the WebSocket server updated to ensure compatibility and security.
- Use secure WebSocket (wss://) in production environments to avoid potential security vulnerabilities.
By following these guidelines, developers can enhance the reliability of WebSocket connections and address premature closure issues effectively.
Understanding Websocket Connection Issues
Dr. Emily Carter (Senior Software Engineer, Real-Time Systems Inc.). “The error ‘Websocket Is Closed Before The Connection Is Established’ typically indicates that the client is attempting to communicate with the server before the handshake process is complete. This can be caused by network latency or incorrect server configurations that prevent a successful connection.”
Mark Thompson (Web Technologies Consultant, FutureWeb Solutions). “In my experience, this issue often arises from improper handling of asynchronous operations in the client code. Developers should ensure that the WebSocket connection is fully established before attempting to send any messages, as premature requests can lead to unexpected closures.”
Lisa Tran (Lead Developer, Streamline Communications). “It is crucial to implement robust error handling and reconnection strategies when working with WebSockets. If the connection closes unexpectedly, the application should be designed to gracefully attempt to reconnect rather than leaving the user with a broken experience.”
Frequently Asked Questions (FAQs)
What does it mean when a WebSocket is closed before the connection is established?
When a WebSocket is closed before the connection is established, it indicates that the handshake process failed, resulting in the connection being terminated prematurely. This can occur due to network issues, server configurations, or client-side errors.
What are common reasons for a WebSocket connection to close prematurely?
Common reasons include server-side misconfigurations, firewall restrictions, incorrect WebSocket URL, or issues with the client-side code that may prevent the connection from being properly established.
How can I troubleshoot a WebSocket connection that closes before establishment?
To troubleshoot, check the WebSocket server logs for errors, verify the WebSocket URL, ensure that the server is reachable, and review any network policies or firewalls that may be blocking the connection.
Are there specific browser settings that can affect WebSocket connections?
Yes, certain browser settings, such as security policies or extensions that block WebSocket connections, can affect the ability to establish a WebSocket connection. Ensure that the browser allows WebSocket traffic.
What tools can I use to diagnose WebSocket connection issues?
Tools such as browser developer tools (e.g., Chrome DevTools), network monitoring software, and WebSocket testing tools like Postman can help diagnose connection issues by providing insights into the connection process and any errors encountered.
Can server-side code affect WebSocket connection establishment?
Yes, server-side code can significantly impact WebSocket connection establishment. Issues such as improper handling of upgrade requests, incorrect response headers, or server overload can lead to connection failures.
The issue of a WebSocket being closed before the connection is fully established is a common challenge faced by developers working with real-time web applications. This problem can arise from various factors, including network instability, server configuration errors, or client-side code issues. Understanding the underlying causes is essential for effectively troubleshooting and resolving these connection failures.
One of the primary reasons for premature WebSocket closure is network-related interruptions. Fluctuations in network connectivity can lead to dropped connections. Additionally, server-side configurations, such as timeouts or resource limitations, can also result in the WebSocket closing unexpectedly. It is crucial to ensure that both client and server configurations are optimized for WebSocket connections to minimize these risks.
Furthermore, developers should implement robust error handling and reconnection strategies within their applications. By anticipating potential connection issues and providing fallback mechanisms, applications can maintain a seamless user experience even in the face of connectivity challenges. Monitoring tools can also be beneficial in diagnosing and analyzing connection problems to facilitate timely resolutions.
addressing the issue of a WebSocket being closed before the connection is established requires a multifaceted approach. By examining network conditions, server configurations, and implementing effective error handling strategies, developers can significantly reduce the occurrence of this
Author Profile
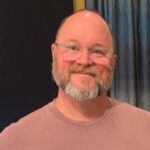
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?