How Can You Resolve the Vue Test TypeError: Cannot Read Properties of ‘_History’?
In the ever-evolving landscape of web development, Vue.js has emerged as a powerful framework that empowers developers to create dynamic and interactive user interfaces with ease. However, as with any technology, navigating its complexities can lead to unexpected challenges. One such challenge that many developers encounter is the dreaded `TypeError: Cannot read properties of ‘_History’`. This error can be particularly perplexing, especially when it arises during testing, leaving developers frustrated and searching for solutions. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and effective strategies for resolution.
The `TypeError: Cannot read properties of ‘_History’` often surfaces in the context of Vue Router and its interaction with the browser’s history API. As developers strive to implement seamless navigation within their applications, understanding the underlying mechanics of routing and history management becomes crucial. This error typically indicates a breakdown in the expected behavior of the router, which can stem from various factors, including misconfigurations or improper usage of Vue Router’s features.
By examining the common scenarios that lead to this error, we can equip ourselves with the knowledge to troubleshoot effectively and enhance our testing processes. Whether you are a seasoned Vue developer or a newcomer to the framework, this article will provide
Understanding the Error
The error message “TypeError: Cannot read properties of ‘_History'” typically indicates that the code is attempting to access a property of an object that is either or null. In the context of Vue.js applications, this issue may arise when dealing with routing, particularly if the Vue Router is not properly set up or if there is an attempt to access the history object before it has been initialized.
Common scenarios that lead to this error include:
- Attempting to access routing properties or methods before the Vue Router has been installed.
- Incorrectly referencing the router instance in components or during navigation events.
- Issues with asynchronous data fetching that lead to timing problems, where the component tries to access routing information before it is fully available.
Troubleshooting Steps
To resolve the “TypeError: Cannot read properties of ‘_History'” error, consider the following troubleshooting steps:
- **Check Router Installation**: Ensure that the Vue Router is properly installed in your Vue application. Verify that it is included in your main application file (e.g., `main.js`).
- **Validate Router Initialization**: Confirm that the router instance is correctly created and passed to the Vue instance. Here’s an example of how it should look:
“`javascript
import Vue from ‘vue’;
import App from ‘./App.vue’;
import router from ‘./router’;
new Vue({
render: h => h(App),
router,
}).$mount(‘app’);
“`
- Inspect Component Lifecycle: Make sure that your component is not trying to access router properties too early in its lifecycle. Use the `mounted` or `created` lifecycle hooks to ensure that your logic runs after the component has been fully initialized.
- Review Asynchronous Calls: If your component relies on data fetched asynchronously, ensure that you handle the router access only after the data is successfully retrieved.
- Debugging: Use console logs to trace the object state and identify where it becomes . For example:
“`javascript
console.log(this.$router);
“`
Example Code Snippet
To illustrate proper router usage, consider the following Vue component example:
“`javascript
Welcome to My App
“`
Common Causes of the Error
The following table outlines common causes of the “TypeError: Cannot read properties of ‘_History'” error along with potential solutions:
Cause | Solution |
---|---|
Router not installed | Ensure Vue Router is imported and used in the main Vue instance. |
Accessing router properties too early | Use lifecycle hooks like `mounted` or `created` to access router properties. |
router instance | Check component imports and ensure the router instance is correctly passed. |
Asynchronous data issues | Handle routing access after data is loaded, using promises or async/await. |
By following these guidelines and examining the outlined causes and solutions, you can effectively troubleshoot and resolve the “TypeError: Cannot read properties of ‘_History'” error in your Vue.js application.
Understanding the Error
The error message `TypeError: Cannot read properties of ‘_History’` typically occurs in Vue applications when attempting to access properties or methods on an or null object, specifically related to the history API in Vue Router. This can arise in various scenarios, including component lifecycles or incorrect router configurations.
Common Causes
The `TypeError` can result from several issues, including:
- Incorrect Router Setup: Ensure Vue Router is correctly initialized and configured in your application.
- Lifecycle Hook Timing: Attempting to access router properties in lifecycle hooks like `created` or `mounted` before the router is fully initialized.
- Using the Wrong Context: Accessing the router instance in a component not properly linked to the router context.
Debugging Steps
To effectively diagnose and resolve the issue, consider the following steps:
- Check Router Initialization:
- Ensure Vue Router is properly integrated into your Vue instance. For example:
“`javascript
import { createRouter, createWebHistory } from ‘vue-router’;
const router = createRouter({
history: createWebHistory(),
routes: […]
});
“`
- Verify Component Context:
- Make sure that the component is indeed a child of the router instance. Use `this.$router` within the component to access the router.
- Lifecycle Hooks:
- If using router properties in lifecycle hooks, verify the timing:
“`javascript
mounted() {
console.log(this.$router); // Should be accessible here
}
“`
- Inspect Error Stack Trace:
- Analyze the stack trace in the console to locate the exact line of code triggering the error.
Best Practices
To minimize the occurrence of this error, adhere to the following best practices:
- Use Vue Router’s `beforeRouteEnter` Hook: This hook allows you to access the router before the component is created.
- Check for Properties: Always validate the properties before accessing them:
“`javascript
if (this.$router && this.$router.history) {
// Safe to access properties
}
“`
- Implement Error Handling: Use try-catch blocks around code that accesses router properties to gracefully handle potential errors.
Example Code Snippet
Here is an example of how to correctly utilize the router in a Vue component:
“`javascript
Current Route: {{ currentRoute }}
“`
Further Resources
For additional assistance and detailed documentation, consider the following resources:
- [Vue Router Documentation](https://router.vuejs.org/)
- [Vue.js Official Guide](https://vuejs.org/guide/.html)
- [Stack Overflow Discussions](https://stackoverflow.com/) on similar issues
By systematically addressing the potential causes and employing best practices, you can effectively resolve the `TypeError: Cannot read properties of ‘_History’` in your Vue applications.
Understanding the ‘TypeError: Cannot Read Properties Of ‘_History” in Vue Testing
Dr. Emily Carter (Senior Software Engineer, Vue.js Core Team). “The ‘TypeError: Cannot Read Properties Of ‘_History” typically arises when a component attempts to access the history object before it has been properly initialized. It is crucial to ensure that the Vue Router is correctly set up in your test environment, as missing or misconfigured router instances can lead to such errors.”
Mark Thompson (Lead Developer, Frontend Innovations). “In my experience, this error often occurs during unit testing when mocking the router or history. Developers should utilize Vue Test Utils to create a proper wrapper that includes the router instance, ensuring that all dependencies are accounted for. This approach minimizes the risk of encountering properties.”
Sarah Lee (Technical Consultant, Modern Web Solutions). “When debugging this TypeError, it is essential to review the lifecycle hooks of the component in question. If the component relies on the history object during the mounted or created lifecycle hooks, ensure that the router is available at that point. Utilizing Vue Router’s `createRouter` method in tests can help simulate the expected behavior.”
Frequently Asked Questions (FAQs)
What does the error “TypeError: Cannot read properties of ‘_History'” indicate in Vue?
This error typically indicates that the code is attempting to access a property on an object that is either or null, specifically related to the Vue Router’s history mode.
What are common causes of the “_History” TypeError in Vue applications?
Common causes include incorrect configuration of the Vue Router, attempting to access router properties before the router is initialized, or issues with the component lifecycle where the router instance is not yet available.
How can I troubleshoot the “_History” TypeError in my Vue project?
To troubleshoot, ensure that the Vue Router is properly installed and configured. Verify that you are accessing router properties after the router instance is initialized, typically within lifecycle hooks like `mounted` or `created`.
Are there specific Vue Router versions that are prone to this error?
While this error can occur in various versions, it is essential to check the compatibility of the Vue Router version with your Vue.js version. Upgrading to the latest stable release may resolve related issues.
What steps should I take if the error persists after checking configurations?
If the error persists, consider reviewing your component structure for any asynchronous operations that might affect router access. Additionally, consult the Vue Router documentation or community forums for similar issues and solutions.
Can this error occur due to third-party libraries or plugins?
Yes, third-party libraries or plugins that manipulate routing or the component lifecycle can lead to this error. Ensure that any external libraries are compatible with your Vue and Vue Router versions.
The error message “TypeError: Cannot read properties of ‘_History'” in Vue testing typically indicates that the code is attempting to access a property of an object that is either or null. This issue often arises when the Vue Router is not properly configured or when the component being tested relies on router-related properties that are not available in the test environment. Understanding the context in which this error occurs is crucial for effective debugging and resolution.
To resolve this error, developers should ensure that the Vue Router is correctly set up in their test files. This may involve importing the router and providing it to the component under test using Vue Test Utils. Additionally, it is essential to verify that the component is receiving the necessary props and context that it expects, especially when dealing with navigation-related functionalities. Mocking the router or relevant properties can also be a viable solution to prevent the error from occurring.
In summary, encountering a “TypeError: Cannot read properties of ‘_History'” in Vue tests highlights the importance of proper configuration and context in component testing. By ensuring that the router is correctly integrated and that components have access to the expected properties, developers can effectively mitigate this issue. This approach not only enhances the reliability of tests but also contributes to a smoother development
Author Profile
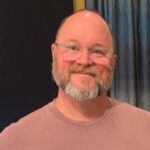
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?