How Can I Send Values to a UserForm Using VBA?
In the world of Excel and VBA (Visual Basic for Applications), userforms serve as powerful tools for data entry and interaction. They allow users to create customized interfaces that can enhance the functionality of their spreadsheets. One of the most compelling features of userforms is the ability to send values dynamically, enabling seamless data manipulation and user engagement. Whether you’re developing a simple data collection form or a complex application, mastering the art of sending values to userforms can significantly elevate your programming skills and improve the user experience.
When working with userforms in VBA, understanding how to send values effectively is crucial for creating responsive and intuitive applications. This process involves utilizing various methods to transfer data from your Excel worksheets or other sources directly into the userform controls, such as text boxes, combo boxes, and labels. By doing so, you can ensure that users have access to the most relevant information at their fingertips, making data entry more efficient and less prone to errors.
Moreover, sending values to userforms opens up a world of possibilities for real-time data validation and feedback. As users input data, you can implement logic that adjusts the form dynamically based on their selections or previous entries. This interactivity not only enhances the functionality of your forms but also provides a more engaging experience for users. As we delve deeper
Setting Up the UserForm
To effectively send values to a UserForm in VBA, the first step is to ensure that the UserForm is properly set up in your Excel environment. This involves creating the UserForm and adding necessary controls such as text boxes, labels, and buttons.
- Open the Visual Basic for Applications (VBA) editor by pressing `ALT + F11`.
- Insert a new UserForm by right-clicking on any of the objects in the Project Explorer and selecting `Insert` > `UserForm`.
- Add controls to the UserForm from the Toolbox. Common controls include:
- TextBox: For user input.
- Label: To display text or instructions.
- CommandButton: To trigger actions (e.g., submitting data).
Ensure that each control has a meaningful `Name` property for easier reference in your code.
Sending Values to UserForm Controls
To send values to the controls on your UserForm, you can set the properties of the controls directly in your VBA code. This is typically done when you want to display data for the user to review or modify.
Here’s a simple example of how to send values to a UserForm:
- Assume you have a UserForm named `UserForm1` with a TextBox named `txtInput` and a Label named `lblMessage`.
- You can set the values of these controls using the following code:
“`vba
Sub ShowUserForm()
UserForm1.txtInput.Value = “Hello, User!”
UserForm1.lblMessage.Caption = “Please enter your data below:”
UserForm1.Show
End Sub
“`
In this example, the TextBox will display “Hello, User!” when the UserForm is shown, and the Label will instruct the user to enter data.
Using Variables to Pass Values
When dealing with dynamic data, it is common to utilize variables to pass values to the UserForm. This allows for more flexibility and can enhance user interaction.
Here’s how you can accomplish this:
- Define your variables in the main module or procedure.
- Assign values to these variables based on your logic or data source.
- Pass these variables to the UserForm controls.
Example code snippet:
“`vba
Sub LoadDataToUserForm()
Dim userName As String
Dim userAge As Integer
userName = “John Doe”
userAge = 30
UserForm1.txtInput.Value = userName
UserForm1.lblMessage.Caption = “Age: ” & userAge
UserForm1.Show
End Sub
“`
This code will populate the TextBox with “John Doe” and the Label with “Age: 30”.
Table: UserForm Control Properties
When configuring UserForm controls, understanding their properties is crucial. Below is a table summarizing some common properties you may want to adjust:
Control Type | Property | Description |
---|---|---|
TextBox | Value | The text displayed in the TextBox. |
Label | Caption | The text displayed on the Label control. |
CommandButton | Caption | The text displayed on the button. |
Understanding these properties will help you effectively manage the UserForm’s user interface and improve the overall user experience.
Understanding UserForms in VBA
UserForms are powerful tools in Excel VBA that allow for the creation of custom dialog boxes where users can input or manipulate data. These forms can enhance user interaction by providing a more intuitive interface than standard Excel sheets.
Key Features of UserForms:
- Customizable controls (text boxes, combo boxes, buttons)
- Ability to handle user input dynamically
- Event-driven programming for interactive functionalities
Sending Values to UserForms
To send values from a worksheet or a module to a UserForm, you need to utilize properties of UserForm controls. This can be accomplished through various methods depending on the source of the values.
Common Scenarios for Sending Values:
- From a worksheet cell
- From a module variable
- Through user interaction with other controls
Example: Sending a Cell Value to a TextBox
To demonstrate sending a value from a worksheet cell to a UserForm, consider the following example. Assume you have a UserForm named `UserForm1` with a TextBox control named `TextBox1`.
Code Example:
“`vba
Sub ShowUserFormWithValue()
Dim cellValue As String
cellValue = ThisWorkbook.Sheets(“Sheet1”).Range(“A1”).Value
UserForm1.TextBox1.Value = cellValue
UserForm1.Show
End Sub
“`
Explanation:
- The value in cell A1 of `Sheet1` is stored in `cellValue`.
- This value is then assigned to `TextBox1` before displaying the UserForm.
Example: Sending Multiple Values to Different Controls
When working with multiple controls, you can send values to various UserForm elements simultaneously.
Code Example:
“`vba
Sub LoadFormData()
With UserForm2
.TextBox1.Value = ThisWorkbook.Sheets(“Sheet1”).Range(“A1”).Value
.TextBox2.Value = ThisWorkbook.Sheets(“Sheet1”).Range(“B1”).Value
.ComboBox1.Value = ThisWorkbook.Sheets(“Sheet1”).Range(“C1”).Value
.Show
End With
End Sub
“`
Explanation:
- This code loads values from three different cells into a UserForm with two text boxes and one combo box.
Handling User Input from UserForms
Once values are sent to the UserForm, handling user input is crucial for effective data management. You can capture the data entered by the user when they interact with the controls.
Example of Capturing Input:
“`vba
Private Sub CommandButton1_Click()
Dim userInput As String
userInput = TextBox1.Value
ThisWorkbook.Sheets(“Sheet1”).Range(“D1”).Value = userInput
Unload Me
End Sub
“`
Explanation:
- When the user clicks the command button, the value from `TextBox1` is written to cell D1 of `Sheet1`.
- The UserForm is then unloaded, closing it.
Dynamic Value Assignment Based on User Selection
You can also dynamically assign values based on user selections in combo boxes or list boxes. This enhances the interactivity of your UserForms.
Example Code for Dynamic Assignment:
“`vba
Private Sub ComboBox1_Change()
Select Case ComboBox1.Value
Case “Option1”
TextBox1.Value = “You selected Option 1”
Case “Option2”
TextBox1.Value = “You selected Option 2”
‘ Add more cases as needed
End Select
End Sub
“`
Explanation:
- This code sets `TextBox1` based on the selection made in `ComboBox1`, providing immediate feedback to the user.
Best Practices for UserForms
When working with UserForms in VBA, consider the following best practices:
- Organize Controls: Group related controls to improve usability.
- Validate Input: Always validate user input before processing to prevent errors.
- Provide Feedback: Use labels or message boxes to inform users of actions or errors.
- Optimize Performance: Limit the amount of data loaded into UserForms to enhance speed.
By following these guidelines, you can create efficient and user-friendly interfaces that streamline data entry and management in Excel.
Expert Insights on Sending Values to UserForms in VBA
Dr. Emily Carter (Senior VBA Developer, Tech Innovations Inc.). “When sending values to a UserForm in VBA, it is crucial to ensure that the data types match the expected input fields. This not only prevents runtime errors but also enhances the user experience by providing immediate feedback on incorrect entries.”
Michael Chen (VBA Programming Consultant, Excel Masters). “Utilizing the ‘Initialize’ event of the UserForm is an effective method to send values dynamically. By setting the values during this event, you can ensure that the UserForm displays the most current data, which is essential for applications requiring real-time updates.”
Sarah Johnson (Business Analyst, Data Solutions Group). “Incorporating error handling when sending values to a UserForm is vital. Implementing robust error-checking routines can significantly reduce user frustration and improve the reliability of your VBA applications, especially in complex workflows.”
Frequently Asked Questions (FAQs)
How can I send a value from a worksheet to a UserForm in VBA?
To send a value from a worksheet to a UserForm, you can reference the UserForm controls directly in your VBA code. For example, use `UserForm1.TextBox1.Value = Worksheets(“Sheet1”).Range(“A1”).Value` to assign the value from cell A1 of Sheet1 to TextBox1 on UserForm1.
Can I pass multiple values to a UserForm in VBA?
Yes, you can pass multiple values to a UserForm by assigning values to different controls. For instance, you can set multiple TextBox values like this: `UserForm1.TextBox1.Value = Worksheets(“Sheet1”).Range(“A1”).Value` and `UserForm1.TextBox2.Value = Worksheets(“Sheet1”).Range(“A2”).Value`.
What is the best way to initialize a UserForm with values from a worksheet?
The best way to initialize a UserForm with values from a worksheet is to use the UserForm’s `Initialize` event. In this event, you can set the values of the controls based on the worksheet data, ensuring they are populated when the UserForm is displayed.
How do I update a UserForm control with a value from a button click event?
To update a UserForm control with a value from a button click event, you can use the following code in the button’s click event: `UserForm1.TextBox1.Value = Worksheets(“Sheet1”).Range(“A1”).Value`. This will set the TextBox value when the button is clicked.
Is it possible to send values to a UserForm from a different module?
Yes, it is possible to send values to a UserForm from a different module by creating a public subroutine or function in the UserForm module. You can then call this subroutine from another module, passing the required values as parameters.
What should I do if the UserForm does not display the updated values?
If the UserForm does not display the updated values, ensure that you are correctly referencing the controls and that the UserForm is being shown after the values are assigned. Additionally, check for any errors in your code that may prevent execution.
In summary, utilizing VBA to send values to a UserForm is a powerful technique that enhances user interaction within Excel applications. By leveraging the capabilities of Visual Basic for Applications, developers can create dynamic forms that respond to user inputs and display relevant data. This functionality not only streamlines data entry processes but also improves the overall user experience by providing immediate feedback and interaction.
Key insights from the discussion highlight the importance of understanding the structure of UserForms and the various controls available, such as text boxes, combo boxes, and labels. Properly assigning values to these controls through VBA code allows for a seamless flow of information, enabling users to view and manipulate data efficiently. Additionally, implementing error handling and validation checks can further enhance the reliability of the UserForm, ensuring that users input accurate data.
Moreover, the integration of VBA with UserForms opens up opportunities for automating repetitive tasks and customizing workflows. This not only saves time but also reduces the likelihood of human error during data entry. By mastering the techniques for sending values to UserForms, users can significantly elevate their Excel applications, making them more robust and user-friendly.
Author Profile
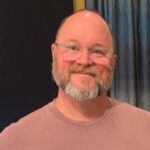
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?