How Can I Resolve the ValueError: Index Contains Duplicate Entries, Cannot Reshape?
In the world of data manipulation and analysis, encountering errors can be both frustrating and enlightening. One such error that often perplexes users is the “ValueError: Index contains duplicate entries, cannot reshape.” This message typically arises in programming environments like Python’s Pandas library, where data structures are manipulated to extract meaningful insights. Understanding this error is crucial for anyone working with data, as it not only highlights issues within your datasets but also paves the way for more robust data handling practices.
When you are working with data, especially in the context of reshaping or pivoting tables, the integrity of your index becomes paramount. Duplicate entries in your index can lead to ambiguity, making it impossible for the system to determine how to reorganize your data. This situation often arises from data collection processes, merging datasets, or simply from oversight in data preparation.
In this article, we will delve into the intricacies of this error, exploring its causes and implications. We will also discuss effective strategies to resolve it, ensuring that your data manipulation tasks proceed smoothly. Whether you’re a seasoned data analyst or a newcomer to the field, understanding how to navigate this error will enhance your ability to work with complex datasets and improve your overall data management skills.
Understanding the Error
The `ValueError: Index Contains Duplicate Entries, Cannot Reshape` typically arises in Python when using libraries such as pandas or NumPy. This error indicates that an operation attempting to reshape data structures is being hampered by non-unique index values. In data analysis and manipulation, having unique indices is often crucial for performing certain operations, including reshaping or pivoting datasets.
When a dataset contains duplicate indices, operations that require a unique mapping of data points fail, leading to this specific error. The implications of this error can be significant, particularly when working with large datasets where duplicate entries can often go unnoticed.
Common Causes
Several situations can lead to encountering this error:
- Data Import Issues: When importing data from external sources, such as CSV files, duplicates might not be immediately apparent.
- Data Merging: Combining two datasets may result in overlapping indices, especially if the merging keys are not unique.
- Manipulation Errors: During data manipulation processes such as filtering, grouping, or joining, indices may inadvertently become duplicated.
Identifying Duplicate Indices
To resolve the issue, first, identify if your DataFrame or Series contains duplicate indices. This can be done using the following pandas command:
“`python
duplicates = df.index.duplicated()
“`
You can also visualize the count of each index using:
“`python
index_counts = df.index.value_counts()
print(index_counts[index_counts > 1])
“`
This will highlight which indices are duplicated, allowing for appropriate action to be taken.
Resolving the Issue
After identifying duplicates, there are several strategies to handle them:
- Remove Duplicates: Use the `drop_duplicates()` method to eliminate rows with duplicate indices.
“`python
df = df[~df.index.duplicated(keep=’first’)]
“`
- Reset the Index: If the index is not critical for your analysis, you can reset it to remove duplicates.
“`python
df.reset_index(drop=True, inplace=True)
“`
- Aggregate Data: If duplicates are meaningful, consider aggregating the data using `groupby()`, applying a suitable aggregation function.
“`python
df = df.groupby(df.index).agg(‘mean’) Example aggregation
“`
Example of Handling Duplicates
The following table illustrates how to manage duplicate indices in a pandas DataFrame:
Index | Value |
---|---|
A | 1 |
A | 2 |
B | 3 |
To handle the above duplicates, you can choose to aggregate the values for index ‘A’:
“`python
df = df.groupby(df.index).agg(‘sum’)
“`
This results in:
Index | Value |
---|---|
A | 3 |
B | 3 |
By applying these techniques, you can effectively resolve the `ValueError` and proceed with your data analysis tasks.
Understanding the Error
The `ValueError: Index contains duplicate entries, cannot reshape` occurs in Python, particularly when using libraries like Pandas and NumPy. This error signifies that an operation attempted to reshape or reorganize data, but the index used for this operation contained duplicate values. This results in ambiguity, as reshaping requires a unique identifier for each entry.
Common scenarios where this error arises include:
- Attempting to pivot a DataFrame with non-unique index values.
- Reshaping an array or DataFrame without ensuring the uniqueness of the index.
- Merging or concatenating DataFrames that have overlapping index values.
Identifying Duplicate Entries
To address the error effectively, the first step is to identify duplicate entries in your DataFrame or array. You can use the following methods:
– **Pandas**:
“`python
duplicates = df[df.duplicated()]
“`
This command returns a DataFrame containing only the duplicate rows.
– **NumPy**:
“`python
unique, counts = np.unique(array, return_counts=True)
duplicates = unique[counts > 1]
“`
This will yield all the duplicate values in the NumPy array.
Resolving Duplicate Issues
Once duplicates are identified, consider the following strategies to resolve the issue:
– **Remove Duplicates**:
“`python
df = df.drop_duplicates()
“`
– **Group Data**:
If duplicates contain meaningful information, you can aggregate them:
“`python
df = df.groupby(‘column_name’).agg(‘mean’) or any other aggregation function
“`
– **Reset Index**:
In cases where duplicates are acceptable but require a new index:
“`python
df.reset_index(drop=True, inplace=True)
“`
– **Renaming Duplicates**:
If you want to keep all data, consider renaming duplicate indices:
“`python
df.index = [f”{i}_{count}” if count > 1 else i for i, count in zip(df.index, df.index.value_counts())]
“`
Example of Handling Duplicates
Here’s an example demonstrating how to handle duplicates before reshaping a DataFrame:
“`python
import pandas as pd
Sample DataFrame with duplicates
data = {
‘A’: [1, 2, 2, 3],
‘B’: [4, 5, 5, 6]
}
df = pd.DataFrame(data)
Identify duplicates
print(“Duplicates:\n”, df[df.duplicated()])
Remove duplicates
df = df.drop_duplicates()
Reshape the cleaned DataFrame
reshaped = df.pivot(index=’A’, columns=’B’, values=’A’)
print(“Reshaped DataFrame:\n”, reshaped)
“`
This example first identifies duplicates, removes them, and then reshapes the DataFrame without encountering the `ValueError`.
Preventing Future Errors
To avoid encountering this error in the future, consider implementing these best practices:
- Data Validation: Always validate your data for duplicates before performing operations that require unique indices.
- Consistent Indexing: When creating DataFrames, ensure that the index values are unique or properly aggregated.
- Use of Try-Except Blocks: Implement error handling to catch the `ValueError` and provide informative messages or fallback mechanisms.
By following these guidelines, you can effectively manage your data structures and prevent issues related to duplicate index entries.
Understanding the ValueError: Index Contains Duplicate Entries
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “The ValueError indicating that the index contains duplicate entries typically arises during data manipulation tasks in Python, particularly when using libraries like Pandas. It is crucial to ensure that the DataFrame’s index is unique before attempting any reshaping operations, as duplicate entries can lead to ambiguity in data representation.”
Mark Thompson (Software Engineer, Data Solutions LLC). “When faced with a ValueError related to duplicate index entries, I recommend first inspecting the DataFrame for duplicates using methods like `DataFrame.duplicated()`. This allows for proactive data cleaning, ensuring that reshaping functions like `pivot()` or `unstack()` can execute without errors.”
Linda Garcia (Machine Learning Consultant, AI Analytics Group). “In scenarios where duplicate indices are unavoidable, consider resetting the index with `DataFrame.reset_index(drop=True)` to create a new unique index. This approach not only resolves the ValueError but also maintains the integrity of the data during reshaping operations.”
Frequently Asked Questions (FAQs)
What does the error “ValueError: Index contains duplicate entries, cannot reshape” mean?
This error indicates that a DataFrame or Series in pandas has duplicate index values, which prevents reshaping operations like pivoting or unstacking from being performed. Each index must be unique for these operations to succeed.
How can I identify duplicate entries in my DataFrame index?
You can identify duplicate entries in your DataFrame index by using the `index.duplicated()` method. This method returns a boolean array indicating which index entries are duplicates. You can then filter your DataFrame based on this information.
What steps can I take to resolve the “ValueError: Index contains duplicate entries” issue?
To resolve this issue, you can either drop duplicates using the `drop_duplicates()` method, reset the index with `reset_index()`, or create a new unique index by using the `set_index()` method with a column that contains unique values.
Can I reshape a DataFrame with a non-unique index?
No, reshaping operations such as pivoting or unstacking require unique index values. If your DataFrame has a non-unique index, you must first ensure that the index is unique before attempting to reshape.
Is there a way to keep duplicate entries while reshaping?
Yes, you can use aggregation functions while reshaping to handle duplicate entries. For instance, when using `pivot_table()`, you can specify an aggregation function to summarize the data, allowing you to retain all information without raising an error.
What is the impact of duplicate index entries on data analysis?
Duplicate index entries can lead to ambiguity in data analysis, making it difficult to retrieve specific rows or perform operations that rely on unique identifiers. This can result in incorrect calculations and misleading results.
The error message “ValueError: Index contains duplicate entries, cannot reshape” typically arises in data manipulation tasks, particularly when working with libraries such as Pandas in Python. This issue occurs when attempting to reshape a DataFrame or Series that has non-unique index values. The underlying problem is that reshaping operations, such as pivoting or unstacking, require a unique index to properly organize the data into a new shape. When duplicates are present, the operation cannot determine how to allocate the data, leading to this error.
To resolve this issue, it is essential to first identify and address the duplicate entries in the index. Techniques such as using the `drop_duplicates()` method or resetting the index with `reset_index()` can be effective in eliminating duplicates. Additionally, ensuring that the data is pre-processed correctly before attempting to reshape it can prevent this error from occurring. It is also advisable to review the data structure and confirm that the intended reshaping operation is appropriate for the dataset at hand.
In summary, the “ValueError: Index contains duplicate entries, cannot reshape” error serves as a reminder of the importance of maintaining unique indices in data manipulation tasks. By implementing proper data validation and cleaning techniques, users can avoid this common
Author Profile
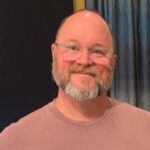
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?