Why Am I Getting a ValueError: A Linearring Requires At Least 4 Coordinates?
In the realm of geospatial data processing and analysis, encountering errors can be a frustrating yet enlightening experience. One such error, “ValueError: A Linearring Requires At Least 4 Coordinates,” serves as a crucial reminder of the underlying principles governing geometric shapes and their representations in programming. Whether you’re a seasoned developer or a curious newcomer to the world of GIS (Geographic Information Systems), understanding this error can unlock new insights into how we work with spatial data. This article delves into the intricacies of this error, exploring its causes, implications, and the best practices to avoid it in your coding endeavors.
Overview
At its core, the “ValueError: A Linearring Requires At Least 4 Coordinates” error arises when attempting to define a linear ring—a fundamental geometric structure used in various applications, from mapping to architectural design. A linear ring is essentially a closed loop that must consist of a minimum of four coordinates to be valid. This requirement ensures that the shape can be properly defined and rendered, forming the basis for more complex geometric operations and analyses.
In this article, we will explore the common scenarios that lead to this error, shedding light on how improper coordinate input can disrupt your workflow. By examining the principles behind linear rings and their significance in ge
Understanding the Error
The error message “ValueError: A Linearring Requires At Least 4 Coordinates” typically arises in programming environments that deal with geometric shapes, particularly when using libraries such as Shapely in Python. This error indicates that a `LinearRing` object, which is a closed line made up of a sequence of points, is being defined with fewer than the required coordinates.
A `LinearRing` must have at least four coordinates due to the following reasons:
- Closure Requirement: The first and last coordinates of a `LinearRing` must be the same to ensure that the shape is closed.
- Shape Definition: A minimum of three points is needed to define a polygon, but a `LinearRing` requires the closure, hence the need for at least four coordinates.
Common Causes
The error can occur in various scenarios:
- Insufficient Points: Attempting to create a `LinearRing` with only two or three distinct points.
- Data Input Errors: The coordinates being supplied may be incorrectly formatted or incomplete.
- Logical Errors: In the logic of the code, there may be conditions that lead to fewer points being passed to the `LinearRing` constructor.
Example Code
Here is an example of how this error might occur in code:
“`python
from shapely.geometry import LinearRing
Attempting to create a LinearRing with insufficient coordinates
points = [(0, 0), (1, 1), (1, 0)] Only 3 points
ring = LinearRing(points) This will raise ValueError
“`
To correct this, ensure that you provide at least four points, as shown below:
“`python
points = [(0, 0), (1, 1), (1, 0), (0, 0)] Correct: 4 points with closure
ring = LinearRing(points) This will work fine
“`
Best Practices
To avoid encountering this error, follow these best practices:
- Input Validation: Implement checks to ensure that the input list of coordinates contains at least four points.
- Automated Testing: Use unit tests to validate the behavior of functions that create `LinearRing` objects.
- Error Handling: Include exception handling to catch `ValueError` and provide meaningful feedback to users.
Coordinate Table
To illustrate the minimum requirements for `LinearRing`, consider the following table:
Number of Coordinates | Valid for LinearRing? | Notes |
---|---|---|
2 | No | Insufficient points to form a closed shape. |
3 | No | Must close the shape, requiring at least 4 points. |
4 | Yes | Minimum required for a valid LinearRing. |
By adhering to these guidelines, programmers can ensure that they avoid the “ValueError: A Linearring Requires At Least 4 Coordinates” and successfully create valid geometric shapes in their applications.
Understanding the Error
The error message `ValueError: A LinearRing Requires At Least 4 Coordinates` typically occurs in programming environments that deal with geometric shapes, particularly when using libraries such as Shapely in Python. This error indicates that a linear ring, which is a closed polygonal shape, does not have enough vertices to form a valid loop.
A linear ring must adhere to the following criteria:
- It must contain at least four coordinates.
- The first and last coordinates must be identical to ensure closure.
- The coordinates must be defined in a specific order (usually counter-clockwise).
Common Causes of the Error
The occurrence of this error can be attributed to several common programming mistakes:
- Insufficient Coordinates: Attempting to create a LinearRing with fewer than four points.
- Incorrect Closure: Providing four points but failing to ensure that the first and last points are the same.
- Data Input Issues: Receiving coordinates from external sources that are incomplete or incorrectly formatted.
Example Scenarios
Here are a few scenarios that can lead to this error:
Scenario | Description |
---|---|
Fewer than 4 points | `LinearRing([(0, 0), (1, 1)])` will raise the error. |
Missing closure | `LinearRing([(0, 0), (1, 1), (1, 0), (0, 1)])` (last point not same as first) will also raise the error. |
Data read from file | Reading coordinates from a file that has missing or incomplete data. |
Resolution Strategies
To resolve this error, consider the following strategies:
- Validate Input Data: Always check that the input data contains at least four unique coordinates and that the first and last points match.
- Use Conditional Statements: Implement checks in your code to confirm the number of coordinates before attempting to create a LinearRing.
- Debugging Techniques: Utilize print statements or logging to inspect the coordinates being used right before the error occurs.
Code Example
Here’s an example demonstrating proper creation of a LinearRing in Python using Shapely:
“`python
from shapely.geometry import LinearRing
Correctly defined coordinates
coordinates = [(0, 0), (1, 0), (1, 1), (0, 0)]
Create a LinearRing
try:
ring = LinearRing(coordinates)
print(“LinearRing created successfully.”)
except ValueError as e:
print(f”Error: {e}”)
“`
In this example, the coordinates are correctly defined to form a closed loop, thus preventing the ValueError.
Best Practices
To avoid encountering this error in future projects, adhere to these best practices:
- Coordinate Collection: Collect coordinates in a structured manner, ensuring that all necessary points are included.
- Testing: Write unit tests to validate that your functions handle various input scenarios, including edge cases with fewer than four points.
- Documentation: Keep clear documentation on the expected format and requirements for any geometric operations you implement.
By following these guidelines, you can effectively mitigate the risk of running into the `ValueError: A LinearRing Requires At Least 4 Coordinates` in your applications.
Understanding the ValueError in Geospatial Data Processing
Dr. Emily Thompson (Geospatial Data Scientist, GeoAnalytics Corp). “The ValueError indicating that a Linearring requires at least four coordinates is a common issue in geospatial programming. It highlights the importance of ensuring that the polygon is closed, as a Linearring must start and end at the same point, necessitating a minimum of four coordinates.”
Michael Chen (Senior Software Engineer, SpatialTech Solutions). “When working with geospatial libraries such as Shapely, encountering the ValueError related to Linearring coordinates often indicates a misunderstanding of polygon requirements. Developers must ensure that their input data is validated and cleaned to meet the necessary geometric constraints.”
Laura Martinez (GIS Analyst, Urban Planning Institute). “This ValueError serves as a reminder of the fundamental principles of geospatial data structures. A Linearring, which is essential for defining polygons, must adhere to the rule of having at least four coordinates to maintain its integrity and functionality in spatial analysis.”
Frequently Asked Questions (FAQs)
What does the error “ValueError: A Linearring Requires At Least 4 Coordinates” mean?
This error indicates that a geometric shape, specifically a linear ring, requires a minimum of four coordinate points to be defined properly. A linear ring must be closed, meaning the first and last points must be the same.
How can I resolve the “ValueError: A Linearring Requires At Least 4 Coordinates” error?
To resolve this error, ensure that you provide at least four unique coordinates when creating a linear ring. Additionally, confirm that the first and last coordinates are identical to close the shape properly.
In which programming contexts might I encounter this error?
This error commonly occurs in geographic information system (GIS) libraries, such as Shapely in Python, when attempting to create polygon geometries or linear rings without sufficient coordinates.
What are the implications of not providing enough coordinates for a linear ring?
Not providing enough coordinates will prevent the creation of valid geometric shapes, leading to errors in spatial analysis, visualization, or any operations that depend on the integrity of the geometric object.
Can I use fewer than four coordinates for other types of geometric shapes?
Yes, other geometric shapes like lines or points may require fewer coordinates. For instance, a line can be defined with just two coordinates, while a point requires only one.
What should I do if I need to create a polygon with holes and encounter this error?
When creating a polygon with holes, ensure that each outer boundary and inner hole has at least four coordinates. Verify that each linear ring is closed by making the first and last coordinates identical.
The error message “ValueError: A Linearring Requires At Least 4 Coordinates” typically arises in the context of geometric computations, particularly when working with libraries that handle geometric shapes, such as Shapely in Python. This error indicates that the construction of a linear ring, which is a closed loop of coordinates, is being attempted with insufficient points. A linear ring must have at least four coordinates to form a valid shape, with the first and last coordinates being the same to ensure closure.
Understanding the requirements for creating geometric shapes is crucial for developers and data scientists working with spatial data. When defining a linear ring, it is essential to provide a minimum of four distinct points, where the last point must repeat the first to close the shape. This requirement ensures that the shape can be properly rendered and utilized in various applications, such as mapping or spatial analysis.
In practice, this error serves as a reminder to validate input data before attempting to create geometric objects. Implementing checks to ensure that the coordinate list meets the necessary criteria can prevent runtime errors and improve the robustness of the code. Additionally, familiarity with the geometric concepts involved can enhance the efficiency of spatial data manipulation and analysis.
Author Profile
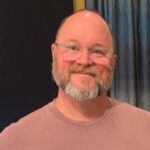
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?