How Can You Effectively Use Update With Inner Join in SQL?
In the world of relational databases, the ability to manipulate and update data efficiently is crucial for maintaining the integrity and relevance of information. One powerful technique that database administrators and developers often employ is the use of an INNER JOIN in SQL updates. This method not only streamlines the process of updating records across multiple tables but also ensures that the changes made are contextually relevant and accurately reflect the relationships between data sets. As organizations increasingly rely on complex databases to drive their operations, mastering this technique can significantly enhance your data management skills.
When updating records in SQL, you may encounter situations where the data you need to modify is spread across different tables. The INNER JOIN clause allows you to connect these tables based on a common attribute, enabling you to execute updates that are both precise and efficient. By leveraging this approach, you can ensure that your updates are not only targeted but also maintain the integrity of the relational structure of your database. Understanding how to implement INNER JOINs in update statements is essential for anyone looking to optimize their SQL proficiency.
In this article, we will delve into the intricacies of using INNER JOINs in SQL updates. We will explore the syntax, best practices, and common scenarios where this technique proves invaluable. Whether you are a seasoned SQL veteran or a newcomer eager
Understanding INNER JOIN in SQL
An INNER JOIN in SQL is a powerful tool that allows you to combine rows from two or more tables based on a related column between them. This means that only the rows with matching values in both tables will be included in the result set.
For example, consider two tables: `Customers` and `Orders`. The `Customers` table contains customer information, while the `Orders` table holds order details. To find all orders along with the corresponding customer names, you can use an INNER JOIN.
Here’s a basic syntax for an INNER JOIN:
“`sql
SELECT columns
FROM table1
INNER JOIN table2
ON table1.common_column = table2.common_column;
“`
In this syntax:
- `columns` represent the fields you want to retrieve.
- `table1` and `table2` are the names of the tables you are joining.
- `common_column` is the field that exists in both tables and is used for the join condition.
Updating Records with INNER JOIN
Updating records using INNER JOIN can be particularly useful when you need to modify data in one table based on the criteria from another. This approach ensures that only related records are updated, maintaining data integrity.
The SQL syntax for updating records with an INNER JOIN is as follows:
“`sql
UPDATE table1
SET table1.column_name = new_value
FROM table1
INNER JOIN table2
ON table1.common_column = table2.common_column
WHERE condition;
“`
In this syntax:
- `table1` is the table containing the records you want to update.
- `column_name` is the column in `table1` that you wish to modify.
- `new_value` is the new value you want to set.
- The `WHERE` clause is optional and can be used to filter the records further.
Example of Update with INNER JOIN
Assume we have the following two tables:
Customers Table
CustomerID | Name |
---|---|
1 | John Doe |
2 | Jane Smith |
Orders Table
OrderID | CustomerID | Status |
---|---|---|
100 | 1 | Pending |
101 | 2 | Shipped |
If you want to update the status of all orders placed by “John Doe” to “Completed”, the SQL query would look like this:
“`sql
UPDATE Orders
SET Status = ‘Completed’
FROM Orders
INNER JOIN Customers
ON Orders.CustomerID = Customers.CustomerID
WHERE Customers.Name = ‘John Doe’;
“`
This query joins the `Orders` and `Customers` tables on `CustomerID`, updating the status of John’s order to “Completed”.
Best Practices for Using INNER JOIN in Updates
When using INNER JOIN in update statements, consider the following best practices:
- Always use the appropriate JOIN type: Ensure INNER JOIN is the correct type for your use case, as it only includes matching records.
- Test your queries: Before executing an update, run a SELECT query with the same JOIN condition to confirm the records that will be affected.
- Backup your data: It’s wise to back up your database before performing bulk updates to prevent data loss.
- Limit updates: Use the WHERE clause to limit the scope of your updates to avoid unintended modifications.
By following these guidelines, you can efficiently and safely update records in your SQL databases.
Understanding Inner Join in SQL
Inner Join is a fundamental concept in SQL that allows you to combine rows from two or more tables based on a related column between them. When performing an update with an inner join, you can modify the values in one table based on values from another table.
Syntax for Update with Inner Join
The syntax for updating records using an inner join typically follows this structure:
“`sql
UPDATE Table1
SET Table1.ColumnName = new_value
FROM Table1
INNER JOIN Table2 ON Table1.CommonColumn = Table2.CommonColumn
WHERE Table2.ConditionColumn = some_value;
“`
- Table1: The primary table you want to update.
- ColumnName: The column in Table1 that will be updated.
- Table2: The table that provides the criteria for the update.
- CommonColumn: The column that is used to match records between Table1 and Table2.
- ConditionColumn: A column in Table2 that helps filter which records to update.
- new_value: The value that you want to set in ColumnName.
Example of Update with Inner Join
Consider two tables: `Employees` and `Departments`. You want to update the `Salary` of employees based on their department’s budget.
“`sql
UPDATE Employees
SET Employees.Salary = Employees.Salary * 1.10
FROM Employees
INNER JOIN Departments ON Employees.DepartmentID = Departments.ID
WHERE Departments.Budget > 50000;
“`
In this example:
- Employees with a matching `DepartmentID` in the Departments table are updated.
- Their salary is increased by 10% if their department’s budget exceeds 50,000.
Common Use Cases
Using an inner join in an update statement is particularly beneficial in scenarios such as:
- Synchronizing Data: Updating records in one table based on data from another.
- Conditional Updates: Applying updates conditionally based on related table values.
- Bulk Updates: Efficiently updating multiple records based on join conditions.
Performance Considerations
When using inner joins in update statements, consider the following:
- Indexing: Ensure that the columns used for joining are indexed to improve performance.
- Data Volume: Large datasets can lead to slow updates; consider limiting the scope with WHERE clauses.
- Transaction Management: Use transactions to maintain data integrity, especially when updating multiple rows.
Consideration | Best Practice |
---|---|
Indexing | Index join columns for faster access |
Data Volume | Filter rows to minimize updates |
Transactions | Use transactions for critical updates |
Limitations
When executing updates with inner joins, be aware of the following limitations:
- Row Count: Ensure that the inner join does not produce an unexpected number of rows for update.
- Permissions: Ensure you have the necessary permissions to update the target table.
- Data Type Compatibility: Ensure data types match when setting new values.
By understanding these aspects of updating with inner joins, you can effectively manage and manipulate your SQL databases.
Expert Insights on Using Update With Inner Join in SQL
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “Using an INNER JOIN in an UPDATE statement allows for precise modifications across multiple tables. It is essential to ensure that the join conditions are correctly defined to avoid unintentional data alterations.”
Mark Thompson (Senior SQL Developer, Data Solutions Group). “When performing an UPDATE with INNER JOIN, it is crucial to understand the implications on performance. Proper indexing on the joined columns can significantly enhance execution speed and efficiency.”
Linda Martinez (Data Analyst, Analytics Pro). “Incorporating INNER JOIN in your UPDATE queries not only streamlines data manipulation but also maintains data integrity. It is advisable to test your queries in a safe environment before executing them in production.”
Frequently Asked Questions (FAQs)
What is an INNER JOIN in SQL?
An INNER JOIN is a type of join that retrieves records from two or more tables where there is a match based on a related column between them. It only returns rows that have corresponding values in both tables.
How do I perform an UPDATE with an INNER JOIN in SQL?
To perform an UPDATE with an INNER JOIN, you can use the following syntax:
“`sql
UPDATE table1
SET table1.column = new_value
FROM table1
INNER JOIN table2 ON table1.common_column = table2.common_column
WHERE table2.condition_column = condition_value;
“`
This updates records in `table1` based on matching records in `table2`.
Can I update multiple tables using INNER JOIN in SQL?
No, SQL does not allow updating multiple tables in a single UPDATE statement. You can only update one table at a time, even if you use INNER JOIN to reference another table for conditions.
What happens if there are no matching records in an INNER JOIN during an UPDATE?
If there are no matching records in the INNER JOIN, the UPDATE statement will not affect any rows in the target table. Only rows that meet the join condition will be updated.
Are there performance considerations when using INNER JOIN in an UPDATE statement?
Yes, using INNER JOIN in an UPDATE statement can impact performance, especially with large datasets. Proper indexing on the join columns can significantly improve execution time.
Can I use WHERE clauses with INNER JOIN in an UPDATE statement?
Yes, you can use WHERE clauses in conjunction with INNER JOIN in an UPDATE statement. This allows for further filtering of the records that will be updated based on additional conditions.
In summary, the use of the UPDATE statement in conjunction with INNER JOIN in SQL is a powerful technique that allows for the modification of records in one table based on the related data in another table. This method is particularly useful when dealing with normalized databases where data is spread across multiple tables. By leveraging INNER JOIN, users can ensure that updates are made only to those records that meet specific criteria, thereby maintaining data integrity and consistency.
One of the key insights from the discussion is the importance of understanding the relationship between the tables involved in the update operation. Properly defining the join conditions is crucial, as it directly affects which records are updated. Additionally, it is essential to be cautious when performing updates, as unintended changes can occur if the join conditions are not precise. Testing queries in a safe environment before executing them on production data is advisable to prevent data loss or corruption.
Furthermore, the performance implications of using INNER JOIN in UPDATE statements should not be overlooked. While this approach can streamline the update process by combining multiple operations into one, it may also lead to increased complexity in query optimization. Therefore, database administrators and developers should monitor performance and consider indexing strategies to enhance execution speed, especially in large datasets.
Author Profile
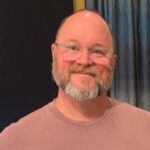
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?