How Can You Rename a Field in TypeScript While Preserving JSDoc Annotations?
In the ever-evolving landscape of web development, TypeScript has emerged as a powerful ally for developers seeking to enhance their JavaScript projects with strong typing and improved tooling. However, as codebases grow and evolve, the need to refactor and rename fields becomes inevitable. While renaming fields can streamline code and improve clarity, it often poses a challenge: how to retain the valuable documentation provided by JSDoc comments. This article delves into the nuances of renaming fields in TypeScript while preserving the integrity of your JSDoc annotations, ensuring that your code remains both clean and well-documented.
Renaming fields in TypeScript can be a straightforward process, but it requires careful consideration to avoid losing the context provided by JSDoc comments. JSDoc serves as a vital resource for developers, offering insights into the purpose and usage of various fields, functions, and classes. When a field is renamed, the associated documentation must be updated accordingly to maintain its relevance. However, there are strategies and tools available that can help streamline this process, allowing developers to focus on writing high-quality code without sacrificing the clarity of their documentation.
In this article, we will explore best practices for renaming fields in TypeScript while ensuring that JSDoc comments remain intact and
Understanding JSDoc in TypeScript
JSDoc is a powerful tool for documenting JavaScript code, and it is equally useful in TypeScript. It allows developers to generate documentation from comments within the code, enhancing clarity and maintainability. When renaming fields in TypeScript, preserving the JSDoc comments can help retain valuable context and descriptions associated with the original field.
To effectively manage JSDoc comments during a field rename, consider the following:
- Consistency: Ensure that the JSDoc comments are updated to reflect any changes in the field name.
- Clarity: Maintain clear and descriptive comments that accurately represent the purpose and usage of the field.
Strategies for Renaming Fields While Preserving JSDoc
When renaming fields in TypeScript, developers should adopt specific strategies to ensure that JSDoc comments remain intact and relevant. Here are some effective approaches:
- Refactor Tools: Utilize IDE features that support refactoring. Most modern IDEs, such as Visual Studio Code or WebStorm, allow you to rename fields while automatically updating references and preserving comments.
- Manual Update: If automated tools are not available, manually review and update the JSDoc comments following the rename to ensure they match the new field name and context.
- Version Control: Use version control systems like Git to track changes. Commit the rename and documentation updates separately to maintain a clear history of changes.
Example of Renaming a Field
Consider the following TypeScript interface with a JSDoc comment:
“`typescript
/**
- Represents a user in the system.
*/
interface User {
/** The unique identifier for the user. */
id: number;
/** The user’s full name. */
name: string;
}
“`
If we decide to rename the `name` field to `fullName`, the updated code should look like this:
“`typescript
/**
- Represents a user in the system.
*/
interface User {
/** The unique identifier for the user. */
id: number;
/** The user’s full name. */
fullName: string; // Updated field name
}
“`
Impact of Renaming on Code and Documentation
Renaming fields can have several implications on both the codebase and the documentation. Here’s a breakdown of these impacts:
Aspect | Impact |
---|---|
Code Consistency | Maintaining consistent naming conventions can enhance readability and reduce confusion. |
Documentation Integrity | Preserving and updating JSDoc comments ensures that documentation remains accurate and useful. |
Refactoring Complexity | Using automated tools can significantly reduce the complexity of refactoring, minimizing the risk of errors. |
By following these strategies and understanding the implications of renaming fields, developers can ensure that the transition is smooth and that the integrity of the code and its documentation is maintained.
Renaming Fields in TypeScript While Preserving JSDoc Comments
Renaming fields in TypeScript can be straightforward, but maintaining JSDoc comments during this process requires careful handling. Here’s how to achieve this effectively.
Method 1: Manual Renaming with Copy-Paste
This method involves manually renaming the field while ensuring you copy the associated JSDoc comments.
- Identify the Field: Locate the field you want to rename in your TypeScript file.
- Copy JSDoc Comments: Before renaming, copy any existing JSDoc comments above the field.
- Rename the Field: Change the field name.
- Reinsert JSDoc Comments: Paste the copied JSDoc comments above the newly renamed field.
Example:
“`typescript
/**
- The user’s name.
*/
let username: string = ‘JohnDoe’;
// Rename ‘username’ to ‘userName’
/**
- The user’s name.
*/
let userName: string = ‘JohnDoe’;
“`
Method 2: Using IDE Refactoring Tools
Modern IDEs provide refactoring tools that can rename fields while preserving JSDoc comments automatically.
- Visual Studio Code:
- Right-click on the field name and select “Rename Symbol” or use the shortcut `F2`.
- Type the new name, and the IDE will handle the JSDoc comments for you.
- WebStorm:
- Place the cursor on the field name and press `Shift + F6`.
- Enter the new field name, and all references, including JSDoc comments, will be preserved.
Considerations When Renaming Fields
When renaming fields, consider the following:
- Consistency: Ensure consistent naming conventions throughout your codebase.
- Impact on References: Renaming a field will affect all references in the code. Use refactoring tools to update them automatically.
- Testing: After renaming, run existing tests to ensure that the functionality remains intact.
Best Practices for JSDoc Comments
To ensure that JSDoc comments remain useful and clear after renaming fields, follow these best practices:
- Use Descriptive Comments: Ensure JSDoc comments clearly describe the purpose and use of the field.
- Update Comments: After renaming, verify that the comments still accurately reflect the field’s role.
- Consistency in Documentation: Maintain a consistent style for all JSDoc comments across your project.
JSDoc Tag | Description |
---|---|
`@param` | Documents a function parameter. |
`@returns` | Documents the return value of a function. |
`@deprecated` | Indicates that a method or field is deprecated. |
By following these methods and best practices, you can efficiently rename fields in TypeScript while ensuring that JSDoc comments are preserved and remain relevant.
Expert Insights on Renaming Fields in TypeScript While Preserving JSDoc Comments
Dr. Emily Carter (Senior Software Engineer, TypeScript Innovations). “When renaming fields in TypeScript, it is crucial to ensure that JSDoc comments are maintained to preserve the documentation integrity. Utilizing tools like TypeScript’s refactoring capabilities can help automate this process, but developers must manually verify that JSDoc annotations remain accurate and relevant after the rename.”
Michael Chen (Lead Developer Advocate, CodeCraft). “Renaming fields while keeping JSDoc intact is a best practice that enhances code readability and maintainability. Developers should adopt a systematic approach, such as using IDE features that allow for renaming with JSDoc preservation, to streamline their workflow and avoid potential documentation loss.”
Sarah Thompson (Technical Writer, DevDocs). “The importance of JSDoc comments cannot be overstated, especially when refactoring code. When renaming fields in TypeScript, it is advisable to review JSDoc annotations to ensure they accurately describe the new field names. This practice not only aids in comprehension but also ensures that future developers can easily navigate the codebase.”
Frequently Asked Questions (FAQs)
What is the process to rename a field in TypeScript while preserving JSDoc comments?
To rename a field in TypeScript while keeping the JSDoc comments, you can copy the JSDoc comment above the field, rename the field, and then paste the JSDoc comment back above the new field name. This ensures that the documentation remains associated with the renamed field.
Can I use IDE features to help with renaming fields in TypeScript?
Yes, most modern IDEs, such as Visual Studio Code, provide refactoring tools that allow you to rename fields. These tools typically preserve JSDoc comments automatically, making the process more efficient.
What happens to JSDoc comments if I manually rename a field?
If you manually rename a field without copying the JSDoc comments, those comments will not automatically transfer to the new field name. This may lead to documentation being out of sync with the code.
Is there a TypeScript-specific way to ensure JSDoc comments stay with renamed fields?
TypeScript does not have a built-in feature specifically for this purpose. However, using IDE refactoring tools is the best practice, as they are designed to handle such cases effectively.
Are there any best practices for maintaining JSDoc comments during field renaming?
Best practices include using IDE refactoring tools, always reviewing JSDoc comments after renaming, and ensuring comments are clear and relevant to the new field name.
What should I do if the JSDoc comments are lost during the renaming process?
If JSDoc comments are lost during renaming, you should check your version control system to revert to a previous version or manually rewrite the comments based on the original context and functionality of the field.
In TypeScript, renaming a field while preserving its associated JSDoc comments is a common task that developers encounter. This process is essential for maintaining code clarity and documentation integrity, especially when refactoring or updating codebases. The ability to rename fields without losing the contextual information provided by JSDoc enhances code maintainability and ensures that the documentation remains relevant and accessible to other developers.
One effective approach to achieve this is by utilizing TypeScript’s built-in features, such as the `as` keyword for type assertions or leveraging IDEs that support refactoring tools. These tools often allow developers to rename fields seamlessly, automatically updating the JSDoc comments as needed. This not only saves time but also minimizes the risk of errors that may arise from manually updating documentation after a field rename.
Overall, understanding how to rename fields in TypeScript while keeping JSDoc intact is crucial for developers aiming to write clean, maintainable code. By employing the right tools and techniques, developers can ensure that their code remains well-documented and easy to navigate, ultimately leading to better collaboration and efficiency within development teams.
Author Profile
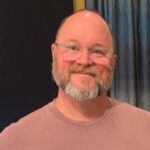
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?