How to Resolve TypeError: NetworkError When Attempting to Fetch Resource?
In the ever-evolving landscape of web development, encountering errors is an inevitable part of the journey. Among the myriad of issues that developers face, the `TypeError: NetworkError when attempting to fetch resource` stands out as a particularly perplexing challenge. This error not only disrupts the flow of application functionality but also raises questions about the underlying network interactions that power modern web applications. As developers strive to create seamless user experiences, understanding this error becomes crucial for troubleshooting and enhancing performance.
When you see the `TypeError: NetworkError when attempting to fetch resource`, it signals a breakdown in the communication between your application and the server. This error can arise from various factors, including incorrect URLs, server-side issues, or even problems with the browser’s handling of requests. For developers, pinpointing the root cause can be a daunting task, as these errors often manifest without clear indications of what went wrong.
In the following sections, we will delve into the common scenarios that trigger this error, explore effective debugging strategies, and provide insights into best practices for preventing such issues in the future. Whether you’re a seasoned developer or just starting in the world of web applications, understanding the intricacies of this error will empower you to build more robust and resilient applications.
Understanding the Error
The `TypeError: NetworkError when attempting to fetch resource` typically indicates that a network request made via the Fetch API has failed. This can occur for several reasons, which can be broadly categorized into client-side issues, server-side issues, and network issues. Understanding these causes is critical for troubleshooting and resolving the error effectively.
Common Causes
- CORS Issues: Cross-Origin Resource Sharing (CORS) policies can block requests from different origins. If the server does not permit cross-origin requests, a network error will be thrown.
- Network Connectivity: Issues with internet connectivity, such as being offline or having a weak connection, can prevent the resource from being fetched.
- URL Errors: Incorrect or malformed URLs can lead to failed requests. This can include typos or missing protocol specifications (e.g., http or https).
- Server Unavailability: The requested resource may be hosted on a server that is down or not reachable, resulting in a network error.
Troubleshooting Steps
To address the `TypeError: NetworkError when attempting to fetch resource`, consider the following troubleshooting steps:
- Check the URL:
- Verify that the URL is correct and accessible.
- Ensure that the correct protocol (HTTP/HTTPS) is used.
- Inspect CORS Configuration:
- Confirm that the server is configured to allow requests from the origin of your application.
- Use browser developer tools to check for CORS-related errors in the console.
- Monitor Network Status:
- Test your internet connection to ensure it is stable and working.
- Use network diagnostics tools to check for issues.
- Review Server Logs:
- If you have access, check the server logs for errors that might indicate why the resource is unavailable.
- Handle Errors Gracefully:
- Implement error handling in your fetch requests to manage and log errors more effectively.
Error Handling Example
Implementing proper error handling can help manage errors gracefully in your application. Here’s a sample code snippet demonstrating how to handle fetch errors:
“`javascript
fetch(‘https://example.com/data’)
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => {
console.error(‘Fetch error:’, error);
});
“`
Best Practices
To minimize the chances of encountering the `TypeError: NetworkError when attempting to fetch resource`, consider the following best practices:
- Use HTTPS: Always prefer secure connections to enhance security and reduce the likelihood of network errors.
- Implement Retry Logic: For transient network issues, implementing a retry mechanism can help recover from temporary failures.
- Optimize CORS Settings: Configure CORS properly to allow only necessary origins while maintaining security.
Summary of Common Causes and Solutions
Cause | Solution |
---|---|
CORS Issues | Check server settings and adjust CORS configuration. |
Network Connectivity | Ensure stable internet connection. |
URL Errors | Verify and correct the URL. |
Server Unavailability | Check server status and logs. |
Understanding the TypeError
A `TypeError` in JavaScript typically occurs when a value is not of the expected type. This error may arise during operations involving objects, arrays, or function calls. When combined with a network error during a fetch operation, it generally indicates that the response received is not what the application anticipates.
Common Causes of TypeError with Fetch:
- Invalid URL: If the URL passed to the fetch function is malformed or unreachable, the fetch will fail.
- Response Parsing: Attempting to parse a response as JSON when it is not in valid JSON format will trigger a TypeError.
- CORS Issues: Cross-Origin Resource Sharing (CORS) policy violations can lead to network errors, which might be misinterpreted as TypeErrors.
- Network Issues: A lack of internet connectivity or server downtime can cause fetch requests to fail.
Diagnosing Network Errors
Network errors occur when a fetch request cannot be completed due to issues outside of the code itself. Diagnosing these errors involves several steps:
- Check the Network Tab:
- Open developer tools in your browser.
- Navigate to the ‘Network’ tab.
- Observe the status of the fetch request to identify if it’s being blocked or failing.
- Inspect CORS Policy:
- Ensure the server you are trying to access allows cross-origin requests.
- Check for the presence of appropriate CORS headers in the server response.
- Validate the API Endpoint:
- Confirm that the API endpoint is correct and operational by testing it in a browser or using tools like Postman.
- Review Application Logs:
- Look at the console for additional error messages that might provide context to the fetch failure.
Handling Fetch Errors Gracefully
To improve user experience and maintain application robustness, handle fetch errors effectively using the following strategies:
– **Implement Try-Catch Blocks**:
Enclose your fetch calls in try-catch statements to catch errors and handle them appropriately.
“`javascript
try {
const response = await fetch(url);
if (!response.ok) throw new TypeError(‘Network response was not ok’);
const data = await response.json();
} catch (error) {
console.error(‘Fetch error:’, error);
}
“`
– **User Notifications**:
Provide feedback to users when a network error occurs. This can be done through alerts or UI notifications.
– **Retry Logic**:
Introduce logic to retry failed requests after a certain period or a specified number of attempts.
“`javascript
async function fetchWithRetry(url, options, retries = 3) {
try {
const response = await fetch(url, options);
if (!response.ok) throw new TypeError(‘Network response was not ok’);
return await response.json();
} catch (error) {
if (retries > 0) {
return fetchWithRetry(url, options, retries – 1);
}
throw error;
}
}
“`
Best Practices for Fetch API Usage
To minimize the occurrence of `TypeError` and network errors, adhere to the following best practices:
Practice | Description |
---|---|
Validate URLs | Always ensure that URLs are well-formed and reachable. |
Use async/await | Simplifies code readability and error handling with asynchronous operations. |
Handle all response types | Always check the type of response received and handle accordingly. |
Monitor network status | Implement checks for connectivity before making fetch requests. |
Log errors | Maintain logs for troubleshooting issues that arise in production. |
By following these guidelines, you can effectively manage and reduce the likelihood of encountering `TypeError` and network errors during fetch operations.
Understanding TypeError: NetworkError When Attempting to Fetch Resource
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A TypeError indicating a NetworkError typically arises when a fetch request fails due to network issues or incorrect URLs. It is crucial to validate the endpoint and ensure that the server is reachable to prevent such errors.”
James Liu (Web Development Consultant, Digital Solutions Group). “In my experience, encountering a TypeError: NetworkError often points to CORS (Cross-Origin Resource Sharing) issues. Developers should ensure that the server is configured to allow requests from the origin of the client application.”
Sarah Thompson (Lead Frontend Developer, CodeCraft Agency). “This error can also be a symptom of improper handling of promises in JavaScript. It is essential to implement robust error handling in your fetch requests to gracefully manage scenarios where resources cannot be fetched.”
Frequently Asked Questions (FAQs)
What does “TypeError: NetworkError when attempting to fetch resource” mean?
This error indicates that a network-related issue occurred while trying to fetch a resource, such as an API or a file. It typically arises due to problems like a failed request, incorrect URL, or server unavailability.
What are common causes of this error?
Common causes include incorrect API endpoints, CORS (Cross-Origin Resource Sharing) policy violations, server downtime, network connectivity issues, or the resource being blocked by a firewall.
How can I troubleshoot this error?
To troubleshoot, check the URL for accuracy, ensure the server is running, inspect network conditions, and review the browser console for CORS-related messages. Testing the request in a tool like Postman can also help isolate the issue.
Is this error specific to certain browsers?
No, this error can occur across different browsers. However, the way each browser handles CORS and network requests may lead to variations in how the error is reported.
Can this error occur in production environments?
Yes, this error can occur in production environments, especially if there are changes in server configurations, network settings, or if the API endpoints are modified without proper updates in the application.
What steps can I take to prevent this error in the future?
To prevent this error, implement robust error handling in your code, validate API endpoints, monitor server status, and ensure proper CORS settings are configured. Regularly testing your application can also help identify potential issues early.
The error message “TypeError: NetworkError when attempting to fetch resource” typically indicates that there is an issue with network connectivity or the resource being requested is unavailable. This error can arise in various scenarios, such as when attempting to fetch data from a server, accessing APIs, or loading external resources like scripts or stylesheets. Understanding the underlying causes of this error is crucial for developers to troubleshoot and resolve it effectively.
Common causes of this error include incorrect URLs, CORS (Cross-Origin Resource Sharing) issues, server downtime, or network interruptions. Developers should ensure that the URLs used in fetch requests are correct and that the server is configured to allow cross-origin requests if applicable. Additionally, checking the server status and network conditions can help identify whether the issue is temporary or persistent.
To mitigate the occurrence of this error, developers can implement error handling in their code to manage exceptions gracefully. Utilizing tools such as network monitoring and debugging can provide insights into the request and response cycle, helping to pinpoint the exact cause of the error. Furthermore, keeping abreast of best practices for API consumption and resource loading can enhance the robustness of applications and reduce the likelihood of encountering this error.
Author Profile
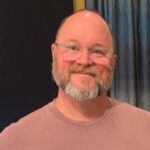
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?