Why Is My Expression Ambiguous Without a Type Annotation?
In the realm of programming languages, clarity and precision are paramount. Yet, even the most seasoned developers can find themselves grappling with the complexities of type systems. One particularly perplexing scenario arises when we encounter expressions that are ambiguous without a type annotation. This situation not only challenges our understanding of how types interact but also underscores the importance of explicitness in coding practices. As we delve into this topic, we’ll explore the implications of ambiguous expressions, the role of type annotations, and how they can enhance both code readability and maintainability.
When an expression lacks a definitive type annotation, it can lead to confusion and potential errors during compilation or runtime. This ambiguity often stems from the flexibility of dynamically typed languages or the intricacies of type inference in statically typed languages. Without a clear indication of what type an expression should be, developers face the risk of misinterpretation, which can result in unintended behavior or bugs that are difficult to trace. By examining the nuances of type ambiguity, we can better appreciate the significance of type annotations in ensuring that our code communicates its intentions effectively.
Moreover, the practice of employing type annotations not only aids in resolving ambiguity but also serves as a form of documentation for future reference. It provides context for other developers (or even our future selves) who may interact
Understanding Ambiguous Expressions
Ambiguous expressions in programming can lead to unexpected behaviors, particularly in languages with strong static typing. When the type of an expression cannot be clearly determined by the compiler or interpreter, it often requires explicit type annotations to resolve any uncertainty. This situation typically arises when dealing with complex data structures or functions where types may not be evident.
For example, consider a function that returns a value based on a condition:
“`python
def get_value(condition):
if condition:
return 42 Integer
else:
return “Hello” String
“`
In the above function, the return type is ambiguous because it can either be an integer or a string, depending on the input condition. Without a type annotation, the compiler may raise warnings or errors about the ambiguous return type.
Type Annotations to Resolve Ambiguity
Type annotations provide a clear specification of expected data types, helping to eliminate ambiguity. By explicitly stating the types of function parameters and return values, developers can improve code clarity and maintainability.
Consider the following example with type annotations:
“`python
def get_value(condition: bool) -> Union[int, str]:
if condition:
return 42
else:
return “Hello”
“`
In this code snippet, the `Union[int, str]` annotation indicates that the return value can be either an integer or a string, thus providing clarity to anyone reading the code.
Common Scenarios for Ambiguous Expressions
Ambiguity often occurs in various programming contexts, including:
- Function Overloading: When multiple functions have the same name but different parameters, it may be unclear which function is being invoked without proper annotations.
- Generics: In languages that support generics, such as TypeScript or C, failing to specify type parameters can result in ambiguous types.
- Dynamic Typing: Languages like Python allow dynamic typing, which can introduce ambiguity if types are not annotated.
Best Practices for Avoiding Ambiguity
To mitigate ambiguity in expressions, consider the following best practices:
- Use type annotations consistently across your codebase.
- Implement static type checking tools such as MyPy for Python or TypeScript’s compiler.
- Document the expected types in your functions, especially when they return complex data structures.
Practice | Description |
---|---|
Consistent Type Annotations | Apply type annotations uniformly to enhance readability and maintainability. |
Static Type Checking | Utilize tools to catch type-related issues before runtime. |
Comprehensive Documentation | Provide detailed documentation regarding the expected types to aid other developers. |
By adhering to these practices, developers can effectively manage ambiguity in expressions, leading to more robust and reliable code.
Understanding Ambiguous Expressions in Programming
Ambiguous expressions often arise in programming languages that utilize type inference, particularly in languages like Python, JavaScript, or TypeScript. An ambiguous expression occurs when the compiler or interpreter cannot determine the type of a variable or expression without additional information. This can lead to errors or unexpected behavior if not handled properly.
Common Scenarios Leading to Ambiguity
Several scenarios can lead to type ambiguity in code:
- Dynamic Typing: Languages that allow variables to change types can create ambiguity.
- Function Overloading: When multiple functions share the same name but differ in parameter types, it may be unclear which function to invoke.
- Default Values: Functions with default parameters may lead to confusion if the types are not specified.
- Empty Returns: Functions that return `null` or “ without explicit type definitions can introduce ambiguity.
Type Annotations: A Solution to Ambiguity
Type annotations provide a means to explicitly define the expected types of variables and function parameters. This practice enhances code readability and helps prevent runtime errors. Below are some key benefits of using type annotations:
- Improved Readability: Clearly defined types make it easier for others (or yourself) to understand the expected behavior of the code.
- Error Prevention: Early detection of type-related errors during development rather than at runtime.
- Documentation: Serving as a form of inline documentation, type annotations clarify the intended use of variables and functions.
Examples of Type Annotations
The following table illustrates how type annotations can be implemented in various programming languages:
Language | Example Code | Explanation |
---|---|---|
TypeScript | `let x: number = 5;` | Specifies that `x` is of type number. |
Python | `def add(a: int, b: int) -> int:` | Indicates function parameters and return type. |
Java | `String name = “John”;` | Declares a variable of type String. |
C | `public int Calculate(int a, int b)` | The method explicitly takes integer parameters. |
Best Practices for Type Annotations
To effectively utilize type annotations, consider the following best practices:
- Use Consistent Naming Conventions: Clear and consistent naming helps convey the purpose of variables and functions.
- Annotate Complex Types: When using complex data structures, provide clear annotations to avoid ambiguity.
- Review Annotations Regularly: As code evolves, ensure that type annotations remain accurate and relevant.
- Leverage Type Inference Where Appropriate: While annotations are beneficial, avoid over-annotating simple expressions where type inference is clear.
Utilizing type annotations is essential in modern programming to prevent ambiguity and enhance code quality. By adopting these practices, developers can create clearer, more maintainable code that reduces the likelihood of runtime errors.
Understanding Ambiguity in Type Expressions: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In programming languages, when a type of expression is ambiguous without a type annotation, it can lead to significant runtime errors. Developers must implement explicit type annotations to ensure clarity and maintainability in their code.”
Michael Chen (Lead Data Scientist, Data Insights Group). “Ambiguous type expressions can hinder the effectiveness of type inference systems. By providing type annotations, we not only enhance the readability of our code but also improve the performance of static analysis tools.”
Sarah Patel (Programming Language Researcher, Institute of Software Studies). “The lack of type annotations in expressions can lead to misinterpretations by compilers or interpreters. This ambiguity can result in unexpected behavior, making it crucial for developers to embrace strong typing practices.”
Frequently Asked Questions (FAQs)
What does it mean when an expression is ambiguous without a type annotation?
An expression is considered ambiguous without a type annotation when the programming language cannot determine its type based solely on the context in which it is used. This often leads to compilation errors or runtime issues.
Why are type annotations important in programming?
Type annotations provide explicit information about the expected data types of variables and expressions, which enhances code readability, enables better error checking, and improves performance by allowing compilers to optimize code more effectively.
In which programming languages is type annotation commonly used?
Type annotations are commonly used in statically typed languages such as Java, C, and TypeScript, as well as in dynamically typed languages like Python, where optional type hints can be provided for clarity.
How can I resolve ambiguity in an expression without a type annotation?
To resolve ambiguity, you can explicitly specify the type of the expression using a type annotation. This clarifies the intended type to the compiler or interpreter, thus eliminating any confusion.
What are the consequences of leaving expressions ambiguous in code?
Leaving expressions ambiguous can lead to unexpected behavior, runtime errors, and difficulties in maintaining or debugging the code. It can also hinder collaboration among developers who may misinterpret the intended use of the expression.
Can type inference help with ambiguous expressions?
Yes, type inference can help with ambiguous expressions by allowing the compiler to deduce the type based on the context in which the expression is used. However, it may not always be sufficient, and explicit type annotations are often recommended for clarity.
In programming, particularly in languages with strong type systems, expressions can often become ambiguous without explicit type annotations. This ambiguity arises when the compiler or interpreter is unable to infer the type of a variable or expression based solely on its context. Without clear type definitions, developers may encounter unexpected behaviors, runtime errors, or difficulties in code maintenance, highlighting the importance of type annotations in ensuring clarity and correctness in code execution.
One of the key takeaways from this discussion is that type annotations serve as a critical tool for enhancing code readability and maintainability. By explicitly defining types, developers can communicate their intentions more clearly, making it easier for others (or themselves at a later date) to understand the expected data types and the operations that can be performed on them. This practice not only aids in reducing errors but also facilitates better collaboration among team members who may work on the same codebase.
Moreover, the use of type annotations can significantly improve the development experience by enabling better tooling support. Many modern integrated development environments (IDEs) and static analysis tools leverage type information to provide features such as autocompletion, error checking, and refactoring capabilities. As a result, adopting type annotations can lead to increased productivity and a smoother development workflow, ultimately contributing
Author Profile
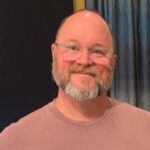
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?