Why Does System.IO.Ports.SerialPort Fail to Open with a USB Virtual COM Port?
In the realm of modern computing, the seamless communication between devices is paramount, especially when it comes to serial ports. As technology evolves, the use of USB virtual COM ports has surged, offering a convenient bridge between traditional serial communication and contemporary USB interfaces. However, many developers and engineers encounter a frustrating hurdle: the infamous failure to open a `System.IO.Ports.SerialPort` when attempting to connect through these virtual ports. This article delves into the intricacies of this issue, exploring its causes, implications, and potential solutions to help you navigate this common yet perplexing challenge.
The `System.IO.Ports.SerialPort` class in .NET provides a robust framework for serial communication, but when paired with USB virtual COM ports, users often face unexpected roadblocks. These failures can stem from a variety of factors, including driver incompatibilities, port configurations, or even hardware limitations. Understanding the underlying mechanics of how serial ports interact with USB technology is essential for troubleshooting and resolving these issues effectively.
As we unpack the complexities surrounding the failure to open a `SerialPort`, we will examine common error messages, diagnostic steps, and best practices for ensuring successful communication. Whether you are a seasoned developer or a newcomer to the world of serial communication, this exploration will equip you with
Common Causes for Serial Port Connection Failures
When attempting to open a USB virtual COM port using `System.IO.Ports.SerialPort`, various issues can prevent a successful connection. Understanding these common causes is essential for troubleshooting the problem effectively.
- Driver Issues: The most frequent cause of connection failures is improper or outdated drivers. Ensure that the USB-to-serial adapter drivers are correctly installed and up to date.
- Port Availability: The specified COM port may already be in use by another application or process. Check the device manager to confirm that the port is not occupied.
- Permissions: Lack of necessary permissions can hinder the ability to open the port. Ensure that your application has the required access rights, particularly on operating systems with strict security policies.
- Incorrect Port Settings: Mismatched settings such as baud rate, parity, data bits, and stop bits can lead to failed connections. Confirm that the settings match those expected by the connected device.
- Hardware Malfunctions: Physical issues with the USB port, cable, or the device itself can also cause failures. Inspect the hardware for any visible signs of damage.
Troubleshooting Steps
To resolve issues related to opening a USB virtual COM port, follow these troubleshooting steps:
- Verify Driver Installation: Check the device manager to ensure that the drivers for the USB-to-serial adapter are properly installed.
- Check Port Status: Use the Windows device manager to confirm that the COM port is not in use or disabled.
- Adjust Permissions: Run your application with elevated privileges to ensure it has the necessary access rights.
- Review Connection Settings: Double-check the serial port parameters in your code to ensure they align with the device specifications.
- Test Hardware: Swap cables or try a different USB port to rule out hardware issues.
Example Code Snippet
The following example demonstrates how to open a serial port using `System.IO.Ports.SerialPort`. Ensure that the port name and settings are correctly configured:
“`csharp
using System;
using System.IO.Ports;
class Program
{
static void Main()
{
SerialPort serialPort = new SerialPort(“COM3”, 9600, Parity.None, 8, StopBits.One);
try
{
serialPort.Open();
Console.WriteLine(“Port opened successfully.”);
}
catch (UnauthorizedAccessException ex)
{
Console.WriteLine(“Access denied: ” + ex.Message);
}
catch (IOException ex)
{
Console.WriteLine(“I/O Error: ” + ex.Message);
}
catch (Exception ex)
{
Console.WriteLine(“Error: ” + ex.Message);
}
finally
{
if (serialPort.IsOpen)
{
serialPort.Close();
Console.WriteLine(“Port closed.”);
}
}
}
}
“`
Best Practices for Serial Port Management
Employing best practices can mitigate many issues associated with serial port management:
- Always Close the Port: Ensure that the port is closed in a `finally` block to avoid leaving it in an open state.
- Use Try-Catch for Exception Handling: Implement robust exception handling to manage potential errors gracefully.
- Monitor Port Usage: Regularly check which applications are using COM ports to prevent conflicts.
- Test with Different Devices: If possible, test the connection with multiple devices to identify whether the issue is device-specific.
Issue | Possible Solution |
---|---|
Driver Issues | Update or reinstall drivers |
Port Already in Use | Close the conflicting application |
Permission Denied | Run as Administrator |
Incorrect Settings | Verify and adjust settings |
Hardware Failure | Inspect and replace hardware if necessary |
Common Causes of SerialPort Open Failures
When encountering issues with opening a `System.IO.Ports.SerialPort` connected via a USB virtual COM port, several common causes may be responsible. Understanding these factors can assist in troubleshooting effectively.
- Port Already in Use: If another application is currently using the COM port, attempts to open it will fail.
- Driver Issues: Outdated or incompatible drivers for the USB virtual COM port can lead to connection problems.
- Incorrect Port Configuration: Mismatched settings, such as baud rate, parity, data bits, and stop bits, can prevent successful communication.
- Permissions: Insufficient permissions can restrict access to the COM port. Ensure the application has the necessary rights.
- Device Disconnection: Physical disconnection or malfunctioning of the USB device may render the port inaccessible.
Troubleshooting Steps
To resolve the issue of a `SerialPort` failing to open, consider the following troubleshooting steps:
- Check for Active Connections:
- Use tools like Device Manager to see if the port is listed as in use.
- Close any applications that might be accessing the port.
- Verify Driver Installation:
- Check the Device Manager for the status of the USB virtual COM port drivers.
- Update or reinstall drivers if necessary.
- Examine Port Settings:
- Ensure the `SerialPort` configuration matches the device specifications:
- Baud Rate: 9600 (or as specified)
- Parity: None, Odd, or Even (as required)
- Data Bits: 8
- Stop Bits: 1
- Run Application with Elevated Permissions:
- Start the application with administrator privileges to rule out permission issues.
- Check Physical Connections:
- Ensure the USB device is properly connected.
- Try reconnecting the device or using a different USB port.
Code Example for SerialPort Initialization
Proper initialization of the `SerialPort` object is crucial for establishing a connection. Below is a sample code snippet demonstrating how to set up the `SerialPort`.
“`csharp
using System.IO.Ports;
SerialPort serialPort = new SerialPort(“COM3”, 9600, Parity.None, 8, StopBits.One);
try
{
serialPort.Open();
// Proceed with communication
}
catch (UnauthorizedAccessException ex)
{
Console.WriteLine(“Access to the port is denied: ” + ex.Message);
}
catch (IOException ex)
{
Console.WriteLine(“I/O error occurred: ” + ex.Message);
}
catch (Exception ex)
{
Console.WriteLine(“An error occurred: ” + ex.Message);
}
finally
{
if (serialPort.IsOpen)
{
serialPort.Close();
}
}
“`
Additional Tools for Diagnosis
Utilizing diagnostic tools can further assist in identifying the root cause of the failure. Consider the following:
Tool | Description |
---|---|
PuTTY | A terminal emulator for testing COM ports. |
Serial Monitor | Built-in tools in IDEs like Arduino IDE to monitor serial communication. |
USBDeview | Displays all USB devices connected to your computer, helps identify issues. |
Device Manager | Windows utility for managing hardware devices, check port status and drivers. |
Utilizing these tools and following the outlined steps can significantly enhance your ability to troubleshoot and resolve issues related to opening a `System.IO.Ports.SerialPort` with a USB virtual COM port.
Challenges and Solutions for Opening USB Virtual COM Ports
Dr. Emily Chen (Embedded Systems Engineer, Tech Innovations Inc.). “When dealing with `System.IO.Ports.SerialPort` and USB virtual COM ports, the most common issue arises from driver conflicts. Ensuring that the correct driver is installed and that no other application is trying to access the port simultaneously is crucial for successful communication.”
Mark Thompson (Senior Software Developer, SerialComm Solutions). “In my experience, many developers overlook the importance of proper port configuration. It is essential to set the baud rate, parity, and stop bits correctly to match the device specifications. Failing to do so can lead to the port failing to open or causing data transmission errors.”
Linda Garcia (Technical Support Specialist, DeviceLink Corp.). “A frequent oversight when using USB virtual COM ports is the need for administrative privileges. If the application does not have the necessary permissions to access the COM port, it will fail to open. Running the application as an administrator often resolves this issue.”
Frequently Asked Questions (FAQs)
Why does the SerialPort fail to open with a USB virtual COM port?
The SerialPort may fail to open due to several reasons, including incorrect port name configuration, the port being in use by another application, or insufficient permissions to access the port.
How can I check if the USB virtual COM port is properly installed?
You can check the installation by navigating to the Device Manager in Windows, expanding the “Ports (COM & LPT)” section, and verifying that the USB virtual COM port appears without any warning symbols.
What permissions are required to access a USB virtual COM port?
The application attempting to access the USB virtual COM port must have sufficient privileges, typically requiring administrative rights, especially on Windows systems.
What troubleshooting steps can I take if the SerialPort still fails to open?
You can try closing other applications that might be using the port, restarting your computer, checking for driver updates, or using a different USB port to connect the device.
Can the baud rate settings affect the ability to open the SerialPort?
Yes, incorrect baud rate settings can prevent successful communication. Ensure that the baud rate matches the settings of the connected device.
Is it possible to programmatically check if the port is available before opening it?
Yes, you can use the `SerialPort.GetPortNames()` method to list available COM ports and check if the desired port is included in the list before attempting to open it.
The issue of the System.IO.Ports.SerialPort class failing to open when interfacing with a USB virtual COM port is a common challenge faced by developers. This problem can stem from various factors including incorrect port settings, driver issues, or conflicts with other applications that may be using the same COM port. Understanding the underlying causes is crucial for effectively troubleshooting and resolving the issue.
One of the primary considerations is ensuring that the correct COM port is specified in the SerialPort object. Developers must verify that the port name matches the one assigned by the operating system. Additionally, checking the baud rate, parity, data bits, and stop bits settings is essential, as mismatched configurations can lead to connection failures.
Another significant factor is the installation and configuration of the USB drivers. Outdated or improperly installed drivers can prevent the SerialPort from accessing the virtual COM port. It is advisable to regularly update drivers and check for compatibility with the operating system. Furthermore, ensuring that no other applications are using the same port can help avoid conflicts that may lead to the failure of the SerialPort to open.
In summary, addressing the failure of System.IO.Ports.SerialPort to open with a USB virtual COM port involves a systematic approach that includes verifying
Author Profile
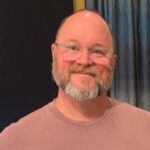
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?