Why Am I Seeing ‘SyntaxError: Positional Argument Follows Keyword Argument’ in My Code?
In the world of programming, particularly in Python, the beauty of writing clean and efficient code can sometimes be overshadowed by the complexities of syntax rules. One common pitfall that both novice and seasoned developers encounter is the dreaded `SyntaxError: positional argument follows keyword argument`. This seemingly cryptic error message can halt your coding progress and leave you scratching your head. Understanding the nuances of argument ordering in function calls is essential for writing robust code and avoiding frustrating debugging sessions.
This article delves into the intricacies of function arguments in Python, shedding light on the fundamental principles that govern their usage. We will explore the distinction between positional and keyword arguments, illustrating how their order can impact the execution of your code. By unraveling the reasons behind this specific syntax error, we aim to equip you with the knowledge to prevent it from disrupting your coding workflow.
As we navigate through this topic, you’ll gain insights into best practices for defining and calling functions, enhancing your programming skills and confidence. Whether you’re a beginner eager to learn or an experienced coder looking to refresh your understanding, this exploration will provide valuable guidance on mastering argument syntax in Python.
Understanding the Error
A `SyntaxError: positional argument follows keyword argument` occurs in Python when a function call is improperly structured. In Python, when you define a function, you can have both positional and keyword arguments. Positional arguments must always precede keyword arguments in the function call to avoid confusion about which value corresponds to which parameter.
Key Points
- Positional Arguments: These are arguments that need to be included in the proper position or order.
- Keyword Arguments: These are arguments that are passed to a function using the name of the parameter. For example, `func(param=value)`.
Example of the Error
Consider the following example:
“`python
def example_function(a, b, c):
return a + b + c
Incorrect usage
result = example_function(1, b=2, 3)
“`
In this case, `3` is a positional argument that follows the keyword argument `b=2`, which leads to a `SyntaxError`.
Correcting the Error
To resolve this error, you need to ensure that all positional arguments are specified before any keyword arguments. The correct way to call the function would be:
“`python
Correct usage
result = example_function(1, 2, c=3)
“`
Summary of Correct Usage
- Always place positional arguments first.
- Follow with keyword arguments.
Order of Arguments | Example | Result |
---|---|---|
Valid | example_function(1, 2, c=3) | Returns 6 |
Invalid | example_function(1, b=2, 3) | Raises SyntaxError |
Best Practices to Avoid the Error
To prevent encountering this error in your code, consider the following best practices:
- Consistent Argument Ordering: Always define your function calls with positional arguments first.
- Use Keyword Arguments When Necessary: If you have many parameters or some are optional, consider using keyword arguments for clarity.
- Readability: Prioritize readability in your function calls, which can help in minimizing such errors.
By adhering to these practices, you can improve both the readability and maintainability of your code while reducing the likelihood of syntax errors.
Understanding the Error
A `SyntaxError: positional argument follows keyword argument` occurs in Python when the order of arguments in a function call does not comply with the language’s syntactic rules. In Python, keyword arguments must be placed after positional arguments in a function call.
Key Concepts
- Positional Arguments: These are the arguments that need to be passed to a function in the exact order defined in the function’s signature.
- Keyword Arguments: These arguments are passed to a function by explicitly stating which parameter they correspond to, using the syntax `parameter_name=value`.
Example of the Error
Consider the following code snippet:
“`python
def example_function(a, b, c):
return a + b + c
result = example_function(1, b=2, 3) This will raise a SyntaxError
“`
In the above example, `1` and `3` are positional arguments, while `b=2` is a keyword argument. The positional argument `3` follows the keyword argument `b=2`, which triggers the `SyntaxError`.
Correcting the Error
To resolve the error, ensure that all positional arguments are provided before any keyword arguments in the function call.
Corrected Example
“`python
result = example_function(1, 2, c=3) This is valid
“`
In this corrected version, all positional arguments (`1` and `2`) precede the keyword argument (`c=3`), complying with Python’s argument order rules.
Common Scenarios Leading to This Error
- Accidental Misplacement: Developers might accidentally place a positional argument after a keyword argument, particularly in complex function calls.
- Refactoring Code: When modifying function calls, it is easy to overlook the order of arguments.
- Using Default Values: When a function uses default values for some parameters, it’s common to forget the position of non-default parameters.
Best Practices to Avoid the Error
To prevent encountering this error, consider the following best practices:
- Be Consistent: Always place positional arguments before keyword arguments.
- Use Clear Naming: When calling functions, use descriptive names for keyword arguments to enhance readability.
- Follow a Style Guide: Adhering to PEP 8 or similar style guides can help maintain clear conventions in code.
- Code Reviews: Regularly review code with peers to catch potential issues before they become errors.
- Automated Tools: Utilize linters or code quality tools that can highlight such issues before runtime.
Debugging Tips
When facing this error, use the following debugging steps:
- Review Function Calls: Examine the function call in the error traceback to identify the order of arguments.
- Check Function Definition: Compare the arguments in the function definition with those in the call to ensure compliance.
- Isolate the Issue: If the function call is complex, simplify it by breaking it down into smaller parts.
Step | Action |
---|---|
Identify | Locate the function call causing the error. |
Compare | Ensure argument order matches function definition. |
Simplify | Break down complex calls into simpler parts. |
Understanding the SyntaxError: Positional Argument Follows Keyword Argument
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “The SyntaxError indicating that a positional argument follows a keyword argument typically arises when developers inadvertently mix argument types in function calls. It is crucial to maintain the correct order: all positional arguments must precede any keyword arguments to ensure clarity and prevent runtime errors.”
Michael Chen (Python Programming Instructor, Tech Academy). “This error is a common pitfall for beginners in Python. Understanding the distinction between positional and keyword arguments is essential. Developers should always review their function definitions and calls to avoid such errors, which can lead to confusion and debugging challenges.”
Laura Simmons (Lead Developer, Innovative Software Solutions). “In practice, the SyntaxError related to positional and keyword arguments serves as a reminder to follow Python’s strict syntax rules. When designing APIs or libraries, clear documentation on argument usage can significantly reduce the likelihood of this error occurring among users.”
Frequently Asked Questions (FAQs)
What does the error “SyntaxError: positional argument follows keyword argument” mean?
This error indicates that in a function call, a positional argument is placed after a keyword argument, which is not allowed in Python. Positional arguments must always precede keyword arguments.
How can I fix the “SyntaxError: positional argument follows keyword argument” error?
To resolve this error, ensure that all positional arguments are listed before any keyword arguments in the function call. Rearranging the arguments will eliminate the error.
Can you provide an example that triggers this error?
Certainly. An example would be: `my_function(arg1, arg2=2, 3)`. Here, `3` is a positional argument following the keyword argument `arg2=2`, which causes the error.
Are there specific scenarios where this error commonly occurs?
This error commonly occurs when developers mistakenly mix positional and keyword arguments, especially in functions with multiple parameters or when using default values.
Is this error specific to any version of Python?
No, this error is present in all versions of Python. The rules regarding the order of positional and keyword arguments have remained consistent across versions.
What best practices can help avoid this error?
To avoid this error, always define the function calls with positional arguments first, and consider using keyword arguments for clarity when many parameters are involved. Additionally, reviewing function definitions can help ensure correct argument ordering.
In programming, particularly in Python, the error message “SyntaxError: positional argument follows keyword argument” indicates a specific issue with the way arguments are passed to functions. This error arises when a positional argument is placed after a keyword argument in a function call. Python requires that all positional arguments precede any keyword arguments to maintain clarity and consistency in function calls.
Understanding the distinction between positional and keyword arguments is crucial for effective coding. Positional arguments are those that are assigned based on their position in the function call, while keyword arguments are assigned based on the name of the parameter. This syntactical rule helps prevent ambiguity in function calls, ensuring that each argument is correctly matched to its corresponding parameter.
To avoid encountering this error, developers should always structure their function calls by placing all positional arguments before any keyword arguments. This practice not only adheres to Python’s syntax rules but also enhances the readability and maintainability of the code. By being mindful of argument order, programmers can prevent common pitfalls and streamline their coding process.
Author Profile
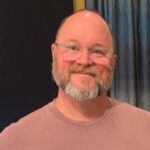
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?