How Can You Efficiently Search for Stored Procedure Text in SQL Server?
In the ever-evolving landscape of database management, SQL Server stands out as a powerful tool for organizations seeking to streamline their data operations. One of the often-overlooked yet crucial aspects of working with SQL Server is the ability to efficiently search through stored procedure text. As businesses accumulate vast amounts of data and complex queries, the need to navigate and manage these stored procedures becomes paramount. Whether you’re a seasoned database administrator or a developer just starting, understanding how to search for and analyze stored procedure text can significantly enhance your productivity and the overall performance of your SQL Server environment.
Searching for specific stored procedures can be a daunting task, especially when dealing with extensive codebases that contain numerous scripts and functions. SQL Server provides various methods to locate and analyze stored procedure text, enabling users to identify dependencies, optimize performance, and ensure code quality. This capability not only aids in troubleshooting and debugging but also empowers teams to maintain a clean and efficient database structure. As we delve deeper into the intricacies of SQL Server’s search functionalities, we’ll explore the tools and techniques available to streamline this process, making it easier than ever to manage your stored procedures effectively.
Whether you’re looking to enhance your query performance or simply seeking to understand the relationships between different procedures, mastering the art of searching stored procedure text in SQL
Searching for Stored Procedure Text
To locate stored procedures in SQL Server based on specific text within their definitions, one can utilize system catalog views. The `sys.sql_modules` and `sys.procedures` views are particularly useful for this purpose. These views provide a means to query the definitions of stored procedures and search for specific keywords or phrases.
A typical SQL query to find stored procedures containing a certain text might look like this:
“`sql
SELECT
p.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.procedures p ON m.object_id = p.object_id
WHERE
m.definition LIKE ‘%search_text%’
ORDER BY
p.name;
“`
This query will return the names and definitions of all stored procedures that include the specified `search_text`.
Understanding the System Views
The following are key columns from the system views used in the above query:
View | Column | Description |
---|---|---|
sys.sql_modules | object_id | Unique identifier for the object (stored procedure). |
sys.sql_modules | definition | The text of the SQL definition for the object. |
sys.procedures | name | The name of the stored procedure. |
sys.procedures | object_id | Unique identifier for the procedure. |
Utilizing these views allows for efficient searching through stored procedures, especially in databases with numerous procedures.
Using Full-Text Search
For databases that have full-text search capabilities enabled, you can perform more advanced searches. Full-text search allows for searching against large text fields, providing better performance and more flexibility than simple LIKE queries.
To implement full-text search on stored procedures, follow these steps:
- Ensure full-text indexing is enabled on your SQL Server instance.
- Create a full-text catalog if one does not already exist.
- Create a full-text index on the stored procedure definitions.
Once the full-text index is in place, you can use the `CONTAINS` function for more complex queries:
“`sql
SELECT
p.name AS ProcedureName,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.procedures p ON m.object_id = p.object_id
WHERE
CONTAINS(m.definition, ‘search_text’)
ORDER BY
p.name;
“`
This method allows for searching phrases, proximity searches, and other powerful text-based queries.
Considerations and Best Practices
When searching for stored procedure text, keep in mind the following best practices:
- Use specific keywords: The more specific your search term, the more relevant your results will be.
- Limit your search scope: If you know the schema or specific name patterns of the procedures, include that in your query to narrow down results.
- Regularly maintain indexes: For databases with frequent changes, ensure that full-text indexes are regularly updated for optimal performance.
- Test your queries: Before deploying queries in production, test them in a development environment to avoid performance issues.
By leveraging these techniques and tools, you can efficiently search stored procedure text in SQL Server, enhancing your database management capabilities.
Searching for Stored Procedure Text in SQL Server
To efficiently locate stored procedures containing specific text within SQL Server, you can utilize system views and functions. The primary system views for this task include `sys.sql_modules` and `sys.objects`. These views provide access to the definitions of SQL objects, including stored procedures.
Using T-SQL to Search Stored Procedure Definitions
You can execute the following T-SQL query to find stored procedures that contain a specific keyword or phrase in their definition:
“`sql
SELECT
o.name AS ProcedureName,
o.type_desc AS ObjectType,
m.definition AS ProcedureDefinition
FROM
sys.sql_modules m
JOIN
sys.objects o ON m.object_id = o.object_id
WHERE
o.type = ‘P’ — Only stored procedures
AND m.definition LIKE ‘%YourSearchKeyword%’ — Replace with your search term
ORDER BY
o.name;
“`
Explanation of the Query:
- `sys.sql_modules`: Contains the definition of SQL Server objects.
- `sys.objects`: Provides information about all objects in the database.
- `o.type = ‘P’`: Filters the results to include only stored procedures.
- `m.definition LIKE ‘%YourSearchKeyword%’`: Searches the procedure definition for the specified keyword.
Advanced Search Options
You may enhance the search capabilities by incorporating additional conditions or functions. Consider the following examples:
- Case Sensitivity: To perform a case-sensitive search, you can adjust the collation of the search term:
“`sql
AND m.definition COLLATE SQL_Latin1_General_CP1_CS_AS LIKE ‘%YourSearchKeyword%’
“`
- Search Across Multiple Object Types: If you want to search through other objects, such as functions or views, you can modify the object type condition:
“`sql
WHERE
o.type IN (‘P’, ‘FN’, ‘IF’, ‘V’) — Procedures, Functions, Views
“`
Using SQL Server Management Studio (SSMS)
Alternatively, you can utilize SQL Server Management Studio’s built-in features:
- Object Explorer:
- Right-click on the database.
- Select Find and then Find Database Object.
- Enter your search term and choose Stored Procedures from the object types.
- View Object Definition:
- In Object Explorer, navigate to the stored procedure.
- Right-click and select Modify to view its definition.
Performance Considerations
When searching for stored procedure definitions, keep in mind:
- Database Size: Larger databases may yield slower search times.
- Indexing: Regular maintenance and indexing can improve performance.
- Frequent Changes: If stored procedures are modified often, consider refreshing your search strategy regularly.
Consideration | Impact |
---|---|
Database Size | Slower searches with larger objects |
Indexing | Enhances performance |
Modification Frequency | Adjust search strategy frequently |
By employing these methods, you can effectively search for specific text within stored procedures in SQL Server, optimizing your development and maintenance processes.
Expert Insights on Searching Stored Procedure Text in SQL Server
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “Efficiently searching for stored procedure text in SQL Server is crucial for maintaining and optimizing database performance. Utilizing system views like sys.sql_modules and sys.procedures allows developers to quickly locate and analyze the code within stored procedures, which is essential for debugging and enhancing application functionality.”
Mark Thompson (Senior SQL Developer, Data Solutions Group). “Implementing full-text search capabilities in SQL Server can significantly streamline the process of searching stored procedure text. By leveraging full-text indexes, developers can execute more complex queries that yield faster and more relevant results, ultimately improving the efficiency of database management tasks.”
Lisa Patel (SQL Server Consultant, Database Dynamics). “Understanding the nuances of stored procedure text search is vital for any SQL Server professional. It is important to consider not only the syntax but also the execution plan of stored procedures when searching, as this can impact performance. Regularly reviewing and refactoring stored procedures can lead to better searchability and maintainability in the long run.”
Frequently Asked Questions (FAQs)
What is a stored procedure in SQL Server?
A stored procedure in SQL Server is a precompiled collection of one or more SQL statements that can be executed as a single unit. It allows for modular programming, improved performance, and easier maintenance.
How can I search for stored procedure text in SQL Server?
You can search for stored procedure text in SQL Server by querying the `sys.sql_modules` or `sys.procedures` system views. Using the `LIKE` operator in a SQL query allows you to find specific keywords or phrases within the procedure definitions.
What SQL query can I use to find a specific stored procedure?
You can use the following SQL query:
“`sql
SELECT OBJECT_NAME(object_id) AS ProcedureName
FROM sys.sql_modules
WHERE definition LIKE ‘%YourSearchTerm%’;
“`
Replace `YourSearchTerm` with the term you are searching for.
Can I search for stored procedures across multiple databases?
Yes, you can search for stored procedures across multiple databases by executing the search query in each database context or by using dynamic SQL to iterate through the databases and execute the search.
What permissions are required to search stored procedure text in SQL Server?
To search stored procedure text, you typically need at least `VIEW DEFINITION` permission on the database. This permission allows you to access the metadata of the stored procedures.
Is there a way to automate the search for stored procedure text?
Yes, you can automate the search for stored procedure text by creating a SQL Server Agent job or a script that runs periodically. This script can utilize the aforementioned queries to log or alert you when specific terms are found in stored procedures.
In summary, searching for stored procedure text in SQL Server is a crucial task for database administrators and developers alike. It allows users to efficiently locate specific procedures based on keywords or phrases, facilitating better code management and optimization. Utilizing system catalog views, such as sys.sql_modules and sys.procedures, enables users to extract relevant information about stored procedures, including their definitions and associated metadata. This capability is essential for maintaining the integrity and performance of SQL Server databases.
Moreover, employing dynamic SQL queries can enhance the search process, allowing for more flexible and powerful searches across multiple stored procedures. By leveraging tools such as SQL Server Management Studio (SSMS) or writing custom scripts, users can streamline their workflow and quickly identify procedures that require updates or debugging. This proactive approach not only improves efficiency but also contributes to overall database health.
Key takeaways from the discussion include the importance of understanding the underlying system views for effective searching, the benefits of using dynamic SQL for complex queries, and the necessity of maintaining clear documentation of stored procedures. By adopting these practices, SQL Server users can ensure that their database environments remain organized and responsive to evolving application needs.
Author Profile
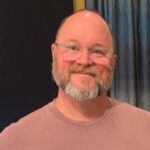
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?