How Can You Effectively Use SQL Query to Filter Data By Date?
In the world of data management, the ability to filter information effectively is paramount, especially when dealing with time-sensitive data. SQL, or Structured Query Language, stands as the backbone of database interaction, allowing users to perform complex queries with ease. Among its many powerful features, filtering by date is one of the most essential techniques for any data analyst or developer. Whether you’re sifting through sales records, user activity logs, or historical data, mastering date filtering can significantly enhance your data retrieval capabilities and insights.
Understanding how to filter SQL queries by date is not just about writing a few lines of code; it’s about unlocking the potential of your data. With the right techniques, you can isolate specific time frames, analyze trends over periods, and generate reports that are both timely and relevant. This skill is particularly crucial in industries where decisions are driven by real-time data, such as finance, e-commerce, and healthcare. As we delve deeper into the intricacies of SQL date filtering, you’ll discover various methods and best practices that will empower you to extract meaningful insights from your datasets.
From leveraging built-in date functions to constructing dynamic queries that adapt to user input, the possibilities are vast. Whether you’re a seasoned SQL veteran or just starting your journey into database management, understanding how to filter by date
Understanding Date Functions in SQL
To effectively filter records by date in SQL, it is crucial to understand the various date functions available. These functions allow you to manipulate and format date values according to your requirements. Commonly used date functions include:
- `CURRENT_DATE`: Returns the current date.
- `DATEADD`: Adds a specified interval to a date.
- `DATEDIFF`: Calculates the difference between two dates.
- `FORMAT`: Formats a date value according to a specified format.
These functions can be utilized in SQL queries to facilitate filtering based on specific date criteria.
Filtering Records by Date
When filtering records by date, you can use the `WHERE` clause in conjunction with date functions to specify the desired date range or specific dates. Here are some examples of how to filter records effectively:
- To select records from a specific date:
“`sql
SELECT * FROM orders
WHERE order_date = ‘2023-10-01’;
“`
- To select records within a date range:
“`sql
SELECT * FROM orders
WHERE order_date BETWEEN ‘2023-10-01’ AND ‘2023-10-31’;
“`
- To select records from the last 30 days:
“`sql
SELECT * FROM orders
WHERE order_date >= DATEADD(DAY, -30, CURRENT_DATE);
“`
Date Filtering with Different SQL Dialects
Different SQL databases may have variations in syntax and available functions. Below is a comparison of how to filter by date in some popular SQL dialects:
SQL Dialect | Example Query |
---|---|
MySQL | SELECT * FROM orders WHERE order_date >= CURDATE() – INTERVAL 30 DAY; |
SQL Server | SELECT * FROM orders WHERE order_date >= DATEADD(DAY, -30, GETDATE()); |
PostgreSQL | SELECT * FROM orders WHERE order_date >= CURRENT_DATE – INTERVAL ’30 days’; |
Understanding these differences is essential for writing effective SQL queries tailored to the specific database system in use.
Best Practices for Date Filtering
When filtering by date in SQL, adhering to best practices can enhance performance and maintainability. Consider the following guidelines:
– **Use indexed date columns**: Ensure that the date column being filtered is indexed to improve query performance.
– **Be explicit with date formats**: Always specify the date format to avoid errors, especially in databases that may interpret date strings differently.
– **Avoid functions on indexed columns**: Using functions on date columns in the `WHERE` clause can negate the use of indexes. For example, instead of `WHERE YEAR(order_date) = 2023`, use `WHERE order_date >= ‘2023-01-01’ AND order_date < '2024-01-01'`.
By following these best practices, you can ensure that your date filtering is both efficient and accurate.
Understanding Date Formats in SQL
In SQL, dates can be stored in various formats depending on the database system being used. It’s essential to understand these formats to ensure accurate queries. Common formats include:
- ISO 8601: `YYYY-MM-DD` (e.g., `2023-10-15`)
- US Format: `MM/DD/YYYY` (e.g., `10/15/2023`)
- European Format: `DD/MM/YYYY` (e.g., `15/10/2023`)
Different SQL databases may have their own functions for handling date formats. For instance:
Database | Function to Format Date |
---|---|
MySQL | `DATE_FORMAT(date, format)` |
PostgreSQL | `TO_CHAR(date, format)` |
SQL Server | `FORMAT(date, format)` |
Filtering Dates in SQL Queries
When filtering records based on date criteria, the SQL `WHERE` clause is utilized. The following are common patterns for date filtering:
- Filtering a specific date:
“`sql
SELECT * FROM orders
WHERE order_date = ‘2023-10-15’;
“`
- Filtering a date range:
“`sql
SELECT * FROM orders
WHERE order_date BETWEEN ‘2023-10-01’ AND ‘2023-10-31’;
“`
- Filtering records before or after a specific date:
“`sql
SELECT * FROM orders
WHERE order_date < '2023-10-01';
```
- Filtering using functions:
“`sql
SELECT * FROM orders
WHERE YEAR(order_date) = 2023;
“`
Using Date Functions for Complex Filters
SQL offers various functions that can be employed to manipulate and filter dates more effectively. Key functions include:
– **CURRENT_DATE**: Returns the current date.
– **DATEADD()**: Adds a specified interval to a date.
– **DATEDIFF()**: Returns the difference between two dates.
Examples of using these functions:
– **Filtering for records in the last 30 days**:
“`sql
SELECT * FROM orders
WHERE order_date >= CURRENT_DATE – INTERVAL ’30 days’;
“`
– **Finding records where the difference between dates exceeds a certain value**:
“`sql
SELECT * FROM orders
WHERE DATEDIFF(NOW(), order_date) > 30;
“`
Performance Considerations When Filtering by Date
When filtering by date, performance can be impacted by several factors. Consider the following best practices:
– **Indexing**: Ensure that date columns are indexed for faster query performance.
– **Avoiding Functions on Indexed Columns**: Applying functions directly on date columns can prevent the use of indexes, leading to slower queries.
– **Date Range Queries**: Use inclusive ranges to minimize the number of records scanned.
Example of an efficient date filter:
“`sql
SELECT * FROM orders
WHERE order_date >= ‘2023-01-01’ AND order_date <= '2023-12-31';
```
Common Pitfalls to Avoid
When working with date filters in SQL, be mindful of the following common mistakes:
- Incorrect Date Formats: Always ensure the date format matches the database’s expectations.
- Timezone Issues: Be aware of how your database handles timezones, especially in systems where timestamps are stored.
- Not Accounting for Time: When filtering by date, neglecting the time component can lead to missing records. Always consider the full datetime if applicable.
By following these guidelines, you can effectively filter records by date in SQL, ensuring accurate data retrieval and optimal performance in your queries.
Expert Insights on SQL Query Filtering by Date
Dr. Emily Carter (Data Scientist, Analytics Innovations). “When filtering SQL queries by date, it’s crucial to ensure that the date format in your query matches the format stored in the database. Using functions like `CAST` or `CONVERT` can help in standardizing formats, which ultimately leads to more efficient queries.”
Michael Chen (Database Administrator, Tech Solutions Inc.). “Implementing indexes on date columns can significantly enhance the performance of SQL queries that filter by date. This optimization reduces the time complexity of data retrieval, especially in large datasets.”
Sarah Patel (SQL Developer, DataCraft Technologies). “Always consider using parameterized queries when filtering by date to prevent SQL injection attacks. This practice not only secures your database but also improves the readability and maintainability of your code.”
Frequently Asked Questions (FAQs)
What is an SQL query filter by date?
An SQL query filter by date is a condition applied in a SQL statement to retrieve records from a database that fall within a specified date range or match a specific date.
How do I filter records by a specific date in SQL?
To filter records by a specific date, use the `WHERE` clause in your SQL query, specifying the date column and the desired date. For example: `SELECT * FROM table_name WHERE date_column = ‘YYYY-MM-DD’;`.
Can I filter by date range in SQL queries?
Yes, you can filter by a date range using the `BETWEEN` operator or by combining conditions with `AND`. For example: `SELECT * FROM table_name WHERE date_column BETWEEN ‘start_date’ AND ‘end_date’;`.
What date formats are acceptable in SQL queries?
SQL typically accepts the ISO 8601 format (YYYY-MM-DD) for date values. However, the acceptable format may vary based on the database system in use.
How can I filter by date and time in SQL?
To filter by date and time, include both the date and time in your condition. For example: `SELECT * FROM table_name WHERE datetime_column = ‘YYYY-MM-DD HH:MM:SS’;`.
Are there functions to manipulate dates in SQL queries?
Yes, SQL provides various date functions such as `DATEADD`, `DATEDIFF`, and `FORMAT`, which allow for manipulation and formatting of date values within queries.
In summary, filtering SQL queries by date is a fundamental practice that allows users to retrieve data within specific time frames. This capability is essential for analyzing trends, generating reports, and ensuring data accuracy. By utilizing date functions and operators, such as BETWEEN, =, and >, users can effectively narrow down their datasets to meet their analytical needs. Understanding the various date formats and the importance of indexing date columns can significantly enhance query performance and efficiency.
Moreover, leveraging date filtering can facilitate the management of time-sensitive data, such as transaction records, user activity logs, and event timelines. Properly structured SQL queries can yield insights into customer behavior, operational performance, and market trends over specified periods. This practice not only aids in data analysis but also supports decision-making processes across various business functions.
Lastly, it is crucial for SQL practitioners to stay informed about best practices related to date filtering. This includes being aware of potential pitfalls, such as time zone discrepancies and data type mismatches. By adhering to these best practices and continuously refining their skills, users can maximize the utility of date filtering in SQL queries, ultimately leading to more informed and strategic outcomes.
Author Profile
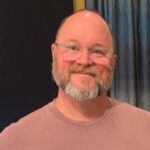
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?