How Can You Effectively Use SQL Lookup Key Values for Columns?
In the world of databases, SQL (Structured Query Language) serves as the backbone for managing and manipulating data. Among its myriad functionalities, the ability to perform lookups is crucial for retrieving specific information quickly and efficiently. Whether you’re a seasoned database administrator or a budding data analyst, mastering the art of SQL lookup key values for columns can significantly enhance your data querying skills. This powerful technique allows you to connect disparate pieces of information, streamline your data analysis, and ultimately make more informed decisions based on the insights you uncover.
At its core, an SQL lookup involves searching for a specific value in one table and using that value to retrieve related data from another table. This process is essential for maintaining data integrity and ensuring that your queries return the most relevant information. By leveraging key values—unique identifiers that link records across tables—you can create relationships that enrich your datasets and provide a comprehensive view of your data landscape. Understanding how to effectively implement these lookups not only simplifies complex queries but also optimizes performance, making your database interactions more efficient.
As we delve deeper into the intricacies of SQL lookup key values for columns, we’ll explore various techniques, including joins, subqueries, and common table expressions. Each method offers unique advantages and can be tailored to fit specific use cases, ensuring that you
Understanding SQL Lookup Key Values
In SQL, a lookup key value refers to a specific identifier used to retrieve corresponding data from a related table. This concept is fundamental in relational database management, where tables are interconnected through keys. Typically, the primary key of one table serves as a foreign key in another table, facilitating efficient data retrieval and maintaining data integrity.
When performing lookups, it is crucial to choose the right key value, as it directly impacts the accuracy of the results. A well-structured query can efficiently join tables based on these keys, allowing for complex data relationships to be navigated seamlessly.
Types of Lookup Keys
There are several types of lookup keys commonly utilized in SQL databases:
- Primary Key: A unique identifier for each record in a table, ensuring that no two records can have the same primary key value.
- Foreign Key: A key in one table that links to a primary key in another table, establishing a relationship between the two tables.
- Composite Key: A combination of two or more columns that together serve as a unique identifier for a record.
- Surrogate Key: An artificially created key, often an integer, used to uniquely identify a record when no natural primary key exists.
Example of SQL Lookup Using Key Values
Consider two tables: `Employees` and `Departments`. The `Employees` table contains a foreign key `DepartmentID` that relates to the primary key `ID` in the `Departments` table.
Employees Table | Departments Table |
---|---|
EmployeeID | ID |
Name | Name |
DepartmentID (FK) | Location |
To perform a lookup that retrieves employee names along with their respective department names, an SQL JOIN operation can be used as follows:
“`sql
SELECT Employees.Name, Departments.Name AS Department
FROM Employees
JOIN Departments ON Employees.DepartmentID = Departments.ID;
“`
This query effectively combines the data from both tables, ensuring that each employee is matched with their corresponding department based on the key value.
Best Practices for Using Lookup Keys
When working with lookup keys in SQL, consider the following best practices:
- Ensure Uniqueness: Always enforce uniqueness on primary keys to avoid data integrity issues.
- Use Indexes: Create indexes on foreign keys to improve query performance during lookups.
- Maintain Referential Integrity: Use constraints to ensure that relationships between tables remain valid.
- Document Relationships: Keep clear documentation of how tables are related, including which fields serve as keys.
By adhering to these practices, developers can create robust and efficient SQL queries that leverage lookup key values effectively.
Understanding SQL Lookup Key Value
In SQL, a lookup key is often used to retrieve values from a particular column in a table based on a specific key or condition. This process is essential for maintaining data integrity and enabling efficient data retrieval from relational databases.
Types of SQL Lookups
There are several common types of SQL lookups that can be utilized depending on the specific use case:
- Simple Lookup: Retrieves data based on a single key value.
- Join Lookup: Combines rows from two or more tables based on a related column.
- Subquery Lookup: Uses a nested query to filter results based on another query’s output.
Implementing a Simple Lookup
A straightforward way to perform a lookup is by using the `SELECT` statement combined with a `WHERE` clause. Here’s an example:
“`sql
SELECT column_name
FROM table_name
WHERE key_column = ‘desired_key_value’;
“`
Example: If you want to find the employee name based on their employee ID:
“`sql
SELECT employee_name
FROM employees
WHERE employee_id = 123;
“`
This query retrieves the `employee_name` for the employee with ID `123`.
Using Joins for Lookups
Joins are powerful tools for performing lookups across multiple tables. The most common types include:
- INNER JOIN: Returns only the rows with matching values in both tables.
- LEFT JOIN: Returns all rows from the left table and the matched rows from the right table.
- RIGHT JOIN: Returns all rows from the right table and the matched rows from the left table.
Example of an INNER JOIN:
“`sql
SELECT e.employee_name, d.department_name
FROM employees e
INNER JOIN departments d ON e.department_id = d.department_id
WHERE e.employee_id = 123;
“`
This query retrieves the employee name and department name where the employee ID is `123`.
Subquery Lookups
Subqueries allow for more complex lookups by nesting one query within another. This is particularly useful for filtering datasets based on aggregated values or conditions from another table.
Example:
“`sql
SELECT employee_name
FROM employees
WHERE department_id IN (SELECT department_id FROM departments WHERE location = ‘New York’);
“`
This query finds all employees who work in departments located in New York.
Performance Considerations
When implementing lookups in SQL, consider the following to optimize performance:
- Indexing: Create indexes on columns that are frequently used in lookup conditions.
- Use of Joins: Ensure that joins are necessary and appropriate for the dataset size.
- Avoiding SELECT * : Specify only the columns needed for your results to reduce data load.
Strategy | Description |
---|---|
Indexing | Enhances retrieval speed for large datasets. |
Efficient Joins | Ensure minimal data is processed by filtering early. |
Column Selection | Reduces overhead by selecting only required fields. |
Implementing these strategies can significantly improve the efficiency of SQL queries involving lookups.
Expert Insights on SQL Lookup Key Values for Columns
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “Utilizing SQL lookup key values for columns is crucial for optimizing query performance. By establishing proper indexing on lookup keys, we can significantly reduce the time it takes to retrieve related data, thereby enhancing overall application efficiency.”
Michael Thompson (Data Analyst, Insightful Analytics). “When designing databases, it’s essential to consider how lookup key values will be used across different tables. Implementing foreign keys not only maintains data integrity but also streamlines data retrieval processes, making it easier to perform complex queries and analyses.”
Sarah Patel (SQL Developer, Cloud Solutions Group). “Incorporating lookup key values effectively can transform how we manage relationships in our databases. By leveraging JOIN operations with well-defined keys, we can ensure that our data remains interconnected and accessible, which is vital for reporting and decision-making purposes.”
Frequently Asked Questions (FAQs)
What is a SQL lookup key value for a column?
A SQL lookup key value refers to a specific identifier used to retrieve or reference data within a database column. It typically serves as a unique value that links related data across different tables.
How can I perform a SQL lookup using a key value?
To perform a SQL lookup using a key value, you can use a SELECT statement with a WHERE clause that specifies the column and the key value. For example: `SELECT * FROM table_name WHERE column_name = ‘key_value’;`
Can I use multiple key values in a SQL lookup?
Yes, you can use multiple key values in a SQL lookup by employing the IN operator in your query. For example: `SELECT * FROM table_name WHERE column_name IN (‘key_value1’, ‘key_value2’);`
What happens if the key value does not exist in the column?
If the key value does not exist in the specified column, the SQL query will return an empty result set, indicating that no matching records were found.
Is it possible to join tables using a lookup key value?
Yes, you can join tables using a lookup key value by specifying the key columns in the JOIN clause. For example: `SELECT * FROM table1 JOIN table2 ON table1.key_column = table2.key_column;`
How do I optimize SQL lookups for performance?
To optimize SQL lookups for performance, ensure that the columns used as keys are indexed, limit the number of columns returned, and avoid using functions on key columns in the WHERE clause, as they can hinder index usage.
utilizing SQL lookup key values for columns is a fundamental practice in database management that enhances data retrieval and integrity. This technique allows users to efficiently reference and associate related data across different tables, thereby streamlining queries and improving overall database performance. By implementing lookup keys, developers can ensure that data is consistent and easily accessible, which is crucial for maintaining the quality of information within relational databases.
Moreover, the use of lookup keys facilitates the normalization of data, reducing redundancy and the potential for errors. This practice not only simplifies the structure of the database but also enhances the clarity of data relationships. By establishing clear key-value pairs, users can execute complex queries that yield meaningful insights, making it easier to analyze trends and patterns within the data.
In summary, adopting SQL lookup key values for columns is an essential strategy for optimizing database operations. It promotes data integrity, reduces redundancy, and enables efficient data retrieval. As organizations continue to rely on data-driven decision-making, mastering the use of lookup keys will be increasingly important for database administrators and developers alike.
Author Profile
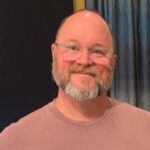
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?