How Can You Select the Most Recent Record in SQL?
In the world of database management, the ability to efficiently retrieve the most recent record is a fundamental skill that can significantly enhance the effectiveness of data analysis and reporting. Whether you’re working with a customer database, tracking sales transactions, or managing user activity logs, knowing how to select the most recent record can provide critical insights that drive decision-making. This article delves into the intricacies of SQL queries designed to pinpoint the latest entries in your datasets, ensuring you stay ahead in the data-driven landscape.
At its core, selecting the most recent record in SQL involves leveraging timestamps or date fields to filter and sort data effectively. This process can vary depending on the database structure and the specific requirements of your query. By understanding the various functions and clauses available in SQL, such as `ORDER BY`, `LIMIT`, and aggregate functions, you can craft queries that not only retrieve the latest records but also optimize performance and accuracy.
Moreover, the techniques for selecting the most recent record can differ across different SQL dialects, such as MySQL, PostgreSQL, and SQL Server. Each system may offer unique functions or syntax that can enhance your query’s efficiency. As we explore these methods, you’ll gain valuable insights into best practices and common pitfalls, empowering you to harness the full potential of your data. Get
Understanding the Query for Most Recent Records
To retrieve the most recent records from a database, you typically utilize the SQL `ORDER BY` clause in conjunction with the `LIMIT` clause. This approach allows you to sort your results based on a date or timestamp field and limit the output to just the most recent entry.
A common SQL query structure for this purpose is as follows:
“`sql
SELECT *
FROM your_table
ORDER BY your_date_column DESC
LIMIT 1;
“`
In this example, `your_table` is the name of the table from which you want to select data, and `your_date_column` is the column that contains date or timestamp values.
Using Group By to Select the Most Recent Record Per Group
When working with grouped data, such as records belonging to various categories, you may want to select the most recent record for each group. This can be achieved using a subquery or a common table expression (CTE).
For instance, if you have a table of orders and you want to find the latest order for each customer, you can employ the following SQL:
“`sql
SELECT customer_id, MAX(order_date) as latest_order
FROM orders
GROUP BY customer_id;
“`
This query returns the most recent order date for each customer. However, if you need the entire record of the most recent order for each customer, you can use a subquery:
“`sql
SELECT o.*
FROM orders o
JOIN (
SELECT customer_id, MAX(order_date) as latest_order
FROM orders
GROUP BY customer_id
) as latest_orders
ON o.customer_id = latest_orders.customer_id AND o.order_date = latest_orders.latest_order;
“`
Performance Considerations
When selecting the most recent records, performance can be a concern, especially with large datasets. Here are some tips to optimize your queries:
- Indexing: Ensure that the date or timestamp column is indexed. This will significantly speed up the sorting process.
- Avoiding SELECT *: Instead of selecting all columns, specify only the columns you need. This reduces the amount of data processed and transferred.
- Using Partitioned Queries: In some databases, you can use window functions to efficiently retrieve the most recent records without subqueries.
Here is an example using the `ROW_NUMBER()` window function:
“`sql
WITH RankedOrders AS (
SELECT *,
ROW_NUMBER() OVER (PARTITION BY customer_id ORDER BY order_date DESC) as rn
FROM orders
)
SELECT *
FROM RankedOrders
WHERE rn = 1;
“`
This method is often more efficient and easier to read, particularly when dealing with complex datasets.
Example Scenario
Consider a database table named `Employee` with the following schema:
employee_id | name | hire_date |
---|---|---|
1 | Alice | 2020-01-15 |
2 | Bob | 2021-03-22 |
3 | Charlie | 2019-07-10 |
4 | David | 2022-05-14 |
To select the most recent hire, you would use:
“`sql
SELECT *
FROM Employee
ORDER BY hire_date DESC
LIMIT 1;
“`
This query would return David’s record, as he was the most recently hired employee.
By understanding these SQL concepts and utilizing the appropriate queries, you can efficiently retrieve the most recent records from your databases, tailored to your specific needs.
Selecting the Most Recent Record in SQL
To retrieve the most recent record from a database table in SQL, several methods can be employed depending on the specific database system being utilized. Below are common approaches, including examples for better clarity.
Using the ORDER BY Clause
The `ORDER BY` clause can be used in combination with the `LIMIT` clause (or its equivalent) to fetch the latest record. This is applicable in databases like MySQL and PostgreSQL.
“`sql
SELECT *
FROM your_table
ORDER BY your_date_column DESC
LIMIT 1;
“`
Explanation:
- `your_table`: The name of the table from which you want to select the record.
- `your_date_column`: The column that contains the date or timestamp information.
- This query sorts the records in descending order based on the date column and limits the result to just one record, which will be the most recent.
Using the MAX() Function
Another approach is to use the `MAX()` function to identify the maximum date or timestamp in the table, followed by a subquery.
“`sql
SELECT *
FROM your_table
WHERE your_date_column = (SELECT MAX(your_date_column) FROM your_table);
“`
Explanation:
- This method is particularly useful when there might be multiple records with the same most recent date.
- The subquery identifies the maximum date, and the outer query selects all records that match this date.
ROW_NUMBER() for Advanced SQL Queries
In SQL Server and Oracle, the `ROW_NUMBER()` window function can be utilized to assign a unique number to each row based on a specified order.
“`sql
WITH RankedRecords AS (
SELECT *,
ROW_NUMBER() OVER (ORDER BY your_date_column DESC) AS RowNum
FROM your_table
)
SELECT *
FROM RankedRecords
WHERE RowNum = 1;
“`
Explanation:
- This approach allows for more complex queries and can easily be adjusted to retrieve the top N most recent records by changing the condition in the final `SELECT` statement.
Handling Ties in Date Values
In scenarios where multiple records share the same most recent date, it’s essential to decide how to handle these ties. Here are a few strategies:
- Select All Tied Records: Use the `MAX()` method as shown above to retrieve all records that share the most recent date.
- Additional Sorting: If additional criteria are available (e.g., ID or another relevant column), you can add further sorting to your query.
“`sql
SELECT *
FROM your_table
WHERE your_date_column = (SELECT MAX(your_date_column) FROM your_table)
ORDER BY another_column;
“`
Performance Considerations
When dealing with large datasets, the efficiency of your query becomes critical. Consider the following tips to optimize performance:
- Indexing: Ensure that the date column is indexed to improve the speed of queries that sort or filter by it.
- Limit Result Sets: Use `LIMIT` or its equivalent to restrict the number of records being processed.
- Analyze Query Execution Plans: Utilize tools provided by your database management system to analyze the performance of your queries.
Selecting the most recent record in SQL can be achieved through various methods, each suited for different scenarios and database systems. Understanding the underlying mechanisms and optimizing your queries will lead to more efficient data retrieval strategies.
Expert Insights on Selecting the Most Recent Record in SQL
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “When selecting the most recent record in SQL, it is crucial to utilize the ORDER BY clause combined with LIMIT to ensure efficiency and accuracy. This method not only retrieves the latest entry but also optimizes query performance by reducing the dataset processed.”
Mark Thompson (Senior Data Analyst, Insight Analytics). “Incorporating window functions, such as ROW_NUMBER(), can significantly enhance the process of identifying the most recent records across partitions. This approach allows for more complex queries while maintaining clarity in the results.”
Lisa Patel (SQL Consultant, Data Solutions Group). “Utilizing timestamps as a primary key for sorting records is an effective strategy. It not only simplifies the retrieval of the latest entries but also ensures data integrity and consistency throughout the database.”
Frequently Asked Questions (FAQs)
What is the SQL query to select the most recent record from a table?
To select the most recent record, you can use the `ORDER BY` clause combined with `LIMIT`. For example:
“`sql
SELECT * FROM your_table
ORDER BY date_column DESC
LIMIT 1;
“`
How can I select the most recent record for each group in SQL?
You can achieve this by using a Common Table Expression (CTE) or a subquery with `ROW_NUMBER()`. For example:
“`sql
WITH RankedRecords AS (
SELECT *, ROW_NUMBER() OVER (PARTITION BY group_column ORDER BY date_column DESC) as rn
FROM your_table
)
SELECT * FROM RankedRecords WHERE rn = 1;
“`
What is the difference between using `MAX()` and `ORDER BY` for selecting the most recent record?
Using `MAX()` retrieves the maximum value of a column, which is efficient for single-column queries. In contrast, `ORDER BY` retrieves the entire row based on the specified column, allowing access to all fields of the most recent record.
Can I select the most recent record based on multiple criteria in SQL?
Yes, you can use `ORDER BY` with multiple columns. For example:
“`sql
SELECT * FROM your_table
ORDER BY date_column DESC, another_column ASC
LIMIT 1;
“`
What if there are ties in the most recent records?
In cases of ties, you can use additional sorting criteria in your `ORDER BY` clause to determine which record to return. This ensures a consistent selection among tied records.
Is it possible to select the most recent record using a subquery?
Yes, you can use a subquery to select the most recent record. For example:
“`sql
SELECT * FROM your_table
WHERE date_column = (SELECT MAX(date_column) FROM your_table);
“`
In SQL, selecting the most recent record from a dataset is a common requirement, especially when dealing with time-stamped data. The primary methods to achieve this involve utilizing the ORDER BY clause in conjunction with the LIMIT clause, or employing window functions such as ROW_NUMBER() or RANK(). These approaches allow users to efficiently retrieve the latest entries based on a specified date or timestamp column, ensuring that the most relevant information is prioritized in queries.
Understanding the context and structure of the dataset is crucial when implementing these techniques. For instance, when using ORDER BY, it is important to ensure that the date or timestamp field is correctly indexed to optimize performance. On the other hand, window functions provide a more flexible approach, allowing for complex queries that can rank records within partitions, making it easier to handle scenarios with ties in the most recent timestamps.
Additionally, it is vital to consider the implications of selecting the most recent record in terms of data integrity and accuracy. In cases where multiple records share the same timestamp, the method of selection can impact the results. Therefore, it is advisable to define clear criteria for determining which record should be considered the “most recent” to avoid ambiguity in the output.
mastering the techniques
Author Profile
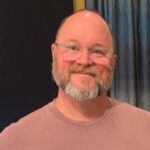
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?