How Can You Use Sapply in R to Replace Values with Else If Conditions?
In the world of data analysis, R has emerged as a powerful tool for statisticians and data scientists alike. One of its most versatile functions is `sapply`, which allows users to apply a function to each element of a list or vector, simplifying complex operations and enhancing productivity. However, as with any programming task, the need for conditional logic often arises, prompting users to explore how to effectively implement `else if` statements within the context of `sapply`. This article delves into the nuances of combining these two elements, equipping you with the knowledge to streamline your data manipulation processes.
When working with `sapply`, the ability to introduce conditional logic can significantly expand the function’s utility. Instead of merely transforming data, you can tailor outputs based on specific criteria, allowing for more nuanced analyses. Understanding how to integrate `else if` statements within `sapply` not only enhances your coding efficiency but also empowers you to handle a wider array of data scenarios. This combination can be particularly useful in situations where data categorization or conditional transformations are necessary, paving the way for cleaner, more interpretable results.
In this article, we will explore practical examples and best practices for using `sapply` alongside `else if` statements in R. Whether you’re a
Understanding sapply and Conditional Replacement
The `sapply` function in R is a powerful tool that allows users to apply a function to each element of a list or vector and return a vector or matrix. When it comes to conditional replacements, particularly using an `else if` structure, it becomes essential to understand how to implement logical conditions effectively within the `sapply` function.
To perform conditional replacements, you can use an anonymous function within `sapply`, leveraging R’s vectorized operations. This approach allows for cleaner code and improved performance over traditional loops.
Implementing Conditional Logic with sapply
Using `sapply`, you can incorporate an `ifelse` statement or a custom function to handle multiple conditions. Below is a general structure for replacing values based on conditions:
“`R
result <- sapply(your_vector, function(x) {
if (condition1) {
return(value1)
} else if (condition2) {
return(value2)
} else {
return(default_value)
}
})
```
This method allows you to specify multiple conditions effectively. For instance, you might want to replace numeric values based on specific thresholds.
Example: Replacing Numeric Values
Consider the following example where you want to categorize numerical values in a vector:
“`R
numbers <- c(4, 15, 22, 8, 19)
categorized <- sapply(numbers, function(x) {
if (x < 10) {
return("Low")
} else if (x < 20) {
return("Medium")
} else {
return("High")
}
})
```
In this example, the `categorized` variable will contain a character vector with the categories assigned based on the specified conditions.
Table of Value Replacement Conditions
The following table illustrates a sample scenario where numeric values are categorized based on defined ranges:
Range | Category |
---|---|
Less than 10 | Low |
10 to 19 | Medium |
20 and above | High |
This table provides a clear overview of the conditions that can be applied within the `sapply` function when categorizing or replacing values based on specific criteria.
Performance Considerations
While `sapply` is efficient for many tasks, it is important to be aware of its performance implications, especially with larger datasets. In cases where performance is critical, consider using `vapply`, which is more rigid but faster as it returns a vector of a specified type. Here’s how it can be implemented:
“`R
categorized <- vapply(numbers, function(x) {
if (x < 10) {
return("Low")
} else if (x < 20) {
return("Medium")
} else {
return("High")
}
}, character(1))
```
In summary, using `sapply` with conditional logic provides a flexible approach to data transformation in R. By structuring your conditions properly and leveraging R's vectorized capabilities, you can efficiently categorize or replace values within your data.
Understanding `sapply` in R
The `sapply` function in R is a user-friendly way to apply a function over a list or vector and simplify the output. It is particularly useful when you want to perform operations on each element of a vector or list, returning results in a simplified format such as a vector or matrix.
- Basic Syntax:
“`R
sapply(X, FUN, …, simplify = TRUE, USE.NAMES = TRUE)
“`
- `X`: A vector or list.
- `FUN`: The function to apply.
- `…`: Additional arguments to `FUN`.
- `simplify`: Logical value indicating if the output should be simplified.
- `USE.NAMES`: Logical value indicating if names should be preserved.
Implementing `else if` Logic within `sapply`
To implement `else if` logic in conjunction with `sapply`, you can define a custom function that incorporates conditional statements. This allows for complex evaluations on each element of your vector or list.
- Example: Categorizing numeric values
“`R
categorize <- function(x) {
if (x < 10) {
return("Low")
} else if (x < 20) {
return("Medium")
} else {
return("High")
}
}
numbers <- c(5, 15, 25)
categories <- sapply(numbers, categorize)
print(categories)
```
In this example, the `categorize` function uses `if`, `else if`, and `else` statements to classify numeric values into three categories. The `sapply` function then applies this classification across the `numbers` vector.
Using Vectorized Alternatives
While `sapply` is effective, R offers vectorized functions that can often replace the need for `sapply` in cases with simple conditions, enhancing performance.
- Using `cut`:
“`R
breaks <- c(-Inf, 10, 20, Inf)
labels <- c("Low", "Medium", "High")
categories <- cut(numbers, breaks = breaks, labels = labels)
print(categories)
```
The `cut` function categorizes data based on defined breakpoints, providing a more efficient alternative for this type of task.
Performance Considerations
When using `sapply` with `else if` statements, consider the following:
- Readability vs. Performance:
- `sapply` is easy to read and understand, but might be slower for large datasets compared to vectorized functions.
- Memory Usage:
- Vectorized functions often use less memory, as they operate on whole vectors at once rather than iterating through each element.
Method | Performance | Readability |
---|---|---|
`sapply` | Moderate | High |
Vectorized | High | Moderate |
By understanding these differences, users can make informed decisions about which method to use based on their specific needs and data size.
Expert Insights on Using Sapply in R with Else If Statements
Dr. Emily Chen (Data Scientist, R Analytics Group). “The `sapply` function in R is an incredibly powerful tool for applying a function to each element of a list or vector. When combined with `else if` statements, it allows for conditional logic to be efficiently executed across datasets. This can be particularly useful for data transformation tasks where multiple conditions need to be evaluated.”
Mark Thompson (Senior Statistician, StatTech Solutions). “Utilizing `sapply` with `else if` can streamline your data processing in R. By structuring your conditions properly, you can avoid complex loops, making your code cleaner and more readable. However, it is essential to ensure that your conditions are mutually exclusive to prevent unexpected results.”
Lisa Patel (R Programming Instructor, Data Science Academy). “Incorporating `else if` within `sapply` can enhance the functionality of your R scripts, especially in scenarios where multiple outcomes are possible based on input values. It is crucial to test your logic thoroughly to ensure that each condition is evaluated as intended, which can significantly improve the robustness of your analysis.”
Frequently Asked Questions (FAQs)
What is the purpose of the `sapply` function in R?
The `sapply` function in R is used to apply a function to each element of a list or vector and simplify the output to a vector or matrix when possible. It is particularly useful for performing operations on each element without the need for explicit loops.
How can I use `sapply` to replace values conditionally in R?
You can use `sapply` in conjunction with a custom function that includes conditional statements, such as `ifelse`, to replace values based on specific criteria. This allows for efficient element-wise operations.
Can I implement an `else if` statement within an `sapply` function?
Yes, you can implement an `else if` statement within a function passed to `sapply`. By defining a function that includes multiple conditions, you can handle various cases for value replacement.
What is the syntax for using `sapply` with conditional replacements?
The syntax typically involves defining an anonymous function within `sapply`, such as:
`sapply(vector, function(x) { if (condition1) { return(value1) } else if (condition2) { return(value2) } else { return(default_value) } })`.
Are there performance considerations when using `sapply` with complex conditions?
Yes, using `sapply` with complex conditions may lead to performance issues, especially with large datasets. In such cases, consider using vectorized operations or the `dplyr` package for better efficiency.
What are some alternatives to `sapply` for conditional replacements in R?
Alternatives to `sapply` include the `lapply` function for lists, the `ifelse` function for vectorized conditions, and the `dplyr` package’s `mutate` function for data frames, which can provide clearer syntax and improved performance.
The use of the `sapply` function in R is a powerful tool for applying a function over a list or vector and simplifying the output. When dealing with conditional logic, the incorporation of `ifelse` or similar constructs can enhance the functionality of `sapply`. This allows for the replacement of values based on specific conditions, effectively managing data transformations in a concise manner. The combination of these functions can streamline data manipulation tasks, making it easier to handle complex datasets.
Furthermore, utilizing `else if` statements within a custom function applied through `sapply` can provide greater flexibility in handling multiple conditions. This approach allows for more granular control over the logic applied to each element of the vector or list. By defining a function that includes `else if` statements, users can implement sophisticated decision-making processes directly within their data processing workflows.
In summary, the integration of `sapply` with conditional statements such as `ifelse` and `else if` is essential for efficient data manipulation in R. It not only simplifies the coding process but also enhances the readability and maintainability of the code. Mastering these techniques can significantly improve one’s ability to perform data analysis and transformation tasks in R, leading to more effective and efficient programming practices.
Author Profile
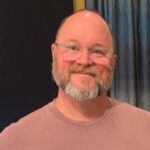
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?