Why is My Rust Program Crashing Without an Error Message?
Rust, a systems programming language known for its focus on safety and performance, has gained immense popularity among developers. However, even the most robust languages are not immune to unexpected issues. One particularly frustrating problem that many Rust developers encounter is the phenomenon of the program crashing without any error message. This perplexing scenario can leave developers scratching their heads, searching for answers in the depths of their code. In this article, we will delve into the potential causes of these silent crashes, explore strategies for troubleshooting, and provide insights to help you navigate this challenging aspect of Rust development.
When a Rust program crashes without an accompanying error message, it can be a significant roadblock in the development process. Such crashes may stem from various sources, including memory management issues, threading problems, or even external dependencies that misbehave. Understanding the underlying reasons for these crashes is crucial for developers aiming to build reliable and efficient applications. By examining common pitfalls and the intricacies of Rust’s ownership model, we can uncover the hidden factors that contribute to these silent failures.
Moreover, the absence of error messages can make diagnosing the issue particularly daunting. Developers may find themselves relying on debugging tools, logging, and systematic testing to identify the root cause of the problem. In the following sections, we will discuss
Troubleshooting Common Causes
When encountering a Rust application that crashes without an accompanying error message, it’s essential to investigate several common causes that could lead to this behavior. Understanding these factors can help pinpoint the issue and guide you toward a solution.
- Memory Management: Rust’s ownership model prevents certain errors at compile time, but runtime issues can still occur. Inspect your code for potential memory leaks or improper handling of data structures that could lead to panics.
- Threading Issues: Concurrency can introduce subtle bugs. Ensure that shared resources are correctly synchronized to prevent data races, which can lead to unpredictable behavior and crashes.
- External Dependencies: Libraries and crates can introduce instability. Verify that all dependencies are up to date, and check for any known issues or compatibility problems with the versions you are using.
- Environment Configuration: Sometimes, issues arise from the environment where the application is running. Ensure that your environment variables and configurations are correctly set up and consistent with your development setup.
Debugging Techniques
Employing effective debugging techniques can illuminate the root cause of a crash without an error message. Here are several strategies to consider:
- Verbose Logging: Incorporate logging throughout your application. This can help track the application’s state leading up to the crash. Use crates such as `log` or `env_logger` to facilitate this.
- Assertions: Use assertions liberally to catch assumptions that may not hold true. This can provide immediate feedback during development and help identify problematic areas.
- Debug Builds: Run your application in debug mode, which provides more detailed information about the execution context. This can be done by compiling with `cargo build` instead of `cargo build –release`.
- Valgrind or AddressSanitizer: Utilize tools like Valgrind or AddressSanitizer to detect memory issues. These tools can reveal problems such as buffer overflows or use-after-free errors that may not produce direct error messages.
Analyzing Core Dumps
When a Rust application crashes, it can generate a core dump, which is a snapshot of the application’s memory at the time of the crash. Analyzing core dumps can provide invaluable insights.
To enable core dumps, you can configure your system settings. Here’s a basic table illustrating how to enable core dumps on various operating systems:
Operating System | Command |
---|---|
Linux | ulimit -c unlimited |
macOS | sudo launchctl limit core unlimited |
Windows | Configure using Windows Error Reporting settings |
Once a core dump is generated, you can analyze it using a debugger like GDB. This allows you to inspect the stack trace and variables at the time of the crash, providing context for the failure.
Seeking Community Support
If you exhaust your troubleshooting efforts without finding a resolution, consider reaching out to the Rust community. Resources such as the Rust Users Forum, the Rust subreddit, or the official Rust Discord server can provide assistance. When seeking help, be sure to include:
- A clear description of the issue
- Steps to reproduce the crash
- Relevant code snippets
- Any logs or output leading up to the crash
By collaborating with the community, you may uncover insights or solutions that are not immediately apparent.
Common Causes of Rust Crashes Without Error Messages
Rust applications may crash without providing error messages due to several underlying issues. Understanding these causes can aid in troubleshooting and resolution.
- Memory Management Issues: Even though Rust has a strong emphasis on safety, it is possible to encounter issues such as:
- Dangling References: Accessing memory that has already been freed can lead to crashes.
- Stack Overflows: Excessive recursion or large stack allocations may exceed the stack size.
- Concurrency Problems: Rust’s concurrency model is designed to prevent data races, but issues may still arise:
- Deadlocks: Improper handling of locks can cause the program to freeze or crash.
- Race Conditions: Although Rust prevents data races at compile-time, logical race conditions can still occur.
- External Dependencies: Libraries written in C or other languages can cause crashes:
- FFI (Foreign Function Interface) Issues: Improper use of FFI can lead to behavior.
- Unreliable Libraries: Bugs in third-party libraries may cause instability.
- Resource Exhaustion: Running out of system resources can lead to crashes:
- Out of Memory: Excessive memory allocation can lead to crashes when the system runs out of memory.
- File Descriptors: Exceeding the limit on open file descriptors can lead to failures.
Debugging Techniques for Unexplained Crashes
Identifying the cause of a crash without error messages involves employing various debugging techniques:
- Run in Debug Mode: Executing the program with debug symbols can provide more insight.
- Use Logging: Incorporating logging at critical points can help track the application’s state:
- Log important variable values.
- Log entry and exit points of functions.
- Valgrind or AddressSanitizer: These tools can help detect memory issues:
- Run the application with Valgrind to identify memory leaks and invalid memory usage.
- Use AddressSanitizer to catch memory errors at runtime.
- GDB (GNU Debugger): Utilize GDB to analyze a core dump:
- Run the application with GDB to catch crashes in real-time.
- Analyze the backtrace to identify the function calls leading to the crash.
Best Practices to Prevent Crashes in Rust
Adopting best practices can minimize the risk of crashes in Rust applications:
- Use Rust’s Ownership System Effectively:
- Emphasize borrowing rather than ownership transfers when possible.
- Avoid using `unsafe` blocks unless absolutely necessary.
- Implement Error Handling:
- Use `Result` and `Option` types to gracefully handle potential errors.
- Employ the `?` operator to propagate errors easily.
- Testing and Code Reviews:
- Write unit tests to cover edge cases and unexpected inputs.
- Conduct peer code reviews to catch potential issues early.
- Continuous Monitoring:
- Use monitoring tools to track application performance and resource usage.
- Implement alerts for abnormal behavior or resource spikes.
Resources for Further Investigation
When facing persistent crashes in Rust applications, the following resources can provide additional support:
Resource | Description |
---|---|
Rust Documentation | Official documentation with comprehensive guides. |
Rust User Forum | Community forum for discussions and troubleshooting. |
GitHub Issues | Check for known issues in libraries you are using. |
Rustacean Principles | Guides on best practices and community standards. |
By leveraging these resources and applying sound debugging practices, developers can effectively diagnose and mitigate the impact of unexpected crashes in Rust applications.
Expert Insights on Rust Crashing Without Error Messages
Dr. Emily Carter (Senior Software Engineer, RustLang Innovations). “The absence of error messages in Rust can often be attributed to issues such as memory safety violations or improper handling of concurrency. It is crucial for developers to utilize tools like `cargo check` and `cargo clippy` to identify potential pitfalls in their code before runtime.”
Michael Chen (Lead Developer, Open Source Software Foundation). “When Rust crashes without an error message, it is often a sign of behavior or a panic that was not properly caught. Implementing robust error handling and logging mechanisms can significantly aid in diagnosing these elusive crashes.”
Sarah Thompson (Systems Architect, Tech Solutions Inc.). “In my experience, crashes without error messages can stem from external factors such as incompatible libraries or system-level issues. Ensuring that all dependencies are up-to-date and compatible with your Rust version is essential for maintaining stability.”
Frequently Asked Questions (FAQs)
What are common reasons for Rust crashing without an error message?
Rust may crash without an error message due to issues such as memory corruption, stack overflows, or improper use of unsafe code. Additionally, external dependencies or system resource limitations can contribute to unexpected crashes.
How can I debug a Rust application that crashes silently?
To debug a silently crashing Rust application, utilize debugging tools such as GDB or LLDB. Compile your code in debug mode and run it under these debuggers to identify the point of failure. Adding logging statements throughout your code can also help trace the execution flow.
Does using the `panic!` macro affect crash reporting in Rust?
Yes, the `panic!` macro can lead to crashes without detailed error messages if not handled properly. To capture panic information, consider using `std::panic::set_hook` to customize the panic behavior and log relevant details before the program exits.
Can external libraries cause Rust applications to crash without errors?
Yes, external libraries can introduce instability, especially if they contain bugs or are incompatible with your Rust version. Always ensure that dependencies are up to date and review their documentation for known issues that may affect stability.
What should I check if my Rust program crashes on specific platforms?
If your Rust program crashes on specific platforms, check for platform-specific dependencies, system configurations, and compatibility issues. Additionally, investigate any platform-specific features or APIs that may not behave consistently across environments.
Is there a way to enable more verbose error reporting in Rust?
To enable more verbose error reporting, use the `RUST_BACKTRACE` environment variable by setting it to `1`. This provides a backtrace when a panic occurs, offering more context about where the crash happened, which can aid in debugging.
Rust crashing without an error message can be a perplexing issue for developers, as it often leaves them without clear guidance on how to resolve the problem. Various factors can contribute to this phenomenon, including memory management issues, external library conflicts, or improper handling of concurrency. Understanding the underlying causes is crucial for effective troubleshooting and ensuring a stable development environment.
One of the key takeaways is the importance of leveraging Rust’s built-in safety features and tools. Utilizing the borrow checker, ownership model, and lifetimes can significantly reduce the likelihood of crashes. Additionally, employing debugging tools such as `cargo run`, `cargo test`, and external profiling tools can help identify problematic areas in the code that may lead to crashes without explicit error messages.
Furthermore, it is essential to stay updated with the latest Rust versions and community practices, as improvements and bug fixes are continually being implemented. Engaging with the Rust community through forums and discussions can also provide insights and shared experiences that may illuminate potential solutions to similar issues. By adopting a proactive approach to coding and debugging, developers can mitigate the risk of encountering crashes in their Rust applications.
Author Profile
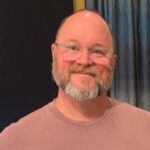
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?