How Can You Use RestSharp to Send a POST Request for Authentication in C?
In the realm of modern web development, seamless communication between client and server is paramount, particularly when it comes to authentication processes. As applications increasingly rely on APIs to manage user identities and permissions, developers must implement robust methods for sending requests and handling responses. One of the most effective tools for this task is RestSharp, a popular .NET library that simplifies HTTP communication. In this article, we will delve into the intricacies of using RestSharp to send POST requests for authentication, providing you with the essential knowledge to enhance your application’s security and functionality.
Understanding how to authenticate users via API calls is a critical skill for any developer. With RestSharp, you can streamline the process of sending POST requests, allowing for efficient handling of authentication tokens and user credentials. This library not only simplifies the syntax required for making HTTP requests but also offers built-in features for managing headers, parameters, and response data. As we explore this topic, you will discover how to leverage RestSharp’s capabilities to create secure and efficient authentication flows that enhance user experience.
Throughout this article, we will guide you through practical examples and best practices for implementing authentication using RestSharp in C#. Whether you are building a new application from scratch or integrating authentication into an existing system, understanding how to utilize RestSharp effectively will empower you
Setting Up RestSharp for Authentication
To utilize RestSharp for sending a POST request for authentication, you first need to install the RestSharp library. This can be accomplished via NuGet Package Manager in Visual Studio. The command to install the latest version is:
Install-Package RestSharp
After installing, you can start crafting your authentication requests. RestSharp provides an intuitive way to build HTTP requests, handle responses, and manage serialization/deserialization.
Creating a POST Request for Authentication
Below is an example of how to create a POST request to authenticate a user using RestSharp in C#. The example assumes the use of a JSON body format, which is commonly required for RESTful APIs.
csharp
using RestSharp;
using System;
public class AuthenticationExample
{
public static void Main(string[] args)
{
// Create a client to connect to the API
var client = new RestClient(“https://api.example.com/auth”);
// Create a request for the endpoint
var request = new RestRequest(Method.POST);
// Add headers if required (e.g., content type)
request.AddHeader(“Content-Type”, “application/json”);
// Create the JSON body for the request
var body = new
{
username = “your_username”,
password = “your_password”
};
// Add the JSON body to the request
request.AddJsonBody(body);
// Execute the request
IRestResponse response = client.Execute(request);
// Check the response status
if (response.IsSuccessful)
{
Console.WriteLine(“Authentication successful!”);
Console.WriteLine(“Response: ” + response.Content);
}
else
{
Console.WriteLine(“Authentication failed. Status: ” + response.StatusCode);
Console.WriteLine(“Error: ” + response.ErrorMessage);
}
}
}
In this code snippet, we set up a RestClient and a RestRequest. The request method is specified as POST, and the necessary headers and body are added. The response is then evaluated to determine if the authentication was successful.
Understanding Response Handling
When working with API responses, it is crucial to handle them properly. Below is a brief overview of the relevant response properties you should consider:
Property | Description |
---|---|
IsSuccessful | Indicates whether the request was successful. |
StatusCode | The HTTP status code returned by the API. |
Content | The body of the response, usually in JSON. |
ErrorMessage | Description of any error that occurred. |
This structured approach to managing responses ensures that errors are caught and handled gracefully, providing a better user experience.
Best Practices for Secure Authentication
When implementing authentication, consider the following best practices:
- Use HTTPS: Always send sensitive information over HTTPS to ensure data encryption during transmission.
- Token-Based Authentication: Consider using token-based authentication (e.g., JWT) for better security and scalability.
- Rate Limiting: Implement rate limiting to prevent abuse of the authentication endpoint.
- Error Handling: Implement robust error handling to manage authentication failures effectively.
By following these practices, you can enhance the security and reliability of your authentication mechanism using RestSharp in C#.
RestSharp Overview
RestSharp is a popular library in C# for handling HTTP requests and responses, particularly useful for working with RESTful APIs. It simplifies the process of sending requests and processing responses, making it a favored choice among developers.
Setting Up RestSharp
To begin using RestSharp, you must install the library via NuGet. This can be done by executing the following command in the Package Manager Console:
bash
Install-Package RestSharp
Alternatively, you can add it through the NuGet Package Manager in Visual Studio.
Creating a Post Request
To send a POST request for authentication, you need to create an instance of the `RestClient` and `RestRequest` classes. Here’s how to do it:
csharp
using RestSharp;
// Create a client
var client = new RestClient(“https://api.example.com”);
// Create a request
var request = new RestRequest(“auth/login”, Method.Post);
Adding Parameters
When sending authentication credentials, you typically need to pass parameters in the request body. RestSharp allows you to add parameters easily:
csharp
request.AddJsonBody(new {
username = “your_username”,
password = “your_password”
});
You can also set headers if necessary, such as for content type:
csharp
request.AddHeader(“Content-Type”, “application/json”);
Executing the Request
Once you have set up your request with the necessary parameters, you can execute it and handle the response:
csharp
var response = await client.ExecuteAsync(request);
if (response.IsSuccessful)
{
// Handle successful response
var content = response.Content; // Process the content as needed
}
else
{
// Handle errors
var errorMessage = response.ErrorMessage;
}
Handling the Response
The response from an API can come in various formats, typically JSON. RestSharp provides the functionality to deserialize the response directly into a C# object. Here’s how you can handle it:
- Define a Model: Create a class that represents the expected response structure.
csharp
public class AuthResponse
{
public string Token { get; set; }
public string UserId { get; set; }
}
- Deserialize the Response: Use RestSharp’s built-in JSON deserialization.
csharp
if (response.IsSuccessful)
{
var authResponse = JsonSerializer.Deserialize
// Use authResponse.Token or authResponse.UserId as needed
}
Common Issues and Troubleshooting
When working with RestSharp for authentication, some common issues may arise:
- Invalid Credentials: Ensure the username and password are correct.
- Incorrect Endpoint: Verify that the URL is accurate and the endpoint exists.
- Network Issues: Check for connectivity problems or server downtime.
- Unhandled Exceptions: Always implement try-catch blocks around your HTTP requests to manage unexpected exceptions.
csharp
try
{
var response = await client.ExecuteAsync(request);
// Process response
}
catch (Exception ex)
{
// Log or handle exception
}
Best Practices
- Use Asynchronous Methods: Always prefer async/await for making HTTP requests to avoid blocking the main thread.
- Secure Credentials: Never hard-code sensitive information. Use secure storage mechanisms or environment variables.
- Implement Logging: Log requests and responses for debugging and monitoring purposes.
- Validate Responses: Always validate the response to ensure it meets the expected schema before processing.
By following these guidelines and utilizing RestSharp effectively, you can simplify your authentication processes when interacting with RESTful APIs.
Expert Insights on Using RestSharp for Authentication in C#
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When implementing authentication in C# using RestSharp, it is crucial to ensure that the request headers are properly configured. This includes setting the ‘Authorization’ header with the appropriate token format, which can significantly enhance security and streamline the authentication process.”
James Liu (Lead Developer, SecureAPI Solutions). “In my experience, using RestSharp for sending POST requests during authentication is straightforward, but developers must be cautious about handling exceptions. Implementing robust error handling ensures that any issues during the authentication phase are captured and managed effectively, which is essential for maintaining application stability.”
Sarah Thompson (API Integration Specialist, CloudTech Services). “Utilizing RestSharp for authentication in C# provides a flexible approach to managing API requests. I recommend leveraging asynchronous programming to enhance performance, especially when dealing with multiple authentication requests concurrently. This can lead to a more responsive application overall.”
Frequently Asked Questions (FAQs)
What is RestSharp?
RestSharp is a popular open-source HTTP client library for .NET that simplifies the process of sending HTTP requests and handling responses, particularly for RESTful APIs.
How do I send a POST request using RestSharp?
To send a POST request using RestSharp, create an instance of `RestClient`, initialize a `RestRequest` with the desired endpoint and method (POST), add any required parameters, and then execute the request using `client.Execute(request)`.
Can I include authentication details in my POST request with RestSharp?
Yes, you can include authentication details by setting the `AddHeader` method to include authorization tokens or by using the `AddJsonBody` method to include credentials in the request body, depending on the API’s authentication requirements.
What types of authentication does RestSharp support?
RestSharp supports various authentication methods, including Basic, OAuth, and API Key authentication. You can set these methods through the `RestClient.Authenticator` property or by adding headers directly to your requests.
How do I handle the response from a POST request in RestSharp?
After executing the request, you can access the response through the `IRestResponse` object returned by `client.Execute(request)`. You can check the status code, read the content, and deserialize the response if needed.
Is it possible to send JSON data in a POST request using RestSharp?
Yes, you can send JSON data in a POST request by using the `AddJsonBody` method on the `RestRequest` object, which automatically sets the appropriate content type and serializes the object to JSON format.
In summary, utilizing RestSharp to send POST requests for authentication in C# is a straightforward process that can significantly streamline interactions with APIs. The library provides a simple and intuitive interface for constructing requests, handling responses, and managing authentication tokens. By following the proper steps, developers can efficiently implement authentication mechanisms in their applications, ensuring secure communication with web services.
Key takeaways from the discussion include the importance of setting the correct request headers, particularly for content type and authorization. Additionally, understanding how to serialize data into the appropriate format, such as JSON, is crucial for successful API interactions. Furthermore, handling exceptions and ensuring robust error management can enhance the reliability of the application during authentication processes.
Overall, RestSharp stands out as a powerful tool for developers looking to implement authentication in C#. Its ease of use, combined with comprehensive documentation and community support, makes it an excellent choice for both novice and experienced programmers. By leveraging RestSharp effectively, developers can create secure and efficient applications that meet modern authentication standards.
Author Profile
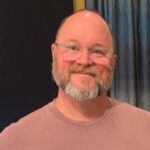
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?