Why Am I Seeing ‘Require Of Es Module Not Supported’ Error in My JavaScript Project?
In the ever-evolving landscape of web development, the transition from traditional JavaScript to ES modules marks a significant leap toward modularity and maintainability. However, as developers embrace this modern approach, they often encounter a perplexing error message: “Require of ES module not supported.” This seemingly cryptic notification can halt progress and lead to confusion, especially for those accustomed to CommonJS modules. Understanding the root causes of this issue is essential for anyone looking to harness the full power of ES modules in their projects.
The error arises from the fundamental differences between module systems in JavaScript, particularly when integrating legacy code or third-party libraries that rely on CommonJS. As ES modules gain traction, developers must navigate the nuances of compatibility and the implications for their codebase. This article delves into the intricacies of the “Require of ES module not supported” error, shedding light on its causes, potential workarounds, and best practices for a smooth transition to ES modules.
By unpacking this topic, we aim to equip developers with the knowledge they need to tackle this common stumbling block. Whether you’re a seasoned programmer or just starting your journey in JavaScript, understanding the intricacies of module systems will empower you to write cleaner, more efficient code while avoiding the pitfalls that
Understanding the ES Module System
The ECMAScript (ES) module system was introduced to enable a standardized way of structuring JavaScript code. It allows developers to import and export modules, promoting code reuse and better organization. However, the error message “Require of ES Module Not Supported” often arises when attempting to use CommonJS-style `require()` statements with ES modules, which can lead to confusion.
When using ES modules, the following syntax is essential:
- Importing Modules:
“`javascript
import { moduleName } from ‘./module.js’;
“`
- Exporting Modules:
“`javascript
export const moduleName = ‘value’;
“`
This contrasts with CommonJS, where the syntax is:
- Requiring Modules:
“`javascript
const moduleName = require(‘./module’);
“`
- Exporting Modules:
“`javascript
module.exports = moduleName;
“`
Understanding these differences is crucial for effectively navigating the module system.
Common Causes of “Require Of ES Module Not Supported”
The error typically occurs in the following scenarios:
- Using `require()` in ES Modules: Attempting to use the `require()` function in files that are treated as ES modules results in this error.
- File Extensions: ES modules usually require the `.mjs` extension or the `”type”: “module”` declaration in the `package.json` file.
- Mixing Module Types: Mixing CommonJS and ES module syntax within the same file or project can lead to compatibility issues.
To effectively resolve these issues, developers should ensure consistent use of either ES modules or CommonJS across their project.
Resolving the Error
To address the “Require of ES Module Not Supported” error, consider the following solutions:
- Use ES Module Syntax: Replace `require()` with `import` statements.
- Check File Extensions: Ensure that your files are using the appropriate extensions (`.mjs` or `.js` with the correct `package.json` configuration).
- Update Node.js: Make sure you are using a version of Node.js that supports ES modules (Node.js 12.x and above).
Here is a quick reference table to help distinguish between CommonJS and ES Modules:
Feature | CommonJS | ES Module |
---|---|---|
Syntax for Imports | const module = require(‘module’); | import module from ‘module’; |
Syntax for Exports | module.exports = value; | export const value = ‘value’; |
File Extension | .js | .mjs or .js (with “type”: “module”) |
Support for Asynchronous Loading | No | Yes (dynamic import available) |
By following these guidelines, developers can effectively avoid the pitfalls associated with module importation in JavaScript, leading to smoother development processes.
Understanding ES Module Support
ES Modules (ECMAScript Modules) are a standardized module system in JavaScript that allow for the import and export of code between different files. However, there are several scenarios where you might encounter the error message `Require of ES Module Not Supported`. This typically arises when mixing ES Modules with CommonJS modules in environments that do not support such interoperability.
Common Causes of the Error
The error can stem from various issues, including:
- Improper File Extension: ES Modules should generally be saved with the `.mjs` extension, while CommonJS modules use `.js`.
- Incorrect Module Type in `package.json`: The `type` field in your `package.json` file must be set to `”module”` for Node.js to recognize files as ES Modules.
- Usage of `require` with ES Modules: ES Modules cannot be loaded using `require()` as it is specific to CommonJS. Instead, the `import` statement should be used.
How to Resolve the Error
To fix the `Require of ES Module Not Supported` error, consider the following solutions:
- Change File Extensions: Rename your files to use the `.mjs` extension if they are ES Modules. This informs the Node.js runtime to treat these files as modules.
- Update `package.json`: Ensure your `package.json` includes the following line:
“`json
{
“type”: “module”
}
“`
- Use Import Instead of Require: Replace any `require` statements with `import` syntax. For example:
“`javascript
// Incorrect for ES Module
const myModule = require(‘./myModule’);
// Correct for ES Module
import myModule from ‘./myModule.js’;
“`
Best Practices for Using ES Modules
To prevent encountering the error in the future, follow these best practices:
- Consistent Module Type: Stick to either ES Modules or CommonJS throughout your project to avoid compatibility issues.
- Use Modern Tools: Utilize build tools like Babel or Webpack that can transpile or bundle your code, ensuring compatibility across different module systems.
- Check Node.js Version: Ensure you are using a version of Node.js that supports ES Modules natively (Node.js 12.x and above).
Example of Working with ES Modules
Below is an example illustrating the proper usage of ES Modules:
**module.mjs**:
“`javascript
export const greet = (name) => {
return `Hello, ${name}!`;
};
“`
main.mjs:
“`javascript
import { greet } from ‘./module.mjs’;
console.log(greet(‘World’));
“`
This setup will work seamlessly if both files are properly named and the environment is configured to handle ES Modules.
Additional Resources
For further reading and deeper understanding of ES Modules and related topics, consider the following resources:
Resource | Description |
---|---|
MDN Web Docs – ES Modules | Comprehensive documentation on ES Modules |
Node.js Documentation – Modules | Official guide on using modules in Node.js |
Babel Documentation | Learn about transpiling ES Modules for older environments |
Webpack Documentation | Guide on bundling JavaScript applications |
Understanding the Challenges of ES Module Support
Dr. Emily Chen (Software Architect, Tech Innovations Inc.). “The error message ‘Require Of ES Module Not Supported’ typically arises due to the incompatibility between CommonJS and ES Module syntax. Developers must ensure that their environments support ES Modules, especially when transitioning from older Node.js versions.”
James Patel (Senior JavaScript Developer, CodeCraft Solutions). “It’s crucial to recognize that the ES Module system is designed for modern JavaScript development. When encountering this error, one should verify the module type in the package.json file and consider using ‘import’ statements instead of ‘require’.”
Lisa Tran (Lead Frontend Engineer, WebDev Agency). “Many developers face this issue when trying to integrate legacy code with new ES Module features. A thorough understanding of module interoperability is essential, and adopting a consistent module strategy can mitigate these errors.”
Frequently Asked Questions (FAQs)
What does “Require Of Es Module Not Supported” mean?
This error indicates that the CommonJS `require` syntax is being used to import an ES module, which is not supported in environments that strictly adhere to ES module standards.
How can I resolve the “Require Of Es Module Not Supported” error?
To resolve this error, switch to using the `import` statement instead of `require` for importing ES modules. Ensure your environment supports ES modules, or adjust your module type in the package configuration.
What environments typically produce the “Require Of Es Module Not Supported” error?
This error commonly occurs in Node.js environments when trying to mix CommonJS and ES module syntax without proper configuration, particularly in versions that enforce module types.
Can I use both CommonJS and ES modules in the same project?
Yes, but you must configure your project correctly. You can use a transpiler like Babel or set your Node.js environment to support both module types, ensuring proper import/export syntax is followed.
What are the differences between CommonJS and ES modules?
CommonJS uses `require` and `module.exports` for module management, while ES modules utilize `import` and `export` syntax. ES modules also support asynchronous loading and are designed for modern JavaScript environments.
Is there a way to convert CommonJS modules to ES modules?
Yes, you can manually convert CommonJS modules by replacing `require` statements with `import` and `module.exports` with `export`. Alternatively, use tools like Babel to automate the conversion process.
The error message “Require Of Es Module Not Supported” typically arises in JavaScript environments where there is an attempt to use CommonJS `require` statements to import ES modules. This incompatibility stems from the differing module systems in JavaScript, where ES modules utilize `import` and `export` syntax, while CommonJS employs `require` and `module.exports`. As modern JavaScript development increasingly shifts towards ES modules for their benefits in static analysis and tree-shaking, understanding this distinction is essential for developers to avoid runtime issues.
One of the key insights from the discussion surrounding this error is the importance of configuring the environment correctly. Developers must ensure that their Node.js version supports ES modules and that the appropriate settings, such as using the `.mjs` file extension or including `”type”: “module”` in the `package.json`, are in place. This adjustment allows for seamless integration of ES module syntax within projects that may have previously relied on CommonJS.
Furthermore, transitioning from CommonJS to ES modules can provide significant advantages, such as improved interoperability with modern JavaScript tooling and frameworks. By embracing ES modules, developers can leverage features like better support for asynchronous loading and enhanced code organization. Thus, addressing the “Require Of Es Module
Author Profile
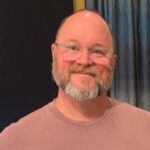
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?