Why is ‘require’ Not Defined in ES Module Scope?
In the ever-evolving landscape of JavaScript, the transition from CommonJS to ES Modules has sparked both excitement and confusion among developers. As modern applications increasingly embrace the modular approach, the error message “Require is not defined in ES Module scope” has become a common stumbling block for many. This seemingly cryptic message can halt development in its tracks, leaving programmers to grapple with the nuances of module loading and the differences between module systems. Understanding this error is essential for anyone looking to harness the full potential of ES Modules in their projects.
At its core, this error highlights a fundamental distinction between the two module systems: CommonJS, which relies on the `require()` function, and ES Modules, which utilize the `import` statement. As developers migrate their codebases to adopt ES Modules, they may encounter this issue when attempting to use `require()` in an environment that strictly adheres to the ES Module syntax. This oversight can lead to frustration, particularly for those accustomed to the more permissive nature of CommonJS.
Navigating this error involves not only understanding the technical differences between module systems but also adapting to the best practices that come with ES Modules. As we delve deeper into the intricacies of this topic, we will explore the causes of this error, practical
Understanding ES Modules
In JavaScript, ES Modules (ECMAScript Modules) provide a standardized way to structure and load modules. Unlike CommonJS, which uses `require()` to import modules, ES Modules utilize the `import` statement. This distinction is crucial as it affects how scripts are executed and how dependencies are managed within a project.
Key characteristics of ES Modules include:
- Static Structure: Imports are resolved at compile time, allowing tools to optimize code more efficiently.
- Lexical Scoping: Variables defined in an ES Module are scoped to the module itself, preventing global namespace pollution.
- Support for Asynchronous Loading: ES Modules can be loaded asynchronously, improving performance in web applications.
Common Errors with ES Modules
One prevalent error developers encounter when transitioning from CommonJS to ES Modules is the message `require is not defined in ES Module scope`. This error signifies that the `require()` function, which is standard in CommonJS, cannot be utilized in an ES Module context.
Reasons for this error include:
- Incorrect File Extension: Ensure that your module files use the `.mjs` extension or that your `package.json` specifies `”type”: “module”`.
- Mixing Module Types: Attempting to use CommonJS syntax in an ES Module leads to compatibility issues.
- Unrecognized Context: The environment or runtime may not support ES Modules, especially in older Node.js versions.
Resolving the Error
To effectively resolve the `require is not defined in ES Module scope` error, consider the following strategies:
- Use Import Instead of Require: Replace instances of `require()` with `import`. For example:
“`javascript
// CommonJS
const express = require(‘express’);
// ES Module
import express from ‘express’;
“`
- Adjust Your Environment: Ensure that your runtime environment supports ES Modules. This might involve updating Node.js or configuring Babel for transpilation.
- Check for Mixed Syntax: Audit your code for any remaining CommonJS syntax and refactor it to be consistent with ES Module standards.
Example of Module Conversion
Consider a scenario where you have a CommonJS module that needs to be converted to an ES Module. Below is a comparison of the two formats.
CommonJS | ES Module |
---|---|
const fs = require('fs'); module.exports = function readFile(filePath) { return fs.readFileSync(filePath, 'utf8'); }; |
import fs from 'fs'; export default function readFile(filePath) { return fs.readFileSync(filePath, 'utf8'); } |
By following these guidelines and understanding the fundamental differences between module types, developers can navigate the transition to ES Modules with confidence, minimizing the occurrence of scope-related errors.
Understanding ES Modules
ES Modules (ECMAScript Modules) are a standardized way to structure JavaScript code for better modularity and organization. Unlike CommonJS, which uses `require`, ES Modules utilize the `import` and `export` syntax. This fundamental difference impacts how modules are loaded and executed.
Key Features of ES Modules:
- Static Structure: The `import` and `export` statements are hoisted, allowing for static analysis and tree-shaking.
- File Extension: ES Modules typically use the `.mjs` extension, though `.js` can also be used when specified in the `package.json` file.
- Asynchronous Loading: ES Modules can be loaded asynchronously in environments that support it, improving performance.
Common Error: Require Is Not Defined
When using ES Modules, developers may encounter the error `require is not defined in ES module scope`. This error arises when attempting to use the CommonJS `require` function in a module that is defined as an ES Module.
Reasons for the Error:
- Module Type: The script is being treated as an ES Module, which does not support CommonJS syntax.
- Incorrect File Extensions: Using the `.js` file extension without specifying `type: “module”` in the `package.json` can default to CommonJS.
- Mixing Module Systems: Attempting to use CommonJS functions within a purely ES Module environment can cause this error.
How to Resolve the Error
To eliminate the `require is not defined in ES module scope` error, consider the following approaches:
- Use `import` Instead of `require`:
Replace all instances of `require` with the ES Module `import` syntax. For example:
“`javascript
// CommonJS
const express = require(‘express’);
// ES Module
import express from ‘express’;
“`
- Specify Module Type in `package.json`:
Ensure your `package.json` file includes `”type”: “module”` to indicate that your project is using ES Modules.
“`json
{
“type”: “module”
}
“`
- Use Dynamic Imports:
If you need to conditionally load modules, use dynamic imports:
“`javascript
const moduleName = await import(‘./module.js’);
“`
Using CommonJS in ES Module Environment
If you must use CommonJS modules within an ES Module environment, follow these strategies:
Strategy | Description |
---|---|
Transpilation | Use tools like Babel to transpile ES Modules to CommonJS. |
Hybrid Approach | Create a wrapper module that uses `require` and exports as ES Module. |
Top-Level `await` | In environments supporting top-level `await`, you can import CommonJS modules dynamically. |
Example of Hybrid Approach:
“`javascript
// wrapper.js
const commonJsModule = require(‘./commonJsModule.js’);
export default commonJsModule;
“`
By adhering to these guidelines, developers can effectively navigate the differences between ES Modules and CommonJS, minimizing the occurrence of errors related to module scope.
Understanding the Scope of ES Modules and the ‘Require’ Function
Dr. Emily Chen (JavaScript Framework Specialist, Tech Innovations Inc.). “The error message ‘Require Is Not Defined In ES Module Scope’ typically arises when developers attempt to use CommonJS syntax in an ES Module environment. It highlights the fundamental differences between these two module systems, emphasizing the need for developers to adapt their code to the ES Module syntax, such as using ‘import’ statements instead of ‘require’.”
James Patel (Senior Software Engineer, Modern Web Solutions). “Incorporating ES Modules into a project requires a clear understanding of how module loading works. The ‘require’ function is not available in ES Module scope because it is part of the CommonJS module system. Developers must ensure that their environment supports ES Modules, or they must transpile their code using tools like Babel to maintain compatibility.”
Linda Gomez (Lead Frontend Developer, CodeCraft Agency). “To resolve the ‘Require Is Not Defined In ES Module Scope’ issue, it is crucial to refactor the codebase to utilize ES Module syntax. This not only resolves the error but also positions the application to take advantage of modern JavaScript features, enhancing performance and maintainability.”
Frequently Asked Questions (FAQs)
What does “Require Is Not Defined In Es Module Scope” mean?
This error indicates that the `require` function, which is commonly used in CommonJS modules, is not available in ECMAScript (ES) module scope. ES modules utilize `import` and `export` statements instead.
Why is the `require` function not available in ES modules?
The `require` function is part of the CommonJS module system, which is different from the ES module system. ES modules are designed to be statically analyzable, allowing for better optimization and tree-shaking, which is not compatible with the dynamic nature of `require`.
How can I resolve the “Require Is Not Defined In Es Module Scope” error?
To resolve this error, replace `require` statements with `import` statements. Ensure that your JavaScript files are recognized as ES modules, either by using the `.mjs` extension or by specifying `”type”: “module”` in your `package.json`.
Can I use both CommonJS and ES modules in the same project?
Yes, you can use both module systems in the same project, but you must be cautious about how they interact. You can import CommonJS modules into ES modules using dynamic `import()`, but the reverse is not straightforward.
What are the benefits of using ES modules over CommonJS?
ES modules provide several advantages, including improved performance due to static analysis, better support for asynchronous loading, and a more standardized syntax that aligns with modern JavaScript practices.
Is it possible to convert existing CommonJS code to ES modules?
Yes, converting CommonJS code to ES modules involves changing `require` statements to `import`, `module.exports` to `export`, and ensuring that the module file is compatible with ES module syntax. Tools like Babel can assist in this conversion.
The error message “Require is not defined in ES module scope” typically arises when attempting to use CommonJS syntax in an ECMAScript (ES) module context. This issue reflects the fundamental differences between the two module systems in JavaScript. CommonJS, which relies on the `require` function for importing modules, contrasts with ES modules that utilize the `import` statement. As JavaScript has evolved, the ES module system has become the standard for module management, particularly in modern applications and frameworks.
To resolve this error, developers must adapt their code to the ES module syntax. This involves replacing `require` statements with `import` statements and ensuring that the module files are saved with the `.mjs` extension or that the `type` field in the `package.json` file is set to `module`. By making these adjustments, developers can effectively leverage the benefits of ES modules, such as improved performance and better support for asynchronous loading.
understanding the distinction between CommonJS and ES modules is crucial for JavaScript developers. As the community increasingly embraces ES modules, adapting to this new standard will enhance code maintainability and compatibility with modern JavaScript features. Developers should familiarize themselves with the ES module syntax to avoid common pitfalls
Author Profile
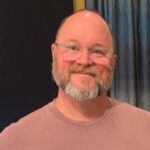
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?