How Can I Fix the ‘ReferenceError: React Is Not Defined’ Issue in My Project?
In the dynamic world of web development, React has emerged as a powerhouse for building user interfaces, allowing developers to create seamless, interactive experiences. However, as with any powerful tool, it comes with its own set of challenges. One common issue that developers encounter is the dreaded `ReferenceError: React is not defined`. This error can be a frustrating roadblock, especially for those who are new to the framework or are transitioning from other JavaScript libraries. Understanding the root causes of this error is crucial for any developer looking to harness the full potential of React.
When you see the `ReferenceError: React is not defined`, it often indicates that the React library has not been properly imported or is missing from your project. This seemingly simple oversight can halt your development process, leaving you puzzled and searching for answers. The error can arise from various scenarios, including improper configuration, missing dependencies, or even typos in your code. As you delve deeper into this topic, you’ll discover the common pitfalls that lead to this error and the best practices to avoid them.
Moreover, resolving this issue is not just about fixing a single error; it’s about understanding the foundational principles of how React operates within your application. By addressing the `ReferenceError`, you will not only enhance your debugging skills
Understanding the Error
The `ReferenceError: React is not defined` typically arises when the React library has not been properly imported into the file where it is being utilized. This error is common among developers who are new to React or those who are working in environments where module imports are not correctly configured. React must be explicitly imported in every file that uses it.
To resolve this error, ensure that the following import statement is present at the top of your JavaScript file:
“`javascript
import React from ‘react’;
“`
If you are using React 17 or newer, you may not need to import React explicitly for JSX. However, it’s good practice to include it if you’re using hooks or other React features.
Common Causes
Several scenarios can lead to this error appearing in your application:
- Missing Import Statement: Failing to include the import statement for React.
- Incorrect Project Configuration: Issues in your project’s configuration files, like `webpack.config.js` or `babel.config.js`, that prevent React from being recognized.
- File Structure: If your file structure is not set up correctly, it may lead to modules not being found.
- Development Environment: Using an older version of React or a misconfigured development environment can also lead to this error.
How to Fix the Error
To troubleshoot and resolve the `ReferenceError: React is not defined`, follow these steps:
- Check Import Statements: Ensure that React is imported in every file that uses it.
- Verify Project Configuration: Review your configuration files to ensure they are set up correctly for React.
- Inspect File Structure: Make sure your project structure aligns with best practices and that files are correctly located.
- Update Packages: Ensure that you are using the latest version of React and other related packages.
Example of Correct Usage
Here is a simple example of a functional component that correctly imports React:
“`javascript
import React from ‘react’;
const MyComponent = () => {
return
;
};
export default MyComponent;
“`
Best Practices
To prevent encountering the `ReferenceError: React is not defined`, consider adopting the following best practices:
- Always import React when using JSX syntax.
- Keep your dependencies updated to the latest versions.
- Utilize a linter to catch errors in your code before runtime.
- Organize your project files to facilitate easier imports.
Tools for Debugging
When faced with this error, several tools can assist in debugging:
Tool | Description |
---|---|
ESLint | A static code analysis tool for identifying problematic patterns in JavaScript. |
React DevTools | A browser extension that allows you to inspect the React component hierarchy in the React application. |
Browser Console | The built-in developer tools in web browsers can help trace errors and log messages. |
Using these tools will help streamline your debugging process and enhance your development efficiency.
Understanding the Error
The `ReferenceError: React is not defined` typically occurs when the React library is not properly imported in your JavaScript or JSX files. This error indicates that the JavaScript engine cannot find the React object, which is essential for rendering components.
Common Causes
Several factors can lead to this error:
- Missing Import Statement: The most common cause is the absence of an import statement for React in your component file.
- Incorrect Import Path: If React is imported incorrectly, the reference may not resolve, leading to this error.
- Build Configuration Issues: Misconfigurations in your build setup (like Webpack or Babel) can also prevent React from being recognized.
- Scope Issues: If React is defined in a different scope or file and not properly passed or imported, this error will occur.
Fixing the Error
To resolve the `ReferenceError: React is not defined`, consider the following solutions:
- Add the Import Statement: Ensure that you have the import statement for React at the top of your file:
“`javascript
import React from ‘react’;
“`
- Check Import Path: Verify that the path to the React library is correct. If you are using a package manager like npm, ensure that React is installed:
“`bash
npm install react
“`
- Verify Build Configuration: Check your build tools (e.g., Webpack, Babel) to ensure they are correctly set up to handle React and JSX. Make sure you have the necessary presets and plugins configured.
- Use React in the Right Scope: Ensure that your usage of React is within the correct scope. If you are using functional components or hooks, make sure React is imported in each file where it is used.
Example of Proper Usage
Here’s an example of a functional component with a proper React import:
“`javascript
import React from ‘react’;
const MyComponent = () => {
return (
Hello, World!
);
};
export default MyComponent;
“`
Testing the Solution
After making the necessary changes, test your application:
- Run Your Development Server: Use `npm start` or your specific command to run the server.
- Check Console for Errors: Open the browser’s console to see if the error persists.
- Inspect Build Output: If using a build tool, inspect the output for any issues related to React.
Preventive Measures
To avoid encountering this error in the future, consider the following best practices:
- Consistent Imports: Always import React in every file that uses JSX.
- Linting Tools: Utilize ESLint with appropriate plugins to catch missing imports before runtime.
- Documentation and Community: Refer to official React documentation for updates on best practices and import management. Engage with community forums for shared experiences and solutions.
By understanding the causes and implementing the fixes outlined above, you can effectively resolve the `ReferenceError: React is not defined` error and maintain a smoother development experience with React.
Expert Insights on Resolving “ReferenceError: React is Not Defined”
Emily Chen (Senior Frontend Developer, Tech Innovations Inc.). “The ‘ReferenceError: React is Not Defined’ typically indicates that the React library has not been properly imported into your project. It is crucial to ensure that you include the React import statement at the top of your component files to avoid this error.”
Michael Torres (JavaScript Educator, Code Academy). “This error often arises when developers forget to import React in functional components, especially in newer versions of React where JSX is used. Always verify your imports, and consider using ESLint to catch such issues early in the development process.”
Sarah Patel (Software Engineer, Open Source Contributor). “In addition to ensuring that React is imported, it is essential to check your project’s configuration, such as Babel or Webpack settings. Misconfigurations can lead to this error, especially in environments where code splitting or lazy loading is employed.”
Frequently Asked Questions (FAQs)
What does the error “ReferenceError: React is not defined” mean?
This error indicates that the React library has not been properly imported or is not accessible in the current scope of your JavaScript file.
How can I resolve the “ReferenceError: React is not defined” error?
To resolve this error, ensure that you have imported React at the top of your file using `import React from ‘react’;` or `const React = require(‘react’);` depending on your module system.
Is it necessary to import React in every file that uses JSX?
Yes, it is necessary to import React in every file that uses JSX, as JSX syntax gets transformed into React function calls, which require React to be in scope.
What should I do if I have imported React but still see this error?
If you have imported React and still encounter this error, check for typos in your import statement, ensure that the import statement is placed before any JSX code, and verify that your build tools are configured correctly.
Can this error occur in a functional component?
Yes, this error can occur in any functional component that uses JSX without the proper import of React.
Does this error affect class components as well?
Yes, class components also require React to be imported, as they rely on the React library for lifecycle methods and rendering.
The error message “ReferenceError: React is not defined” typically indicates that the React library has not been properly imported or is not available in the scope where it is being used. This issue often arises in JavaScript applications, particularly those built with React, when developers forget to include the necessary import statement at the top of their component files. Without this import, the JavaScript engine cannot recognize the React object, leading to the ReferenceError.
To resolve this error, developers should ensure that they have the correct import statement, such as `import React from ‘react’;`, included in their component files. Additionally, it is important to verify that the React library is correctly installed in the project, which can be checked through the project’s package.json file or by running a package manager command like `npm list react` or `yarn list react`. Ensuring that React is included in the project dependencies is crucial for the successful execution of React components.
Another key takeaway is that this error can also occur in environments where React is expected to be globally available but is not, such as in certain configurations of build tools or when using React in a non-standard setup. Developers should be mindful of their project configuration, including Webpack or Babel settings, to
Author Profile
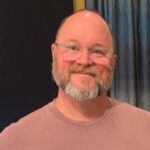
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?