Why Was ‘waitFor’ Removed from React Testing Library and What Does It Mean for Your Tests?
In the ever-evolving landscape of web development, testing frameworks play a crucial role in ensuring that applications function as intended. Among these frameworks, React Testing Library (RTL) has gained significant traction for its user-centric approach to testing React components. However, as with any technology, updates and changes are inevitable. One such change that has stirred conversations within the developer community is the removal of the `waitFor` utility from RTL. This shift raises important questions about testing strategies and how developers can adapt to maintain robust testing practices.
The removal of `waitFor` from React Testing Library marks a pivotal moment for developers who rely on this tool to manage asynchronous operations in their tests. As applications become increasingly complex, the need for effective testing methods that can handle dynamic content and state changes is more critical than ever. The implications of this change are far-reaching, prompting developers to rethink their testing approaches and explore alternative solutions to ensure their applications remain reliable and performant.
In this article, we will delve into the reasons behind the removal of `waitFor`, its impact on testing methodologies, and the best practices that can be adopted in light of this change. By understanding the context and implications of this update, developers can better navigate the evolving landscape of React testing and continue to produce high-quality, maintainable
Understanding the Changes in React Testing Library
The React Testing Library has undergone significant changes recently, particularly with the removal of the `waitFor` function. This function was widely used to handle asynchronous updates in component testing. However, its removal has been a topic of discussion among developers, leading to questions about how to adapt existing tests and the implications for future testing strategies.
Reasons for the Removal of `waitFor`
The decision to remove `waitFor` was driven by several key factors:
- Simplicity: The React Testing Library aims to provide a simpler API that encourages developers to write tests that are more aligned with user behavior.
- Encouraging Best Practices: The removal promotes the use of more explicit waiting strategies, which can lead to more readable and maintainable tests.
- Performance Improvements: Eliminating `waitFor` can enhance performance by reducing unnecessary re-renders and checks during testing.
Alternative Approaches to Handle Asynchronous Testing
With `waitFor` no longer available, developers must adopt alternative strategies for managing asynchronous behavior in their tests. Here are some recommended approaches:
– **Using `findBy` Queries**: The `findBy` queries automatically wait for an element to appear in the DOM before proceeding. This is a straightforward way to handle async components.
“`javascript
const element = await screen.findByText(/your text/i);
“`
– **Utilizing `waitForElementToBeRemoved`**: This function can be employed to wait for an element to be removed from the DOM, ensuring that your test only continues once the element is gone.
“`javascript
await waitForElementToBeRemoved(() => screen.getByText(/loading/i));
“`
- Manual Promises: You can also use manual promises to control asynchronous behavior, though this is less common.
Transitioning Tests to New Patterns
As the React Testing Library evolves, it is essential to refactor existing tests to align with the new patterns. Below is a comparison of old and new approaches to illustrate this transition:
Old Approach | New Approach |
---|---|
await waitFor(() => { expect(screen.getByText(/data loaded/i)).toBeInTheDocument(); }); |
const element = await screen.findByText(/data loaded/i); expect(element).toBeInTheDocument(); |
await waitFor(() => screen.getByText(/loading/i)); |
await waitForElementToBeRemoved(() => screen.getByText(/loading/i)); |
By updating tests to use these new methods, developers can ensure that their testing practices remain effective and in line with the library’s evolving standards. These changes not only simplify testing strategies but also enhance the overall quality and reliability of the tests.
Understanding the Removal of `waitFor` in React Testing Library
The `waitFor` function in React Testing Library has been a commonly used method for handling asynchronous operations in tests. Its removal has prompted discussions regarding alternative approaches to testing in React applications.
Reasons for Removal
– **Simplification of API**: The React Testing Library aims to provide a simpler API that encourages best practices. Removing `waitFor` helps streamline testing patterns.
– **Encouraging Better Practices**: Developers are encouraged to rely on more explicit asynchronous patterns, such as using `findBy` queries, which inherently wait for elements to appear in the DOM.
– **Performance Considerations**: The removal can lead to more efficient tests, as reliance on `waitFor` may lead to unnecessary waits and potential performance bottlenecks.
Alternatives to `waitFor`
Instead of using `waitFor`, developers can use several alternatives that align with the library’s philosophy of testing user interactions and application behavior.
– **`findBy` Queries**: These queries automatically wait for elements to appear.
- Example:
“`javascript
const button = await screen.findByRole(‘button’, { name: /submit/i });
“`
– **`waitForElementToBeRemoved`**: This method is beneficial when you expect an element to disappear.
- Example:
“`javascript
await waitForElementToBeRemoved(() => screen.queryByText(/loading/i));
“`
– **`act` Utility**: For more complex scenarios where multiple state updates occur, wrapping logic in `act` can ensure that all updates are processed.
- Example:
“`javascript
await act(async () => {
// Perform actions that trigger updates
});
“`
Migration Strategies
To adapt to the removal of `waitFor`, consider the following strategies:
– **Refactor Test Cases**: Review existing tests that utilize `waitFor` and refactor them to use `findBy` or other built-in utilities.
– **Utilize `async/await` Patterns**: Ensure that asynchronous test patterns are clearly defined, making use of `async` functions to handle promises effectively.
– **Leverage Custom Queries**: If specific waiting behavior is needed beyond built-in methods, consider creating custom queries that encapsulate waiting logic.
Example Transition
Here’s a simple before-and-after example demonstrating the transition from `waitFor` to a more effective approach:
**Before Removal:**
“`javascript
import { render, screen, waitFor } from ‘@testing-library/react’;
import MyComponent from ‘./MyComponent’;
test(‘loads and displays greeting’, async () => {
render(
await waitFor(() => expect(screen.getByText(/hello/i)).toBeInTheDocument());
});
“`
**After Removal:**
“`javascript
import { render, screen } from ‘@testing-library/react’;
import MyComponent from ‘./MyComponent’;
test(‘loads and displays greeting’, async () => {
render(
expect(await screen.findByText(/hello/i)).toBeInTheDocument();
});
“`
Best Practices Moving Forward
- Focus on User-Centric Testing: Always prioritize tests that mimic user behavior and interactions.
- Stay Updated: Keep abreast of changes in the React Testing Library and adjust practices accordingly.
- Test Readability: Aim for tests that are easy to read and understand, enhancing maintainability and collaboration.
By adopting these alternatives and strategies, developers can ensure that their testing practices remain robust and effective in the absence of `waitFor`.
Expert Insights on the Removal of WaitFor in React Testing Library
Dr. Emily Carter (Senior Software Engineer, Testing Innovations Inc.). The removal of WaitFor from React Testing Library is a significant shift in testing practices. It encourages developers to adopt more declarative testing strategies, reducing reliance on arbitrary waits and promoting better synchronization with the component’s state.
Michael Chen (Lead Developer Advocate, React Community). The decision to remove WaitFor aligns with the library’s philosophy of encouraging best practices. By eliminating this function, developers are pushed to rethink their testing approaches and utilize more robust methods for handling asynchronous operations.
Sarah Thompson (Quality Assurance Specialist, Code Quality Solutions). While the removal of WaitFor may initially seem disruptive, it ultimately serves to streamline the testing process. Developers will need to focus on writing cleaner, more maintainable tests that accurately reflect user interactions without relying on timing hacks.
Frequently Asked Questions (FAQs)
What changes were made to the `waitFor` function in React Testing Library?
The `waitFor` function has undergone some modifications to improve its performance and usability. The updates include changes to the default timeout and the way it handles asynchronous operations, making it more intuitive for developers.
Why was `waitFor` removed in certain scenarios?
`waitFor` was removed in specific scenarios to streamline the testing process and reduce unnecessary complexity. The goal was to encourage the use of more straightforward alternatives that better align with React’s rendering behavior.
What should I use instead of `waitFor`?
Developers can use `findBy` queries or `waitForElementToBeRemoved` as alternatives to `waitFor`. These options provide a more declarative approach to handling asynchronous elements in tests.
How do the changes to `waitFor` affect existing tests?
Existing tests may require refactoring to accommodate the changes. Developers should review their test cases and replace deprecated usages of `waitFor` with the recommended alternatives to ensure continued functionality.
Are there any performance improvements with the new approach?
Yes, the new approach aims to enhance performance by reducing the overhead associated with waiting for elements. This results in faster test execution and a more efficient testing process overall.
Where can I find the latest documentation on React Testing Library?
The latest documentation is available on the official React Testing Library website. It includes comprehensive guides, examples, and updates regarding the changes and best practices for testing React applications.
In recent updates to the React Testing Library, the `waitFor` utility has undergone significant changes, reflecting the library’s ongoing evolution to improve testing practices. The primary focus of these changes is to enhance the reliability and clarity of tests by encouraging developers to adopt more precise and explicit querying methods. The removal of certain functionalities associated with `waitFor` signifies a shift towards promoting better practices in asynchronous testing, ensuring that tests remain stable and easier to understand.
One of the key takeaways from the discussion surrounding the removal of `waitFor` is the emphasis on using `findBy` queries instead. These queries inherently handle asynchronous operations, allowing developers to write cleaner and more maintainable tests without relying on the more generic `waitFor`. This transition not only streamlines the testing process but also reduces the potential for flaky tests that can arise from improper use of waiting mechanisms.
Furthermore, the changes encourage developers to rethink their testing strategies by focusing on the end state of the UI rather than the timing of events. This approach aligns with best practices in testing, promoting a more user-centric perspective that prioritizes the actual behavior of the application. Overall, the removal of `waitFor` and the shift towards more explicit querying methods represent a positive step
Author Profile
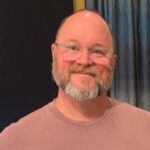
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?