How Can You Run Another Python Script Outside of the Main Thread?
In the world of Python programming, the ability to run scripts concurrently can significantly enhance the efficiency and responsiveness of your applications. Whether you’re developing a web server that needs to handle multiple requests or a data processing application that must perform tasks in parallel, understanding how to execute another Python script outside of the main thread is a crucial skill. This technique not only allows for better resource management but also opens up new possibilities for creating more complex and responsive applications.
As you delve into the intricacies of threading and process management in Python, you’ll discover various methods to initiate and control the execution of separate scripts. From leveraging the built-in `threading` module to utilizing the more robust `concurrent.futures` library, the options are abundant. Each approach offers unique benefits and challenges, making it essential to choose the right one based on your specific use case.
In this article, we’ll explore the fundamental concepts behind running Python scripts in a separate thread, including the importance of thread safety and synchronization. We will also discuss practical examples and best practices that will empower you to implement these techniques effectively in your projects. Whether you’re a novice looking to expand your knowledge or an experienced developer seeking to refine your skills, this exploration of threading in Python promises to be both enlightening and practical.
Using the `threading` Module
To run another Python script out of the main thread, you can utilize the `threading` module, which allows for parallel execution of code. This is particularly useful when you want to execute long-running tasks without blocking the main program’s execution.
Here is a basic example of how to implement this:
“`python
import threading
import subprocess
def run_script(script_name):
subprocess.run([‘python’, script_name])
Create a thread to run the script
thread = threading.Thread(target=run_script, args=(‘other_script.py’,))
thread.start()
Continue with other tasks in the main thread
print(“Main thread is doing other work.”)
“`
In this example, the `run_script` function uses `subprocess.run` to execute another Python script. The thread created will run this function, allowing the main thread to continue executing other tasks concurrently.
Using the `multiprocessing` Module
For CPU-bound tasks, the `multiprocessing` module is often a better choice than `threading`, as it avoids the Global Interpreter Lock (GIL) that can limit performance in multi-threaded applications. This module allows you to spawn separate processes, each with its own Python interpreter.
Here’s how you can use `multiprocessing` to run another script:
“`python
from multiprocessing import Process
import subprocess
def run_script(script_name):
subprocess.run([‘python’, script_name])
if __name__ == “__main__”:
process = Process(target=run_script, args=(‘other_script.py’,))
process.start()
Continue with the main program
print(“Main process is executing other code.”)
“`
This code snippet defines a `run_script` function and then creates a new process to execute it. The `if __name__ == “__main__”:` guard is essential to prevent unintended behavior when using multiprocessing on Windows.
Key Considerations
When running another Python script out of the main thread, consider the following:
- Thread Safety: Ensure that shared resources are accessed in a thread-safe manner to avoid data corruption.
- Error Handling: Implement error handling in the scripts being run to catch issues without crashing the main program.
- Resource Management: Monitor system resources, as spawning multiple processes can lead to increased memory and CPU usage.
Module | Use Case | Overhead |
---|---|---|
threading | I/O-bound tasks | Low |
multiprocessing | CPU-bound tasks | Higher |
By choosing the appropriate module for your task, you can effectively run another Python script in a separate thread or process, enabling better resource utilization and responsiveness in your applications.
Running a Python Script in a Separate Thread
To execute another Python script from a main script without blocking the main thread, the `threading` module can be utilized. This allows the main program to continue running while the other script executes independently.
“`python
import threading
import subprocess
def run_script(script_name):
subprocess.call([‘python’, script_name])
thread = threading.Thread(target=run_script, args=(‘other_script.py’,))
thread.start()
“`
In this example, the `run_script` function calls the specified Python script using `subprocess.call`. The `threading.Thread` object is created with the target function and the script name as an argument, and then started with `thread.start()`. This approach is efficient for I/O-bound tasks.
Using the `multiprocessing` Module
For CPU-bound tasks, it is often better to use the `multiprocessing` module. This module allows scripts to run in separate processes, which can take advantage of multiple cores.
“`python
from multiprocessing import Process
import subprocess
def run_script(script_name):
subprocess.call([‘python’, script_name])
process = Process(target=run_script, args=(‘other_script.py’,))
process.start()
“`
This method creates a new process for the script, ensuring that it runs independently of the main program. The `Process` class is instantiated similarly to `Thread`, and it is started with `process.start()`.
Handling Output and Errors
When running scripts in a separate thread or process, capturing output and errors is crucial for debugging and logging purposes. You can modify the subprocess call to redirect output and error streams.
“`python
import subprocess
import threading
def run_script(script_name):
with open(‘output.log’, ‘w’) as outfile, open(‘error.log’, ‘w’) as errfile:
subprocess.call([‘python’, script_name], stdout=outfile, stderr=errfile)
thread = threading.Thread(target=run_script, args=(‘other_script.py’,))
thread.start()
“`
In this example, both standard output and error output are directed to their respective log files, `output.log` and `error.log`.
Thread Safety Considerations
When using threads, it’s important to consider thread safety, especially when accessing shared resources. Here are some guidelines to ensure safe execution:
- Use Locks: Implement thread locks to prevent simultaneous access to shared resources.
- Avoid Global Variables: Minimize the use of global variables to reduce the risk of race conditions.
- Use Thread-safe Data Structures: Prefer data structures designed for concurrent access, like `queue.Queue`.
Best Practices
Implementing a Python script in another thread or process can be streamlined by following these best practices:
- Use Virtual Environments: Ensure scripts run in isolated environments to avoid dependency issues.
- Error Handling: Implement try-except blocks to handle exceptions gracefully.
- Clean Up Resources: Always clean up threads or processes after execution to free up system resources.
Best Practice | Description |
---|---|
Virtual Environments | Isolate dependencies to prevent conflicts. |
Error Handling | Use try-except to manage exceptions. |
Resource Cleanup | Ensure threads/processes terminate correctly. |
By adhering to these practices, running scripts concurrently becomes more manageable, leading to more robust applications.
Expert Insights on Running Python Scripts in a Separate Thread
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Running a Python script out of the main thread is an effective way to enhance application responsiveness. Utilizing the `threading` module allows developers to execute scripts concurrently, which is particularly beneficial in GUI applications where user interaction should remain fluid.”
Mark Thompson (Lead Python Developer, CodeMasters). “When executing another Python script from a separate thread, it is crucial to manage resources carefully. Implementing thread-safe mechanisms, such as locks or queues, can prevent data corruption and ensure that shared resources are accessed safely.”
Linda Zhang (Python Programming Instructor, Advanced Coding Academy). “Using the `subprocess` module in conjunction with threading can provide a robust solution for running separate Python scripts. This approach allows for better control over input and output streams, enabling effective communication between the main thread and the script being executed.”
Frequently Asked Questions (FAQs)
How can I run another Python script from a main script?
You can use the `subprocess` module to run another Python script from your main script. For example, use `subprocess.run([‘python’, ‘script_name.py’])` to execute the script.
What is the difference between using `subprocess` and `os.system`?
`subprocess` provides more powerful facilities for spawning new processes and retrieving their results, while `os.system` is a simpler interface that only runs a command in a subshell and returns the exit status.
Can I run a Python script in a separate thread?
Yes, you can use the `threading` module to run a Python script in a separate thread. However, be cautious with thread safety and the Global Interpreter Lock (GIL) when using threads.
How do I handle exceptions when running another script?
You can handle exceptions by wrapping the `subprocess.run` call in a try-except block. This allows you to catch and manage any errors that occur during the script execution.
Is it possible to pass arguments to the script being run?
Yes, you can pass arguments by adding them to the list in `subprocess.run`. For example, `subprocess.run([‘python’, ‘script_name.py’, ‘arg1’, ‘arg2’])` will pass `arg1` and `arg2` to the script.
What are the implications of running a script out of the main thread?
Running a script out of the main thread can improve responsiveness in GUI applications but may introduce complexity in managing shared resources and synchronization between threads.
Running another Python script out of the main thread is a common requirement in various applications, particularly when dealing with tasks that may block the main execution flow. Utilizing threading or multiprocessing modules allows developers to execute scripts concurrently, enhancing performance and responsiveness. It is crucial to understand the differences between these two approaches, as threading is more suited for I/O-bound tasks, while multiprocessing is ideal for CPU-bound operations.
When implementing this functionality, developers should consider the potential challenges, such as managing shared resources and ensuring thread safety. Proper synchronization mechanisms, such as locks or queues, can help mitigate issues that arise from concurrent access to shared data. Additionally, understanding the Global Interpreter Lock (GIL) in CPython is essential, as it can affect the performance of multi-threaded applications.
running another Python script out of the main thread can significantly improve application efficiency. By leveraging the appropriate concurrency model and implementing best practices for resource management, developers can create robust and responsive applications. This approach not only enhances user experience but also optimizes resource utilization, making it a valuable technique in modern Python programming.
Author Profile
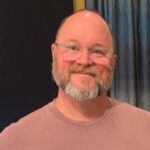
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?