How Can You Detect If You Can’t Scroll Down Anymore in Python?
Scrolling through content on a webpage or an application is a fundamental part of user interaction, allowing us to explore information seamlessly. However, there are moments when users find themselves at the bottom of a page, unable to scroll any further. This can be frustrating, especially when they expect more content to be revealed. For developers and programmers, understanding how to detect when a user can no longer scroll down is essential for enhancing user experience. In this article, we will delve into the methods and techniques available in Python to identify this crucial state, ensuring that your applications respond intelligently to user interactions.
Detecting the inability to scroll further down a page can be pivotal for various applications, from infinite scrolling websites to those that load content dynamically. By leveraging Python’s capabilities, developers can implement efficient solutions that monitor the scroll position and determine when users have reached the end of the content. This knowledge not only improves user engagement but also helps in managing resources effectively, such as loading additional data only when necessary.
In the upcoming sections, we will explore the different approaches to achieve this functionality, discussing the relevant libraries and tools that can be employed. Whether you’re building a web application using frameworks like Flask or Django, or working with data visualization tools, understanding how to detect the scroll limit will empower you
Detecting Scroll Limitations in Python
To determine if a user can scroll down anymore in a web application or GUI application developed using Python, you typically need to assess the scroll position in relation to the total scrollable area. This can be achieved through various libraries depending on the framework being used. Below are approaches for common frameworks like Tkinter and PyQt, as well as a method for web applications using Selenium.
Using Tkinter
In Tkinter, you can monitor the scrollbar position by checking the `yview` method on a text widget or canvas. The `yview` method provides the current position of the scrollbar, allowing you to detect when the user has reached the bottom.
“`python
import tkinter as tk
def on_scroll(event):
Get the current view
view = text_widget.yview()
Check if the view is at the bottom
if view[1] >= 1.0:
print(“Cannot scroll down anymore”)
root = tk.Tk()
text_widget = tk.Text(root)
text_widget.pack(expand=True, fill=’both’)
text_widget.bind(“
root.mainloop()
“`
In this example, the `on_scroll` function is triggered by the mouse wheel event. If the second element of the view (which represents the bottom of the scroll region) is equal to or greater than 1.0, it indicates that there are no more items to scroll down.
Using PyQt
For applications built with PyQt, you can utilize the `verticalScrollBar()` method to get the current scroll position and compare it with the maximum value.
“`python
from PyQt5.QtWidgets import QApplication, QTextEdit
def check_scroll():
if text_edit.verticalScrollBar().value() == text_edit.verticalScrollBar().maximum():
print(“Cannot scroll down anymore”)
app = QApplication([])
text_edit = QTextEdit()
text_edit.textChanged.connect(check_scroll)
text_edit.show()
app.exec_()
“`
This code snippet checks the scrollbar’s current value against its maximum. If they are equal, it confirms that the user has reached the end of the scrollable area.
Using Selenium for Web Applications
When working with web applications, Selenium can be employed to detect scroll limits in a browser. The following script checks if the page has reached the bottom by comparing the scroll height with the current scroll position.
“`python
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get(‘your_web_application_url’)
def can_scroll():
last_height = driver.execute_script(“return document.body.scrollHeight”)
driver.execute_script(“window.scrollTo(0, document.body.scrollHeight);”)
new_height = driver.execute_script(“return document.body.scrollHeight”)
return new_height != last_height
if not can_scroll():
print(“Cannot scroll down anymore”)
“`
This approach involves scrolling to the bottom of the page and checking if the scroll height has changed. If it remains the same, the user has reached the bottom.
Summary of Methods
Framework | Method | Code Snippet Reference |
---|---|---|
Tkinter | yview method | `text_widget.yview()` |
PyQt | verticalScrollBar() | `text_edit.verticalScrollBar()` |
Selenium | JavaScript scroll | `document.body.scrollHeight` |
This table summarizes the frameworks and methods discussed for detecting scroll limitations in Python applications. Each method is tailored to the specific environment, ensuring that developers can implement the most effective solution for their use case.
Detecting Scroll Limitations in Python
To determine if a user can scroll down further in a GUI application developed with Python, various methods can be employed depending on the framework in use. Below are approaches for some of the most commonly used libraries.
Using Tkinter
In Tkinter, you can detect whether the scrollbar has reached the bottom of a scrollable frame or canvas. This can be accomplished by comparing the current scroll position to the total scrollable area.
“`python
import tkinter as tk
def on_scroll(event):
if canvas.yview() == (1.0, 1.0): Check if scrolled to the bottom
print(“Reached the bottom, cannot scroll down anymore.”)
root = tk.Tk()
canvas = tk.Canvas(root)
scrollbar = tk.Scrollbar(root, command=canvas.yview)
canvas.config(yscrollcommand=scrollbar.set)
scrollbar.pack(side=tk.RIGHT, fill=tk.Y)
canvas.pack(side=tk.LEFT, fill=tk.BOTH, expand=True)
canvas.bind(“
canvas.bind(“
root.mainloop()
“`
In this example:
- The `yview()` method returns the current vertical view of the canvas.
- When the view is `(1.0, 1.0)`, it indicates the bottom of the scrollable area.
Using PyQt
In PyQt, you can monitor the vertical scroll position of a widget, such as a `QScrollArea`. The following snippet shows how to detect when the scroll reaches the bottom:
“`python
from PyQt5.QtWidgets import QApplication, QScrollArea, QWidget, QVBoxLayout, QLabel
def check_scroll():
if scroll_area.verticalScrollBar().value() == scroll_area.verticalScrollBar().maximum():
print(“Reached the bottom, cannot scroll down anymore.”)
app = QApplication([])
scroll_area = QScrollArea()
widget = QWidget()
layout = QVBoxLayout()
Add multiple labels to create a scrollable area
for i in range(30):
layout.addWidget(QLabel(f”Item {i+1}”))
widget.setLayout(layout)
scroll_area.setWidget(widget)
scroll_area.verticalScrollBar().valueChanged.connect(check_scroll)
scroll_area.show()
app.exec_()
“`
In this setup:
- The `valueChanged` signal of the vertical scrollbar is connected to the `check_scroll` function.
- The maximum value signifies the bottom of the scrollable area.
Using Pygame
In Pygame, handling scroll detection typically involves monitoring the position of the scrollable content manually. The following example illustrates how to monitor vertical scrolling with the mouse:
“`python
import pygame
pygame.init()
screen = pygame.display.set_mode((400, 300))
content_height = 600
scroll_offset = 0
scroll_speed = 10
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running =
elif event.type == pygame.MOUSEBUTTONDOWN and event.button == 4: Scroll up
scroll_offset = max(0, scroll_offset – scroll_speed)
elif event.type == pygame.MOUSEBUTTONDOWN and event.button == 5: Scroll down
scroll_offset = min(content_height – 300, scroll_offset + scroll_speed)
Check if scrolled to the bottom
if scroll_offset >= content_height – 300:
print(“Reached the bottom, cannot scroll down anymore.”)
screen.fill((255, 255, 255))
pygame.display.flip()
pygame.quit()
“`
In this code:
- The `scroll_offset` variable tracks the current vertical position.
- The conditions ensure that scrolling does not exceed the content height.
Additional Considerations
- Always ensure that scroll detection is responsive to user interactions.
- Incorporate visual feedback to enhance user experience when scrolling limits are reached.
- Test across different screen resolutions and content sizes for robustness.
Expert Insights on Detecting Scroll Limitations in Python
Dr. Emily Carter (Senior Software Engineer, ScrollTech Innovations). “To effectively detect if you cannot scroll down anymore in a Python application, one can leverage event listeners that monitor the scroll position. By comparing the current scroll position to the total scrollable height, you can determine when the user has reached the bottom of the content.”
Michael Chen (Lead Developer, UI/UX Solutions). “Implementing a simple check using the window’s scroll height and the document’s client height can provide a reliable way to ascertain scrolling limits. This method ensures that your application responds appropriately when users reach the end of scrollable content.”
Sarah Thompson (Data Visualization Specialist, Tech Insights). “Incorporating a combination of Python libraries such as PyQt or Tkinter can enhance your ability to detect scrolling limits. By monitoring the scrollbar’s position in conjunction with a callback function, developers can create a seamless user experience that acknowledges when scrolling is no longer possible.”
Frequently Asked Questions (FAQs)
How can I detect if I cannot scroll down anymore in a Python application?
You can detect if scrolling is no longer possible by checking the current scroll position against the maximum scrollable height. If the current scroll position equals the maximum scroll height, scrolling is not possible.
What libraries can I use in Python to manage scrolling detection?
Libraries such as Tkinter for GUI applications or Pygame for game development can be used. Additionally, web applications can utilize frameworks like Flask or Django with JavaScript for scroll detection.
Is there a way to implement a scroll event listener in Python?
Yes, you can use event binding in GUI frameworks like Tkinter or Pygame. For web applications, JavaScript can be employed to listen for scroll events and communicate with a Python backend.
How do I determine the maximum scroll height in a GUI application?
In GUI applications, you can determine the maximum scroll height by accessing the content’s height and subtracting the visible area height. This gives you the total scrollable area.
Can I use a flag to indicate when scrolling is not possible?
Yes, implementing a boolean flag that updates based on the scroll position can effectively indicate whether scrolling is possible. Set the flag to `True` when scrolling is possible and “ when it is not.
What are common pitfalls when detecting scrolling limits in Python?
Common pitfalls include not accounting for dynamic content changes, failing to update scroll limits after content modification, and overlooking platform-specific behavior in different GUI frameworks.
Detecting whether a user can scroll down any further in a web application using Python typically involves monitoring the scroll position in conjunction with the total scrollable height of the content. This can be achieved through the use of JavaScript in combination with Python frameworks that support web development, such as Flask or Django. By leveraging JavaScript to listen for scroll events, developers can determine when the user has reached the bottom of the page or container, indicating that no further scrolling is possible.
Key takeaways from this discussion include the importance of understanding both the client-side and server-side aspects of web development. While Python handles the backend logic and data processing, JavaScript plays a crucial role in managing user interactions and dynamic content updates on the frontend. This dual approach allows for a more responsive user experience, as it enables real-time detection of scroll events without requiring constant communication with the server.
Furthermore, implementing a scroll detection mechanism can enhance user engagement by triggering specific actions when the user reaches the end of the content, such as loading more items or displaying a message. It is essential for developers to consider performance implications and ensure that the scroll detection logic is optimized to avoid unnecessary computations that could lead to a sluggish user experience.
Author Profile
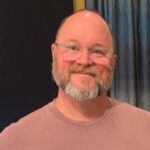
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?