Why Does My Python For Loop Only Execute the First Time?
In the world of programming, loops are essential tools that allow developers to execute a block of code multiple times. Among these, the `for` loop in Python is particularly popular for its simplicity and versatility. However, there are instances where you may want a `for` loop to execute only during its first iteration, skipping any subsequent cycles. This seemingly simple requirement can lead to intriguing discussions about control flow, conditional statements, and the nuances of Python’s syntax. Whether you’re a novice coder or an experienced programmer looking to refine your skills, understanding how to manipulate loops effectively can greatly enhance your coding efficiency.
When working with loops, especially in Python, the ability to control execution flow is paramount. The `for` loop typically iterates over a sequence, performing the same action for each item. However, there are scenarios where you might want to conditionally execute code only during the first pass of the loop. This can be particularly useful in situations such as initializing variables, setting up configurations, or performing tasks that should only occur once while still iterating through a collection.
To achieve this behavior, Python offers various techniques that leverage conditional statements within the loop structure. By employing flags, counters, or even leveraging the power of Python’s built-in functions, you can create a
Understanding Python For Loops
In Python, a for loop is designed to iterate over a sequence, such as a list, tuple, string, or any other iterable. However, there are scenarios where you may want the loop to execute its block of code only the first time it runs, effectively skipping subsequent iterations. This can be particularly useful in cases where an initialization or setup task needs to be performed only once before processing the remaining items.
Using a Flag Variable
One common technique to ensure that a block of code within a for loop executes only on the first iteration is to utilize a flag variable. This variable can be set to `True` initially and then changed to “ after the first execution. Here’s a simple example:
“`python
first_time = True
for item in iterable:
if first_time:
Code to execute only on the first iteration
print(“This runs only once.”)
first_time =
Code to execute on every iteration
print(item)
“`
This method is straightforward and works well when there are only a few lines of code to execute for the first iteration.
Using a Break Statement
Another approach is to utilize a break statement immediately after the first execution of the desired code block. This technique is less common but can be effective in certain scenarios. Here’s how you can implement it:
“`python
for item in iterable:
Code to execute only on the first iteration
print(“This runs only once.”)
break Exits the loop after the first iteration
“`
Keep in mind that using a break statement will terminate the loop entirely after the first execution, so subsequent items will not be processed.
Table of Options
The following table summarizes the different methods to execute code only during the first iteration of a for loop:
Method | Description | Example Code |
---|---|---|
Flag Variable | Uses a boolean flag to track the first iteration. |
first_time = True |
Break Statement | Exits the loop after the first execution of the code block. |
for item in iterable: |
Using Enumerate for Index Tracking
If you need to know the index of the current iteration, you can leverage the `enumerate()` function, which provides both the index and the value during iteration. This can also serve to control the execution of code on the first iteration:
“`python
for index, item in enumerate(iterable):
if index == 0:
Code to execute only on the first iteration
print(“This runs only once.”)
Code to execute on every iteration
print(item)
“`
This method is particularly useful when dealing with more complex data structures or when the index is relevant to the processing logic.
Understanding Python’s For Loop Behavior
In Python, the `for` loop is designed to iterate over a sequence, such as a list, tuple, or string. However, if there is a need to execute the loop’s body only during the first iteration, specific techniques can be employed.
Using a Flag Variable
One straightforward method to control execution is by utilizing a flag variable that tracks whether the loop is on its first iteration.
“`python
items = [1, 2, 3, 4]
first_iteration = True
for item in items:
if first_iteration:
print(“First iteration:”, item)
first_iteration =
“`
In the above example, the print statement executes only during the first iteration.
Using `enumerate()` for Index Tracking
Another effective approach involves using the `enumerate()` function to track the index of the current iteration. This method allows you to check if the index is zero, indicating the first iteration.
“`python
items = [1, 2, 3, 4]
for index, item in enumerate(items):
if index == 0:
print(“First iteration:”, item)
“`
This method is clean and leverages Python’s built-in capabilities for better readability.
Conditional Execution with `continue`
You can also use the `continue` statement to skip the body of the loop during subsequent iterations after the first one.
“`python
items = [1, 2, 3, 4]
first_iteration_done =
for item in items:
if first_iteration_done:
continue
print(“First iteration:”, item)
first_iteration_done = True
“`
In this structure, once the first iteration is complete, the `continue` statement prevents any further execution of the loop body.
Implementation of Custom Function
For more complex scenarios, encapsulating the logic within a custom function can enhance maintainability and reusability.
“`python
def process_first_only(iterable):
first = True
for item in iterable:
if first:
print(“First iteration:”, item)
first =
items = [1, 2, 3, 4]
process_first_only(items)
“`
This function can be reused across different parts of a program, maintaining clarity and encapsulating the logic effectively.
Conclusion on Loop Control Techniques
Employing one of these methods allows for targeted execution during the first iteration of a loop in Python. Each approach has its merits, with flag variables and index tracking being the most common.
Understanding the Use of Python For Loops for Single Iteration
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, if you want a for loop to execute only the first iteration, you can utilize the ‘break’ statement immediately after the first execution. This allows for a clean exit from the loop without needing to restructure your code significantly.”
Mark Thompson (Lead Software Engineer, CodeCraft Solutions). “Using a for loop to perform an action only once can also be achieved by leveraging a conditional statement within the loop. By checking a flag variable, you can ensure that the loop’s body executes solely during the first iteration, providing both clarity and control.”
Lisa Chen (Python Programming Instructor, Future Coders Academy). “When teaching Python, I emphasize that while for loops are typically designed for multiple iterations, understanding how to manipulate loop behavior with conditions or breaks is crucial for efficient coding practices, especially for scenarios requiring single execution.”
Frequently Asked Questions (FAQs)
How can I execute a block of code only during the first iteration of a Python for loop?
You can use a boolean flag to track whether the code has been executed. Set the flag to `True` after the first execution to prevent further execution in subsequent iterations.
Is there a way to use the `enumerate` function to run code only on the first iteration?
Yes, you can use `enumerate` to check if the index is `0`. If it is, execute the desired block of code. This approach is clean and avoids the need for additional flags.
Can I use a `break` statement to exit the loop after the first iteration?
While you can use a `break` statement to exit the loop immediately after the first iteration, this method will terminate the loop entirely, which may not be the desired outcome if you need to process more items.
What is the best practice for executing code only on the first iteration in a for loop?
The best practice is to use an `if` statement to check for the first iteration, either by using a counter or by checking the index with `enumerate`. This keeps the code readable and efficient.
Are there any built-in functions in Python that can help with this task?
Python does not have a built-in function specifically for executing code only on the first iteration of a loop. However, using control structures like `if` or `enumerate` provides a straightforward solution.
Can I achieve this behavior using list comprehensions or generator expressions?
List comprehensions and generator expressions are not suitable for executing code conditionally based on iteration count, as they are designed for generating lists or iterators. Use a standard for loop for this purpose.
In Python, the for loop is a fundamental construct used for iterating over sequences such as lists, tuples, and strings. However, there are scenarios where one might want the loop to execute only during its first iteration, effectively skipping subsequent iterations. This can be achieved through various methods, including the use of flags, conditional statements, or the `break` statement. Understanding how to control loop execution is essential for writing efficient and effective code.
One effective technique to execute code only during the first iteration of a for loop is to utilize a boolean flag. By initializing a flag variable before the loop and checking its value within the loop, one can ensure that specific code runs only once. Alternatively, using the `break` statement allows for immediate termination of the loop after the first iteration, which can be particularly useful in scenarios where the loop’s purpose is to perform a single action based on an iterable.
Key takeaways from this discussion include the importance of understanding loop control mechanisms in Python. Mastery of these techniques not only enhances code readability but also improves performance by preventing unnecessary iterations. Additionally, recognizing the various methods to execute code conditionally within a loop empowers developers to write more precise and optimized scripts, ultimately leading to better programming practices.
Author Profile
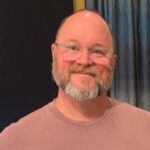
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?