What is the Python Equivalent of PHP’s Isset Function?
When transitioning from PHP to Python, developers often encounter a variety of differences in syntax and functionality that can pose challenges. One such feature that PHP developers rely on is the `isset()` function, which checks if a variable is set and is not null. Understanding how to achieve similar functionality in Python is crucial for ensuring smooth code execution and maintaining the integrity of your applications. In this article, we will explore the Python equivalent of PHP’s `isset()`, providing you with the insights necessary to navigate this common programming hurdle.
In Python, the approach to checking the existence of variables and their values differs from PHP’s straightforward function. While PHP’s `isset()` provides a simple one-liner to verify variable status, Python employs a more nuanced approach that leverages its dynamic typing and exception handling. This means that developers need to adapt to Python’s idioms and practices, which can enhance code readability and maintainability.
As we delve deeper into this topic, we will examine the various methods available in Python for checking variable existence and value, including the use of `try` and `except` blocks, the `in` keyword, and the built-in `get()` method for dictionaries. By the end of this article, you’ll not only grasp how to replicate the functionality of `isset()` in
Understanding PHP’s `isset` Function
The `isset` function in PHP is used to determine if a variable is set and is not `NULL`. This function can be particularly useful in checking if a variable has been initialized before attempting to use it. The syntax for `isset` is straightforward:
“`php
isset($variable);
“`
If the variable exists and is not `NULL`, `isset` returns `true`; otherwise, it returns “.
Python’s Equivalent to `isset`
In Python, there is no direct equivalent of the `isset` function, but similar functionality can be achieved using various methods. The most common approach is to utilize the `in` operator with dictionaries or `try-except` blocks for general variable checking.
To check if a variable exists in a dictionary (which is analogous to checking for a variable in PHP), you can use:
“`python
if ‘key’ in my_dict:
Do something
“`
For checking if a variable has been defined, you can employ a `try-except` block:
“`python
try:
variable
except NameError:
Variable is not defined
“`
Comparison of Methods
The following table summarizes the methods to check for variable existence in Python compared to PHP’s `isset`.
Language | Method | Example | Returns |
---|---|---|---|
PHP | isset | isset($variable) | true/ |
Python | Check in dictionary | ‘key’ in my_dict | true/ |
Python | Try-Except |
try: variable except NameError: pass |
Variable is defined or not |
Best Practices
When translating the logic of `isset` to Python, consider the following best practices:
- Use Dictionaries: For structured data, prefer using dictionaries where you can easily check for key existence.
- Avoid Unnecessary Checks: In Python, it’s common to handle missing variables through exception handling instead of pre-checking.
- Initialization: Always initialize your variables to avoid `NameError` exceptions.
By adopting these methods, Python developers can effectively manage variable existence checks akin to PHP’s `isset` functionality.
Understanding PHP’s `isset` Function
In PHP, the `isset` function is used to determine if a variable is set and is not `NULL`. This function returns `true` if the variable exists and has a value other than `NULL`; otherwise, it returns “.
Example Usage in PHP:
“`php
$var = “Hello, World!”;
if (isset($var)) {
echo “Variable is set.”;
} else {
echo “Variable is not set.”;
}
“`
This functionality is essential for avoiding errors when trying to access variables that may not have been initialized.
Python Equivalent of PHP’s `isset`
In Python, there isn’t a direct equivalent to PHP’s `isset`. However, similar functionality can be achieved using several methods, primarily involving the `in` keyword and the `get()` method of dictionaries.
Checking for Variable Existence:
- Using `locals()` or `globals()`: You can check if a variable exists in the local or global scope.
“`python
if ‘var_name’ in locals():
print(“Variable is set.”)
else:
print(“Variable is not set.”)
“`
- Using `try` and `except`: This approach allows you to check if a variable can be accessed without triggering a `NameError`.
“`python
try:
var_name
except NameError:
print(“Variable is not set.”)
else:
print(“Variable is set.”)
“`
Checking Dictionary Keys
When working with dictionaries, the method `dict.get()` can be effectively used to check for the existence of a key.
Example:
“`python
my_dict = {‘key1’: ‘value1’, ‘key2’: None}
Using ‘in’ operator
if ‘key1’ in my_dict:
print(“Key exists.”)
Using get() method
if my_dict.get(‘key2’) is not None:
print(“Key is set and has a value.”)
else:
print(“Key is either not set or has a value of None.”)
“`
Comparison of Methods
Method | Description | Output Example |
---|---|---|
`locals()`/`globals()` | Check if variable exists in current/global scope | “Variable is set.” |
`try`/`except` | Handle potential `NameError` when accessing | “Variable is not set.” |
`in` operator (dict) | Check if a key exists in a dictionary | “Key exists.” |
`dict.get()` | Retrieve value or `None` if key does not exist | “Key is either not set or has a value of None.” |
By using these methods, Python developers can effectively manage variable existence checks similar to how it is done in PHP with `isset`.
Understanding Python’s Approach to Variable Checking Compared to PHP’s Isset
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). In Python, the equivalent of PHP’s `isset` is typically achieved using the `in` operator or the `get()` method for dictionaries. This allows developers to check for the existence of a variable or key without raising an error if it does not exist, thereby promoting cleaner and more readable code.
Michael Thompson (Lead Python Developer, CodeMasters). While PHP’s `isset` checks whether a variable is set and is not null, in Python, one can use the `is not None` condition to achieve similar functionality. This distinction is crucial as it emphasizes Python’s emphasis on explicitness and clarity in variable handling.
Sarah Lee (Full-Stack Developer, Web Solutions Group). In transitioning from PHP to Python, developers should note that Python does not have a direct equivalent to `isset`. Instead, using exception handling with `try-except` blocks or leveraging the `locals()` function can provide similar checks, allowing for more robust error management in applications.
Frequently Asked Questions (FAQs)
What is the Python equivalent of PHP’s isset function?
The Python equivalent of PHP’s `isset` function is the `in` keyword or the `get()` method for dictionaries. These methods check for the existence of a variable or key without raising an error if it is not defined.
How do you check if a variable is defined in Python?
In Python, you can use a try-except block to check if a variable is defined. Alternatively, you can use the `locals()` or `globals()` functions to see if a variable exists in the current or global scope.
Can you check if a dictionary key exists in Python?
Yes, you can check if a dictionary key exists using the `in` keyword. For example, `if key in my_dict:` will return `True` if the key exists in the dictionary.
What happens if you try to access an variable in Python?
If you attempt to access an variable in Python, it raises a `NameError`, indicating that the variable is not defined in the current scope.
Is there a built-in function in Python to check variable existence like isset?
Python does not have a direct equivalent to `isset`, but you can use the `get()` method for dictionaries or the `in` keyword to check variable existence in a more Pythonic way.
How can you handle missing keys in a dictionary in Python?
You can handle missing keys in a dictionary by using the `get()` method, which allows you to specify a default value if the key is not found. For example, `my_dict.get(key, default_value)` will return `default_value` if `key` is not present.
the PHP function `isset()` is commonly used to determine if a variable is set and is not null. In Python, the equivalent functionality can be achieved using various approaches, primarily through the use of the `in` operator and the `get()` method for dictionaries. These methods allow developers to check for the existence of a variable or key, thus ensuring that their code handles variables gracefully.
One key takeaway is that while PHP’s `isset()` checks for both existence and nullity, Python’s approach requires a more explicit handling of these conditions. For instance, checking if a variable exists can be done using the `locals()` or `globals()` functions, while dictionary keys can be verified using the `in` keyword. This distinction highlights the differences in how both languages manage variable scope and existence.
Furthermore, understanding these equivalents is crucial for developers transitioning between PHP and Python. It emphasizes the importance of adapting to each language’s idioms and best practices. By leveraging Python’s capabilities effectively, developers can write more robust and error-free code that mirrors the functionality provided by PHP’s `isset()`.
Author Profile
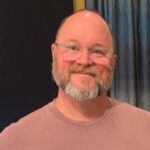
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?