How Can You Use PowerShell to Check If a File Exists?
In the fast-paced world of IT and system administration, efficiency and accuracy are paramount. One of the most common tasks that administrators face is ensuring that essential files are present and accounted for. Whether it’s configuration files, logs, or scripts, missing files can lead to significant disruptions and downtime. This is where PowerShell, a powerful scripting language and command-line shell, comes into play. With its robust capabilities, PowerShell provides a straightforward approach to checking for file existence, enabling users to automate and streamline their workflows.
Understanding how to check if a file exists using PowerShell is not just a matter of convenience; it’s a fundamental skill that can enhance your scripting prowess. By leveraging simple commands and scripts, you can quickly ascertain the presence of files, which is crucial for tasks such as backup verification, system monitoring, and troubleshooting. PowerShell’s versatility allows users to integrate these checks into larger scripts, ensuring that processes run smoothly without manual intervention.
As we delve deeper into this topic, we will explore various methods and best practices for utilizing PowerShell to check for file existence. From basic commands to more advanced scripting techniques, this guide will equip you with the knowledge needed to enhance your file management processes and safeguard your system’s integrity. Whether you are a seasoned IT professional or a newcomer to
Using Test-Path Cmdlet
The simplest method to check if a file exists in PowerShell is by utilizing the `Test-Path` cmdlet. This cmdlet returns a Boolean value indicating whether the specified path exists. The syntax is straightforward:
powershell
Test-Path “C:\path\to\your\file.txt”
If the file exists, this command will return `True`; if not, it will return “. This method can be particularly useful in scripts where conditional operations depend on the existence of files.
Example of Test-Path
Here’s an example demonstrating the use of `Test-Path` in a script:
powershell
$filePath = “C:\path\to\your\file.txt”
if (Test-Path $filePath) {
Write-Host “File exists.”
} else {
Write-Host “File does not exist.”
}
This script checks for the specified file and prints a message based on its existence.
Checking for Directory Existence
In addition to checking for files, `Test-Path` can also be used to verify if a directory exists. The same syntax applies:
powershell
Test-Path “C:\path\to\your\directory”
This will yield `True` if the directory exists and “ otherwise.
Using FileInfo Class
Another method to check if a file exists is by using the .NET `FileInfo` class. This approach provides more detailed information about the file, such as its attributes, size, and last access time.
Example code snippet:
powershell
$fileInfo = New-Object System.IO.FileInfo(“C:\path\to\your\file.txt”)
if ($fileInfo.Exists) {
Write-Host “File exists.”
} else {
Write-Host “File does not exist.”
}
This method is particularly useful when additional file properties need to be accessed after checking for existence.
Common Use Cases
When working with file existence checks in PowerShell, there are several common scenarios:
- Script automation: Ensuring required files are present before executing further commands.
- Logging: Checking for log files before attempting to read or append data.
- Backup verification: Confirming the existence of backup files before performing restore operations.
Comparison Table of Methods
Method | Returns | Usage |
---|---|---|
Test-Path | Boolean (True/) | Basic existence check |
FileInfo Class | FileInfo Object | Existence and detailed properties |
Each method has its advantages, and the choice may depend on the specific requirements of your PowerShell script.
Using PowerShell to Check if a File Exists
In PowerShell, verifying the existence of a file can be efficiently accomplished using several built-in methods. The most common approach is to utilize the `Test-Path` cmdlet, which returns a Boolean value indicating whether the specified path exists.
Basic Syntax of Test-Path
The syntax for using `Test-Path` is straightforward:
powershell
Test-Path -Path “C:\Path\To\File.txt”
This command checks if the file `File.txt` exists in the specified directory. The output will be `True` if the file exists and “ if it does not.
Examples of Checking File Existence
Here are several practical examples demonstrating how to use `Test-Path` in different scenarios:
- Check a specific file:
powershell
$filePath = “C:\Example\document.txt”
if (Test-Path -Path $filePath) {
Write-Host “File exists.”
} else {
Write-Host “File does not exist.”
}
- Check a file using a variable:
powershell
$filePath = “C:\Example\image.png”
$fileExists = Test-Path -Path $filePath
Write-Host “Does the file exist? $fileExists”
- Check for multiple files:
powershell
$files = @(“C:\Example\file1.txt”, “C:\Example\file2.txt”)
foreach ($file in $files) {
if (Test-Path -Path $file) {
Write-Host “$file exists.”
} else {
Write-Host “$file does not exist.”
}
}
Using Try-Catch for Error Handling
In some cases, you may want to include error handling while checking for file existence. Utilizing a `try-catch` block can help manage exceptions gracefully.
powershell
try {
$filePath = “C:\Example\file.txt”
if (Test-Path -Path $filePath) {
Write-Host “File exists.”
} else {
Write-Host “File does not exist.”
}
} catch {
Write-Host “An error occurred: $_”
}
Checking File Existence in Remote Paths
When dealing with files on remote systems, `Test-Path` can still be utilized, provided you have the necessary permissions and the path is accessible.
powershell
$remoteFilePath = “\\RemoteServer\SharedFolder\file.txt”
if (Test-Path -Path $remoteFilePath) {
Write-Host “Remote file exists.”
} else {
Write-Host “Remote file does not exist.”
}
Understanding the Output of Test-Path
The output of `Test-Path` can be utilized in various ways:
Output | Description |
---|---|
True | The specified file or directory exists. |
The specified file or directory does not exist. |
Using this information, scripts can be designed to take different actions based on whether a file exists or not.
PowerShell provides a robust method for checking file existence through the `Test-Path` cmdlet, allowing for efficient scripting and error handling in various scenarios.
Expert Insights on Using PowerShell to Check File Existence
Emily Carter (Senior Systems Administrator, Tech Solutions Inc.). “Utilizing PowerShell to check if a file exists is a fundamental skill for any systems administrator. The simplicity of the command ‘Test-Path’ allows for efficient scripting, enabling automation and error handling in various IT processes.”
James Thompson (Cloud Infrastructure Engineer, Cloud Innovators). “In cloud environments, where file management can become complex, leveraging PowerShell to verify file existence is crucial. It not only helps in maintaining data integrity but also aids in troubleshooting deployment issues effectively.”
Linda Garcia (Cybersecurity Analyst, SecureTech). “From a cybersecurity perspective, using PowerShell to check for file existence can be a proactive measure. It allows for monitoring critical files and ensuring that unauthorized changes do not occur, which is vital for maintaining system security.”
Frequently Asked Questions (FAQs)
How can I check if a file exists using PowerShell?
You can check if a file exists in PowerShell by using the `Test-Path` cmdlet. For example, `Test-Path “C:\path\to\your\file.txt”` will return `True` if the file exists and “ if it does not.
What is the syntax for using Test-Path in PowerShell?
The syntax is straightforward: `Test-Path -Path “C:\path\to\your\file.txt”`. You can replace the path with the location of the file you want to check.
Can I check for the existence of a directory using PowerShell?
Yes, `Test-Path` can also be used to check for directories. Simply provide the directory path, such as `Test-Path “C:\path\to\your\directory”`.
What is the return type of the Test-Path cmdlet?
The `Test-Path` cmdlet returns a Boolean value: `True` if the file or directory exists and “ if it does not.
Is it possible to check for multiple files at once in PowerShell?
Yes, you can check for multiple files by using a loop or an array. For example, you can use `foreach` to iterate through an array of file paths and apply `Test-Path` to each.
What happens if the file path contains spaces in PowerShell?
If the file path contains spaces, enclose the path in quotes. For example, use `Test-Path “C:\path with spaces\file.txt”` to ensure PowerShell correctly interprets the path.
In summary, utilizing PowerShell to check if a file exists is a straightforward yet powerful capability that enhances automation and scripting tasks. The primary command used for this purpose is the `Test-Path` cmdlet, which evaluates the existence of a specified file or directory. This command can be integrated into scripts to make conditional decisions based on the presence or absence of files, thereby streamlining workflows and improving efficiency.
Moreover, the versatility of PowerShell allows users to not only check for files but also to handle various scenarios such as validating file paths, managing errors, and executing alternative actions based on the results of the existence check. By incorporating logical structures like `if` statements, users can create robust scripts that respond dynamically to the file system’s state.
Key takeaways include the importance of understanding the syntax and parameters of the `Test-Path` cmdlet, as well as the potential for combining this command with other PowerShell functionalities to create comprehensive solutions. Additionally, leveraging PowerShell’s capabilities can significantly reduce manual tasks and enhance overall productivity in system administration and development environments.
Author Profile
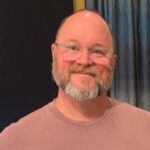
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?