How Can You Run Another PowerShell Script from Within a PowerShell Script?
In the world of automation and system administration, PowerShell stands out as a powerful tool for managing and configuring Windows environments. Its versatility allows users to execute a wide range of tasks, from simple file manipulations to complex system configurations. However, as scripts grow in complexity, the need to modularize and organize code becomes increasingly important. This is where the ability to run one PowerShell script from another comes into play. By leveraging this feature, administrators can streamline their workflows, enhance code reusability, and maintain cleaner, more manageable scripts.
When you run another PowerShell script from within a script, you unlock a myriad of possibilities for automation. This practice not only promotes better organization but also allows for the execution of shared functions and variables, making your scripts more efficient and easier to maintain. Whether you’re orchestrating a series of tasks or breaking down a large script into manageable components, understanding how to effectively call one script from another is essential for any PowerShell user.
As we delve deeper into this topic, we will explore the various methods available for executing PowerShell scripts, the benefits of script modularization, and best practices to ensure smooth execution. By the end of this article, you’ll be equipped with the knowledge to enhance your PowerShell scripting capabilities and take your
Executing a PowerShell Script from Another Script
To run a PowerShell script from another PowerShell script, you can use the `&` call operator, which allows you to execute a command, script, or executable. This method is straightforward and effective for chaining scripts together.
For example, if you have a script named `ScriptA.ps1` that you want to call from `ScriptB.ps1`, you can include the following line in `ScriptB.ps1`:
“`powershell
& “C:\Path\To\ScriptA.ps1”
“`
This will invoke `ScriptA.ps1` when `ScriptB.ps1` is executed. Ensure that the path to the script is enclosed in quotes if it contains spaces.
Passing Parameters Between Scripts
When you need to pass parameters from one script to another, you can define parameters in the called script and provide values when invoking it. Here’s how to set this up:
- Define parameters in the called script using the `param` block.
- Call the script with the necessary arguments.
For instance, in `ScriptA.ps1`, you may have:
“`powershell
param (
[string]$Name,
[int]$Age
)
Write-Output “Name: $Name, Age: $Age”
“`
In `ScriptB.ps1`, you would call `ScriptA.ps1` like this:
“`powershell
& “C:\Path\To\ScriptA.ps1” -Name “John Doe” -Age 30
“`
This approach allows for flexible and dynamic script execution based on input parameters.
Using Dot Sourcing to Run a Script
Dot sourcing is another method to run a PowerShell script, which allows the called script to share its variables and functions with the calling script. This can be useful when you want to retain the context after the script has been executed.
To dot source a script, use a dot followed by a space and the script path:
“`powershell
. “C:\Path\To\ScriptA.ps1”
“`
After dot sourcing, any variables or functions defined in `ScriptA.ps1` will be available in `ScriptB.ps1`.
Handling Script Execution Policies
Before executing scripts, it is essential to ensure that your PowerShell execution policy permits script execution. The execution policy can be set to various levels, such as:
- Restricted: No scripts can be run.
- AllSigned: Only scripts signed by a trusted publisher can be run.
- RemoteSigned: Scripts created locally can run, but downloaded scripts require a signature.
- Unrestricted: All scripts can be run.
To check the current execution policy, run:
“`powershell
Get-ExecutionPolicy
“`
To change the execution policy, use:
“`powershell
Set-ExecutionPolicy RemoteSigned
“`
Be aware that changing the execution policy may require administrative privileges.
Policy | Description |
---|---|
Restricted | No scripts can be executed. |
AllSigned | Only signed scripts can be run. |
RemoteSigned | Local scripts can run; remote scripts require a signature. |
Unrestricted | All scripts can be run. |
By understanding these concepts, you can effectively manage and execute PowerShell scripts from within other scripts, enhancing your automation capabilities.
Executing a PowerShell Script from Another Script
To run a PowerShell script from another PowerShell script, you can utilize the `&` call operator or the `Invoke-Expression` cmdlet. Each method has its specific use cases and benefits.
Using the Call Operator (`&`)
The call operator is the most straightforward method to execute another script. It allows you to run scripts directly without needing to encapsulate the command in quotes.
Example:
“`powershell
& “C:\Path\To\Your\Script.ps1”
“`
Key points:
- The path can be absolute or relative.
- If the script path contains spaces, it must be enclosed in quotes.
Using `Invoke-Expression` Cmdlet
The `Invoke-Expression` cmdlet evaluates a string as a command. This method can be useful when the script path is stored in a variable.
Example:
“`powershell
$scriptPath = “C:\Path\To\Your\Script.ps1”
Invoke-Expression $scriptPath
“`
Considerations:
- This method is less preferred due to potential security risks when executing arbitrary strings.
Passing Arguments to the Script
When invoking another PowerShell script, you may need to pass arguments. Both methods described above support argument passing.
Example using the Call Operator:
“`powershell
& “C:\Path\To\Your\Script.ps1” -Param1 Value1 -Param2 Value2
“`
Example using `Invoke-Expression`:
“`powershell
$scriptPath = “C:\Path\To\Your\Script.ps1 -Param1 Value1 -Param2 Value2”
Invoke-Expression $scriptPath
“`
Parameters:
- Each parameter should be prefixed with a hyphen.
- Values can be strings, integers, or other data types depending on the script’s design.
Handling Execution Policy
PowerShell scripts are subject to execution policies that may prevent scripts from running. Adjust the execution policy if necessary.
Common Execution Policies:
- Restricted: No scripts can be run.
- AllSigned: Only scripts signed by a trusted publisher can be run.
- RemoteSigned: Scripts created locally can run; scripts from the internet must be signed.
- Unrestricted: All scripts can run.
Change Execution Policy:
To change the execution policy, use the following command:
“`powershell
Set-ExecutionPolicy RemoteSigned
“`
Important:
- Execution policies can be set at different scopes (Process, CurrentUser, LocalMachine).
- Use caution when changing policies, especially on production systems.
Using `.ps1` Files vs. Functions
While scripts are typically stored as `.ps1` files, you can also define functions within your main script to encapsulate reusable code.
Example of a Function:
“`powershell
function Invoke-MyFunction {
param (
[string]$Param1,
[int]$Param2
)
Function logic here
}
Invoke-MyFunction -Param1 “Value” -Param2 123
“`
Benefits of Functions:
- Improved readability and organization.
- Easier to debug and test individual components of your code.
- Better encapsulation of logic, reducing redundancy.
Best Practices
- Always use full paths when calling scripts to avoid ambiguity.
- Validate input parameters to ensure they meet expected types and formats.
- Document your scripts to enhance maintainability.
- Consider using error handling techniques such as `try/catch` to manage exceptions when invoking scripts.
By following these guidelines, you can effectively manage and execute PowerShell scripts within other PowerShell scripts, ensuring both functionality and security.
Expert Insights on Running PowerShell Scripts
Dr. Emily Carter (Senior Systems Administrator, Tech Solutions Inc.). “When executing one PowerShell script from another, it is crucial to understand the execution policy settings of your environment. Using the `&` operator or `Start-Process` cmdlet can streamline this process, but ensure that scripts are signed if your policy requires it.”
James Liu (PowerShell Automation Specialist, Cloud Innovations). “Chaining scripts can significantly enhance automation workflows. Utilizing parameters effectively allows for dynamic input, making the scripts versatile and reusable across various tasks.”
Maria Gonzalez (IT Security Consultant, SecureTech Advisory). “Always be cautious when running scripts from other scripts. Implementing error handling and logging is essential to maintain security and ensure that any issues can be traced back effectively.”
Frequently Asked Questions (FAQs)
How can I run another PowerShell script from within a PowerShell script?
You can run another PowerShell script by using the `&` (call) operator followed by the path to the script. For example: `& “C:\Path\To\YourScript.ps1″`.
What is the difference between using `&` and `.` (dot sourcing) to run a script?
Using `&` runs the script in a new scope, meaning variables defined in the called script do not affect the calling script. Dot sourcing (`. C:\Path\To\YourScript.ps1`) runs the script in the current scope, allowing variable definitions and functions to persist in the calling script.
Can I pass parameters to a PowerShell script when calling it from another script?
Yes, you can pass parameters by including them after the script path. For example: `& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2`.
What happens if the called script encounters an error?
If the called script encounters an error, it will stop execution unless you handle the error using `try`/`catch` blocks or set `$ErrorActionPreference` to `Continue`. This allows the calling script to manage errors appropriately.
Is it possible to run a script asynchronously in PowerShell?
Yes, you can run a script asynchronously using `Start-Job`. For example: `Start-Job -ScriptBlock { & “C:\Path\To\YourScript.ps1” }`. This allows the calling script to continue executing while the called script runs in the background.
How can I ensure that the called script runs in a specific execution policy?
You can temporarily change the execution policy for the session by using `Set-ExecutionPolicy` before calling the script. For example: `Set-ExecutionPolicy -Scope Process -ExecutionPolicy Bypass; & “C:\Path\To\YourScript.ps1″`. This change will only apply to the current session.
In summary, running another PowerShell script from within a PowerShell session is a straightforward yet powerful feature that enhances automation and script modularity. This capability allows users to execute scripts dynamically, facilitating the reuse of code and the organization of complex tasks into manageable components. By utilizing the `&` call operator or the `Invoke-Expression` cmdlet, users can seamlessly integrate multiple scripts, leading to improved efficiency and reduced redundancy in scripting practices.
Moreover, understanding the context in which scripts are executed is crucial. PowerShell provides various scopes and execution policies that can affect how scripts run and interact with one another. It is essential for users to be aware of these aspects to ensure that their scripts operate as intended without encountering permission issues or scope conflicts. Additionally, leveraging parameters when calling scripts can enhance flexibility, allowing for the passing of arguments and customizing the execution based on specific needs.
Overall, the ability to run another PowerShell script not only streamlines workflows but also empowers users to create more sophisticated automation solutions. As PowerShell continues to evolve, mastering this functionality will be increasingly important for professionals looking to optimize their scripting capabilities and enhance their overall productivity in managing system administration tasks.
Author Profile
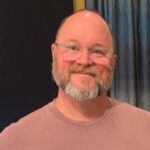
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?