How Can You Resolve PHP Timezone Mismatches with Server Timezone Settings?
### Introduction
In the world of web development, time is more than just a number; it’s a critical component that can influence everything from user experience to data integrity. When working with PHP applications, developers often encounter the perplexing issue of timezone mismatches between server settings and application expectations. This discrepancy can lead to a myriad of problems, including incorrect timestamps, scheduling errors, and even data loss. Understanding how to navigate these timezone challenges is essential for ensuring that your application runs smoothly and accurately reflects the intended time-related functionalities.
Timezone mismatches can arise from various factors, including server configurations, PHP settings, and even user preferences. When a server is set to a different timezone than the one specified in your PHP application, it can create confusion and lead to unexpected behavior in your code. For instance, if your application is designed to log events in a specific timezone but the server is operating on UTC, the logged times may appear skewed, misleading both developers and users alike.
Moreover, the implications of these mismatches extend beyond mere inconvenience. They can affect data consistency, impact scheduled tasks, and complicate debugging processes. As we delve deeper into this topic, we will explore the common causes of timezone mismatches, best practices for configuring PHP and server timezones, and practical
Understanding Timezone Configuration
When dealing with PHP applications, it’s essential to understand how timezone settings affect the behavior of date and time functions. PHP relies on the timezone configuration set in the php.ini file, which may differ from the server’s default timezone settings. This mismatch can lead to unexpected behavior in applications, especially those that rely heavily on accurate date and time calculations.
To check and set the timezone in PHP, you can use the following functions:
- `date_default_timezone_set(‘Timezone’)`: This function sets the default timezone for all date and time functions in the script.
- `date_default_timezone_get()`: This retrieves the current default timezone set in the script.
It’s advisable to set the timezone explicitly at the beginning of your PHP scripts to avoid any discrepancies.
Common Causes of Timezone Mismatch
Several factors can contribute to timezone mismatches:
- Server Configuration: The server’s timezone may be set to UTC or a different timezone than the one expected by the application.
- PHP Configuration: The default timezone in the PHP configuration file (php.ini) may not be set correctly.
- Database Timezone: If your application interacts with a database, the timezone settings of the database server may differ from the application, affecting timestamps and date calculations.
- User Input: Applications that allow users to set their timezones may lead to mismatches if not handled properly.
Checking and Setting Timezones
To ensure consistency across your application, it is crucial to verify and set timezones appropriately. Here’s a step-by-step approach:
- Check the server timezone:
bash
date
- Check the PHP timezone:
You can create a simple PHP script:
php
echo date_default_timezone_get();
- Setting the PHP timezone:
Add the following line to your script:
php
date_default_timezone_set(‘America/New_York’);
- Database timezone settings:
Ensure your database server (e.g., MySQL) is set to the same timezone:
sql
SET time_zone = ‘America/New_York’;
Timezone Configuration Table
The following table summarizes common timezones and their identifiers in PHP:
Timezone Name | PHP Identifier |
---|---|
Pacific Standard Time | America/Los_Angeles |
Central Standard Time | America/Chicago |
Eastern Standard Time | America/New_York |
Greenwich Mean Time | GMT |
Central European Time | Europe/Berlin |
By following these guidelines and ensuring that all components of your application are synchronized in terms of timezone, you can mitigate the risk of timezone-related issues effectively.
Understanding PHP Timezone Configuration
PHP uses the timezone setting to manage date and time functions accurately. The default timezone can significantly impact how timestamps are generated and displayed. To ensure consistency across applications and servers, it is crucial to configure the PHP timezone correctly.
- Setting the Timezone in PHP: You can set the timezone in your PHP scripts using the `date_default_timezone_set()` function. For example:
php
date_default_timezone_set(‘America/New_York’);
- Checking Current Timezone: To verify the current timezone setting, use:
php
echo date_default_timezone_get();
- Configuration in `php.ini`: The timezone can also be set in the `php.ini` file. Locate the following line and modify it accordingly:
date.timezone = “America/New_York”
- List of Supported Timezones: PHP provides a list of supported timezones. You can retrieve this list using:
php
$timezones = timezone_identifiers_list();
foreach ($timezones as $timezone) {
echo $timezone . “\n”;
}
Identifying Timezone Mismatches
Timezone mismatches may arise when the server’s timezone does not align with the application’s settings. This can lead to discrepancies in date and time calculations. Common scenarios include:
- Database Timezone: If your database server uses a different timezone, it may store timestamps in UTC, while your application operates in a local timezone.
- User Preferences: Users may operate in different timezones, necessitating a dynamic approach to timezone handling in your application.
Resolving Timezone Issues
Addressing timezone mismatches involves several steps:
– **Synchronize Server and Application Timezones**: Ensure the server timezone aligns with the application’s timezone settings.
Action | Description |
---|---|
Check Server Timezone | Use the command `date` in the server terminal. |
Update PHP Settings | Use `date_default_timezone_set()` in your PHP code. |
Adjust Database Settings | Configure the database timezone to match the application. |
– **Convert Dates and Times**: When displaying dates, convert them to the user’s timezone. Use PHP’s `DateTime` class for effective conversions:
php
$date = new DateTime(“now”, new DateTimeZone(“UTC”));
$date->setTimezone(new DateTimeZone(“America/New_York”));
echo $date->format(‘Y-m-d H:i:s’);
- Log Timezone Information: Implement logging to track the timezone being used at various points in your application. This can help identify where mismatches occur.
Best Practices for Timezone Management
To maintain consistency and avoid future issues, adopt the following best practices:
- Use UTC for Storage: Store all timestamps in UTC in your database to avoid confusion.
- Convert on Display: Always convert timestamps to the user’s local timezone when displaying data.
- Regularly Review Settings: Periodically check and update the timezone settings on both the server and application.
By following these guidelines, you can effectively manage PHP timezone configurations and avoid mismatches that may impact your application’s functionality.
Addressing Php Timezone Mismatch Server Timezone Issues
Dr. Emily Chen (Senior Software Engineer, TimeZone Solutions Inc.). “A common issue developers face with PHP is the mismatch between server timezones and application timezones. It is crucial to explicitly set the timezone in your PHP configuration using the `date_default_timezone_set()` function to ensure consistency across your application.”
Mark Thompson (Web Development Consultant, TechSavvy Group). “When dealing with timezone mismatches in PHP, it is essential to understand the server’s default timezone settings. Often, server configurations default to UTC, which can lead to discrepancies if your application is set to a different timezone. Regular audits of your server settings can mitigate these issues.”
Lisa Patel (Lead PHP Developer, CloudTech Innovations). “To avoid timezone mismatches, I recommend using UTC as the standard timezone for all server-side operations. This practice simplifies the conversion process for users in different timezones and reduces the likelihood of errors during data processing and storage.”
Frequently Asked Questions (FAQs)
What causes a PHP timezone mismatch with the server timezone?
A PHP timezone mismatch occurs when the timezone set in the PHP configuration does not align with the server’s default timezone. This can lead to discrepancies in date and time functions, affecting applications that rely on accurate timekeeping.
How can I check the current timezone setting in PHP?
You can check the current timezone setting in PHP by using the `date_default_timezone_get()` function. This function returns the timezone identifier currently set in the PHP environment.
How do I change the PHP timezone to match the server timezone?
To change the PHP timezone, you can use the `date_default_timezone_set()` function in your PHP script. Alternatively, you can modify the `php.ini` configuration file by setting the `date.timezone` directive to the desired timezone.
What are the common PHP timezone identifiers?
Common PHP timezone identifiers include ‘America/New_York’, ‘Europe/London’, ‘Asia/Tokyo’, and ‘UTC’. A complete list of identifiers can be found in the PHP manual under the Timezones section.
What issues can arise from a PHP timezone mismatch?
Issues that can arise from a PHP timezone mismatch include incorrect timestamps in databases, inaccurate scheduling of tasks, and confusion in user interfaces that display date and time information, leading to potential operational errors.
How can I synchronize PHP timezone settings with the server’s timezone?
To synchronize PHP timezone settings with the server’s timezone, ensure that both the PHP configuration and the server’s system timezone are set to the same timezone. This can be verified and adjusted through the server’s operating system settings and PHP configuration files.
The issue of PHP timezone mismatch with server timezone is a common challenge faced by developers. It arises when the default timezone setting in PHP does not align with the server’s timezone configuration. This misalignment can lead to discrepancies in date and time calculations, affecting the functionality of applications that rely on accurate time data. Understanding how to configure and manage timezones in PHP is crucial for ensuring that applications operate correctly across different environments.
To address timezone mismatches, developers can utilize PHP’s built-in functions such as `date_default_timezone_set()` to explicitly set the desired timezone for their applications. Additionally, it is essential to check the server’s timezone settings, which can typically be done through the server’s configuration files or command-line tools. By ensuring both PHP and the server are synchronized, developers can mitigate issues related to time discrepancies.
Another key takeaway is the importance of consistently using UTC (Coordinated Universal Time) for storing timestamps in databases. This practice not only simplifies the handling of time data across different geographical locations but also reduces the risk of errors during daylight saving time changes. Furthermore, developers should consider implementing timezone-aware date and time handling in their applications to accommodate users in various locations effectively.
addressing PHP timezone mism
Author Profile
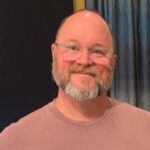
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?