How Can You Effectively Catch Exceptions in Perl?
In the world of programming, handling errors gracefully is as crucial as writing the initial code itself. For developers using Perl, mastering the art of exception handling can significantly enhance the robustness and reliability of their applications. Whether you’re building a simple script or a complex system, understanding how to catch exceptions allows you to anticipate potential issues and respond to them effectively, ensuring a smoother user experience and minimizing downtime. This article delves into the techniques and best practices for catching exceptions in Perl, empowering you to write cleaner, more resilient code.
When working with Perl, exceptions can arise from various sources, including user input errors, system failures, or unexpected behavior from external libraries. By implementing proper exception handling, you can create a safety net that not only captures these errors but also provides meaningful feedback, making debugging easier and improving overall application performance. Perl offers several built-in mechanisms for managing exceptions, and understanding these tools is essential for any developer looking to elevate their coding skills.
As we explore the intricacies of exception handling in Perl, we will cover the fundamental concepts, including how to use the `eval` block to catch errors, the importance of the `$@` variable for error reporting, and best practices for structuring your code to handle exceptions gracefully. Whether you’re a seasoned Perl
Understanding Exceptions in Perl
In Perl, exceptions are a mechanism for handling errors that occur during the execution of a program. The language provides built-in support for exception handling, allowing developers to manage errors gracefully without terminating the program unexpectedly.
Exceptions in Perl can arise from various sources, including:
- Syntax errors
- Runtime errors
- Custom error conditions defined by the programmer
To effectively catch and handle exceptions, Perl employs the `eval` block. This block captures any errors that occur within its scope, allowing for controlled error management.
Using the eval Block
The `eval` block is the primary method for catching exceptions in Perl. When code is executed inside an `eval`, any errors thrown will not cause the program to terminate; instead, they can be captured and handled appropriately.
Here is a simple example demonstrating the use of `eval`:
“`perl
my $result = eval {
Code that might generate an error
my $value = 1 / 0; This will cause a division by zero error
return $value;
};
if ($@) {
print “An error occurred: $@\n”; $@ contains the error message
} else {
print “Result is $result\n”;
}
“`
In this example, the division by zero error is caught, and the error message is stored in the special variable `$@`. The code following the `eval` block can then check this variable to determine if an error occurred.
Custom Exception Handling
Perl also allows for custom exceptions using the `die` function, which can be used to throw an exception. The thrown exception can be caught using `eval`, providing a mechanism for defining specific error conditions.
Here’s how to define and catch a custom exception:
“`perl
sub risky_function {
die “Custom error: Something went wrong!”;
}
my $result = eval {
risky_function();
};
if ($@) {
print “Caught exception: $@\n”;
}
“`
By using `die`, developers can create specific error messages that can be meaningful to the context of the application.
Best Practices for Exception Handling
When implementing exception handling in Perl, consider the following best practices:
- Always use `eval` to encapsulate code that might throw an exception.
- Check the `$@` variable immediately after the `eval` block to determine if an error occurred.
- Use descriptive messages with `die` to facilitate easier debugging.
- Avoid using `eval` for control flow; it should be reserved for error handling.
Comparison of Exception Handling Mechanisms
Method | Description | Use Case |
---|---|---|
eval | Captures runtime errors and exceptions. | When executing potentially faulty code. |
die | Throws an exception intentionally. | When a function cannot continue due to an error. |
try/catch (via modules) | Provides a more structured exception handling. | For complex applications requiring detailed error handling. |
Utilizing these methods and practices will enhance the robustness of Perl applications by allowing for effective error management and reducing program crashes due to unhandled exceptions.
Understanding Exception Handling in Perl
Perl uses the `eval` block for exception handling, allowing developers to catch runtime errors. When an error occurs, it does not terminate the program; instead, it can be handled gracefully.
Using `eval` to Catch Exceptions
The `eval` block executes code and captures any exceptions that arise. Syntax for using `eval` is straightforward:
“`perl
eval {
Code that may throw an exception
};
if ($@) {
Handle the exception
}
“`
- The code inside the `eval` block is executed.
- If an error occurs, `$@` is populated with the error message.
- If no error occurs, `$@` remains .
Example of Exception Handling
Consider the following example that demonstrates exception handling:
“`perl
eval {
open my $fh, ‘<', 'nonexistent_file.txt' or die "Cannot open file: $!";
Further file processing code
};
if ($@) {
print "Caught an exception: $@\n";
}
```
In this code:
- Attempting to open a non-existent file will trigger an exception.
- The error message will be captured in `$@`.
Using `try` and `catch` with CPAN Modules
Perl does not natively support `try` and `catch` constructs, but you can utilize CPAN modules such as `Try::Tiny` for a more structured approach.
“`perl
use Try::Tiny;
try {
Code that may throw an exception
die “An error occurred”;
} catch {
my $error = $_; Captured error
warn “Caught error: $error\n”;
};
“`
Advantages of Using `Try::Tiny`:
- Simplifies error handling syntax.
- Reduces scope for errors.
- Allows for more readable and maintainable code.
Best Practices for Exception Handling
- Always check `$@` after an `eval` block to determine if an exception occurred.
- Use descriptive error messages to facilitate debugging.
- Limit the scope of `eval` to only the necessary code to avoid unintended behaviors.
- Consider using modules like `Try::Tiny` for cleaner syntax and more robust error handling.
Common Scenarios for Exception Handling
Scenario | Example Code |
---|---|
File I/O operations | `open` or `close` file handles |
Database interactions | Handling connection errors with DBI |
User input validations | Checking for valid data formats |
External command execution | Using `qx//` or backticks to run shell commands |
Logging Exceptions
Logging exceptions can aid in diagnosing issues. Use the following approach:
“`perl
use Log::Log4perl;
Log::Log4perl->init(‘log4perl.conf’);
eval {
Risky operation
};
if ($@) {
my $logger = Log::Log4perl->get_logger();
$logger->error(“Caught exception: $@”);
}
“`
- Configure logging in a separate configuration file.
- Capture error messages and log them for future analysis.
Implementing robust exception handling in Perl is essential for building resilient applications. By using `eval`, CPAN modules like `Try::Tiny`, and adhering to best practices, developers can effectively manage errors and enhance the reliability of their code.
Expert Insights on Exception Handling in Perl
Dr. Emily Carter (Senior Software Engineer, Perl Solutions Inc.). “In Perl, catching exceptions effectively requires a solid understanding of the eval block. By using eval, developers can encapsulate code that may produce errors, allowing for graceful handling without crashing the program.”
Michael Thompson (Lead Developer, Open Source Perl Community). “Utilizing the ‘die’ and ‘warn’ functions in conjunction with eval can significantly enhance error management. It is crucial to implement a strategy that not only catches exceptions but also logs them for future debugging.”
Sarah Patel (Technical Writer, Perl Programming Journal). “When handling exceptions in Perl, it is essential to adopt a consistent approach. Using custom exception classes can improve code readability and maintainability, making it easier for teams to manage errors in complex applications.”
Frequently Asked Questions (FAQs)
What is exception handling in Perl?
Exception handling in Perl refers to the process of responding to unexpected events or errors that occur during the execution of a program. It allows developers to manage errors gracefully without crashing the application.
How do I catch exceptions in Perl?
In Perl, exceptions can be caught using the `eval` block. Code that may throw an exception is placed inside the `eval`, and if an error occurs, it can be captured using the special variable `$@`, which contains the error message.
What is the purpose of the `die` function in Perl?
The `die` function in Perl is used to raise an exception. When `die` is called, it terminates the program and returns an error message. This message can be caught by an `eval` block for further handling.
Can I use `try` and `catch` in Perl?
Perl does not have built-in `try` and `catch` constructs like some other programming languages. However, you can implement similar functionality using `eval` for error trapping and `die` for throwing exceptions.
How can I handle multiple exceptions in Perl?
To handle multiple exceptions in Perl, you can use multiple `eval` blocks or check the contents of `$@` after each `eval` execution. You can also define custom error handling routines to differentiate between various types of errors.
Is there a way to create custom exceptions in Perl?
Yes, you can create custom exceptions in Perl by defining your own error classes. This involves using the `bless` function to associate an error message with an object, allowing you to throw and catch specific types of exceptions in your code.
In Perl, exception handling is primarily managed through the use of the `eval` block, which allows developers to catch runtime errors and handle them gracefully. By wrapping potentially problematic code within an `eval`, any errors that occur will not terminate the program but can instead be captured and processed. This mechanism is essential for writing robust and resilient Perl applications that can recover from unexpected situations.
Another important aspect of exception handling in Perl is the use of the special variable `$@`, which holds the error message if an exception occurs during the execution of the `eval` block. Developers can utilize this variable to determine the nature of the error and implement appropriate recovery strategies. Additionally, Perl provides the `die` function to signal an error condition, which can be caught and managed within the `eval` structure, enhancing the control over error handling.
Key takeaways from the discussion on catching exceptions in Perl include the importance of using `eval` for error management, the role of the `$@` variable in identifying errors, and the utility of the `die` function for signaling exceptions. By mastering these tools, Perl developers can create more reliable and user-friendly applications that effectively manage errors and exceptions, ultimately leading to a better user experience.
Author Profile
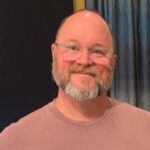
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?