How Can You Pass a Variable to a UserForm from a Module in VBA?
In the world of Excel VBA, userforms are powerful tools that enhance user interaction and streamline data entry processes. However, one of the common challenges developers face is the effective passing of variables from standard modules to userforms. This seemingly straightforward task can significantly impact the functionality and user experience of your applications. Whether you’re creating a simple data input form or a complex dashboard, understanding how to transfer data seamlessly between modules and userforms is essential for creating robust and efficient solutions.
Passing variables to userforms not only allows for dynamic content but also empowers developers to customize the user experience based on specific criteria or user input. By leveraging VBA’s capabilities, you can create a more interactive interface that responds to the needs of the user in real-time. This process involves understanding the scope of your variables, the lifecycle of userforms, and how to manipulate properties effectively to achieve the desired outcome.
As we delve deeper into this topic, we will explore various techniques and best practices for passing variables to userforms in VBA. From defining your variables to utilizing them within the userform, you’ll gain insights that will elevate your programming skills and enhance your projects. Whether you’re a beginner or an experienced developer, mastering this skill will undoubtedly broaden your horizons in the realm of Excel automation.
Understanding UserForms in VBA
UserForms in VBA are powerful tools that allow developers to create custom dialog boxes for user interaction. They can be used to gather input, display information, or guide users through complex processes. When working with UserForms, one common requirement is passing variables from a module to the UserForm, enabling dynamic content and functionality.
To pass variables to a UserForm, it’s essential to understand the structure of the UserForm and how to manipulate its properties effectively.
Creating a UserForm
To create a UserForm in VBA:
- Open the Visual Basic for Applications (VBA) editor (press `ALT + F11`).
- Right-click on any of the items in the Project Explorer.
- Select `Insert` -> `UserForm`.
- Design your UserForm using the toolbox by adding controls like text boxes, labels, and buttons.
Once your UserForm is created, you can define properties and methods to manage how it behaves.
Passing Variables to a UserForm
To pass variables from a module to a UserForm, you can utilize properties. Here’s a structured approach:
- Define Public Properties: In the UserForm’s code module, define public properties that can hold the values you want to pass.
“`vba
‘ In UserForm code
Public UserInput As String
“`
- Set Property Values: In your main module, set the values of these properties before showing the UserForm.
“`vba
‘ In Module code
Sub ShowUserForm()
Dim myForm As New UserForm1
myForm.UserInput = “Hello, User!”
myForm.Show
End Sub
“`
- Use the Properties in UserForm: You can then use the value of the property within the UserForm to update controls or display information.
“`vba
‘ In UserForm code
Private Sub UserForm_Initialize()
Me.Label1.Caption = UserInput
End Sub
“`
This method ensures that when the UserForm initializes, it retrieves the value passed from the module.
Example of Passing Multiple Variables
When passing multiple variables, you can define several public properties in the UserForm. Here’s an example showcasing how to pass multiple variables:
“`vba
‘ In UserForm code
Public UserName As String
Public UserAge As Integer
“`
In the main module, set these properties before displaying the UserForm:
“`vba
‘ In Module code
Sub ShowUserForm()
Dim myForm As New UserForm1
myForm.UserName = “Alice”
myForm.UserAge = 30
myForm.Show
End Sub
“`
Within the UserForm, you can access these properties as follows:
“`vba
‘ In UserForm code
Private Sub UserForm_Initialize()
Me.Label1.Caption = “Name: ” & UserName
Me.Label2.Caption = “Age: ” & UserAge
End Sub
“`
Table of Common UserForm Controls
Below is a table summarizing common controls used in UserForms and their typical use cases:
Control | Description | Common Use |
---|---|---|
TextBox | Allows user input of text | Collecting user input |
Label | Displays static text | Providing instructions or information |
ComboBox | Drop-down list for user selection | Choosing from a list of options |
CommandButton | Button to execute actions | Submitting data or closing the form |
Using these controls effectively within UserForms enhances user experience by creating intuitive interfaces for data entry and interaction.
Passing Variables to a UserForm in VBA
To effectively pass variables from a module to a UserForm in VBA, it’s essential to understand the different methods available. Each method has its use cases and advantages, depending on the complexity and requirements of your project.
Using Public Variables
One of the simplest ways to pass variables is by declaring them as public variables in a standard module. This approach allows any UserForm to access the variable directly.
- Step 1: Declare a public variable in a module.
“`vba
‘ Module1
Public myVariable As String
“`
- Step 2: Assign a value to the variable in your code.
“`vba
Sub AssignValue()
myVariable = “Hello, UserForm!”
UserForm1.Show
End Sub
“`
- Step 3: Access the variable in the UserForm.
“`vba
‘ UserForm1
Private Sub UserForm_Initialize()
Me.Label1.Caption = myVariable
End Sub
“`
Using Properties in UserForms
Another effective method is to create properties within the UserForm. This allows for encapsulated data transfer and can enhance code readability.
- Step 1: Define a property in the UserForm.
“`vba
‘ UserForm1
Private pMyVariable As String
Public Property Let MyVariable(Value As String)
pMyVariable = Value
End Property
Public Property Get MyVariable() As String
MyVariable = pMyVariable
End Property
“`
- Step 2: Set the property before showing the UserForm.
“`vba
Sub ShowUserForm()
Dim frm As UserForm1
Set frm = New UserForm1
frm.MyVariable = “Data to display”
frm.Show
End Sub
“`
- Step 3: Use the property in the UserForm.
“`vba
Private Sub UserForm_Initialize()
Me.Label1.Caption = Me.MyVariable
End Sub
“`
Using Parameters in UserForm Methods
If you prefer to pass variables directly during the UserForm invocation, you can create a method that accepts parameters.
- Step 1: Define a method in the UserForm that accepts parameters.
“`vba
‘ UserForm1
Public Sub InitializeForm(ByVal inputValue As String)
Me.Label1.Caption = inputValue
End Sub
“`
- Step 2: Call the method before showing the UserForm.
“`vba
Sub ShowUserForm()
Dim frm As UserForm1
Set frm = New UserForm1
frm.InitializeForm “Dynamic Value”
frm.Show
End Sub
“`
Example Table: Method Comparison
Method | Complexity | Encapsulation | Ease of Use |
---|---|---|---|
Public Variables | Low | No | High |
UserForm Properties | Medium | Yes | Medium |
Parameters in Methods | High | Yes | Low |
Each of these methods can be utilized based on the specific needs of your application, allowing for flexible and maintainable code.
Expert Insights on Passing Variables to UserForms in VBA
Dr. Emily Carter (Senior VBA Developer, Tech Solutions Corp). “Passing variables to UserForms in VBA is essential for creating dynamic applications. By using properties within the UserForm, developers can easily transfer data from modules, ensuring that the UserForm reflects the most current information and enhances user interaction.”
Michael Tran (Lead Software Engineer, CodeCrafters Inc). “Utilizing the ‘Show’ method in conjunction with public variables is a straightforward approach to pass values to UserForms. This method not only simplifies the code but also improves maintainability by keeping the data flow clear and organized.”
Sarah Johnson (VBA Programming Consultant, Excel Wizards). “To effectively pass variables to UserForms, it is crucial to define the variables at the module level and ensure they are accessible. This practice not only promotes reusability but also minimizes the likelihood of errors during runtime, leading to a more robust application.”
Frequently Asked Questions (FAQs)
How can I pass a variable from a module to a userform in VBA?
To pass a variable from a module to a userform, you can create a public property in the userform. Set the variable in the module and then call the userform, accessing the property to retrieve the value.
What is the purpose of using public properties in a userform?
Public properties in a userform allow you to expose internal data to other modules or forms. This enables you to pass values easily and maintain encapsulation within your userform.
Can I pass multiple variables to a userform from a module?
Yes, you can pass multiple variables by defining multiple public properties in the userform. You can set each property from the module before displaying the userform.
What is the syntax for setting a public property in a userform?
The syntax for setting a public property in a userform is as follows:
“`vba
Public Property Let MyVariable(value As Variant)
myVariable = value
End Property
Public Property Get MyVariable() As Variant
MyVariable = myVariable
End Property
“`
How do I show the userform after passing the variable?
After setting the desired properties in the userform, you can display it using the `Show` method. For example:
“`vba
Dim myForm As New UserForm1
myForm.MyVariable = myValue
myForm.Show
“`
Is it possible to pass an array to a userform in VBA?
Yes, you can pass an array to a userform by defining a public property that accepts an array. Use the `Public Property Let` method to handle the array input appropriately.
In summary, passing variables to a UserForm from a module in VBA is a fundamental technique that enhances the interactivity and functionality of applications built within Excel or other Microsoft Office programs. This process typically involves defining public variables within a standard module, which can then be accessed by the UserForm. By utilizing this method, developers can ensure that data is seamlessly transferred between different components of their applications, allowing for a more dynamic user experience.
Moreover, the implementation of this technique requires a clear understanding of the scope of variables and the appropriate event handlers within the UserForm. By effectively managing the lifecycle of the variables, developers can create robust forms that respond accurately to user inputs. This not only improves the usability of the UserForm but also minimizes errors and enhances data integrity.
Key takeaways from the discussion include the importance of defining variables with the correct scope, utilizing event procedures to handle user interactions, and ensuring that data is appropriately initialized and displayed within the UserForm. By mastering these concepts, VBA developers can significantly elevate the quality and effectiveness of their applications, resulting in a more professional and user-friendly interface.
Author Profile
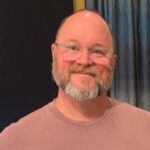
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?