How Can I Resolve the Paramiko.Ssh_Exception.Sshexception: Error Reading SSH Protocol Banner?
In the world of network administration and secure communications, the SSH (Secure Shell) protocol plays a pivotal role in ensuring that data is transmitted safely and efficiently. However, as with any technology, challenges can arise that may disrupt seamless connectivity. One such challenge is the `Paramiko.Ssh_Exception.Sshexception: Error Reading Ssh Protocol Banner`, a cryptic error that can leave even seasoned developers scratching their heads. Understanding this error is crucial for anyone working with SSH connections, as it can indicate underlying issues that, if left unaddressed, may compromise the integrity of your secure communications.
At its core, this error signifies a failure in the initial handshake process between the client and server when establishing an SSH connection. The SSH protocol begins with a banner exchange, where the client and server identify themselves and negotiate parameters for the session. When this exchange is interrupted or malformed, it can trigger the `Sshexception`, leading to confusion and frustration for users trying to establish a connection. Factors such as network issues, server misconfigurations, or even firewall restrictions can contribute to this problem, making it essential to diagnose the root cause effectively.
As we delve deeper into the intricacies of this error, we will explore its common causes, potential solutions, and best practices for troubleshooting.
Understanding the Cause of `Paramiko.Ssh_Exception.Sshexception`
The `Paramiko.Ssh_Exception.Sshexception: Error Reading Ssh Protocol Banner` error typically arises due to issues encountered during the initial handshake of the SSH protocol. This phase is crucial, as it establishes the connection parameters and sets the stage for secure communication. The following are common reasons for this exception:
- Network Connectivity Issues: Problems such as firewall restrictions, DNS resolution failures, or network timeouts can prevent proper communication between the client and server.
- SSH Server Configuration: If the SSH server is misconfigured or not running, it may not send the expected protocol banner, leading to this exception.
- Protocol Mismatch: The client and server may not support compatible versions of the SSH protocol, causing the handshake to fail.
- Timeouts: If the server takes too long to respond during the banner exchange, the client may time out and raise this exception.
Troubleshooting Steps
To resolve the `Error Reading Ssh Protocol Banner`, consider the following troubleshooting steps:
- Check Network Connectivity:
- Use tools like `ping` or `traceroute` to ensure the client can reach the SSH server.
- Verify that firewalls or security groups allow SSH traffic (port 22 by default).
- Verify SSH Server Status:
- Ensure the SSH server is active and listening on the correct port.
- Check the server’s logs for any errors or warnings that may indicate issues.
- Test with SSH Client:
- Use a command-line SSH client to manually connect to the server. This can help determine if the issue lies with the Paramiko implementation or the server itself.
- Review SSH Configuration:
- Check both client and server configurations for any discrepancies in supported protocols or authentication methods.
- Increase Timeout Settings:
- Adjust timeout settings in the Paramiko client to allow for slower responses from the server.
Example SSH Connection Code Using Paramiko
Here’s an example of how to establish an SSH connection using Paramiko, including error handling for the `Sshexception`:
“`python
import paramiko
hostname = ‘your.ssh.server’
username = ‘your_username’
password = ‘your_password’
try:
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect(hostname, username=username, password=password, timeout=10)
except paramiko.SSHException as e:
print(f”SSH Exception: {e}”)
except Exception as e:
print(f”General Exception: {e}”)
finally:
client.close()
“`
Table of Common SSH Error Codes
Error Code | Description |
---|---|
0 | Success |
1 | Connection refused |
2 | Timeout error |
3 | Authentication failed |
4 | Protocol mismatch |
By methodically assessing the potential causes and following the outlined troubleshooting steps, users can effectively resolve the `Paramiko.Ssh_Exception.Sshexception: Error Reading Ssh Protocol Banner` error and establish a successful SSH connection.
Understanding the SshException
The `Paramiko.Ssh_Exception.Sshexception: Error Reading Ssh Protocol Banner` typically arises when there is an issue with the SSH connection setup. This exception indicates that the client could not read the expected SSH protocol banner from the server.
Common Causes
Several factors can lead to this exception, including:
- Network Issues: Loss of connectivity or misconfigured firewall settings can prevent the client from reaching the server.
- Server Configuration: The SSH service on the server may not be running, or it could be configured to use a non-standard port.
- Protocol Mismatch: The server might be using an incompatible version of the SSH protocol.
- Time-Outs: A delay in server response can trigger a timeout, causing the client to fail in reading the banner.
- Non-SSH Services: Attempting to connect to a service that does not use SSH can result in unexpected data being read.
Troubleshooting Steps
To resolve the `SshException`, consider the following troubleshooting steps:
- Verify Server Availability: Ensure that the SSH server is operational and reachable.
- Use `ping` to check connectivity.
- Use `telnet
` to check if the SSH port is open.
- Check SSH Configuration: Review the SSH configuration files on the server.
- Ensure `sshd` is running: `sudo systemctl status sshd`
- Confirm the SSH port is correct (default is 22).
- Inspect Firewall Settings: Ensure that firewalls on both the client and server allow traffic on the SSH port.
- Use Verbose Mode: Enable verbose output in your Paramiko connection to get more details:
“`python
import paramiko
paramiko.util.log_to_file(“paramiko.log”, level=”DEBUG”)
“`
- Test SSH with Command Line: Attempt to connect using SSH from a command line to see if the issue persists:
“`bash
ssh -v user@hostname
“`
Example Code Snippet
Here is an example of how to handle `SshException` in your code:
“`python
import paramiko
try:
client = paramiko.SSHClient()
client.set_missing_host_key_policy(paramiko.AutoAddPolicy())
client.connect(‘hostname’, username=’user’, password=’pass’)
except paramiko.SSHException as e:
print(f”SSH connection failed: {e}”)
except Exception as e:
print(f”An error occurred: {e}”)
finally:
client.close()
“`
Best Practices
To minimize the occurrence of this exception, adhere to the following best practices:
- Regularly Update Software: Keep both client and server software updated to ensure compatibility.
- Monitor Logs: Regularly check server logs for SSH-related errors to proactively address issues.
- Implement Security Measures: Use secure authentication methods and change the default SSH port to reduce exposure to attacks.
Resources for Further Investigation
Utilize the following resources to gather more information regarding SSH issues:
Resource | Description |
---|---|
Paramiko Documentation | Official documentation for Paramiko library. |
OpenSSH Manual | Comprehensive guide on OpenSSH configurations. |
Network Troubleshooting Guide | General troubleshooting steps for network issues. |
By following these guidelines and utilizing the resources provided, you can effectively address and prevent the `SshException` in your SSH connections.
Understanding the Challenges of SSH Protocol Banners
Dr. Emily Carter (Network Security Analyst, CyberDefense Solutions). “The ‘Paramiko.Ssh_Exception.Sshexception: Error Reading Ssh Protocol Banner’ typically indicates an issue with the SSH server’s response. This can arise from misconfigurations or network interruptions that prevent the client from receiving the expected banner.”
Mark Thompson (Senior DevOps Engineer, Tech Innovations Inc.). “When encountering this error, it is crucial to verify the SSH server settings. An improperly set up server could fail to send the protocol banner, leading to connection issues that manifest as this specific exception.”
Lisa Chen (Lead Software Developer, SecureConnect Technologies). “In my experience, this error often surfaces in environments with strict firewall rules. Ensuring that the necessary ports are open and that the server is reachable can help mitigate these connection problems.”
Frequently Asked Questions (FAQs)
What does the error “Paramiko.Ssh_Exception.Sshexception: Error Reading Ssh Protocol Banner” indicate?
This error indicates that the Paramiko library encountered an issue while trying to read the SSH protocol banner from the server. This can occur due to network issues, server misconfiguration, or the server not responding as expected.
What are common causes of the “Error Reading Ssh Protocol Banner”?
Common causes include network connectivity problems, SSH server not running, firewall restrictions, or the server sending an unexpected response that does not conform to the SSH protocol.
How can I troubleshoot this error?
To troubleshoot, check network connectivity to the SSH server, ensure the SSH service is running on the server, verify firewall settings, and confirm that the server is configured correctly to accept SSH connections.
Does this error occur only with Paramiko, or can it happen with other SSH clients?
While this specific error message is from Paramiko, similar issues can occur with other SSH clients if they encounter problems reading the SSH banner. The underlying causes will typically be the same.
What steps can I take if the server is behind a firewall?
If the server is behind a firewall, ensure that the firewall is configured to allow traffic on the SSH port (usually port 22). Additionally, check for any network address translation (NAT) issues that might affect connectivity.
Is there a way to increase the timeout for reading the SSH banner in Paramiko?
Yes, you can increase the timeout by setting the `timeout` parameter when creating the SSH client or during the connection method. This allows more time for the server to respond before raising an exception.
The error message “Paramiko.Ssh_Exception.Sshexception: Error Reading Ssh Protocol Banner” indicates a failure in establishing a secure SSH connection using the Paramiko library in Python. This issue typically arises when the client is unable to read the SSH protocol banner from the server, which is a critical step in the SSH handshake process. The banner is essential for identifying the server’s SSH version and capabilities, and any disruption in this communication can lead to connection failures.
Several factors can contribute to this error. Network issues, such as firewalls or incorrect routing, may prevent the client from reaching the server. Additionally, server misconfigurations, such as incorrect SSH settings or the server being down, can also lead to this exception. It is crucial for users to ensure that the server is operational and correctly configured to accept SSH connections. Furthermore, verifying that the client can reach the server over the designated port (typically port 22) is essential for troubleshooting this issue.
addressing the “Error Reading Ssh Protocol Banner” requires a systematic approach to diagnose potential network and server configuration issues. Users should check connectivity, firewall settings, and server status as initial steps in troubleshooting. Understanding the importance of the SSH protocol banner in the
Author Profile
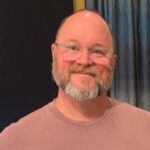
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?