Why Does the Error ‘ORA-01427: Single Row Subquery Returns More Than One Row’ Occur and How Can You Fix It?
In the world of database management, encountering errors can be a common yet frustrating experience, especially when they disrupt the flow of your queries. One such error that developers often face is the infamous “ORA-01427: single-row subquery returns more than one row.” This error serves as a crucial reminder of the importance of understanding how subqueries function within SQL, particularly in Oracle databases. Whether you’re a seasoned database administrator or a budding programmer, grasping the nuances of this error can significantly enhance your troubleshooting skills and improve the efficiency of your data retrieval processes.
When working with SQL, subqueries are powerful tools that allow users to perform complex queries by nesting one query within another. However, the ORA-01427 error arises when a subquery that is expected to return a single value inadvertently returns multiple rows. This situation can lead to confusion and disrupt the intended logic of your SQL statements. Understanding the root causes of this error is essential for anyone looking to write effective and efficient SQL code.
In this article, we will explore the intricacies of the ORA-01427 error, examining its common triggers and the best practices to avoid it. By delving into the mechanics of subqueries and their expected outputs, we aim to equip you with the knowledge needed
Understanding the Error
The `ORA-01427: single-row subquery returns more than one row` error occurs in Oracle databases when a subquery is expected to return a single row but instead returns multiple rows. This situation typically arises in SQL statements where a subquery is used in a context that requires a single value, such as in a condition for a WHERE clause or an assignment in a SELECT statement.
This error can manifest in various scenarios, such as:
- Using a subquery in the SELECT list that is not restricted to a single row.
- Employing a subquery in a comparison operation where only a single value is acceptable.
Common Causes
Several factors can lead to this error. Understanding these can help in troubleshooting and resolving the issue:
- Unrestricted Subquery: The subquery does not have conditions limiting its result to one row.
- Join Conditions: Multiple records in the parent query may match multiple records in the subquery.
- Inaccurate Aggregation: The absence of aggregate functions or GROUP BY clauses can cause unexpected multiple results.
How to Resolve the Error
To resolve the `ORA-01427` error, you can take the following approaches:
- Limit the Subquery Results: Use conditions to ensure that the subquery returns only one row. This can be done using:
- `ROWNUM`
- `LIMIT` (in SQL standards)
- Aggregate functions like `MAX()` or `MIN()`
- Refactor the Query: Consider restructuring the query to eliminate the need for a subquery if it can be done with a JOIN.
- Use EXISTS or IN: Instead of using a direct comparison that expects a single value, you can use `EXISTS` or `IN` to handle multiple rows gracefully.
Approach | Description |
---|---|
Subquery Limitation | Modify the subquery to include conditions that ensure only one row is returned. |
Refactor with JOIN | Rewrite the query using JOINs to combine tables instead of using subqueries. |
Using EXISTS/IN | Change the query to use `EXISTS` or `IN`, which can handle multiple rows. |
Examples
- Subquery Limitation:
“`sql
SELECT employee_id
FROM employees
WHERE department_id = (SELECT department_id FROM departments WHERE location_id = 100);
“`
If the subquery returns multiple `department_id`s, it will trigger the error. To fix it:
“`sql
SELECT employee_id
FROM employees
WHERE department_id = (SELECT MAX(department_id) FROM departments WHERE location_id = 100);
“`
- Using EXISTS:
Instead of using a subquery that returns multiple values, you can use:
“`sql
SELECT employee_id
FROM employees e
WHERE EXISTS (SELECT 1 FROM departments d WHERE d.department_id = e.department_id);
“`
These strategies will help you avoid the `ORA-01427` error and improve the overall robustness of your SQL queries.
Understanding the Error
The `ORA-01427: single-row subquery returns more than one row` error occurs in Oracle databases when a subquery intended to return a single value instead returns multiple values. This situation typically arises within SQL statements that use operators like `=`, `<`, or `>`, which expect a single result.
Key reasons for encountering this error include:
- Improper Subquery Logic: The subquery is not adequately constrained to ensure it returns a single row.
- Data Issues: The underlying data in the table may contain unexpected duplicates.
Common Scenarios Leading to the Error
Several common scenarios can trigger the `ORA-01427` error:
- Using Subqueries in SELECT Statements: When attempting to select from a table using a subquery that should return a single value but returns multiple rows.
- WHERE Clause Conditions: Using a subquery in the `WHERE` clause that does not properly filter results.
- Join Conditions: Improper join conditions where a subquery is expected to return a single record for each row in the main query.
Example of the Error
Consider the following SQL statement:
“`sql
SELECT employee_id, (SELECT department_id FROM departments WHERE location_id = 1000) AS dept_id
FROM employees;
“`
If the subquery returns multiple `department_id`s for `location_id = 1000`, it will raise the `ORA-01427` error.
How to Resolve the Error
To resolve the `ORA-01427` error, consider the following approaches:
- Refine the Subquery: Ensure that the subquery is designed to return only one row.
“`sql
SELECT employee_id,
(SELECT department_id FROM departments WHERE location_id = 1000 AND ROWNUM = 1) AS dept_id
FROM employees;
“`
- Use Aggregate Functions: If you need a summary value, apply aggregate functions like `MAX()`, `MIN()`, or `COUNT()`.
“`sql
SELECT employee_id,
(SELECT MAX(department_id) FROM departments WHERE location_id = 1000) AS dept_id
FROM employees;
“`
- Check for Duplicates: Validate the data in the subquery’s table to eliminate duplicates that might cause multiple rows to return.
- Utilize `IN` Instead of `=`: If the intention is to check against multiple values, consider using the `IN` operator.
“`sql
SELECT employee_id
FROM employees
WHERE department_id IN (SELECT department_id FROM departments WHERE location_id = 1000);
“`
Best Practices to Avoid the Error
To mitigate the risk of encountering the `ORA-01427` error, follow these best practices:
- Always Test Subqueries: Execute subqueries independently to check the returned results before integrating them into larger queries.
- Leverage Joins: Instead of using subqueries, consider utilizing joins which often provide clearer logic and performance benefits.
- Implement Data Constraints: Use primary and unique keys to prevent unintentional duplicates in your tables.
- Thoroughly Review Query Logic: Regularly review query structures to ensure that the logic aligns with the expected results.
By following these guidelines, you can effectively handle and prevent the `ORA-01427: single-row subquery returns more than one row` error in your SQL queries.
Understanding the Challenges of Subqueries in SQL
Dr. Lisa Chen (Database Systems Analyst, Tech Innovations Inc.). “The error ‘ORA-01427: single-row subquery returns more than one row’ typically arises when a subquery that is expected to return a single value instead returns multiple rows. This often indicates a flaw in the query logic, where the conditions set for the subquery do not sufficiently narrow down the results.”
Michael Thompson (Senior Database Administrator, Data Solutions Group). “To resolve the ORA-01427 error, developers should review the subquery criteria. Utilizing aggregate functions or refining the WHERE clause can help ensure that the subquery yields a single result, thus preventing this common pitfall in SQL programming.”
Sarah Patel (SQL Performance Expert, Query Optimization Co.). “It’s crucial for developers to understand the context in which subqueries are used. When a subquery is expected to return a single row, implementing techniques like DISTINCT or limiting the results with ROWNUM can be effective strategies to avoid the ORA-01427 error.”
Frequently Asked Questions (FAQs)
What does the error “Ora 01427: single-row subquery returns more than one row” mean?
This error indicates that a subquery designed to return a single value is returning multiple rows, which violates the expectation of a single result.
How can I identify the subquery causing the Ora 01427 error?
To identify the problematic subquery, review the SQL statement and locate any subqueries used in the SELECT, WHERE, or HAVING clauses. Testing each subquery independently can help pinpoint the one returning multiple rows.
What are common scenarios that lead to the Ora 01427 error?
Common scenarios include using a subquery in a WHERE clause that matches multiple rows in the parent query, or when using aggregate functions without proper grouping, leading to unexpected results.
How can I resolve the Ora 01427 error?
To resolve the error, ensure that the subquery is modified to return a single row. This can be achieved by using aggregate functions (like MAX or MIN), adding appropriate filters, or restructuring the query logic.
Is it possible to use a subquery that returns multiple rows in a single-row context?
No, a subquery that returns multiple rows cannot be used in a context that expects a single value. Instead, consider using JOINs or IN clauses to accommodate multiple results.
What are some best practices to avoid the Ora 01427 error?
Best practices include validating subqueries to ensure they return a single row, using explicit limits (like ROWNUM or LIMIT), and employing JOINs when multiple rows are expected to match. Regularly testing and reviewing SQL queries can also help prevent this error.
The Oracle error code ORA-01427 indicates that a single-row subquery has returned more than one row, which violates the expectation of the query. This error typically arises in SQL statements where a subquery is used in a context that requires a single value, such as in a WHERE clause or when assigning a value to a variable. Understanding the context in which this error occurs is crucial for effective troubleshooting and resolution.
To address the ORA-01427 error, it is essential to analyze the subquery involved. One must ensure that the subquery is designed to return only a single row. This can be achieved through various methods, including using aggregate functions, adding appropriate filters, or restructuring the query to avoid ambiguity. Additionally, utilizing the DISTINCT keyword can help eliminate duplicate results that might lead to multiple rows being returned.
Key takeaways from the discussion on ORA-01427 include the importance of validating subqueries and ensuring they align with the expected output of the main query. Developers should also consider the use of JOINs as an alternative approach to subqueries, which can sometimes provide clearer logic and avoid the pitfalls that lead to this error. Ultimately, careful query design and thorough testing are vital in preventing the occurrence of ORA
Author Profile
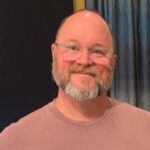
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?