How Can I Resolve the Operand Type Clash: Int Is Incompatible With Date?
In the world of programming and database management, the seamless interaction between different data types is crucial for maintaining the integrity and functionality of applications. However, developers often encounter frustrating errors that can halt progress and lead to confusion. One such error is the “Operand Type Clash: Int Is Incompatible With Date.” This message serves as a stark reminder of the importance of understanding data types and their compatibility within various operations. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and how to effectively resolve it to ensure smooth data handling.
Overview
The “Operand Type Clash” error typically arises when an operation attempts to mix incompatible data types, such as integers and dates. This clash can occur in various scenarios, such as when performing calculations, comparisons, or data manipulations within a database query or programming logic. Understanding the underlying principles of data types is essential for developers, as it not only helps in troubleshooting these errors but also fosters better coding practices.
As we navigate through the complexities of this error, we will examine common situations that lead to operand type clashes, the significance of type casting, and best practices for preventing such issues in the future. By gaining insight into these aspects, developers can enhance their problem-solving skills and create
Understanding Operand Type Clash
Operand type clashes occur when there is a mismatch between the types of data being manipulated in an operation. In programming, particularly in SQL databases, this issue often arises when attempting to perform operations that expect a specific data type but receive another. A common scenario is the clash between integer types and date types. This can lead to errors that hinder data retrieval and manipulation.
When an operation expects a date but receives an integer, the database management system (DBMS) throws an error indicating that the operand types are incompatible. This is particularly prevalent in conditions involving comparisons or arithmetic operations.
Common Scenarios of Operand Type Clash
There are several scenarios where an operand type clash might occur. Understanding these can help developers avoid such errors:
- Date Comparisons: Trying to compare a date column with an integer value.
- Function Parameters: Passing an integer to a function that expects a date parameter.
- Arithmetic Operations: Performing calculations that involve both dates and integers.
Examples of Operand Type Clash
Here are some practical examples that illustrate how operand type clashes can occur:
- SQL Query Example:
“`sql
SELECT * FROM orders WHERE order_date = 20230101;
“`
In this case, `order_date` is a date field, and `20230101` is an integer, leading to an operand type clash.
- Function Usage:
“`sql
SELECT DATEDIFF(NOW(), 20230101);
“`
The `DATEDIFF` function expects a date as its second argument, but an integer is provided.
Resolving Operand Type Clashes
To prevent or resolve operand type clashes, consider the following strategies:
- Data Type Conversion: Explicitly convert data types to match the expected types in operations.
- Use of Proper Functions: Ensure that the correct functions are applied to the appropriate data types.
- Validation: Validate inputs to ensure they conform to the expected data types before processing.
Data Type Conversion Example
A common method to resolve type clashes is through data type conversion. Below is a table illustrating how to convert integers to dates in SQL.
Operation | Example | Result |
---|---|---|
Convert Integer to Date | SELECT CAST(20230101 AS DATE); | 2023-01-01 |
Using CONVERT Function | SELECT CONVERT(DATE, 20230101); | 2023-01-01 |
Utilizing the appropriate conversion methods ensures that the operands are compatible, thereby preventing errors related to operand type clashes. This approach not only promotes code reliability but also enhances overall system performance.
Understanding Operand Type Clash in Databases
Operand type clash errors occur when there is a mismatch between the data types of operands in an operation, particularly in SQL queries. This specific error, “Operand Type Clash: Int Is Incompatible With Date,” indicates that an integer value is being compared or operated on in a way that is incompatible with a date value.
Common Scenarios Leading to Operand Type Clash
The following situations frequently result in operand type clashes involving integers and dates:
- Incorrect Data Type in WHERE Clause: A query may attempt to filter records using an integer instead of a date. For example:
“`sql
SELECT * FROM Orders WHERE OrderDate = 20230101;
“`
Here, `20230101` should be a date, not an integer.
- Improper Use of Functions: Using functions that expect a date as input but providing an integer can cause this clash. For instance:
“`sql
SELECT * FROM Users WHERE DATEDIFF(DAY, UserCreated, 100);
“`
The second argument should be a date, not an integer.
- Type Casting Errors: In some cases, implicit type casting does not occur as expected, leading to mismatches. For example:
“`sql
SELECT * FROM Events WHERE EventDate = CAST(12345 AS INT);
“`
The cast should be to a date format.
How to Resolve Operand Type Clash Errors
Resolving operand type clash errors requires careful review of SQL queries and an understanding of data types. Consider the following approaches:
- Use Correct Data Types: Ensure that the data types of operands match. For instance, use `DATE` or `DATETIME` for date comparisons:
“`sql
SELECT * FROM Orders WHERE OrderDate = ‘2023-01-01’;
“`
- Explicit Type Casting: When necessary, explicitly cast values to the correct type:
“`sql
SELECT * FROM Users WHERE UserCreated = CAST(‘2023-01-01’ AS DATE);
“`
- Check Database Schema: Verify the data types defined in the database schema for the fields involved. This can prevent unexpected errors when executing queries.
Best Practices to Avoid Operand Type Clash
To minimize the risk of operand type clash errors, adhere to the following best practices:
- Consistent Data Types: Maintain consistency in data types across your database. Use the same type for columns that will be compared or operated on together.
- Use Parameterized Queries: When constructing queries in application code, utilize parameterized queries to ensure that the correct data types are used.
- Regularly Review Queries: Periodically audit SQL queries for potential type mismatches, especially after schema changes or data type modifications.
- Testing: Implement comprehensive testing strategies to catch operand type clashes before deployment. This can include unit tests and integration tests focusing on data type handling.
By understanding the causes of operand type clashes and implementing best practices, developers can effectively manage and prevent errors related to incompatible data types in SQL queries. Proper data handling ensures smoother database interactions and enhances the reliability of applications.
Understanding Operand Type Clash in Database Management
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “The error message ‘Operand Type Clash Int Is Incompatible With Date’ typically arises when there is an attempt to perform operations between incompatible data types. It is crucial for developers to ensure that the data types in their queries match the expected types in the database schema to prevent such conflicts.”
Mark Thompson (Senior Software Engineer, Data Solutions Corp.). “When encountering an operand type clash, particularly between integers and dates, it is essential to review the logic of your SQL statements. Often, implicit type conversions can lead to unexpected behavior, and explicit conversions should be used to ensure clarity and correctness in data handling.”
Linda Garcia (Data Integrity Specialist, Reliable Data Services). “Addressing the ‘Operand Type Clash’ error requires a thorough understanding of both the data types involved and the operations being executed. Implementing strong type-checking practices during the development phase can significantly reduce the occurrence of such errors in production environments.”
Frequently Asked Questions (FAQs)
What does the error “Operand Type Clash Int Is Incompatible With Date” mean?
This error indicates that there is a type mismatch in a database operation where an integer value is being used in a context that requires a date value, such as in a comparison or assignment.
How can I resolve the “Operand Type Clash Int Is Incompatible With Date” error?
To resolve this error, ensure that the data types of the operands being compared or assigned are compatible. Convert the integer to a date format or adjust the query to use a date value instead.
In which scenarios is this error commonly encountered?
This error is commonly encountered in SQL queries, particularly in WHERE clauses, JOIN conditions, or when inserting data into a table where the column expects a date type.
What SQL functions can be used to convert an integer to a date?
You can use functions such as `CAST()` or `CONVERT()` in SQL to transform an integer to a date format, depending on the specific database system being used.
Can this error occur in programming languages other than SQL?
Yes, this error can occur in various programming languages when performing operations involving different data types, particularly when strict type checking is enforced.
Is there a way to prevent this error from occurring in the future?
To prevent this error, always ensure that data types are explicitly defined and validated before performing operations. Implementing strong data validation and type checking in your code can also help avoid such issues.
The error message “Operand Type Clash: Int Is Incompatible With Date” typically arises in database management systems, particularly when executing SQL queries. This issue occurs when a query attempts to perform operations or comparisons between incompatible data types, such as integers and date values. Understanding the context in which this error appears is crucial for effective troubleshooting and resolution.
One common scenario that leads to this error is when a developer inadvertently uses an integer value in a place where a date is expected, such as in a WHERE clause or during a JOIN operation. This type mismatch can result from a variety of factors, including incorrect assumptions about data types, legacy data issues, or simply typographical errors in the code. Identifying the source of the mismatch is essential for correcting the query and ensuring data integrity.
To mitigate this error, developers should ensure that data types are explicitly defined and correctly matched in their SQL statements. Utilizing functions to convert data types when necessary can also prevent these clashes. Additionally, thorough testing and validation of queries before execution can help identify potential issues early in the development process. By adhering to best practices regarding data type management, developers can reduce the likelihood of encountering operand type clashes in their database operations.
Author Profile
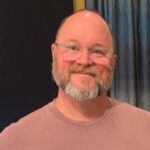
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?