How Can You Set Up Notifications for Changes to Custom Resources in Kubernetes?
In the dynamic world of Kubernetes, managing custom resources effectively is crucial for maintaining the health and performance of your applications. As organizations increasingly rely on Kubernetes for container orchestration, the ability to monitor and respond to changes in custom resources becomes a pivotal aspect of operational excellence. Whether you’re deploying microservices, managing stateful applications, or orchestrating complex workflows, understanding how to notify your team or systems when these resources change can streamline processes and enhance responsiveness.
At its core, the concept of notifying when a custom resource changes revolves around leveraging Kubernetes’ powerful event-driven architecture. By tapping into the Kubernetes API, developers can set up mechanisms that trigger alerts or actions whenever a specified custom resource undergoes modifications. This capability not only aids in proactive monitoring but also facilitates automation, allowing teams to react swiftly to changes that could impact application performance or stability.
In this article, we will explore various strategies and tools available for implementing change notifications for custom resources in Kubernetes. From leveraging built-in features to integrating external systems, you’ll discover how to create a robust notification system that keeps your applications running smoothly and your team informed. Prepare to dive into the intricacies of Kubernetes custom resources and unlock the potential of real-time notifications in your cloud-native environment.
Understanding Kubernetes Custom Resources
Kubernetes custom resources extend the capabilities of Kubernetes by allowing users to define their own resource types. This is particularly useful for managing complex applications and services that require a specific configuration or operational logic not covered by standard Kubernetes objects. Custom resources are defined using Custom Resource Definitions (CRDs), which enable users to specify the schema and behavior of the resource.
Key concepts related to custom resources include:
- CRD: A Custom Resource Definition that defines the structure and validation of a custom resource.
- Controller: A component that watches for changes to custom resources and performs actions based on those changes.
- API Version: Each custom resource can have multiple versions, allowing for changes and improvements over time.
Setting Up Notifications for Custom Resource Changes
To effectively monitor changes to custom resources in Kubernetes, one can implement an event-driven architecture. This involves using Kubernetes’ built-in mechanisms for watching resources and triggering actions when changes occur. The process typically involves the following steps:
- Define the Custom Resource: Create a CRD that outlines the desired specifications for your custom resource.
- Implement a Controller: Develop a controller that watches for changes to instances of the custom resource. The controller can be written in various programming languages, often using client libraries provided by Kubernetes.
- Use Informers: Informers are a high-level abstraction that help manage the lifecycle of Kubernetes resources, providing caching and event handling capabilities. They can be used to listen for changes and trigger notifications.
- Integrate Notification Mechanism: Choose a notification mechanism based on your requirements. This could be a webhook, messaging queue, or direct integration with monitoring tools.
Example of a Custom Resource Notification Workflow
The following table illustrates a simplified workflow for notifying stakeholders when a custom resource changes.
Step | Description |
---|---|
1 | A user updates a custom resource instance. |
2 | The controller detects the change via the informer. |
3 | The controller processes the change, determining the required action. |
4 | A notification is sent to the designated endpoint (e.g., webhook or message queue). |
5 | Stakeholders are alerted about the change through their preferred notification channels. |
Best Practices for Monitoring Custom Resources
Implementing effective monitoring for custom resource changes requires adherence to best practices, which include:
- Decouple Notifications: Use a dedicated service for sending notifications to ensure that the main application logic remains clean and maintainable.
- Rate Limiting: Implement rate limiting on notifications to prevent overwhelming stakeholders with too many alerts in a short time.
- Logging and Auditing: Maintain logs of changes to custom resources, including who made the change and the time it occurred. This provides an audit trail for compliance and troubleshooting.
- Testing: Rigorously test the controller and notification mechanisms to ensure they work as intended under various scenarios, including failure cases.
By following these guidelines, you can create a robust system for notifying stakeholders of changes to Kubernetes custom resources, enhancing operational awareness and response capabilities.
Understanding Custom Resource Definitions (CRDs)
Custom Resource Definitions (CRDs) enable users to extend Kubernetes capabilities by defining new resource types. These resources can be managed just like built-in Kubernetes resources, allowing for enhanced flexibility and functionality in cluster management.
- Definition: A CRD is a way to define a custom resource in Kubernetes, which can be manipulated through standard Kubernetes APIs.
- Use Cases: CRDs are commonly used for:
- Managing application configurations
- Integrating with external systems
- Implementing custom controllers for specific operational needs
Monitoring Changes to Custom Resources
To effectively notify when a custom resource changes, one must monitor the Kubernetes API for events related to that resource. This can be achieved through several methods:
- Kubernetes Watch API: The Watch API allows you to subscribe to changes in resources. You can set up a watch on a specific CRD to receive notifications about create, update, and delete events.
- Client Libraries: Utilize client libraries (like client-go for Go, or kubernetes-client for Python) to establish a watch on CRDs and handle events programmatically.
- Event Exporters: Tools like Kubernetes Event Exporter can be configured to emit events based on changes to CRDs.
Implementing Notifications
Implementing notifications for changes in custom resources can be accomplished through various approaches, depending on the desired integration and complexity.
- Webhook Notifications:
- Create a webhook that receives HTTP POST requests when the resource changes.
- Configure the custom controller to trigger the webhook on resource updates.
- Message Queues:
- Utilize message brokers (e.g., Kafka, RabbitMQ) to publish change events.
- Set up consumers to process these events and trigger further actions.
- Email or Messaging Alerts:
- Integrate with notification services (e.g., Slack, email) to send alerts when changes occur.
- Use tools like Prometheus with Alertmanager for advanced alerting capabilities based on metrics.
Example Code Snippet for Watching CRD Changes
Below is an example of how to watch for changes in a custom resource using the Go client library:
“`go
package main
import (
“context”
“fmt”
“time”
metav1 “k8s.io/apimachinery/pkg/apis/meta/v1”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/clientcmd”
“k8s.io/client-go/tools/clientcmd/api”
“k8s.io/apimachinery/pkg/watch”
)
func main() {
config, err := clientcmd.BuildConfigFromFlags(“”, “path/to/kubeconfig”)
if err != nil {
panic(err)
}
clientset, err := kubernetes.NewForConfig(config)
if err != nil {
panic(err)
}
watchInterface, err := clientset.RESTClient().
Get().
Resource(“mycustomresources”).
Namespace(“default”).
Watch(context.TODO())
if err != nil {
panic(err)
}
for event := range watchInterface.ResultChan() {
switch event.Type {
case watch.Added:
fmt.Println(“Custom resource added:”, event.Object)
case watch.Modified:
fmt.Println(“Custom resource modified:”, event.Object)
case watch.Deleted:
fmt.Println(“Custom resource deleted:”, event.Object)
}
}
}
“`
Best Practices for Managing Notifications
To ensure a robust notification system for changes in custom resources, adhere to the following best practices:
- Rate Limiting: Implement rate limiting to avoid overwhelming your notification system with excessive events.
- Error Handling: Include comprehensive error handling for network requests and message delivery failures.
- Logging: Maintain logs of changes for audit purposes and troubleshooting.
- Testing: Regularly test your notification system to confirm it responds correctly to various changes.
Effective monitoring and notification of changes in Kubernetes custom resources enhance operational awareness and enable proactive management of applications and infrastructure. By leveraging available tools and methods, teams can ensure they are promptly informed of relevant changes, streamlining their workflows and improving responsiveness.
Expert Insights on Monitoring Changes in Kubernetes Custom Resources
Dr. Emily Chen (Kubernetes Architect, Cloud Innovations Inc.). “Implementing a notification system for changes in custom resources is crucial for maintaining the integrity and performance of Kubernetes clusters. Leveraging tools like Kubernetes’ built-in watch functionality can provide real-time updates, ensuring that developers can respond promptly to configuration changes.”
Michael Thompson (DevOps Engineer, Agile Solutions). “To effectively notify teams of changes in custom resources, integrating a CI/CD pipeline with monitoring tools such as Prometheus or Grafana can be beneficial. This approach not only enhances visibility but also automates alerts, reducing the likelihood of human error during deployments.”
Laura Patel (Cloud Native Consultant, TechSphere). “Utilizing Kubernetes’ admission controllers alongside custom resource definitions (CRDs) can significantly streamline change notifications. By enforcing policies at the admission stage, teams can ensure that only authorized changes trigger alerts, thereby enhancing security and operational efficiency.”
Frequently Asked Questions (FAQs)
What is a custom resource in Kubernetes?
A custom resource in Kubernetes is an extension of the Kubernetes API that allows users to define their own resource types. This enables the management of application-specific configurations and behaviors that are not covered by the default Kubernetes resources.
How can I notify when a custom resource changes in Kubernetes?
To notify when a custom resource changes, you can use Kubernetes’ built-in watch functionality, which allows you to listen for changes to specific resources. Additionally, you can implement a controller or operator that reacts to these changes and triggers notifications through various channels, such as webhooks or messaging systems.
What tools can I use to monitor changes in custom resources?
Tools such as Kubernetes client libraries (e.g., client-go for Go, kubernetes-client for Python) can be used to monitor changes. Additionally, operators built with frameworks like Operator SDK or KubeBuilder can facilitate monitoring and handling of custom resource events.
Can I use Kubernetes events to track changes in custom resources?
Yes, Kubernetes events can be used to track changes in custom resources. Events provide insights into the lifecycle of resources and can be leveraged to trigger notifications or logging mechanisms whenever a relevant change occurs.
Is it possible to integrate custom resource change notifications with external systems?
Yes, it is possible to integrate custom resource change notifications with external systems. You can set up webhooks, use message brokers like Kafka or RabbitMQ, or leverage cloud-native tools like Argo Events to connect Kubernetes events to external applications or services.
What are the best practices for handling notifications on custom resource changes?
Best practices include implementing robust error handling and retries for notifications, ensuring idempotency in notification processing, and utilizing structured logging for monitoring. Additionally, consider using backoff strategies to manage load during high-frequency change events.
In summary, notifying when a custom resource in Kubernetes changes is a critical aspect of managing Kubernetes clusters effectively. Custom resources allow users to extend Kubernetes capabilities, enabling the creation of tailored applications and services. Monitoring changes to these resources is essential for maintaining the desired state of applications, ensuring that any modifications are tracked and responded to appropriately. Various methods, such as using Kubernetes event notifications, webhooks, and operators, can facilitate this monitoring process.
Key takeaways from the discussion include the importance of leveraging Kubernetes’ built-in mechanisms, such as the watch API, to listen for changes to custom resources. This approach allows for real-time notifications and can trigger automated responses to changes, enhancing operational efficiency. Additionally, implementing tools like Prometheus or custom controllers can provide further insights and monitoring capabilities, ensuring that teams can quickly react to any alterations in the state of their resources.
Ultimately, establishing a robust notification system for changes in custom resources not only improves the reliability of Kubernetes applications but also fosters a proactive approach to resource management. By integrating these practices, organizations can enhance their operational workflows, reduce downtime, and ensure that their Kubernetes environments remain in sync with their business objectives.
Author Profile
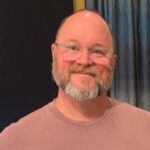
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?