Why Does Node Throw ‘Cannot Use Import Statement Outside A Module’ Error?
In the ever-evolving landscape of JavaScript development, the transition from traditional CommonJS modules to the modern ES6 module syntax has sparked both excitement and confusion among developers. One common error that many encounter during this shift is the perplexing message: “Node cannot use import statement outside a module.” This seemingly cryptic notification can halt progress and leave developers scratching their heads, unsure of how to navigate the intricacies of module systems in Node.js. Understanding the root causes of this issue is essential for anyone looking to harness the full potential of JavaScript’s modular capabilities.
At its core, the error arises from the way Node.js handles different types of modules. While CommonJS has been the standard for years, the of ES6 modules has brought about new conventions and requirements that developers must adhere to. This article delves into the nuances of module systems, exploring why this error occurs and how to effectively resolve it. By unpacking the underlying principles of module usage in Node.js, we aim to empower developers with the knowledge needed to streamline their workflows and embrace modern JavaScript practices.
As we journey through the intricacies of this topic, we will examine the configuration settings, file extensions, and other critical elements that influence module behavior in Node.js. Whether you’re a seasoned
Understanding the Module System in Node.js
Node.js uses the CommonJS module system by default, which allows developers to manage dependencies and share code across files using `require()`. However, the of ES6 modules brought a new syntax using `import` and `export`, leading to some confusion, especially when trying to integrate both systems in a Node.js environment.
When you encounter the error message “Cannot use import statement outside a module,” it typically indicates that Node.js is not recognizing the file as an ES module. This can occur for several reasons, including:
- The file extension is not `.mjs` or `.js` with the correct configuration.
- The `type` field in `package.json` is not set to `”module”`.
- The code is being run in an environment that does not support ES modules.
To resolve this issue, you need to ensure that your Node.js environment is configured correctly to use ES modules.
Configuring Node.js for ES Modules
To enable ES module syntax in Node.js, follow these steps:
- Set the `type` field in `package.json`:
Add `”type”: “module”` to your `package.json` file. This instructs Node.js to treat `.js` files as ES modules.
“`json
{
“name”: “your-project”,
“version”: “1.0.0”,
“type”: “module”,
…
}
“`
- Use the correct file extension:
You can also save your files with a `.mjs` extension, which Node.js will automatically recognize as an ES module, regardless of the `type` field in `package.json`.
- Run your scripts with the proper command:
Ensure you are using a compatible version of Node.js that supports ES modules, typically version 12.x or later. Use the command line to run your script:
“`bash
node your-script.js
“`
Common Scenarios and Solutions
When developing with ES modules in Node.js, it is essential to understand common scenarios that may cause issues.
Scenario | Solution |
---|---|
Using `import` in a `.js` file | Ensure `type` is set to `module` in `package.json`. |
Using `import` in a `.mjs` file | Simply ensure the file is saved with the `.mjs` extension. |
Mixing `require()` and `import` | Avoid mixing module systems; choose one and refactor your code. |
Running code in a Node.js version < 12.x | Upgrade Node.js to at least version 12.x or later. |
Best Practices for ES Modules in Node.js
To work effectively with ES modules in Node.js, consider the following best practices:
- Consistent use of imports: Stick to either `import` or `require` throughout your codebase to maintain clarity and avoid confusion.
- Refactoring: If migrating from CommonJS to ES modules, refactor your imports and exports systematically to ensure all dependencies are updated.
- Testing: Always test your modules in a controlled environment to catch any issues with module resolution or runtime errors.
- Documentation: Keep your project documentation updated to reflect the use of ES modules, which will help onboarding new developers or collaborators.
By following these guidelines and understanding the module system in Node.js, you can effectively manage your project’s dependencies and leverage the benefits of ES modules.
Understanding the Error
The error message “Cannot use import statement outside a module” in Node.js typically occurs when the JavaScript runtime encounters an `import` statement in a context where it is not recognized. This is primarily due to the module system configuration in your environment. Node.js supports two module systems: CommonJS (CJS) and ECMAScript Modules (ESM).
Key Points:
- CommonJS (CJS): This is the default module system in Node.js, using `require()` and `module.exports`.
- ECMAScript Modules (ESM): This uses `import` and `export` syntax and is supported in Node.js starting from version 12 with the `–experimental-modules` flag, and natively in later versions.
Causes of the Error
The error can arise from several scenarios:
- File Extension: Using `.js` files by default assumes CommonJS unless specified otherwise.
- Package Configuration: The `package.json` file may not indicate the intended module system.
- Node Version: The version of Node.js being used may not support ESM.
How to Resolve the Error
To resolve this error, consider the following solutions:
1. Use `.mjs` Extension
- Rename your file from `.js` to `.mjs`. Node.js will treat `.mjs` files as ESM.
2. Update `package.json`
- Set the module type in your `package.json`:
“`json
{
“type”: “module”
}
“`
3. Ensure Node.js Compatibility
- Verify that you are running a version of Node.js that supports ES modules (v12.0.0 and above).
4. Use Babel or TypeScript
- If you need to maintain compatibility with CommonJS or older Node.js versions, consider using Babel or TypeScript to transpile your code.
Examples
Here are examples to illustrate the solutions:
Using `.mjs` Extension:
“`javascript
// file: example.mjs
import { myFunction } from ‘./myModule.mjs’;
myFunction();
“`
Configuring `package.json`:
“`json
{
“name”: “my-app”,
“version”: “1.0.0”,
“type”: “module”,
“main”: “index.js”
}
“`
Babel Configuration:
“`json
// .babelrc
{
“presets”: [“@babel/preset-env”]
}
“`
Common Use Cases
Here are scenarios where the error may frequently occur:
Scenario | Possible Solution |
---|---|
Using `import` in a `.js` file | Rename to `.mjs` or set `”type”: “module”` in `package.json` |
Mixing `import` and `require()` | Stick to one module system throughout your project |
Running an old Node.js version | Upgrade to a newer version that supports ESM |
Best Practices
To avoid the “Cannot use import statement outside a module” error, consider the following best practices:
- Consistently Use One Module System: Choose either ESM or CommonJS and stick with it throughout your project.
- Upgrade Node.js Regularly: Ensure your Node.js version supports the latest features and module systems.
- Utilize Linting Tools: Implement linting tools to catch module-related issues early in the development process.
Understanding the “Node Cannot Use Import Statement Outside A Module” Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘Node Cannot Use Import Statement Outside A Module’ typically arises when developers attempt to use ES6 module syntax in a Node.js environment that is not configured to support it. It is crucial to ensure that the ‘type’ field in the package.json file is set to ‘module’ or to use CommonJS syntax with ‘require’ for compatibility.”
Mark Thompson (JavaScript Framework Specialist, CodeMaster Academy). “Many developers transitioning from front-end frameworks to Node.js encounter this error. It highlights the importance of understanding the module system in Node. To resolve this, one can either convert the file to a module by adding ‘.mjs’ extension or adjust the Node.js runtime settings to accept ES modules.”
Linda Zhang (Technical Writer, Node.js Foundation). “This error serves as a reminder of the evolving nature of JavaScript and Node.js. As ES modules become more prevalent, developers should familiarize themselves with both the syntax and the configuration necessary to avoid such issues, ensuring a smoother development experience.”
Frequently Asked Questions (FAQs)
What does the error “Cannot use import statement outside a module” mean?
This error indicates that the JavaScript file is not recognized as a module by Node.js, preventing the use of the `import` statement. By default, Node.js treats files as CommonJS modules unless specified otherwise.
How can I resolve the “Cannot use import statement outside a module” error?
To resolve this error, you can either change the file extension to `.mjs`, set `”type”: “module”` in your `package.json`, or use CommonJS syntax with `require()` instead of `import`.
What is the difference between CommonJS and ES Modules in Node.js?
CommonJS uses `require()` and `module.exports` for module management, while ES Modules utilize `import` and `export` syntax. ES Modules are designed for modern JavaScript and support static analysis, which allows for better optimization.
Can I use the `import` statement in a Node.js environment without any configuration?
No, you cannot use the `import` statement in a Node.js environment without configuration. You must either rename your file or modify the `package.json` to indicate that you are using ES Modules.
What are the implications of setting “type”: “module” in package.json?
Setting `”type”: “module”` in `package.json` allows you to use ES Module syntax throughout your project. However, it also means that all files will be treated as modules, which may affect existing CommonJS code.
Are there any performance differences between using CommonJS and ES Modules?
Yes, ES Modules can offer better performance in certain scenarios due to their static structure, which allows for tree-shaking and more efficient bundling. However, the actual performance difference may vary based on the specific use case and environment.
The error message “Node cannot use import statement outside a module” typically arises when attempting to use ES6 module syntax in a Node.js environment that does not recognize the file as a module. This issue often occurs when the JavaScript file is not configured to be treated as an ES module, which can lead to confusion for developers transitioning from browser-based JavaScript to server-side Node.js applications.
To resolve this error, developers should ensure that their Node.js environment is set up to support ES modules. This can be achieved by either using the `.mjs` file extension for JavaScript files or by including the `”type”: “module”` field in the `package.json` file. These configurations inform Node.js to treat the specified files as ES modules, thereby allowing the use of the `import` statement without encountering errors.
It is also essential to consider the version of Node.js being used, as support for ES modules has evolved over time. Developers should ensure they are using a version of Node.js that fully supports ES module syntax to avoid compatibility issues. Furthermore, understanding the differences between CommonJS and ES module syntax can help developers make informed decisions about module usage in their applications.
In summary, the “Node cannot use
Author Profile
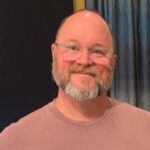
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?