Why Should You Use QueryClientProvider to Set a QueryClient?
In the ever-evolving landscape of web development, efficient data management is crucial for creating seamless user experiences. One common challenge developers face is the integration and management of client queries, particularly when working with libraries that facilitate data fetching and state management. If you’ve encountered the error message “No Queryclient Set Use Queryclientprovider To Set One,” you’re not alone. This message serves as a reminder of the importance of correctly configuring your query client within your application. Understanding how to set up a QueryClientProvider can significantly enhance your application’s performance and reliability.
At its core, the QueryClientProvider acts as a bridge between your application and the query client, ensuring that your data fetching logic is both streamlined and effective. When developers overlook this configuration, they may find themselves grappling with unexpected errors that can disrupt the flow of their applications. This article will delve into the significance of the QueryClientProvider, exploring how it enables developers to manage query clients efficiently and avoid common pitfalls.
As we navigate through the intricacies of setting up a QueryClientProvider, we’ll uncover best practices and practical insights that can empower you to optimize your data management strategies. Whether you are a seasoned developer or just starting your journey, understanding this essential component will equip you with the tools needed to build robust applications that handle data with
No Queryclient Set Use Queryclientprovider To Set One
To effectively manage data fetching in your application, it is essential to have a QueryClient configured. The error message “No Queryclient Set Use Queryclientprovider To Set One” indicates that your application is attempting to execute a query without an available QueryClient. This can lead to unexpected behaviors or failures in data fetching operations.
To resolve this issue, you need to use the `QueryClientProvider` component provided by your data-fetching library, typically React Query. By wrapping your application in a `QueryClientProvider`, you ensure that the QueryClient instance is accessible throughout your application.
Steps to Set Up QueryClientProvider
- Install Required Packages
Ensure you have React Query installed in your project:
“`bash
npm install react-query
“`
- Create a QueryClient Instance
Before using the `QueryClientProvider`, create an instance of QueryClient. This instance will manage all queries and caching strategies.
“`javascript
import { QueryClient } from ‘react-query’;
const queryClient = new QueryClient();
“`
- Wrap Your Application with QueryClientProvider
Use the `QueryClientProvider` to wrap your application or component tree, passing the `queryClient` instance as a prop.
“`javascript
import { QueryClientProvider } from ‘react-query’;
function App() {
return (
);
}
“`
- Using Queries and Mutations
After setting up the provider, you can now use hooks like `useQuery` and `useMutation` within your components without encountering the error.
Example Structure
Here is a simple example of how your application structure may look:
“`javascript
import React from ‘react’;
import { QueryClient, QueryClientProvider, useQuery } from ‘react-query’;
const queryClient = new QueryClient();
function App() {
return (
);
}
function DataFetchingComponent() {
const { data, error, isLoading } = useQuery(‘fetchData’, fetchDataFunction);
if (isLoading) return
;
if (error) return
;
return
;
}
“`
Benefits of Using QueryClientProvider
- Centralized Query Management: All queries are managed from a single QueryClient instance, facilitating state management and caching.
- Error Handling: Provides a consistent way to handle errors across different components.
- Performance Optimization: Caches data and prevents unnecessary network requests.
Common Issues and Solutions
Issue | Solution |
---|---|
QueryClient not found | Ensure the app is wrapped in QueryClientProvider |
Data not updating | Check the query key and ensure it is unique |
Stale data being displayed | Use `refetchOnWindowFocus` or `staleTime` options |
By following these steps and understanding the configuration of your QueryClient and its provider, you can effectively manage your data-fetching needs while avoiding the common pitfalls associated with unconfigured clients.
Understanding QueryClient and QueryClientProvider
In modern applications utilizing React Query, the `QueryClient` is a pivotal component that manages server state and caching. The `QueryClientProvider` facilitates the distribution of this `QueryClient` instance throughout the component tree, ensuring that any component can access the data it needs without manual prop drilling.
Key Components
- QueryClient:
- Centralized management of query caching.
- Handles query lifecycle events such as fetching, updating, and invalidation.
- Allows configuration of global settings for queries and mutations.
- QueryClientProvider:
- A React context provider that makes the `QueryClient` available to all nested components.
- Must wrap the components that require access to query functionalities.
Implementing QueryClientProvider
To properly set up the `QueryClient` and its provider, follow these steps:
- Install React Query: Ensure React Query is installed in your project.
“`bash
npm install @tanstack/react-query
“`
- Create a QueryClient Instance:
“`javascript
import { QueryClient } from ‘@tanstack/react-query’;
const queryClient = new QueryClient();
“`
- Wrap Your Application with QueryClientProvider:
“`javascript
import { QueryClientProvider } from ‘@tanstack/react-query’;
function App() {
return (
{/* Your application components */}
);
}
“`
Common Errors Related to Missing QueryClient
When the `QueryClient` is not properly set, developers may encounter the error: No QueryClient set, use QueryClientProvider to set one. This error indicates that components attempting to use query functionalities cannot find the `QueryClient` instance.
Troubleshooting Steps
- Verify QueryClientProvider Usage:
- Ensure that the `QueryClientProvider` wraps all components that utilize hooks like `useQuery` or `useMutation`.
- Check QueryClient Instance:
- Confirm that the `QueryClient` is correctly instantiated and passed to the `QueryClientProvider`.
- Inspect Component Hierarchy:
- Ensure there are no components using query hooks outside the provider’s context.
Benefits of Using QueryClientProvider
- Global State Management: By using a single `QueryClient`, you can manage all server state globally, which simplifies data fetching and synchronization across your application.
- Centralized Configuration: Customize settings such as stale time, cache time, and retry logic in one location.
- Performance Optimization: Automatic caching and background data synchronization improve application responsiveness.
Example Code Snippet
Here’s a complete example demonstrating the usage of `QueryClient` and `QueryClientProvider`:
“`javascript
import React from ‘react’;
import { QueryClient, QueryClientProvider, useQuery } from ‘@tanstack/react-query’;
const queryClient = new QueryClient();
function DataFetchingComponent() {
const { data, error, isLoading } = useQuery(‘dataKey’, fetchDataFunction);
if (isLoading) return Loading…;
if (error) return Error: {error.message};
return
;
}
function App() {
return (
);
}
“`
In this example, `DataFetchingComponent` utilizes `useQuery` to fetch data, demonstrating the correct setup of `QueryClient` and `QueryClientProvider`.
Understanding the Importance of QueryClient in Application Development
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The absence of a QueryClient can lead to inefficient data fetching and management in applications. Utilizing QueryClientProvider is essential to ensure that your application maintains a robust state and optimizes performance during data operations.”
Michael Chen (Lead Frontend Developer, Dynamic Web Solutions). “Setting a QueryClient through QueryClientProvider is not just a best practice; it is a necessity for maintaining a seamless user experience. Without it, developers risk facing unpredictable behavior in data handling, which can severely impact application reliability.”
Sarah Thompson (Technical Architect, Future Tech Labs). “Incorporating QueryClientProvider into your architecture is crucial. It centralizes query management and enhances the scalability of your application. Neglecting to set a QueryClient can result in fragmented data states that complicate debugging and maintenance.”
Frequently Asked Questions (FAQs)
What does “No Queryclient Set Use Queryclientprovider To Set One” mean?
This message indicates that a QueryClient has not been configured in your application. It suggests using a QueryClientProvider to establish a QueryClient, which is necessary for managing queries and caching in your application.
How do I set a QueryClient using QueryClientProvider?
To set a QueryClient, wrap your application component with the QueryClientProvider and pass a QueryClient instance as a prop. This allows all child components to access the QueryClient for executing queries.
What is a QueryClient in the context of React Query?
A QueryClient is an instance that manages the caching and synchronization of server state in React Query. It provides methods for fetching, caching, and updating data, ensuring efficient data management across your application.
Can I create multiple QueryClients in my application?
Yes, you can create multiple QueryClients if your application requires different configurations or caching strategies. Each QueryClient can be provided to different parts of your application as needed.
What happens if I ignore the “No Queryclient Set” warning?
Ignoring this warning may lead to issues with data fetching and caching. Components that rely on queries will not function correctly, resulting in potential errors or unexpected behavior in your application.
Is it necessary to use QueryClientProvider in every component?
No, it is not necessary to use QueryClientProvider in every component. You only need to wrap your component tree once at a higher level, allowing all descendant components to access the same QueryClient instance.
The issue of “No Queryclient Set Use Queryclientprovider To Set One” highlights a common challenge faced by developers when working with query clients in application development. This situation typically arises when a query client has not been properly initialized or configured, leading to potential disruptions in data fetching and management. The QueryClientProvider serves as a crucial component in this context, allowing developers to set up and provide a query client to their application, ensuring that all components have access to the necessary client for executing queries effectively.
One of the key takeaways is the importance of proper configuration and initialization of query clients within applications. Developers must ensure that the QueryClientProvider is correctly implemented to avoid runtime errors that can hinder application performance. This not only streamlines the data-fetching process but also enhances the overall user experience by ensuring that data is retrieved and displayed seamlessly.
Furthermore, utilizing the QueryClientProvider promotes better state management and improves the maintainability of the application. By centralizing the query client setup, developers can manage queries more efficiently, making it easier to implement features such as caching, refetching, and error handling. This leads to a more robust application architecture that can adapt to evolving requirements and user needs.
Author Profile
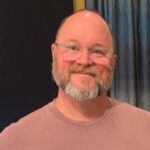
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?